在学完javaswing的编程后,我自己动手实现了一个万年历和时钟。以下是我实现的代码:
1、DrawClock类:
package calender;
import java.awt.Color;
import java.awt.Graphics;
import java.util.Date;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.border.TitledBorder;
public class DrawClock extends JPanel implements Runnable {
Thread newThread;
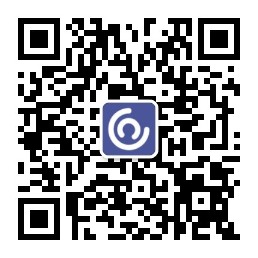
public static int RADIUS=80;
public int centerX=150;
public int centerY=120;
public int hr,min,sec;
public int[] xPoint=new int[4];
public int[] yPoint=new int[4];
public double hrAlpha,minAlpha,secAlpha,theta;
private JTextField timezone;
public void start(){
newThread=new Thread(this);
newThread.start();
}
public void stop(){
newThread=null;
}
public void paint(Graphics g){
super.paint(g);
//画出刻度
double minuteAlpha = Math.PI/30.0;
int count =0;
for(double alpha=0;alpha<2.0*Math.PI;alpha+=minuteAlpha){
int tX= (int)(centerX+RADIUS*0.9*Math.sin(alpha));
int tY= (int)(centerY-RADIUS*0.9*Math.cos(alpha));
if(count%5==0){
g.setColor(Color.BLUE);
g.fill3DRect(tX, tY, 3, 3, false);
if(count%3==0){
g.setColor(Color.RED);
int m=count/15;
switch(m){
case 1:g.drawString("3", centerX+RADIUS-18,centerY+5);
break;
case 2:g.drawString("6", centerX-3, centerY+RADIUS-10);
break;
case 3:g.drawString("9", centerX-RADIUS+11, centerY+6);
break;
default :g.drawString("12", centerX-5, centerY-RADIUS+22);
}
}
}else{
g.setColor(Color.GRAY);
g.fill3DRect(tX, tY, 2, 2, false);
}
count++;
}
//画出时针
g.setColor(Color.gray);
drawPointer(g,centerX+2,centerY+2,(int)(RADIUS*0.75),hrAlpha);
g.setColor(Color.CYAN);
drawPointer(g,centerX,centerY,(int)(RADIUS*0.75),hrAlpha);
//画出分针
g.setColor(Color.GRAY);
drawPointer(g,centerX+2,centerY+2,(int)(RADIUS*0.83),minAlpha);
g.setColor(Color.CYAN);
drawPointer(g,centerX,centerY,(int)(RADIUS*0.83),minAlpha);
//画出秒针
g.setColor(Color.GRAY);
g.drawLine(centerX, centerY, (int)(centerX+(RADIUS*0.79)*Math.sin(secAlpha)), (int)(centerY-(RADIUS*0.79)*Math.cos(secAlpha)));
setBorder(new TitledBorder("系统时间"));
setBackground(Color.WHITE);
g.drawRect(85, 210, 130, 20);
g.setColor(Color.WHITE);
g.setColor(Color.DARK_GRAY);
Date nowDate =new Date();
g.drawString(nowDate.toLocaleString(), 100, 225);
}
//得到系统时间
public Date getDate(){
Date nowDate =new Date();
return nowDate;
}
//刷新图层
public void update(Graphics g){
paint(g);
}
public void run() {
while(newThread!=null){
repaint();
try {
Thread.sleep(800);
} catch (InterruptedException e) {
e.printStackTrace();
}
Date timeNow =new Date();
int hours=timeNow.getHours();
int minutes=timeNow.getMinutes();
int seconds =timeNow.getSeconds();
hr=hours%12;
min=minutes;
sec=seconds;
theta=Math.PI/6.0/20.0 ;
hrAlpha=(double)(hr*3600+min*60+sec)/(12*3600.0)*2.0*Math.PI;
minAlpha=(double)(min*60+sec)/3600.0*2.0*Math.PI;
secAlpha =(double)(sec)/60.0*2.0*Math.PI;
}
}
private void drawPointer(Graphics g, int x, int y, int len, double theta) {
xPoint[0]=(int)(x+len*0.3*Math.sin(theta-Math.PI));
yPoint[0]=(int)(y-len*0.3*Math.cos(theta-Math.PI));
xPoint[1]=(int)(xPoint[0]+len*0.3*Math.sin(theta-(5.0/180)*Math.PI));
yPoint[1]=(int)(yPoint[0]-len*0.3*Math.cos(theta-(5.0/180)*Math.PI));
xPoint[2]=(int)(xPoint[0]+len*Math.sin(theta));
yPoint[2]=(int)(yPoint[0]-len*Math.cos(theta));
xPoint[3]=(int)(xPoint[0]+len*0.3*Math.sin(theta+(5.0/180)*Math.PI));
yPoint[3]=(int)(yPoint[0]-len*0.3*Math.cos(theta+(5.0/180)*Math.PI));
g.fillPolygon(xPoint, yPoint,4);
}
}
2、日历类:
package calender;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Date;
import java.util.GregorianCalendar;
import javax.swing.JComboBox;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.border.TitledBorder;
public class Calender extends JPanel implements ActionListener {
public static final String HOUR_OF_DAY=null;
JComboBox yearCom=new JComboBox();
JComboBox monthCom = new JComboBox();
JLabel year_i=new JLabel("年");
JLabel month_i=new JLabel("月");
Date nowDate =new Date();
