1. 点赞点踩样式准备
# 在base.html文件中 head标签内 添加css模块:
{% block css %}
{% endblock %}
# 修改 article_detail.html内容:
{% extends 'base.html' %}
{% block css %}
<style>
#div_digg {
float: right;
margin-bottom: 10px;
margin-right: 30px;
font-size: 12px;
width: 125px;
text-align: center;
margin-top: 10px;
}
.diggit {
float: left;
width: 46px;
height: 52px;
background: url(/static/img/upup.gif) no-repeat;
text-align: center;
cursor: pointer;
margin-top: 2px;
padding-top: 5px;
}
.buryit {
float: right;
margin-left: 20px;
width: 46px;
height: 52px;
background: url(/static/img/downdown.gif) no-repeat;
text-align: center;
cursor: pointer;
margin-top: 2px;
padding-top: 5px;
}
.clear {
clear: both;
}
.diggword {
margin-top: 5px;
margin-left: 0;
font-size: 12px;
color: #808080;
}
</style>
{% endblock %}
{% block content %}
<h1>{
{ article_detail.title }}</h1>
<div class="article_content">
{
{ article_detail.content|safe }}
</div>
{# 点赞点踩样式开始#}
<div class="clearfix">
<div id="div_digg">
<div class="diggit active">
<span class="diggnum" id="digg_count">{
{ article_detail.up_num }}</span>
</div>
<div class="buryit active">
<span class="burynum" id="bury_count">{
{ article_detail.down_num }}</span>
</div>
<div class="clear"></div>
<div class="diggword" id="digg_tips" style="color: red;"></div>
<div class="error_msg" style="color: red"></div>
</div>
</div>
{# 点赞点踩样式结束#}
{% endblock %}
