题目
力扣链接
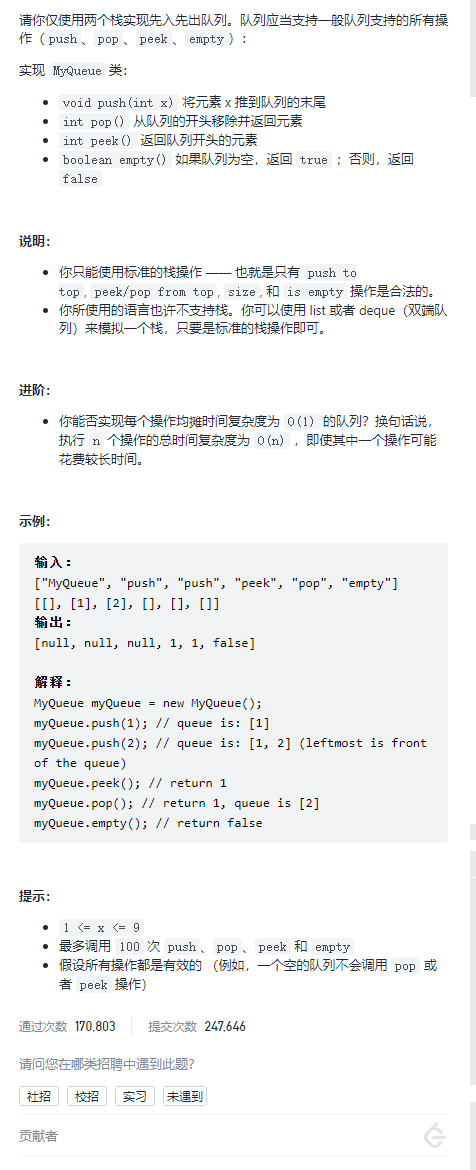
思路
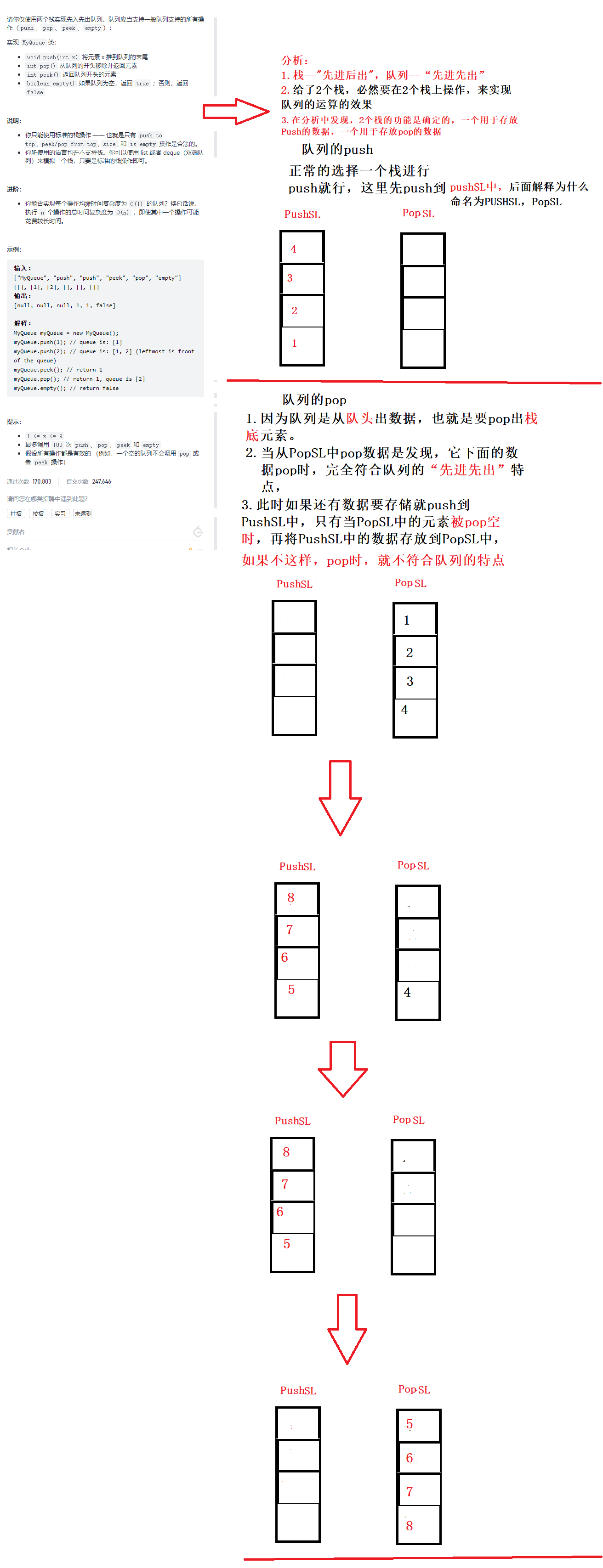
代码
typedef int SDateType;
typedef struct
{
SDateType* arr;
size_t sz;
size_t capacity;
}stack;
void StackInit(stack* s)
{
assert(s);
s->arr = NULL;
s->sz = 0;
s->capacity = 0;
}
bool StackEmpty(stack* s)
{
assert(s);
return s->sz == 0;
}
void StackDestroy(stack* s)
{
assert(s);
free(s->arr);
s->arr = NULL;
}
SDateType StackTop(stack* s)
{
assert(s);
assert(!StackEmpty(s));
return s->arr[s->sz - 1];
}
void StackPush(stack* s, SDateType x)
{
assert(s);
if (s->sz == s->capacity)
{
s->capacity = s->capacity == 0 ? 4 : 2 * s->capacity;
SDateType *a = (SDateType *)realloc(s->arr,sizeof(SDateType) * (s->capacity));
if (a == NULL)
{
exit(-1);
}
s->arr = a;
}
s->arr[s->sz] = x;
s->sz++;
}
void StackPop(stack* s)
{
assert(s);
assert(!StackEmpty(s));
s->sz--;
}
typedef struct {
stack pushST;
stack popST;
} MyQueue;
MyQueue* myQueueCreate() {
MyQueue* myqueue =(MyQueue*)malloc(sizeof(MyQueue));
if(myqueue==NULL)
{
perror("myqueue");
exit(-1);
}
