一. 概述:
基于Annotation装配,在和Spring IoC有关的配置技巧,这些技巧都是基于XML形式的配置文件进行的。在Spring中,尽管使用XML配置文件实现Bean的装配工作,但如果应用中有很多Bean时,则会导致XML配置文件过于臃肿,给后续的维护和升级工作带来了一定的困难。为此,从Spring2.0版本开始引入注解的配置方式,将Bean的配置和Bean实现类结合在一起,可进一步减少配置文件的代码量。
二. 常用的IoC注解
三. 两种方式注入
(一)、 @Configuration和@Bean
文件目录如下,一个GoodsHandler接口对应着它的两个实现类GoodsHandlerImpl类和OthergoodsHandlerImpl类, 还有一个配置类AnnotationConfig和测试类RunTest4
1. GoodsHandler接口:
public interface GoodsHandler {
public void showInfo();
}
2. GoodsHandlerImpl.java
public class GoodsHandlerImpl implements GoodsHandler{
@Override
public void showInfo() {
System.out.println("show goods information");
}
}
3. OthergoodsHandlerImpl.java
public class OtherGoodsHandlerImpl implements GoodsHandler{
@Override
public void showInfo() {
System.out.println("other information");
}
}
4. GoodsHandlerApp.java
public class GoodsHandlerApp {
@Value("GoodsHandlerApp---description")
private String description;
private GoodsHandler goodsHandler;
public GoodsHandlerApp(GoodsHandler goodsHandler){
this.goodsHandler = goodsHandler;
}
public void goodsHandler(){
this.goodsHandler.showInfo();
System.out.println(this.description);
}
}
5. AnnotationConfig.java
@Configuration
public class AnnotationConfig {
@Bean
public GoodsHandlerImpl goodsHandlerimpl(){return new GoodsHandlerImpl();}
@Bean
public OtherGoodsHandlerImpl othergoodsHandlerimpl(){return new OtherGoodsHandlerImpl();}
@Bean
public GoodsHandlerApp goodsHandlerApp(GoodsHandler goodsHandlerimpl){return new GoodsHandlerApp(goodsHandlerimpl);}
}
6. RunTest4.java
public class RunTest4 {
public static void main(String[] args){
ApplicationContext apc = new AnnotationConfigApplicationContext(AnnotationConfig.class);
GoodsHandlerApp goodsHandlerApp = apc.getBean(GoodsHandlerApp.class);
goodsHandlerApp.goodsHandler();
}
}
输出:
(二)、 @Configuration,@ComponentScan和@Component
1. 接口和测试类没有发生改变
2. GoodsHandlerImpl.java
@Component("goodsHandlerImpl")
public class GoodsHandlerImpl implements GoodsHandler{
@Override
public void showInfo() {
System.out.println("show goods information");
}
}
3. OthergoodsHandlerImpl.java
@Component("otherGoodsHandlerImpl")
public class OtherGoodsHandlerImpl implements GoodsHandler{
@Override
public void showInfo() {
System.out.println("other information");
}
}
4. GoodsHandlerApp.java
@Component
public class GoodsHandlerApp {
@Value("GoodsHandlerApp---description")
private String description;
private GoodsHandler goodsHandler;
public GoodsHandlerApp(@Qualifier(value = "goodsHandlerImpl")GoodsHandler goodsHandler){
this.goodsHandler = goodsHandler;
}
public void goodsHandler(){
this.goodsHandler.showInfo();
System.out.println(this.description);
}
}
5. AnnotationConfig.java
@Configuration
@ComponentScan(basePackages = "org.example.anno")
public class AnnotationConfig {
}
扫描二维码关注公众号,回复:
13778995 查看本文章
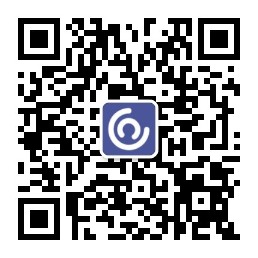