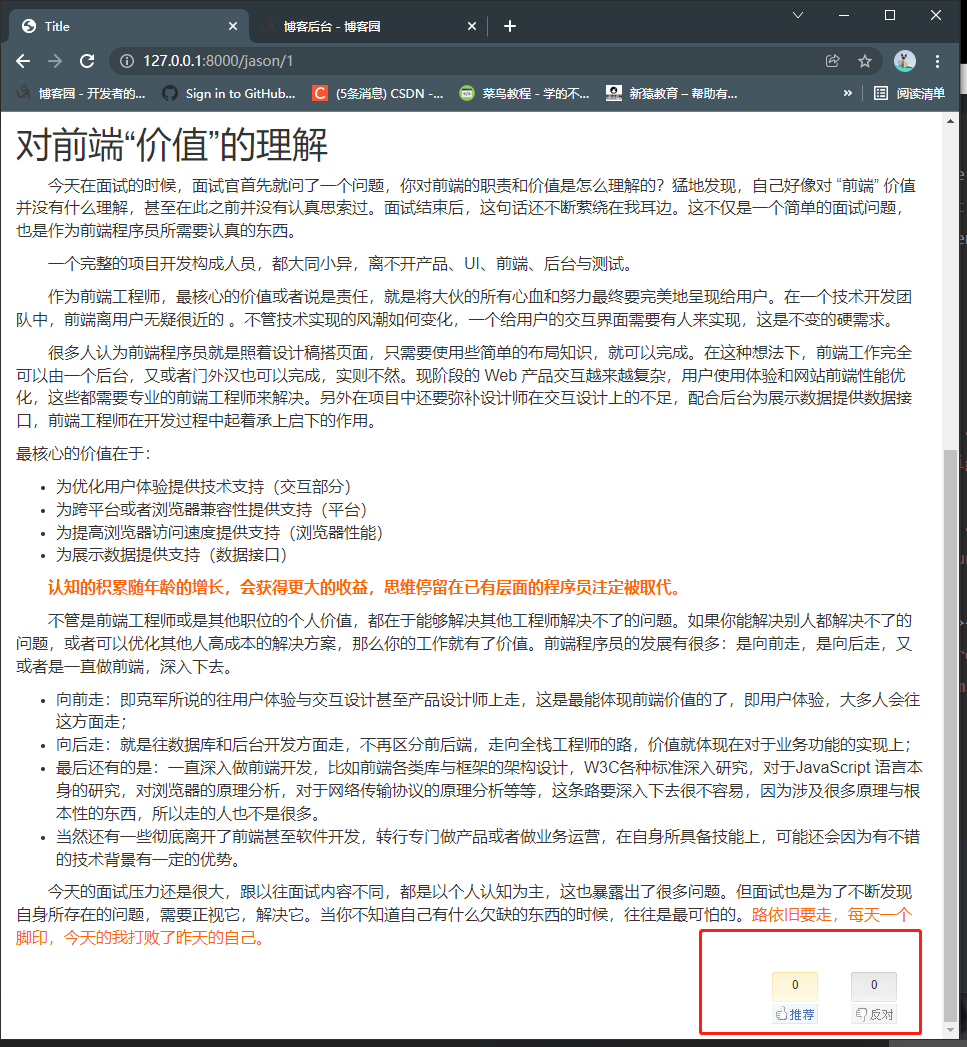
2. 前端逻辑书写
# 在base.html文件中 body标签内 添加:
{% block js %}
{% endblock %}
# 在 article_detail.html 添加js模块:
{% block js %}
<script>
$('.active').click(function () {
var is_up = $(this).hasClass('diggit'); // true false
var article_id = '{
{ article_id }}';
// 发送ajax请求
$.ajax({
url: '/up_or_down/',
type: 'post',
data: {'is_up': is_up, article_id: article_id},
success: function (res) {
console.log(res);
}
})
})
</script>
{% endblock %}
# 在views.py中添加功能:
# 9. 点赞点踩
def up_or_down(request):
print(123)
# 添加路由:
# 点赞点踩表
url(r'^up_or_down/', views.up_or_down),
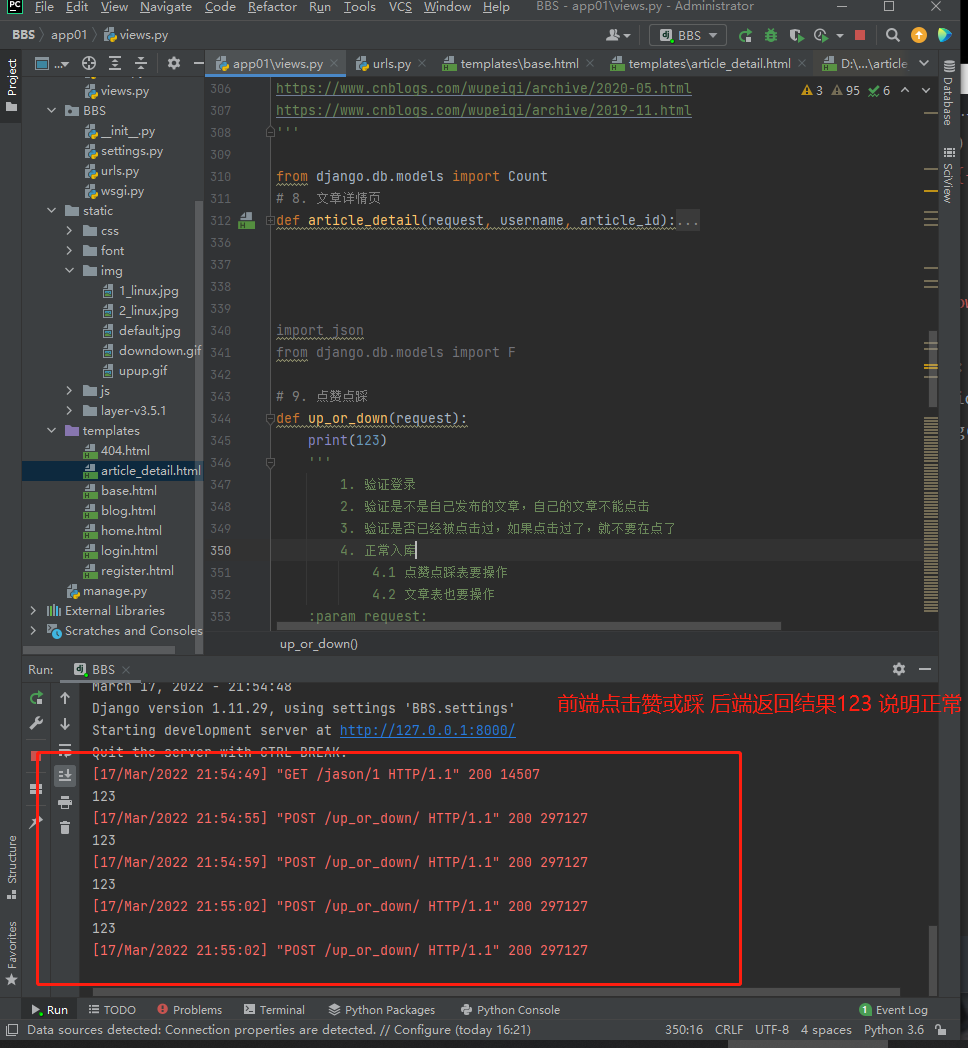
3. 后端逻辑实现
# 在views.py中添加 点赞点踩功能:
import json
from django.db.models import F
# 9. 点赞点踩
def up_or_down(request):
'''
1. 验证登录
2. 验证是不是自己发布的文章,自己的文章不能点击
3. 验证是否已经被点击过,如果点击过了,就不要在点了
4. 正常入库
4.1 点赞点踩表要操作
4.2 文章表也要操作
:param request:
:return:
'''
if request.method == 'POST':
user_id = request.session.get('id')
back_dic = {'status': 200, 'msg': '支持成功', 'data': {}}
# 1. 接收参数
is_up = request.POST.get('is_up') # true <class 'str'>
article_id = request.POST.get('article_id')
# print(is_up, type(is_up))
# 2. 验证参数是否登录
if not request.session.get('username'):
back_dic['status'] = 1010
back_dic['msg'] = '<a href="/login/" style="color: red">请先登录</a>'
return JsonResponse(back_dic)
# 验证是不是自己的文章
article_obj = models.Article.objects.filter(pk=article_id).first()
