依赖
poi是导出excel需要引入的包;poi-ooxml和poi-ooxml-schemas是导出word需要引入的包
poi是操作xls的,poi-ooxml是操作xlsx的
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml-schemas</artifactId>
<version>4.1.2</version>
</dependency>
POI结构
HSSF——读写xls xls的最大行列数量:65536行,256列
XSSF——读写xlsx xlsx的最大行列数量:1048576行,16384列
HWPF——读写DOC
HSLF——读写PPT
读写excle
一、写入excle【xls】 new HSSFWorkbook()
1、设置行高:setHeight();设置列宽:setColumnWidth();数值单位是1/256,如要2个字符的位置,则要填入:2*256
2、日期格式:采用new SimpleDateFormat("yyyy/MM/dd");中的.format()方法进行格式化
3、workboot 工作簿;sheet工作表;最后写入是workbook的write()方法
public static void write() throws IOException {
//1.创建工作簿
HSSFWorkbook workbook = new HSSFWorkbook();
//2.创建工作表
HSSFSheet sheet1 = workbook.createSheet("工作表1");
//列宽:单位1/256 20个字符宽度20*256
//poi中的行高=excel中的行高*20
// sheet1.setDefaultColumnWidth(20*256);
//3.创建1行
HSSFRow row = sheet1.createRow(0);
//像素:excel默认行高13.5磅,像素=(13.5/72)*96=18
row.setHeight((short)(3*256));
//一个字符8个像素
String[] row1=new String[]{"日期","今日观众数","今日购票人数","总计"};
//4.创建单元格
for (int i=0;i<4;i++){
//列宽
sheet1.setColumnWidth(i,20*256);
HSSFCell cell = row.createCell(i);
cell.setCellValue(row1[i]);
}
//设置日期
HSSFRow row2 = sheet1.createRow(1);
HSSFCell cell = row2.createCell(1);
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy/MM/dd");
cell.setCellValue(simpleDateFormat.format(new Date()));
//5.创建表
FileOutputStream fos = new FileOutputStream("D:\\JavaProject\\观众统计表.xls");
//6.写
workbook.write(fos);
System.out.println("文件写入中");
fos.close();
System.out.println("文件写入完毕");
}
最后的表格:
扫描二维码关注公众号,回复:
14564262 查看本文章
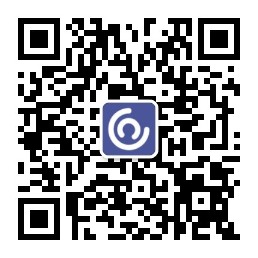
4、设置工作簿的样式
workbook中的createCellStyle()方法
public static void main(String[] args) throws IOException {
//xls
XSSFWorkbook workbook = new XSSFWorkbook();
XSSFSheet sheet = workbook.createSheet("测试1");
//工作簿的样式
XSSFCellStyle style = workbook.createCellStyle();
style.setBorderTop(BorderStyle.THICK);
style.setBorderBottom(BorderStyle.THICK);
//三行四列
for (int i=0;i<3;i++){
//行
XSSFRow row = sheet.createRow(i);
for (int j=0;j<4;j++){
XSSFCell cell = row.createCell(j);
cell.setCellStyle(style);
cell.setCellValue(1);
}
}
workbook.write(new FileOutputStream(new File("D:\\JavaProject\\测试表.xls")));
workbook.close();
System.out.println("结束");
}
最后表格:
二、写入excle【xlsx】 new XSSFWorkbook()
public static void main(String[] args) {
//xlsx 百万级数量
XSSFWorkbook sheets = new XSSFWorkbook();
XSSFSheet sheet = sheets.createSheet("测试百万数量级");
sheet.createRow(0);
}
百万级别导入:
public class SheetHandler implements XSSFSheetXMLHandler.SheetContentsHandler {
//private User user=null;
//每一行开始
public void startRow(int i) {
if (i>0){
//第一行为title不需要new实例
//new实例
}else{
//user==null
}
}
//每一行结束
public void endRow(int i) {
if (i>0){
System.out.println("导入结束");
}
}
//每一个单元格
public void cell(String s, String s1, XSSFComment xssfComment) {
//判断是不是第一行
//if(user!=null)
//获得单元格首字母
String letter = s.substring(0, 1);
switch (letter){
case 'A'{
System.out.println("打印A");
//do sth
}case'B'{
System.out.println("打印B");
//do sth
}
}
}
}
三、Opencsv读写excle
CsvWriter中的writeNext()方法是读取String[]数组,写入每一行
CsvWriter中的readNext()方法是读取表格中的每一行,读取后可以转换为需要的实体类
public void write() throws IOException {
CSVWriter csvWriter = new CSVWriter(new OutputStreamWriter(new FileOutputStream("D:\\JavaProject\\opencsv测试表.xls"), "utf-8"));
String[] title=new String[]{"日期","姓名","年龄"};
csvWriter.writeNext(title);
//分页查询内容
//数组为空,while break
//数组不为空,写入csv中
csvWriter.writeNext(new String[]{});
//page++
csvWriter.flush();
csvWriter.close();
}
public void read() throws IOException, CsvException {
CSVReader reader = new CSVReader(new FileReader("D:\\JavaProject\\opencsv测试表.xls"));
String[] title = reader.readNext();//读取标题
//while
String[] strings = reader.readNext();
//判断strings是否为空,不为空转为实体类,为空while break
}
Mysql导入百万数量级数据脚本
在函数中编写文件:
delimiter $$ ---重新定义; 不必自动提交 因为;是自动提交
drop procedure if exists test_insert $$ --如果有test_insert()这个存储过程就删除
create procedure test_insert() --创建存储过程
begin
declare n int default 1;
set autocommit=0;
while n<=5000000 do
insert into 'tb_user1' values() --填入自己需要插入的数据库和值
set n=n+1;
end while;
commit;
end $$