一、用video.js实现视频播放
1、安装video.js插件
// 安装video.js插件
npm install video.js -S
// 如果需要播放rtmp直播流,需安装一下插件
npm install videojs-flash -S
2、在组件代码里使用
<template>
<div data-vjs-player>
<video ref="videoNode" class="video-js vjs-default-skin">
抱歉,您的浏览器不支持
</video>
</div>
</template>
引入videojs插件
import videojs from "video.js";
//播放器中文,不能使用.js文件
import videozhCN from "video.js/dist/lang/zh-CN.json";
//样式文件注意要加上
import "video.js/dist/video-js.css";
//如果要播放RTMP要使用flash 需要先npm i videojs-flash
import "videojs-flash";
export default {
data() {
return {
player: null,
controls: true
};
},
//初始化播放器
mounted(){
let options = {
autoplay: true, // 自动播放
language: "zh-CN",
controls: this.controls, // 控制条
preload: "auto", // 自动加载
errorDisplay: true, // 错误展示
// fluid: true, // 跟随外层容器变化大小,跟随的是外层宽度
width: "500px",
height: "500px",
// controlBar: false, // 设为false不渲染控制条DOM元素,只设置controls为false虽然不展示,但是存在
// textTrackDisplay: false, // 不渲染字幕相关DOM
userActions: {
hotkeys: true // 是否支持热键
},
notSupportedMessage: "此视频暂无法播放,请稍后再试",
techOrder: ["h5","flash"], // 定义Video.js技术首选的顺序
sources: [
{
src: './video/xxx.mp4', // 视频或者直播地址
type: 'video/mp4',
//type: 'rtmp/flv',
}
]
};
this.player = videojs(
this.$refs.videoNode,
options,
function onPlayerReady() {
videojs.log(`Your player is ready!`);
}
);
videojs.addLanguage("zh-CN", videozhCN);
},
beforeDestroy() {
if (this.player) {
this.player.dispose();
}
}
}
注意:controls 如果不是true的话,不会显示播放按钮
3、播放按钮居中
播放按钮默认在左上角,是作者认为会遮挡内容考虑的,不过这个是可以根据参数修改的,只需要给video标签加一个class(vjs-big-play-centered)就可以了。
<video ref="videoNode" class="video-js vjs-default-skin vjs-big-play-centered"></video>
二、用videojs-markers实现视频进度条打点
要实现的功能是视频的进度条上面有一些小点,然后鼠标放上去会出现一些文字提示。
1、安装videojs-markers
npm i videojs-markers -S
2、引入videojs-markers
// 引入videojs-markers
import "videojs-markers";
//引入样式
import "videojs-markers/dist/videojs.markers.css";
3、使用videojs-markers
this.player.markers({
markerStyle: {
// 标记点样式
width: "0.7em",
height: "0.7em",
bottom: "-0.20em",
"border-radius": "50%",
"background-color": "orange",
position: "absolute"
},
//鼠标移入标记点的提示
markerTip: {
display: true, // 是否显示
/*
用于动态构建标记提示文本的回调函数。
只需返回一个字符串,参数标记是传递给插件的标记对象
*/
text: function(marker) {
return marker.text;
}
},
markers: [
{
time: 20,
text:'点位一',
class: 'red'
},
{
time: 40,
text:'点位二',
class: 'green'
},
{
time: 130,
text:'点位三',
class: 'yellow'
},
{
time: 200,
text:'点位四'
}
],
});
注意:markerStyle配置的是各个打点的默认颜色,如果要每个打点都有自己各自的颜色,可以在markers里给每个打点添加class名字,然后在css里单独设置颜色。
三、video.js 配置项
1、常用配置选项
- autoplay:true/false 播放器准备好之后,是否自动播放 【默认false】
- controls:true/false 是否拥有控制条 【默认true】,如果设为false,界面上不会出现任何控制按钮,启动视频播放的唯一方法是使用autoplay属性或通过Player API
- height: 视频容器的高度,字符串或数字(以像素为单位),比如: height: 300 或者 height: '300px'
- width: 视频容器的宽度, 字符串或数字(以像素为单位)
- loop : true/false 视频播放结束后,是否循环播放
- muted : true/false 是否静音