# 通过文章查用户,
if article_obj.blog.userinfo.username == request.session.get('username'):
# 当前点击的文章是自己的
back_dic['status'] = 1011
back_dic['msg'] = '不能点击自己的文章哦,宝贝~'
return JsonResponse(back_dic)
# 验证是否点击过,参考点赞点踩表
is_click = models.UpAndDown.objects.filter(article_id=article_id, user_id=user_id).first()
if is_click:
# 已经点过
back_dic['status'] = 1012
back_dic['msg'] = '你已经点过喽,宝贝~'
return JsonResponse(back_dic)
# 对传递过来的参数is_up做反序列话,需要转为布尔值
is_up = json.loads(is_up)
print(is_up, type(is_up))
if is_up:
# 操作文章表
models.Article.objects.filter(pk=article_id).update(up_num=F('up_num') + 1)
back_dic['msg'] = '点赞成功'
else:
# 点踩
models.Article.objects.filter(pk=article_id).update(down_num=F('down_num') + 1)
back_dic['msg'] = '反对成功'
# 操作点赞点踩表
models.UpAndDown.objects.create(is_up=is_up, article_id=article_id, user_id=user_id)
return JsonResponse(back_dic)
# 修改article_detail.html:
$('.active').click(function () {
var is_up = $(this).hasClass('diggit'); // true false
var article_id = '{
{ article_id }}';
// 发送ajax请求
$.ajax({
url: '/up_or_down/',
type: 'post',
data: {'is_up': is_up, article_id: article_id},
success: function (res) {
console.log(res);
if (res.status == 200) {
layer.msg(res.msg)
} else if (res.status == 1010) {
$('.error_msg').append(res.msg)
} else {
layer.msg(res.msg)
}
}
})
})
# 添加路由 :
# 点赞点踩表
url(r'^up_or_down/', views.up_or_down),
"""
注意:::
所有路由 最好短的放上面 避免匹配不到对应路由就已经结束匹配!!!
