文章目录
一、整合 spring boot
1、引入依赖
<!-- 分布式事务seata包 -->
<!--seata begin-->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-seata</artifactId>
<version>2.1.3.RELEASE</version>
<exclusions>
<exclusion>
<groupId>io.seata</groupId>
<artifactId>seata-spring-boot-starter</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>io.seata</groupId>
<artifactId>seata-spring-boot-starter</artifactId>
<version>1.4.2</version>
</dependency>
<!--seata end-->
2、初始化数据库
创建数据库,名称就叫seata;
branch_table表
CREATE TABLE branch_table (
branch_id bigint(20) NOT NULL,
xid varchar(128) NOT NULL,
transaction_id bigint(20) DEFAULT NULL,
resource_group_id varchar(32) DEFAULT NULL,
resource_id varchar(256) DEFAULT NULL,
branch_type varchar(8) DEFAULT NULL,
status tinyint(4) DEFAULT NULL,
client_id varchar(64) DEFAULT NULL,
application_data varchar(2000) DEFAULT NULL,
gmt_create datetime(6) DEFAULT NULL,
gmt_modified datetime(6) DEFAULT NULL,
PRIMARY KEY (branch_id),
KEY idx_xid (xid)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
global_table表
CREATE TABLE global_table (
xid varchar(128) NOT NULL,
transaction_id bigint(20) DEFAULT NULL,
status tinyint(4) NOT NULL,
application_id varchar(32) DEFAULT NULL,
transaction_service_group varchar(32) DEFAULT NULL,
transaction_name varchar(128) DEFAULT NULL,
timeout int(11) DEFAULT NULL,
begin_time bigint(20) DEFAULT NULL,
application_data varchar(2000) DEFAULT NULL,
gmt_create datetime DEFAULT NULL,
gmt_modified datetime DEFAULT NULL,
PRIMARY KEY (xid),
KEY idx_gmt_modified_status (gmt_modified,status),
KEY idx_transaction_id (transaction_id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
lock_table表
CREATE TABLE lock_table (
row_key varchar(128) NOT NULL,
xid varchar(96) DEFAULT NULL,
transaction_id bigint(20) DEFAULT NULL,
branch_id bigint(20) NOT NULL,
resource_id varchar(256) DEFAULT NULL,
table_name varchar(32) DEFAULT NULL,
pk varchar(36) DEFAULT NULL,
gmt_create datetime DEFAULT NULL,
gmt_modified datetime DEFAULT NULL,
PRIMARY KEY (row_key),
KEY idx_branch_id (branch_id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
3、 业务库表
seata需要在每个业务库添加一张undo_log表,用于AT模式下的二阶段回滚
undo_log表
CREATE TABLE undo_log (
branch_id bigint(20) NOT NULL COMMENT 'branch transaction id',
xid varchar(100) NOT NULL COMMENT 'global transaction id',
context varchar(128) NOT NULL COMMENT 'undo_log context,such as serialization',
rollback_info longblob NOT NULL COMMENT 'rollback info',
log_status int(11) NOT NULL COMMENT '0:normal status,1:defense status',
log_created datetime(6) NOT NULL COMMENT 'create datetime',
log_modified datetime(6) NOT NULL COMMENT 'modify datetime',
UNIQUE KEY ux_undo_log (xid,branch_id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COMMENT='AT transaction mode undo table';
4、代理数据源
【注意:SeaTa的AT模式下必须使用代理数据源,但是SeaTa的TCC模式下必须不使用代理数据源,这里我们以AT模式为例子】
代理数据源配置如下:
package io.seata.samples.order.config;
import com.alibaba.druid.pool.DruidDataSource;
import io.seata.rm.datasource.DataSourceProxy;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.mybatis.spring.transaction.SpringManagedTransactionFactory;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import javax.sql.DataSource;
@Configuration
public class DataSourceConfig {
@Bean
@ConfigurationProperties(prefix = "spring.datasource")
public DataSource druidDataSource() {
DruidDataSource druidDataSource = new DruidDataSource();
return druidDataSource;
}
@Primary
@Bean("dataSource")
public DataSourceProxy dataSource(DataSource druidDataSource) {
return new DataSourceProxy(druidDataSource);
}
@Bean
public SqlSessionFactory sqlSessionFactory(DataSourceProxy dataSourceProxy) throws Exception {
SqlSessionFactoryBean factoryBean = new SqlSessionFactoryBean();
factoryBean.setDataSource(dataSourceProxy);
factoryBean.setMapperLocations(new PathMatchingResourcePatternResolver()
.getResources("classpath*:/mapper/*.xml"));
