一、说明
经常会被问到需要点击软件的,主要都是玩游戏的盆友,但是也有其它用途的。所以简单弄了一个,打算每当有时间,有需求,就加一些小功能。
这里主要是要记录一下相关开发工作,也记录一些使用/更新的信息。
二、更新记录
1、版本说明
【2022/08/22】版本v1.0(初始版本,主要包含间隔点击模式、识别图片点击模式)
2、下载地址
三、功能描述
1、点击模式
目前实现两种点击模式(仅支持鼠标左键单击)。
间隔模式:一句话描述,按设置好的间隔时间鼠标左键单击用户设定的位置。
图片模式:一句话描述,用户截图想要识别的图片,上传到软件,然后软件按设置好的间隔时间识别屏幕是否出现了用户上传的图片,如果识别到了,则鼠标左键单击用户设定的位置。
后面会增加一些点击模式,比如文字识别,颜色识别等。
2、基础设置
主要设置
扫描二维码关注公众号,回复:
14503140 查看本文章
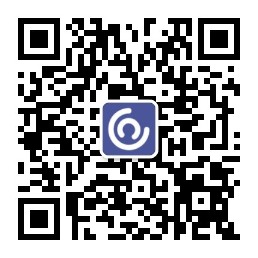
四、源码简述
1、用户控件
2、枚举/实体
3、windows api
internal class Win32Api
{
[StructLayout(LayoutKind.Sequential)]
public class POINT
{
public int x;
public int y;
}
[StructLayout(LayoutKind.Sequential)]
public class MouseHookStruct
{
public POINT pt;
public int hwnd;
public int wHitTestCode;
public int dwExtraInfo;
}
public delegate int HookProc(int nCode, IntPtr wParam, IntPtr lParam);
//安装钩子
[DllImport("user32.dll", CharSet = CharSet.Auto, CallingConvention = CallingConvention.StdCall)]
public static extern int SetWindowsHookEx(int idHook, HookProc lpfn, IntPtr hInstance, int threadId);
//卸载钩子
[DllImport("user32.dll", CharSet = CharSet.Auto, CallingConvention = CallingConvention.StdCall)]
public static extern bool UnhookWindowsHookEx(int idHook);
//调用下一个钩子
[DllImport("user32.dll", CharSet = CharSet.Auto, CallingConvention = CallingConvention.StdCall)]
public static extern int CallNextHookEx(int idHook, int nCode, IntPtr wParam, IntPtr lParam);
[DllImport("User32")]
public extern static void SetCursorPos(int x, int y);
//定义快捷键
[DllImport("user32.dll", SetLastError = true)]
public static extern bool RegisterHotKey(IntPtr hWnd, int id, KeyModifiers fsModifiers, int vk);
[Flags()]
public enum KeyModifiers
{
None = 0,
Alt = 1,
Ctrl = 2,
Shift = 4,
WindowsKey = 8
}
/// <summary>
/// 取消注册热键
/// </summary>
/// <param name="hWnd">要取消热键的窗口的句柄</param>
/// <param name="id">要取消热键的ID</param>
/// <returns></returns>
[DllImport("user32.dll", SetLastError = true)]
public static extern bool UnregisterHotKey(IntPtr hWnd, int id);
/// <summary>
/// 向全局原子表添加一个字符串,并返回这个字符串的唯一标识符,成功则返回值为新创建的原子ID,失败返回0
/// </summary>
/// <param name="lpString"></param>
/// <returns></returns>
[DllImport("kernel32", SetLastError = true)]
public static extern short GlobalAddAtom(string lpString);
/// <summary>
/// 热键的对应的消息ID
/// </summary>
public const int WM_HOTKEY = 0x312;
[DllImport("kernel32", SetLastError = true)]
public static extern short GlobalDeleteAtom(short nAtom);
[DllImport("user32.dll", CharSet = CharSet.Auto, CallingConvention = CallingConvention.StdCall)]
public static extern int mouse_event(int dwflags, int dx, int dy, int cbuttons, int dwextrainfo);
public const int mouseeventf_move = 0x0001; //移动鼠标
public const int mouseeventf_leftdown = 0x0002; //模拟鼠标左键按下
public const int mouseeventf_leftup = 0x0004; //模拟鼠标左键抬起
public const int mouseeventf_rightdown = 0x0008; //模拟鼠标右键按下
public const int mouseeventf_rightup = 0x0010; //模拟鼠标右键抬起
public const int mouseeventf_middledown = 0x0020; //模拟鼠标中键按下
public const int mouseeventf_middleup = 0x0040; //模拟鼠标中键抬起
public const int mouseeventf_absolute = 0x8000; //标示是否采用绝对坐标
}
4、定时器
timer = new System.Timers.Timer();
timer.Enabled = true;
timer.Interval = settingModel.intervals; //执行间隔时间,单位为毫秒
timer.Elapsed += new System.Timers.ElapsedEventHandler(myTimedTask);
5、快捷键
int f1 = Win32Api.GlobalAddAtom("F1");
int f5 = Win32Api.GlobalAddAtom("F5");
int f6 = Win32Api.GlobalAddAtom("F6");
Win32Api.RegisterHotKey(this.Handle, f1, Win32Api.KeyModifiers.None, (int)Keys.F1);
Win32Api.RegisterHotKey(this.Handle, f5, Win32Api.KeyModifiers.None, (int)Keys.F5);
Win32Api.RegisterHotKey(this.Handle, f6, Win32Api.KeyModifiers.None, (int)Keys.F6);
/// <summary>
/// 监视Windows消息
/// </summary>
/// <param name="m"></param>
protected override void WndProc(ref Message m)
{
switch (m.Msg)
{
case Win32Api.WM_HOTKEY:
ProcessHotkey(m);//按下热键时调用ProcessHotkey()函数
break;
}
base.WndProc(ref m); //将系统消息传递自父类的WndProc
}
/// <summary>
/// 按下设定的键时调用该函数
/// </summary>
/// <param name="m"></param>
private void ProcessHotkey(Message m)
{
IntPtr id = m.WParam;//IntPtr用于表示指针或句柄的平台特定类型
int sid = id.ToInt32();
if (sid == f1)
{
//do something
}
else if (sid == f5)
{
//do something
}
else if (sid == f6)
{
//do something
}
}