"""
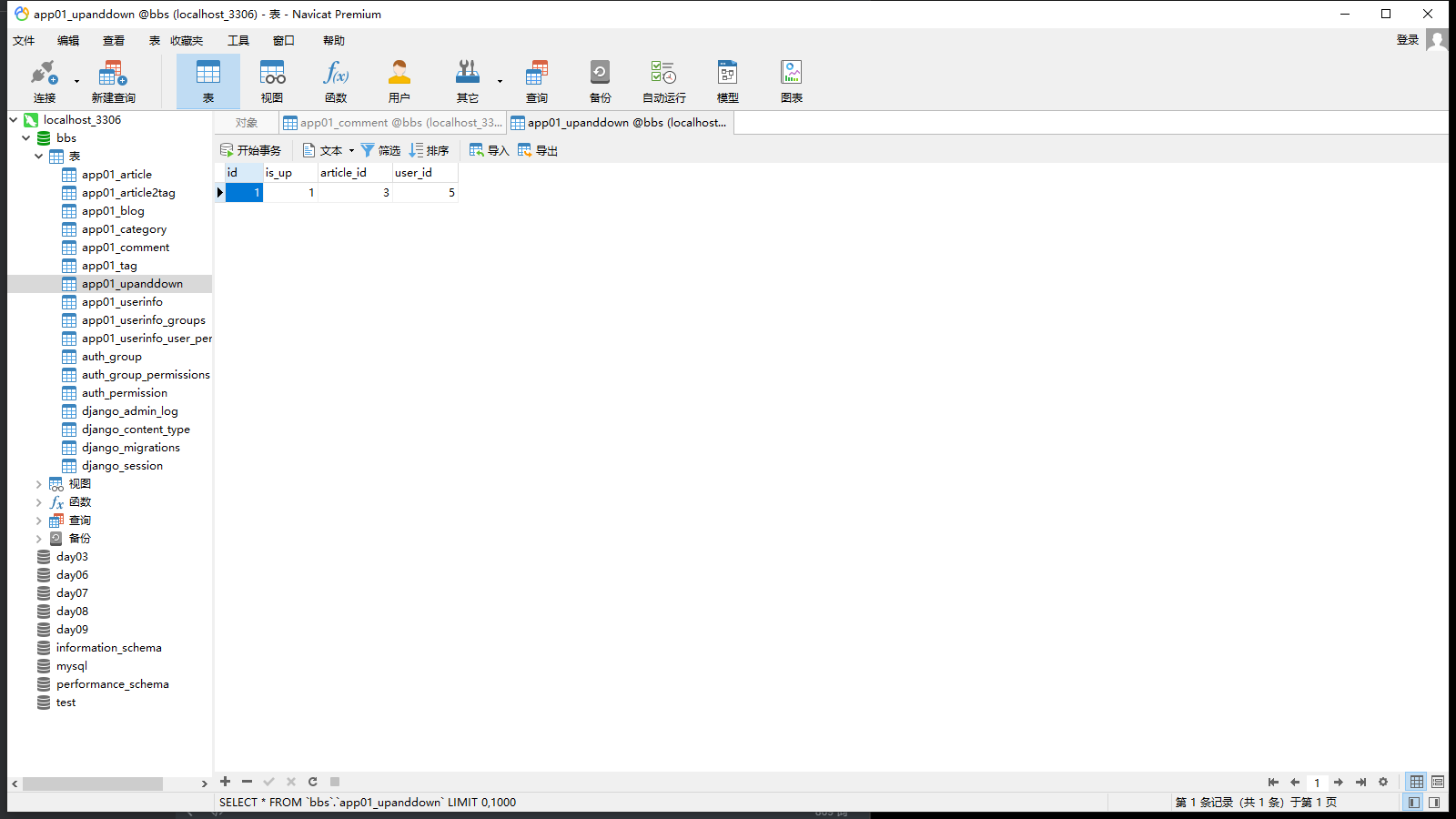
4. 前端逻辑实现
# 修改article_detail.html:
{% block js %}
<script>
$('.active').click(function () {
var is_up = $(this).hasClass('diggit'); // true false
var article_id = '{
{ article_id }}';
var _this = $(this)
// 发送ajax请求
$.ajax({
url: '/up_or_down/',
type: 'post',
data: {'is_up': is_up, article_id: article_id},
