队列实现栈
1.解题思路
- 首先明确栈和队列分别有什么特点:栈是先进后出,队列是先进先出;
- 假设现在有qu1和qu2俩个队列;
- 现在你有一组数字[12,23,45,55,35];
- 你先将12,23,34进入队列qu2,因为实现的是栈的功能,现在要进行出栈操作,栈是后进先出,那么应该最先出去的是34;
- 但是现在34在队尾,所以你是不是要先把12,34先移动到旁边那个队列qu1这样,将qu2剩余的34直接弹出就可以;
- 假设你现在要弹23,同样的道理;(前提是另外一个队列为空)
- 假设你弹出34之后,没有弹出23,而是继续往进去加数字,那么应该加入哪个队列;
- 现在qu1有数字,那么你就加入到qu1中(哪个队列不为空就加入到哪一个中);
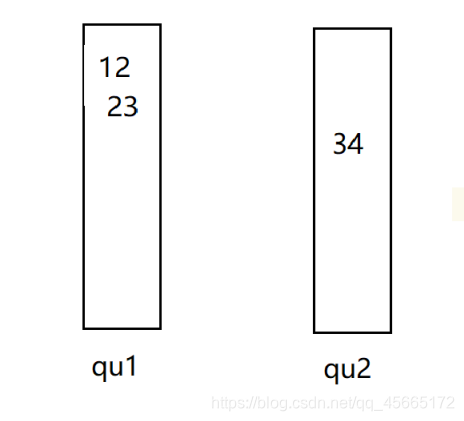
2.具体实现
2.1入队
public void push(int x) {
if (!qu1.isEmpty()){
qu1.offer(x);
}else if (!qu2.isEmpty()){
qu2.offer(x);
} else {
qu1.offer(x);
}
usedSize++;
}
2.2出队
在这里插入代码片
public int pop() {
if (empty()){
return -1;
}
int size = usedSize;
if (!qu1.isEmpty()){
for (int i = 0; i < size-1; i++) {
qu2.offer(qu1.poll());
}
usedSize--;
return qu1.poll();
}else{
for (int i = 0; i < size-1; i++) {
qu1.offer(qu2.poll());
}
usedSize--;
return qu2.poll();
}
}
2.3弹栈顶
public int top() {
if (empty()){
return -1;
}
int tmp = -1;
int size = usedSize;
if (!qu1.isEmpty()){
for (int i = 0; i < size ; i++) {
tmp = qu1.poll();
qu2.offer(tmp);
}
}else{
for (int i = 0; i < size; i++) {
tmp = qu2.poll();
qu1.offer(tmp);
}
}
return tmp;
}
2.4判断是否为空
public boolean empty() {
if(qu1.isEmpty() && qu2.isEmpty()){
return true;
}
return false;
}
3.完整代码
import java.util.LinkedList;
import java.util.Queue;
class MyStack {
private Queue<Integer> qu1;
private Queue<Integer> qu2;
public int usedSize;
public MyStack() {
qu1 = new LinkedList<>();
qu2 = new LinkedList<>();
}
public void push(int x) {
if (!qu1.isEmpty()){
qu1.offer(x);
}else if (!qu2.isEmpty()){
qu2.offer(x);
} else {
qu1.offer(x);
}
usedSize++;
}
public int pop() {
if (empty()){
return -1;
}
int size = usedSize;
if (!qu1.isEmpty()){
for (int i = 0; i < size-1; i++) {
qu2.offer(qu1.poll());
}
usedSize--;
return qu1.poll();
}else{
for (int i = 0; i < size-1; i++) {
qu1.offer(qu2.poll());
}
usedSize--;
return qu2.poll();
}
}
public int top() {
if (empty()){
return -1;
}
int tmp = -1;
int size = usedSize;
if (!qu1.isEmpty()){
for (int i = 0; i < size ; i++) {
tmp = qu1.poll();
qu2.offer(tmp);
}
}else{
for (int i = 0; i < size; i++) {
tmp = qu2.poll();
qu1.offer(tmp);
}
}
return tmp;
}
public boolean empty() {
if(qu1.isEmpty() && qu2.isEmpty()){
return true;
}
return false;
}
}
public class TestDemo2 {
public static void main(String[] args) {
MyStack myStack = new MyStack();
myStack.push(10);
myStack.push(20);
myStack.push(30);
System.out.println(myStack.top());
}
}