- poster: 播放前显示的视频画面,播放开始之后自动移除。通常传入一个URL
- preload: 预加载
- 'auto':立即开始加载视频(如果浏览器支持)。某些移动设备不会预加载视频,以保护用户的带宽/数据使用。
- 'metadata':仅加载视频的元数据,其中包括视频的持续时间和尺寸等信息。有时,元数据将通过下载几帧视频来加载。
- 'none':不要预加载任何数据。浏览器将等待用户点击“播放”开始下载。
- children: Array | Object 可选子组件 从基础的Component组件继承而来的子组件,数组中的顺序将影响组件的创建顺序
2、特定的配置选项
除非另有说明,否则默认情况下每个选项undefined。
aspectRatio
类型: string
将播放器置于流体模式,并在计算播放器的动态大小时使用该值。该值应表示比率 - 由冒号(例如"16:9"或"4:3")分隔的两个数字。
autoSetup
类型: boolean
阻止播放器为具有data-setup属性的媒体元素运行autoSetup 。
注意:必须在与videojs.options.autoSetup = falsevideojs源加载生效的同一时刻全局设置。
children
类型: Array|Object
此选项继承自基Component类。
fluid
类型: boolean
何时true,Video.js播放器将具有流畅的大小。换句话说,它将扩展以适应其容器。
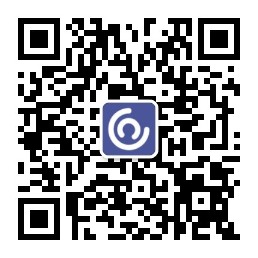
此外,如果元素具有"vjs-fluid",则此选项自动设置为true。
inactivityTimeout
类型: number
Video.js表示用户通过"vjs-user-active"和"vjs-user-inactive"类以及"useractive"事件与玩家进行交互。
在inactivityTimeout决定了不活动的许多毫秒声明用户闲置之前是必需的。值为0表示没有inactivityTimeout,用户永远不会被视为非活动状态。
language
键入:string,默认值:浏览器默认值或’en’
与播放器中的一种可用语言匹配的语言代码。这为播放器设置了初始语言,但始终可以更改。
languages
类型: Object
自定义播放器中可用的语言。此对象的键将是语言代码,值将是具有英语键和翻译值的对象。
注意:通常,不需要此选项,最好将自定义语言传递给videojs.addLanguage()
nativeControlsForTouch
类型: boolean
明确设置关联技术选项的默认值。
notSupportedMessage
类型: string
允许覆盖Video.js无法播放媒体源时显示的默认消息。
playbackRates
类型: Array
严格大于0的数字数组,其中1表示常速(100%),0.5表示半速(50%),2表示双速(200%)等。如果指定,Video.js显示控件(类vjs-playback-rate)允许用户从选择数组中选择播放速度。选项以从下到上的指定顺序显示。
videojs('my-player', {
playbackRates: [0.5, 1, 1.5, 2]
});
plugins
类型: Object
这支持在初始化播放器时使用自定义选项自动初始化插件 - 而不是要求您手动初始化它们。
videojs('my-player', {
plugins: {
foo: {bar: true},
boo: {baz: false}
}
});
以上大致相当于:
var player = videojs('my-player');
player.foo({bar: true});
player.boo({baz: false});
虽然,由于plugins选项是对象,因此无法保证初始化顺序!
sources
类型: Array
一组对象,它们反映了本机元素具有一系列子元素的能力。这应该是带有src和type属性的对象数组。
videojs('my-player', {
sources: [{
src: './video/video.mp4',
type: 'video/mp4'
}, {
src: './video/video.webm',
type: 'video/webm'
}]
});
使用元素将具有相同的效果:
<video ...>
<source src="./video/video.mp4" type="video/mp4">
<source src="./video/video.webm" type="video/webm">
</video>
techCanOverridePoster
类型: boolean
使技术人员有可能覆盖玩家的海报并融入玩家的海报生命周期。当使用多个技术时,这可能很有用,每个技术都必须在播放新源时设置自己的海报。
techOrder
输入:Array,默认值:[‘html5’]
定义Video.js技术首选的顺序。默认情况下,这意味着Html5首选技术。其他注册的技术将在此技术之后按其注册顺序添加。
vtt.js
类型: string
允许覆盖vtt.js的默认URL,该URL可以异步加载到polyfill支持WebVTT。
此选项将用于Video.js(即video.novtt.js)的“novtt”版本中。否则,vtt.js与Video.js捆绑在一起。
3、组件选项
children
类型: Array|Object
如果Array- 这是默认值 - 这用于确定哪些子节点(按组件名称)以及在播放器(或其他组件)上创建它们的顺序
// The following code creates a player with ONLY bigPlayButton and
// controlBar child components.
videojs('my-player', {
children: [
'bigPlayButton',
'controlBar'
]
});
该children选项还可以作为传递Object。在这种情况下,它用于提供options任何/所有孩子,包括禁用它们false
// This player's ONLY child will be the controlBar. Clearly, this is not the
// ideal method for disabling a grandchild!