success: function (res) {
console.log(res);
if (res.status == 200) {
$('.error_msg').text(res.msg);
var old_num = Number(_this.children().text());
_this.children().text(old_num + 1)
} else if (res.status == 1010) {
$('.error_msg').append(res.msg)
} else {
layer.msg(res.msg)
}
}
})
})
</script>
{% endblock %}
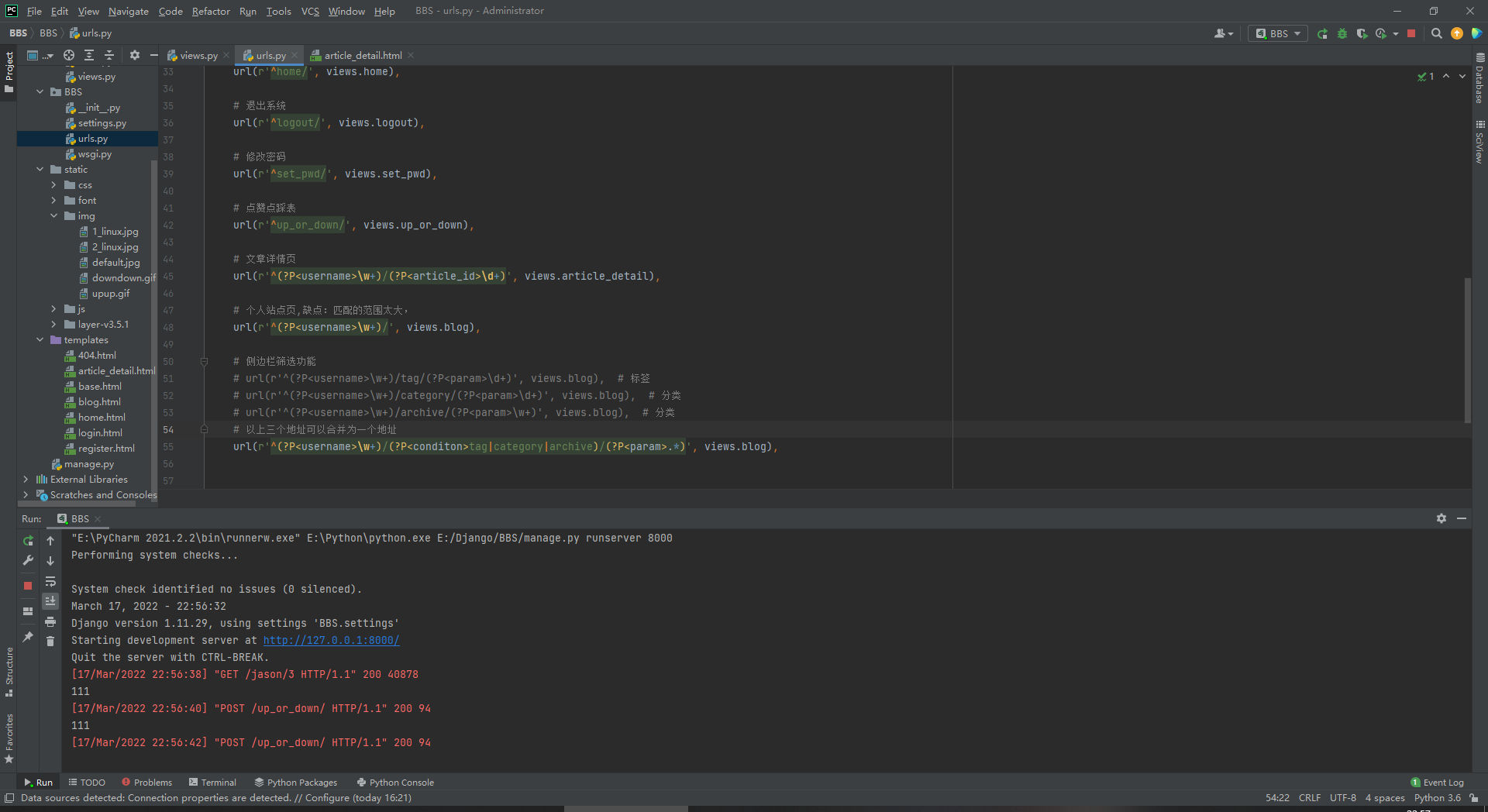
5. 评论样式准备