JLabel[] labDay= new JLabel[49];
int now_year=nowDate.getYear()+1900;
int now_month=nowDate.getMonth();
boolean bool =false;
String year_int=null;
int month_int=0;
JPanel paneYM=new JPanel();
JPanel paneDay =new JPanel();
public Calender(){
super();
for(int year=now_year-30;year<=now_year+30;year++){
yearCom.addItem(year+"");
}
for(int i=1;i<13;i++){
monthCom.addItem(i+"");
}
yearCom.setSelectedIndex(30);
paneYM.add(new JLabel(" "));
paneYM.add(yearCom);
paneYM.add(year_i);
monthCom.setSelectedIndex(now_month);
paneYM.add(monthCom);
paneYM.add(month_i);
paneYM.add(new JLabel(" "));
monthCom.addActionListener( this);
yearCom.addActionListener(this);
paneDay.setLayout(new GridLayout(7,7,10,10));
for(int i=0;i<49;i++){
labDay[i] =new JLabel(" ");
paneDay.add(labDay[i]);
}
this.setDay();
this.setLayout(new BorderLayout());
this.add(paneDay,BorderLayout.CENTER);
this.add(paneYM,BorderLayout.NORTH);
this.setSize(100,200);
this.setBorder(new TitledBorder("日历"));
setSize(300,300);
}
//设置日历中的日期
void setDay() {
if(bool){
year_int=now_year+"";
month_int=now_month;
}else{
year_int=yearCom.getSelectedItem().toString();
month_int=monthCom.getSelectedIndex();
}
int year_sel=Integer.parseInt(year_int)-1900;
Date date= new Date(year_sel,month_int,1);
GregorianCalendar cal=new GregorianCalendar();
cal.setTime(date);
String[] week={"星期日","星期一","星期二","星期三","星期四","星期五","星期六"};
int day=0;
int day_week=0;
for(int i=0;i<7;i++){
labDay[i].setText(week[i]);
}
if(month_int==0||month_int==2||month_int==4||month_int==6||month_int==9||month_int==11){
day=31;
}else if(month_int==1 ||month_int==3||month_int==5||month_int==7||month_int==8||month_int==10){
day=30;
}else{
if(cal.isLeapYear(year_sel)){
day=29;
}else{
day=28;
}
}
day_week=7+date.getDay();
int count=1;
for(int i=day_week;i
if(i %7==0||i==13||i==20||i==27||i==48||i==34||i==41){
if(i==day_week+nowDate.getDate()-1){
labDay[i].setForeground(Color.BLUE);
labDay[i].setText(count+"'");
}else{
labDay[i].setForeground(Color.RED);
labDay[i].setText(count+"");
}
}else{
if(i==day_week+nowDate.getDate()-1){
labDay[i].setForeground(Color.BLUE);
labDay[i].setText(count+"'");
}else{
labDay[i].setForeground(Color.BLACK);
labDay[i].setText(count+"");
}
}
}
if(day_week==0){
for(int i=day;i<49;i++){
labDay[i].setText(" ");
}
}else{
for(int i=7;i
labDay[i].setText(" ");
}
for(int i=day_week+day;i<49;i++){
labDay[i].setText(" ");
}
}
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource()==yearCom||e.getSource()==monthCom){
bool=false;
this.setDay();
}
}
}
3、Clock时钟类:
package calender;
import java.awt.BorderLayout;
import java.awt.Color;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.border.TitledBorder;
public class Clock extends JPanel {
private UIManager.LookAndFeelInfo looks[];
private DrawClock clock;
private JPanel paneClock;
JPanel paneCal;
public Clock(){
super();
looks=UIManager.getInstalledLookAndFeels();
changeTheLookAndFeel(2);
clock=new DrawClock();
clock.start();
this.setBackground(Color.GRAY);
this.setLayout(new BorderLayout());
this.setOpaque(false);
this.add(clock);
this.setBorder(new TitledBorder("时间日期 "));
setSize(300,300);
setVisible(true);
}
private void changeTheLookAndFeel(int i) {
try{
UIManager.setLookAndFeel(looks[i].getClassName());
SwingUtilities.updateComponentTreeUI(this);
}catch(Exception e){
e.printStackTrace();
}
}
}
4、测试启动类:
package calender;
import java.awt.BorderLayout;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Test extends JFrame { public Test(){ Clock clock=new Clock(); Calender cal=new Calender(); JPanel jp2 = new JPanel(); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setSize(600, 300); this.setVisible(true); this.setContentPane(clock); this.getContentPane().add(cal,BorderLayout.WEST); this.setResizable(false); } public static void main(String[] args){ Test test =new Test(); test.setTitle("万年历和时钟"); } }