videojs('my-player', {
children: {
controlBar: {
fullscreenToggle: false
}
}
});
${componentName}
类型: Object
可以通过组件名称的低驼峰案例变体(例如controlBarfor ControlBar)为组件提供自定义选项。这些可以嵌套在孙子关系的表示中。
例如,要禁用全屏控件
videojs('my-player', {
controlBar: {
fullscreenToggle: false
}
});
${techName}
类型: Object
Video.js回放技术(即“技术”)可以作为传递给该videojs功能的选项的一部分给予自定义选项。它们应该在技术名称的小写变体下传递(例如"flash"或"html5")。
flash
swf
指定Video.js SWF文件在Flash技术位置的位置
videojs('my-player', {
flash: {
swf: '//path/to/videojs.swf'
}
});
但是,更改全局默认值通常更合适:
videojs.options.flash.swf = ‘//path/to/videojs.swf’
nativeControlsForTouch
类型: boolean
只有技术支持Html5,此选项可以设置true为强制触摸设备的本机控件。
nativeAudioTracks
类型: boolean
可以设置为false禁用本机音轨支持。最常用于videojs-contrib-hls。
nativeTextTracks
类型: boolean
可以设置为false强制模拟文本轨道而不是本机支持。该nativeCaptions选项也存在,但只是一个别名nativeTextTracks。
nativeVideoTracks
类型: boolean
可以设置为false禁用本机视频轨道支持。最常用于videojs-contrib-hls。
四、常用事件
- 播放 this.play()
- 停止 – video没有stop方法,可以用pause 暂停获得同样的效果
- 暂停 this.pause()
- 销毁 this.dispose()
- 监听 this.on(‘click‘,fn)
- 触发事件this.trigger(‘dispose‘)
var options = {};
var player = videojs(‘example_video_1‘, options, function onPlayerReady() {
videojs.log(‘播放器已经准备好了!‘);
// In this context, `this` is the player that was created by Video.js.<br>
// 注意,这个地方的上下文, `this` 指向的是Video.js的实例对像player
this.play();
// How about an event listener?<br> // 如何使用事件监听?
this.on(‘ended‘, function() {
videojs.log(‘播放结束了!‘);
});
});
五、播放器自定义组件
1、在控制栏上添加按钮
var button = this.player.getChild('ControlBar').addChild('button', {
controlText: 'My button',
className: 'vjs-visible-text',
clickHandler: function(event) {
videojs.log('Clicked');
}
});
2、创建用于在播放器顶部显示标题的组件
// Get the Component base class from Video.js
var Component = videojs.getComponent('Component');
// The videojs.extend function is used to assist with inheritance. In
// an ES6 environment, `class TitleBar extends Component` would work
// identically.
var TitleBar = videojs.extend(Component, {
// The constructor of a component receives two arguments: the
// player it will be associated with and an object of options.
constructor: function(player, options) {
// It is important to invoke the superclass before anything else,
// to get all the features of components out of the box!
Component.apply(this, arguments);
// If a `text` option was passed in, update the text content of
// the component.
if (options.text) {
this.updateTextContent(options.text);
}
},
// The `createEl` function of a component creates its DOM element.
createEl: function() {
return videojs.createEl('div', {
// Prefixing classes of elements within a player with "vjs-"
// is a convention used in Video.js.
className: 'vjs-title-bar'
});
},
// This function could be called at any time to update the text
// contents of the component.
updateTextContent: function(text) {
// If no text was provided, default to "Title Unknown"
if (typeof text !== 'string') {
text = 'Title Unknown';
}
// Use Video.js utility DOM methods to manipulate the content
// of the component's element.
videojs.emptyEl(this.el());
videojs.appendContent(this.el(), text);
}
});
// Register the component with Video.js, so it can be used in players.
videojs.registerComponent('TitleBar', TitleBar);
// Create a player.
var player = videojs('my-player');
// Add the TitleBar as a child of the player and provide it some text
// in its options.
player.addChild('TitleBar', {text: 'The Title of The Video!'});
3、添加一个自定义的放大功能
子组件嵌套
const MenuButton = videojs.getComponent('MenuButton')
const Menu = videojs.getComponent('Menu')
const MenuItem = videojs.getComponent('MenuItem')
const items = ['100%', '80%', '50%', 'auto']
const myMenu = new Menu(this.player)
const myMenuItemList = []
for (let i = 0; i < items.length; i++) {
myMenuItemList.push(new MenuItem(this.player, { label: items[i] }))
myMenuItemList[i].on('click', $event => {
this.setShowPercent($event)
})
myMenu.addItem(myMenuItemList[i])
}
this.player.myMenu = myMenu
this.player.myMenuItemList = myMenuItemList
const myMenuButton = new MenuButton(this.player)
myMenuButton.addChild(myMenu)
myMenuButton.controlText('显示比例')
myMenuButton.addClass('my-menu-button')
this.player.myMenuButton = myMenuButton
this.player.controlBar.addChild(myMenuButton)
4、关于自定义按钮的文本不显示的解决方法
其实就是自定义按钮默认是不显示文本的,需要我们手动添加className:vjs-visible-text
this.player = videojs(...)
var Button = videojs.getComponent('Button');
var button = new Button(this.player, {
className: 'vjs-visible-text',
controlText: '按钮文本',
clickHandler: function(event) {
videojs.log('Clicked');
}
});
var button = this.player.getChild('ControlBar').addChild(button)
this.player = videojs(...)
var button = this.player.getChild('ControlBar').addChild('button', {
controlText: 'My button',
className: 'vjs-visible-text',
clickHandler: function(event) {
videojs.log('Clicked');
}
});
this.player = videojs(...)
const MenuButton = videojs.getComponent('MenuButton')
const myMenuButton = new MenuButton(this.player)
myMenuButton.addChild(myMenu)
myMenuButton.controlText('显示比例')
myMenuButton.addClass('my-menu-button')
// 添加显示文本的类名
myMenuButton.children()[0].addClass('vjs-visible-text')
this.player.myMenuButton = myMenuButton
this.player.controlBar.addChild(myMenuButton)