# 修改article_detail.html:
{# 点赞点踩样式结束#}
{# 评论样式开始#}
<div >
<p>
<span class="glyphicon glyphicon-comment"></span>发表评论
</p>
<p>
<textarea name="" id="" cols="30" rows="10"></textarea>
</p>
<p>
<input type="button" class="btn btn-success" value="提交">
</p>
</div>
{# 评论样式结束#}
<div style="height: 1000px"></div>
{% endblock %}
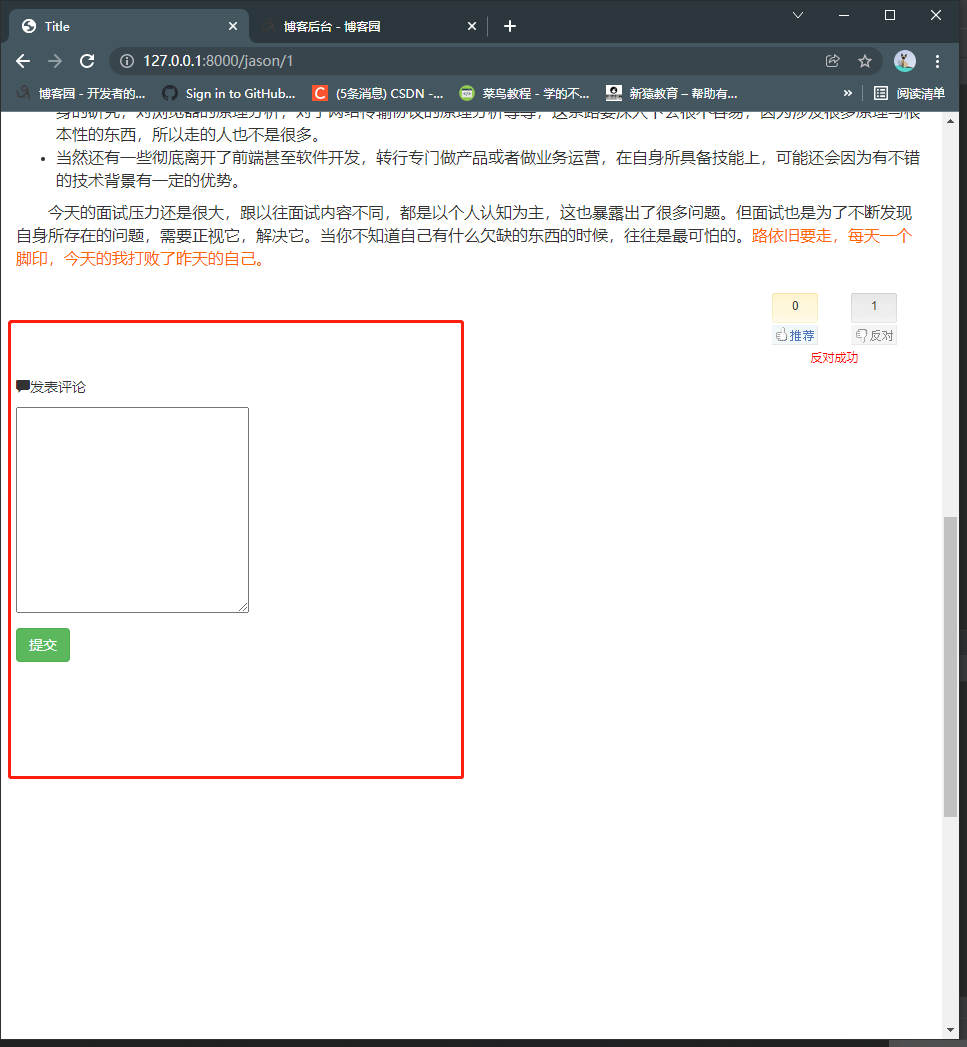