factoryBean.setTransactionFactory(new SpringManagedTransactionFactory());
return factoryBean.getObject();
}
}
5、配置文件
spring.application.name=order-service
server.port=8082
spring.datasource.url=jdbc:mysql://localhost:3306/seata_order?useSSL=false&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=root
#!!!分布式事务组,这个要与file.conf 中 service 里的 vgroup_mapping.my_test_tx_group 一样才行
spring.cloud.alibaba.seata.tx-service-group=my_test_tx_group
logging.level.io.seata=info
logging.level.io.seata.samples.order.persistence.OrderMapper=debug
二、微服务项目中的配置
1、在每个微服务里面都放入 registry.conf 和 file.conf 两个文件
扫描二维码关注公众号,回复:
13551400 查看本文章
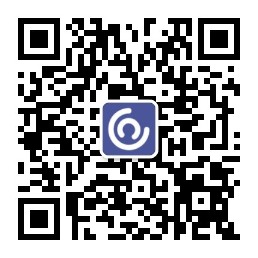
2、修改registry.conf文件
registry {
# file 、nacos 、eureka、redis、zk、consul、etcd3、sofa、custom
# type = "file"
# 这里我们用nacos
type = "nacos"
#这里是nacos 的一些配置项
nacos {
application = "seata-server"
serverAddr = "cdh1"
namespace = "e385bfe2-e743-4910-8c32-e05759f9f9f4"
cluster = "SEATA_GROUP"
username = "nacos"
password = "nacos"
}
eureka {
serviceUrl = "http://localhost:8761/eureka"
weight = "1"
}
redis {
serverAddr = "localhost:6379"
db = "0"
password = ""
timeout = "0"
}
zk {
serverAddr = "127.0.0.1:2181"
sessionTimeout = 6000
connectTimeout = 2000
username = ""
password = ""
}
consul {
serverAddr = "127.0.0.1:8500"
aclToken = ""
}
etcd3 {
serverAddr = "http://localhost:2379"
}
sofa {
serverAddr = "127.0.0.1:9603"
region = "DEFAULT_ZONE"
datacenter = "DefaultDataCenter"
group = "SEATA_GROUP"
addressWaitTime = "3000"
}
file {
name = "file.conf"
}
custom {
name = ""
}
}
config {
# file、nacos 、apollo、zk、consul、etcd3、springCloudConfig、custom
# 配置项我们依然用file ,也就是刚才我们每个微服务里都放了的 file.conf
type = "file"
nacos {
serverAddr = "127.0.0.1:8848"
namespace = ""
group = "SEATA_GROUP"
username = ""
password = ""
dataId = "seata.properties"
}
consul {
serverAddr = "127.0.0.1:8500"
aclToken = ""
}
apollo {
appId = "seata-server"
apolloMeta = "http://192.168.1.204:8801"
namespace = "application"
apolloAccesskeySecret = ""
}
zk {
serverAddr = "127.0.0.1:2181"
sessionTimeout = 6000
connectTimeout = 2000
username = ""
password = ""
nodePath = "/seata/seata.properties"
}
etcd3 {
serverAddr = "http://localhost:2379"
}
file {
name = "file.conf"
}
custom {
name = ""
}
}
3、file.conf本地配置
如果配置中心的类型都不是file时,就不需要file.conf文件了
如果配置中心的类型是file时,就需要file.conf
transport {
# tcp udt unix-domain-socket
type = "TCP"
#NIO NATIVE
server = "NIO"
#enable heartbeat
heartbeat = true
# the client batch send request enable
enableClientBatchSendRequest = true
#thread factory for netty
threadFactory {
bossThreadPrefix = "NettyBoss"
workerThreadPrefix = "NettyServerNIOWorker"
serverExecutorThread-prefix = "NettyServerBizHandler"
shareBossWorker = false
clientSelectorThreadPrefix = "NettyClientSelector"
clientSelectorThreadSize = 1
clientWorkerThreadPrefix = "NettyClientWorkerThread"
# netty boss thread size,will not be used for UDT
bossThreadSize = 1
#auto default pin or 8
workerThreadSize = "default"
}
shutdown {
# when destroy server, wait seconds
wait = 3
}
serialization = "seata"
compressor = "none"
}
service {
#transaction service group mapping
# my_test_tx_group为分组名称,需要与应用中的配置分组名称一致
# "default"为seata-server的集群名称,即seata-server注册到注册中心的名称
vgroupMapping.my_test_tx_group = "default"
#only support when registry.type=file, please don't set multiple addresses
# 当registry.type=file时,客户端通过ip:port连接到seata-server
default.grouplist = "127.0.0.1:8091"
#degrade, current not support
enableDegrade = false
#disable seata
disableGlobalTransaction = false
}
client {
rm {
asyncCommitBufferLimit = 10000
lock {
retryInterval = 10
retryTimes = 30
retryPolicyBranchRollbackOnConflict = true
}
reportRetryCount = 5
tableMetaCheckEnable = false
reportSuccessEnable = false
}
tm {
commitRetryCount = 5
rollbackRetryCount = 5
}
undo {
dataValidation = true
logSerialization = "jackson"
# undo表名称,SEATA AT模式需要UNDO_LOG表
logTable = "undo_log"
}
log {
exceptionRate = 100
}
}
到此配置完成!