简单实现学生信心的增删改查:
创建数据库student和表studeninfo
在phpstudey WWW目录下创建项目student文件夹
1.首先创建coon文件夹,里面创建连接数据库的coon.php,代码如下:
<?php
$server='localhost';
$username='root';
$pwd='123456';
$db='student';
$conn=mysqli_connect($server,$username,$pwd,$db) or die('数据库连接失败!'.mysqli_error());
mysqli_query($conn,'set names utf8');
?>
2.创建插入页面,insert.php,详细代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<form method="post" action="insert_ok.php">
<table width="500px" border="1" bgcolor="#BBBBBB" align="center">
<tr>
<td height="40px">学号:
</td>
<td>
<input type="text" name="sno">
</td>
</tr>
<tr>
<td height="40px">姓名:
</td>
<td>
<input type="text" name="sname">
</td>
</tr>
<tr>
<td>年龄:
</td>
<td>
<input type="text" name="age">
</td>
</tr>
<tr>
<td>性别:
</td>
<td>
<input type="radio" name="sex" value="男">男
<input type="radio" name="sex" value="女">女
</td>
</tr>
<tr>
<td colspan="2" align="center">
<input type="submit" name="sub" value="提交">
<input type="reset" name="reset" value="重置">
</td>
</tr>
</table>
</form>
</body>
</html>
创建insert_ok.php:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>插入学生信息</title>
</head>
<body>
<?php
include_once('coon/conn.php');
if (!($_POST['sno']&&$_POST['sname']&&$_POST['age']&&$_POST['sex'])) {
echo "输入数据不允许为空,单击<a href='javascript:onclick=history.go(-1)'>这里</a>返回";
}else{
$str="insert into studentinfo values('".$_POST['sno']."','".$_POST['sname']."','".$_POST['age']."','".$_POST['sex']."')";
$result=mysqli_query($conn, $str);
if($result){
echo "数据添加成功,点击<a href='chakan.php'>查看</a>";
}else{
echo "<script>alet('添加失败');history.go(-1);";
}
}
?>
</body>
</html>
添加学生信息到数据库:
扫描二维码关注公众号,回复:
14122216 查看本文章
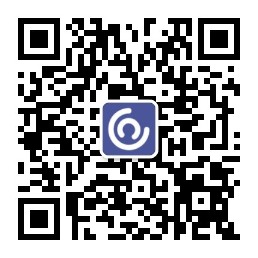
3.查看信息页面,chakan.php,详细代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<table width="760" border="0" align="center" cellpadding="0" cellspacing="0">
<tr>
<td align="center"><table width="700" border="0">
<tr>
<td width="10" align="center"></td>
<td width="50" align="center"><span class="STYLE1">学号</span></td>
<td width="252" align="center"><span class="STYLE1">姓名</span></td>
<td width="67" align="center"><span class="STYLE1">年龄</span></td>
<td width="156" align="center"><span class="STYLE1">性别</span></td>
</tr>
<?php
include_once('coon/conn.php');
mysqli_query($conn,'set names utf8');
$str = "select * from studentinfo";
$result = mysqli_query($conn,$str);
while($myrow = mysqli_fetch_row($result)){
?>
<tr>
<td align="center"></td>
<td align="center"><span class="STYLE2" style="color:blue"><?php echo $myrow[0];?></span></td>
<td align="center"><span class="STYLE2" style="color:blue"><?php echo $myrow[1]; ?></span></td>
<td align="center"><span class="STYLE2" style="color:blue"><?php echo $myrow[2].'岁'; ?></span></td>
<td align="center"><span class="STYLE2" style="color:blue"><?php echo $myrow[3]; ?></span></td>
<td align="center"><span class="STYLE2" style="color:blue"><?php echo "<a href=update.php?action=update&id=".$myrow[0].">修改</a>/<a href=delete.php?action=del&id=".$myrow[0]." onclick='return del();'>删除</a>" ?></span></td>
</tr>
<?php
}
?>
</table>
<tr>
<td align="right"><span class="STYLE2"><?php
$rows = mysqli_num_rows($result);
echo "查询结果为:".$rows.'条记录';
?><a href="insert.php">:继续添加学生信息</a></span>
</td>
</tr>
</table>
</body>
</html>
4.创建更新信息页面update.php,详细代码如下:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title></title>
</head>
<body>
<?php
include_once('coon/conn.php');
if ($_GET['action']=='update') {
$str = "select * from studentinfo where sno=".$_GET['id'];
$result = mysqli_query($conn,$str);
$myrow = mysqli_fetch_row($result);
}
?>
<table width="760" border="0" align="center" cellpadding="0" cellspacing="0">
<tr>
<td align="center">
<form name="update" method="post" action="update_ok.php">
<input type="hidden" name="action" value="update">
<input type="hidden" name="id" value="<?php echo $myrow[0] ?>">
<table width = '300'align="center" border="0" bgcolor="#BBBBBB">
<tr>
<td height="30" align="right">姓名:</td>
<td><input type="text" name="sname" value="<?php echo $myrow[1] ?>"></td>
</tr>
<tr>
<td height="30" align="right">年龄:</td>
<td><input type="text" name="age" value="<?php echo $myrow[2] ?>"></td>
</tr>
<tr>
<td height="30" align="right">性别:</td>
<td><input type="text" name="sex" value="<?php echo $myrow[3] ?>"></td>
</tr>
<tr>
<td colspan="2" align="center"><input type="submit" name="提交" value="修改">
<input type="reset" name="reset" value="重置"></td>
</tr>
</table>
</form>
</td>
</tr>
</table>
</body>
</html>
创建update_ok.php:
<?php
header("Content-type:text/html;charset=UTF-8");
include_once('coon/conn.php');
if ($_POST['action']=='update') {
if (!($_POST['sname'] && $_POST['age']&& $_POST['sex'])) {
echo "输入不允许为空。点击<a href='javascript:onclick=history.go(-1)'>这里</a>返回";
}else{
$str = "update studentinfo set sname='".$_POST['sname']."',age='".$_POST['age']."',sex='".$_POST['sex']."' where sno=".$_POST['id'];
$result = mysqli_query($conn,$str);
if ($result) {
echo"修改成功,点击<a href='chakan.php'>这里</a>返回";
}else{
echo "修改失败!";
}
}
}
?>
点击修改:
修改小明的年龄为:21
5.创建删除delete.php,详细代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<table width="760" border="0" align="center" cellpadding="0" cellspacing="0">
<tr>
<td align="center">
<?php
include_once('coon/conn.php');
//if ($_GET['action'] == 'del') {
$str = "delete from studentinfo where sno =".$_GET['id'];
$result = mysqli_query($conn,$str);
if ($result) {
echo "<script>alert('删除成功');location = 'chakan.php';</script>";
}else{
echo "删除失败!";
}
//}
?>
</td>
</tr>
</table>
</body>
</html>
创建delete_ok.php代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<table width="760" border="0" align="center" cellpadding="0" cellspacing="0">
<tr>
<td align="center">
<?php
include_once('coon/conn.php');
//if ($_GET['action'] == 'del') {
$str = "delete from bookinfo where id =".$_GET['id'];
$result = mysqli_query($conn,$str);
if ($result) {
echo "<script>alert('删除成功');location = 'select.php';</script>";
}else{
echo "删除失败!";
}
//}
?>
</td>
</tr>
</table>
</body>
</html>
点击删除小明的学生信息: