1、MainActivity.java
package com.beibeibeibei.getvscodeversion;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button web_btn = findViewById(R.id.web_btn);
web_btn.setOnClickListener(view -> {
Uri uri = Uri.parse("https://code.visualstudio.com/");
Intent intent = new Intent(Intent.ACTION_VIEW, uri);
startActivity(intent);
});
Button share_btn = findViewById(R.id.share_btn);
share_btn.setOnClickListener(view -> update_link());
Button update_btn = findViewById(R.id.update_btn);
update_btn.setOnClickListener(view -> update_version());
update_version();
}
@SuppressLint("SetTextI18n")
public void update_version() {
TextView main_text = findViewById(R.id.main_text);
runOnUiThread(() -> main_text.setText("正在加载中…"));
new Thread(() -> {
HttpURLConnection conn = null;
BufferedReader br = null;
try {
URL url = new URL("https://code.visualstudio.com/updates");
conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(8000);
conn.setReadTimeout(8000);
InputStream in = conn.getInputStream();
br = new BufferedReader(new InputStreamReader(in));
StringBuilder sb = new StringBuilder();
String s;
while ((s = br.readLine()) != null) {
sb.append(s);
}
String result = sb.toString();
String result2 = GetVersionFromHTML(result);
runOnUiThread(() -> main_text.setText(result2));
} catch (IOException e) {
} finally {
if (conn != null) {
conn.disconnect();
}
if (br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}).start();
}
public String GetVersionFromHTML(String html) {
String search_key = "Update ";
List<Integer> li = new ArrayList<>();
List<String> li2 = new ArrayList<>();
li2.add("0.00.0");
int pos = html.indexOf(search_key);
li.add(pos);
while (pos != -1) {
pos = html.indexOf(search_key, pos + search_key.length());
li.add(pos);
}
for (int i = 0; i < li.size(); i++) {
int p = li.get(i);
if (p != -1) {
String s = html.substring(p, p + 20);
String re = "[^(\\d).]";
String s2 = Pattern.compile(re).matcher(s).replaceAll("").trim();
if (s2.length() != 0) {
li2.add(s2);
}
}
}
String search_key2 = "https://update.code.visualstudio.com/";
pos = html.indexOf(search_key2);
li.add(pos);
while (pos != -1){
pos = html.indexOf(search_key2, pos + search_key2.length());
li.add(pos);
}
for (int i = 0; i < li.size(); i++) {
int p = li.get(i);
if (p != -1) {
String s = html.substring(p + 33, p + 45);
String re = "[^(\\d).]";
String s2 = Pattern.compile(re).matcher(s).replaceAll("").trim();
if (s2.length() != 0) {
li2.add(s2);
}
}
}
Collections.sort(li2, new ComVersion());
if(li2.size() > 0){
return li2.get(0);
}else {
return "0.00.0";
}
}
public void update_link() {
Toast.makeText(getApplicationContext(), "正在获取下载链接", Toast.LENGTH_SHORT).show();
new Thread(() -> {
HttpURLConnection conn = null;
BufferedReader br = null;
try {
URL url = new URL("https://blog.csdn.net/qq_39124701/article/details/129400983");
conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(8000);
conn.setReadTimeout(8000);
InputStream in = conn.getInputStream();
br = new BufferedReader(new InputStreamReader(in));
StringBuilder sb = new StringBuilder();
String s;
while ((s = br.readLine()) != null) {
sb.append(s);
}
String result = sb.toString();
String result2 = GetLinkFromHTML(result);
Intent intent = new Intent();
intent.setAction(Intent.ACTION_SEND);
intent.putExtra(Intent.EXTRA_TEXT, "VSCode下载地址:" + result2);
intent.setType("text/plain");
startActivity(intent);
} catch (IOException e) {
} finally {
if (conn != null) {
conn.disconnect();
}
if (br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}).start();
}
public String GetLinkFromHTML(String HTML) {
String patten = "(?<=>)https://vscode.cdn.azure.cn/stable/[A-Za-z\\d\\-.?/]+(?<!<)";
Pattern r = Pattern.compile(patten);
Matcher m = r.matcher(HTML);
if (m.find()) {
return HTML.substring(m.start(), m.end());
} else {
return "https://vscode.cdn.azure.cn/stable/ee2b180d582a7f601fa6ecfdad8d9fd269ab1884/VSCodeSetup-x64-1.76.2.exe?1";
}
}
}
class ComVersion implements Comparator<String> {
@Override
public int compare(String o1, String o2) {
String[] li1 = o1.split("\\.");
String[] li2 = o2.split("\\.");
int n1 = Integer.parseInt(li1[0]) * 210 + Integer.parseInt(li1[1]) * 2 + Integer.parseInt(li1[2]);
int n2 = Integer.parseInt(li2[0]) * 210 + Integer.parseInt(li2[1]) * 2 + Integer.parseInt(li2[2]);
return -(n1 - n2);
}
}
2、activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<LinearLayout
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="10dp"
android:gravity="center"
android:orientation="vertical"
tools:layout_editor_absoluteX="10dp"
tools:layout_editor_absoluteY="10dp">
<Space
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/vscode_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/text_vscode" />
<TextView
android:id="@+id/main_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/text_loading"
android:textSize="60sp" />
<Space
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/web_btn"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="@string/btn_text_web"
tools:ignore="ButtonStyle" />
<Space
android:layout_width="10dp"
android:layout_height="match_parent" />
<Button
android:id="@+id/share_btn"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="@string/btn_text_share"
tools:ignore="ButtonStyle" />
</LinearLayout>
<Button
android:id="@+id/update_btn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/btn_text_update" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
3、strings.xml
<resources>
<string name="app_name">获取最新VSCode版本</string>
<string name="text_loading">正在加载…</string>
<string name="text_vscode">VSCode的最新版本是</string>
<string name="btn_text_update">刷新</string>
<string name="btn_text_share">VSCode下载地址分享</string>
<string name="btn_text_web">VSCode官网</string>
<string name="img_text_description">图片描述</string>
</resources>
4、AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET"/>
5、ic_launcher-playstore.png
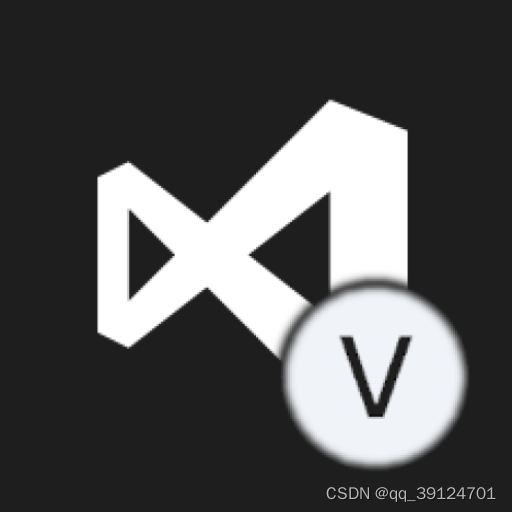