2.3.1 对异常类型进行断言
对捕获的异常进行断言主要用在比如异常测试时,当我们给定了特定的值,程序产生我们期望的异常是正确的,此时就可以通过对捕获到的异常类型进行断言,当然也可以按照python代码的方式先对异常进行捕获,然后对异常的类型进行断言,比如如下代码(pytest-demo/ch02/ex_005/test_demo.py),,写了一个div的函数计算两个数的除法,当对此函数进行测试的时候,期望当除数给0的时候,异常类型为ZeroDivisionError,按照python语法的处理方式如下:
def div(a,b):
return a/b
def test_demo():
try:
c=div(10,0)
except Exception as e:
assert isinstance(e,ZeroDivisionError)
执行结果如下,显示用例通过,没有问题
G:\demo\pytest-demo\ch02\ex_005>pipenv run pytest
========================================================================= test session starts ==========================================================================
platform win32 -- Python 3.10.4, pytest-7.1.2, pluggy-1.0.0
rootdir: G:\demo\pytest-demo\ch02\ex_005
collected 1 item
test_demo.py . [100%]
========================================================================== 1 passed in 0.06s ===========================================================================
G:\demo\pytest-demo\ch02\ex_005>
其实pytest提供了一种更为简单便捷的对异常类型进行断言的方法,如下代码(pytest-demo/ch02/ex_006/test_demo.py)
import pytest
def div(a,b):
return a/b
def test_demo():
with pytest.raises(ZeroDivisionError):
c=div(10,0)
执行结果如下:可以看出,这种方式更加简洁方便
G:\demo\pytest-demo\ch02\ex_006>pipenv run pytest
========================================================================= test session starts ==========================================================================
platform win32 -- Python 3.10.4, pytest-7.1.2, pluggy-1.0.0
rootdir: G:\demo\pytest-demo\ch02\ex_006
collected 1 item
test_demo.py . [100%]
========================================================================== 1 passed in 0.01s ===========================================================================
G:\demo\pytest-demo\ch02\ex_006>
2.3.2 对捕获的异常信息进行断言
对捕获的异常信息进行判断,同样也可以使用python捕获异常的方法,如下(pytest-demo/ch02/ex_006/test_demo.py)
def div(a,b):
return a/b
def test_demo():
try:
c=div(10,0)
except Exception as e:
assert "division by zero" in str(e)
执行结果如下,显然,这种方式对异常信息进行判断也是可以的
G:\demo\pytest-demo\ch02\ex_007>pipenv run pytest -s
========================================================================= test session starts ==========================================================================
platform win32 -- Python 3.10.4, pytest-7.1.2, pluggy-1.0.0
rootdir: G:\demo\pytest-demo\ch02\ex_007
collected 1 item
test_demo.py .
========================================================================== 1 passed in 0.01s ===========================================================================
G:\demo\pytest-demo\ch02\ex_007>
对异常信息进行判断同样pytest也提供了简洁的方法,如下(pytest-demo/ch02/ex_008/test_demo.py)
import pytest
def div(a,b):
return a/b
def test_demo():
with pytest.raises(ZeroDivisionError) as e:
c=div(10,0)
assert "division by zero" in str(e.value)
执行结果如下,可以看出,这种方式非常的简单便捷
G:\demo\pytest-demo\ch02>cd ex_008
G:\demo\pytest-demo\ch02\ex_008>pipenv run pytest
========================================================================= test session starts ==========================================================================
platform win32 -- Python 3.10.4, pytest-7.1.2, pluggy-1.0.0
rootdir: G:\demo\pytest-demo\ch02\ex_008
collected 1 item
test_demo.py . [100%]
========================================================================== 1 passed in 0.01s ===========================================================================
G:\demo\pytest-demo\ch02\ex_008>
2.3.3 同时对捕获的异常和异常信息进行判断断言
同时对异常类型和一唱歌信息进行判断断言时,当然同样也可以使用python捕获异常的方法,这里仍然不厌其烦的再演示一遍,其实不要小看了这些在学的时候感觉有点low的方法,其实在企业应用实战中,并非所有的场景所有的代码都是最优的最好的最简洁的,相反,很多时候其实主要是追求是否能达到需求,需求达到了,实现的方式倒不必在意,当然掌握更好的方式更好,但是实际应用中还是想到哪种方法就用哪种方法,目的就是满足实际中的需求