StackInit(&(myqueue->pushST));
StackInit(&(myqueue->popST));
return myqueue;
}
bool myQueueEmpty(MyQueue* obj) {
assert(obj);
return StackEmpty(&(obj->pushST))&&StackEmpty(&(obj->popST));
}
void myQueuePush(MyQueue* obj, int x) {
assert(obj);
StackPush(&(obj->pushST),x);
}
int myQueuePop(MyQueue* obj) {
assert(obj);
assert(!myQueueEmpty(obj));
int ret=0;
if(!StackEmpty(&(obj->popST)))
{
ret=StackTop(&(obj->popST));
StackPop(&(obj->popST));
}
else
{
while(!StackEmpty(&(obj->pushST)))
{
StackPush(&(obj->popST),StackTop(&(obj->pushST)));
StackPop(&(obj->pushST));
}
ret=StackTop(&(obj->popST));
StackPop(&(obj->popST));
}
return ret;
}
int myQueuePeek(MyQueue* obj) {
assert(obj);
assert(!myQueueEmpty(obj));
int ret=0;
if(!StackEmpty(&(obj->popST)))
{
ret= StackTop(&(obj->popST));
}else
{
while(!StackEmpty(&(obj->pushST)))
{
StackPush(&(obj->popST),StackTop(&(obj->pushST)));
StackPop(&(obj->pushST));
}
ret=StackTop(&(obj->popST));
}
return ret;
}
void myQueueFree(MyQueue* obj) {
assert(obj);
StackDestroy(&(obj->pushST));
StackDestroy(&(obj->popST));
}