Feign
feign是声明式的web service客户端,它让微服务之间的调用变得更简单了,类似controller调用service。Spring Cloud集成了Ribbon和Eureka,可在使用Feign时提供负载均衡的http客户端。
使用gradle进行引入feign
dependencies {
//feign
implementation('org.springframework.cloud:spring-cloud-starter-openfeign:2.0.2.RELEASE')
//web
implementation('org.springframework.boot:spring-boot-starter-web')
//eureka client
implementation('org.springframework.cloud:spring-cloud-starter-netflix-eureka-client:2.1.0.M1')
//test
testImplementation('org.springframework.boot:spring-boot-starter-test')
}
feign底层是使用了ribbon作为负载均衡的客户端,而ribbon的负载均衡也是依赖于eureka 获得各个服务的地址,所以要引入eureka-client。
SpringbootApplication启动类加上@FeignClient注解,以及@EnableDiscoveryClient。
@SpringBootApplication(exclude = {
DataSourceAutoConfiguration.class, PageHelperAutoConfiguration.class})
@EnableAsync
@EnableTransactionManagement
@EnableEurekaClient
@EnableHystrix
@EnableFeignClients
@Slf4j
public class NftWebApplication {
public static void main(String[] args) {
SpringApplication.run(NftWebApplication.class, args);
log.info("(♥◠‿◠)ノ゙ NftWeb服务启动成功 ლ(´ڡ`ლ)゙ \n");
}
}
yaml配置:
server:
port: 8082
#配置eureka
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka
instance:
status-page-url-path: /info
health-check-url-path: /health
#服务名称
spring:
application:
name: product
profiles:
active: ${
boot.profile:dev}
#feign的配置,连接超时及读取超时配置
feign:
client:
config:
default:
connectTimeout: 5000
readTimeout: 5000
loggerLevel: basic
配置好以后,接下来我们进行feign的使用
@FeignClient(value = "CART")
public interface CartFeignClient {
@PostMapping("/cart/{productId}")
Long addCart(@PathVariable("productId")Long productId);
}
声明完为feign client后,其他spring管理的类,如service就可以直接注入使用了,如下代码:
//这里直接注入feign client
@Autowired
private CartFeignClient cartFeignClient;
@PostMapping("/toCart/{productId}")
public ResponseEntity addCart(@PathVariable("productId") Long productId){
Long result = cartFeignClient.addCart(productId);
return ResponseEntity.ok(result);
}
使用feign之后,我们调用eureka 注册的其他服务,在代码中就像各个service之间相互调用那么简单。
FeignClient注解的一些属性
Feign自定义处理返回的异常,自定义好异常处理类后,要在@Configuration修饰的配置类中声明此类。
public class StashErrorDecoder implements ErrorDecoder {
@Override
public Exception decode(String methodKey, Response response) {
if (response.status() >= 400 && response.status() <= 499) {
//这里是给出的自定义异常
return new StashClientException(
response.status(),
response.reason()
);
}
if (response.status() >= 500 && response.status() <= 599) {
//这里是给出的自定义异常
return new StashServerException(
response.status(),
response.reason()
);
}
//这里是其他状态码处理方法
return errorStatus(methodKey, response);
}
}
Feign原理简述
1.启动时,程序会进行包扫描,扫描所有包下所有@FeignClient注解的类,并将这些类注入到spring的IOC容器中。当定义的Feign中的接口被调用时,通过JDK的动态代理来生成RequestTemplate。
2.RequestTemplate中包含请求的所有信息,如请求参数,请求URL等。
3.RequestTemplate声场Request,然后将Request交给client处理,这个client默认是JDK的HTTPUrlConnection,也可以是OKhttp、Apache的HTTPClient等。
4.最后client封装成LoadBaLanceClient,结合ribbon负载均衡地发起调用。
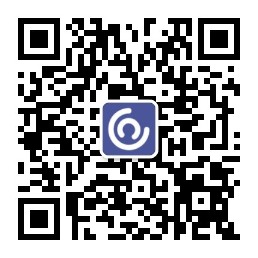
为提高通信效率,Feign开启GZIP压缩
application.yml配置信息如下:
feign:
compression:
request: #请求
enabled: true #开启
mime-types: text/xml,application/xml,application/json #开启支持压缩的MIME TYPE
min-request-size: 2048 #配置压缩数据大小的下限
response: #响应
enabled: true #开启响应GZIP压缩
特别注意:
由于开启GZIP压缩之后,Feign之间的调用数据通过二进制协议进行传输,返回值需要修改为ResponseEntity<byte[]>才可以正常显示,否则会导致服务之间的调用乱码。
eg:
@PostMapping("/order/{productId}")
ResponseEntity<byte[]> addCart(@PathVariable("productId") Long productId);
作用在所有Feign Client上的配置方式
方式一:通过java bean 的方式指定。
@EnableFeignClients注解上有个defaultConfiguration属性,可以指定默认Feign Client的一些配置。
@EnableFeignClients(defaultConfiguration = DefaultFeignConfiguration.class)
@EnableDiscoveryClient
@SpringBootApplication
@EnableCircuitBreaker
public class ProductApplication {
public static void main(String[] args) {
SpringApplication.run(ProductApplication.class, args);
}
}
DefaultFeignConfiguration内容:
@Configuration
public class DefaultFeignConfiguration {
@Bean
public Retryer feignRetryer() {
return new Retryer.Default(1000,3000,3);
}
}
方式二:通过配置文件方式指定。
feign:
client:
config:
default:
connectTimeout: 5000 #连接超时
readTimeout: 5000 #读取超时
loggerLevel: basic #日志等级