这里首先还是演示一下使用python捕获异常的方式,如下(pytest-demo/ch02/ex_009/test_demo.py)
def div(a,b):
return a/b
def test_demo():
try:
c=div(10,0)
except Exception as e:
assert isinstance(e, ZeroDivisionError)
assert "division by zero" in str(e)
执行结果如下,即同时使用两个assert语句,分别对捕获到的异常的类型和信息进行断言
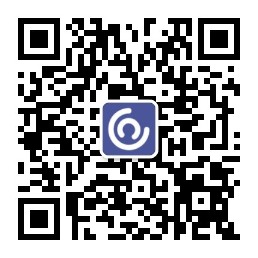
G:\demo\pytest-demo\ch02\ex_009>pipenv run pytest
========================================================================= test session starts ==========================================================================
platform win32 -- Python 3.10.4, pytest-7.1.2, pluggy-1.0.0
rootdir: G:\demo\pytest-demo\ch02\ex_009
collected 1 item
test_demo.py . [100%]
========================================================================== 1 passed in 0.01s ===========================================================================
G:\demo\pytest-demo\ch02\ex_009>
同样,pytest提供了简单的方式,即在指定异常类型的同时通过正则表达式指定异常信息的匹配规则,如下(pytest-demo/ch02/ex_010/test_demo.py)
import pytest
def div(a,b):
return a/b
def test_demo():
with pytest.raises(ZeroDivisionError,match=r"division\s*by\s*zero"):
c=div(10,0)
执行结果如下,即同时判断了异常的类型和异常信息
G:\demo\pytest-demo\ch02\ex_010>pipenv run pytest
========================================================================= test session starts ==========================================================================
platform win32 -- Python 3.10.4, pytest-7.1.2, pluggy-1.0.0
rootdir: G:\demo\pytest-demo\ch02\ex_010
collected 1 item
test_demo.py . [100%]
========================================================================== 1 passed in 0.01s ===========================================================================
G:\demo\pytest-demo\ch02\ex_010>
2.3.4 对一个函数的可能产生的异常进行断言
通过pytest.raise对一个函数进行检查可能出现的异常,如下格式,会执行func函数,并将args和**kwargs传入func函数,然后判断此时func中报出的异常是否与第一个参数异常一致,如果一致就通过,如果不一致就失败
pytest.raises(ExpectedException, func, args, **kwargs),如下(pytest-demo/ch02/ex_01/test_demo.py)
import pytest
def div(a,b):
return a/b
def test_demo01():
pytest.raises(ZeroDivisionError,div,10,0)
def test_demo02():
pytest.raises(ZeroDivisionError,div,10,5)
执行结果如下,可以看出,这里有两个测试用例,第二个测试用例因为没有产生除数为零的异常,而期望产生异常,因此用例失败了
G:\demo\pytest-demo\ch02\ex_011>pipenv run pytest
========================================================================= test session starts ==========================================================================
platform win32 -- Python 3.10.4, pytest-7.1.2, pluggy-1.0.0
rootdir: G:\demo\pytest-demo\ch02\ex_011
collected 2 items
test_demo.py .F [100%]
=============================================================================== FAILURES ===============================================================================
_____________________________________________________________________________ test_demo02 ______________________________________________________________________________
def test_demo02():
> pytest.raises(ZeroDivisionError,div,10,5)
E Failed: DID NOT RAISE <class 'ZeroDivisionError'>
test_demo.py:11: Failed
======================================================================= short test summary info ========================================================================
FAILED test_demo.py::test_demo02 - Failed: DID NOT RAISE <class 'ZeroDivisionError'>
===================================================================== 1 failed, 1 passed in 0.12s ======================================================================
G:\demo\pytest-demo\ch02\ex_011>