进程调度
#include <iostream>
#include <cstring>
#include <cstdlib>
using namespace std;
typedef struct PCB {
char proName[10];
int proNum;
}PCB;
typedef struct QNode {
PCB pcb;
struct QNode *next;
}QNode, *QuenePtr;
class LinkQueue {
QuenePtr front;
QuenePtr rear;
public :
int size;
void InitQueue (LinkQueue *q) {
q -> front = q -> rear = (QuenePtr) malloc(sizeof (QNode));
if (!q -> front) cout << "OVERFLOW\n";
q -> front -> next = NULL;
q -> size = 0;
}
void EnQueue (LinkQueue *q, PCB *pcb) {
QuenePtr p;
p = (QuenePtr) malloc (sizeof(QNode));
if (!p) cout << "OVERFLOW\n";
p -> pcb = * pcb;
p -> next = NULL;
q -> rear -> next = p;
q -> rear = p;
q -> size++;
}
int DeQueue (LinkQueue *Q, PCB *pcb) {
QuenePtr p;
if (Q -> front == Q -> rear) return -1;
p = Q -> front -> next;
*pcb = p -> pcb;
Q -> front -> next = p -> next; //
// p = p -> next;
if (Q -> rear == p) Q -> rear = Q -> front; //尾指针指向头结点
free(p);
Q -> size--;
return 1;
}
void PrintQueue (LinkQueue *q) {
QuenePtr p;
p = q -> front -> next;
while (p) {
cout << p -> pcb.proName << endl;
p = p -> next;
}
cout << '\n';
}
};
LinkQueue creatLQ, exitLQ;
LinkQueue readyLQ, runningLQ, blockLQ;
class Status : public LinkQueue {
public :
void printAllQueue() {
cout << "-------各个队列当前的状态为-------\n";
cout << "执行队列: \n";
PrintQueue(&runningLQ);
cout << "就绪队列: \n";
LinkQueue :: PrintQueue(&readyLQ);
cout << "阻塞队列: \n";
LinkQueue :: PrintQueue(&blockLQ);
cout << "创建队列: \n";
LinkQueue :: PrintQueue(&creatLQ);
cout << "终止队列: \n";
LinkQueue :: PrintQueue(&exitLQ);
}
//就绪--->执行
void Dispatch(PCB pcb) {
if (runningLQ.size == 1) {
cout << "Error!! 存在进程在执行,调度失败!\n";
} else if (readyLQ.size == 0) {
cout << "Error! 就绪队列为空,无进程可调度!\n";
} else {
DeQueue (&readyLQ, &pcb);
EnQueue (&runningLQ, &pcb);
printAllQueue();
}
}
//执行--->就绪
void TLE(PCB pcb) {
if (runningLQ.size == 0) {
cout << "Error! 当前没有进程正在执行!\n";
} else if (readyLQ.size == 0) {
printAllQueue();
} else {
DeQueue(&runningLQ, &pcb);
EnQueue(&readyLQ, &pcb);
DeQueue(&readyLQ, &pcb); //就绪队列为空, 故一进入就绪队列就转化为执行状态
EnQueue(&runningLQ, &pcb);
printAllQueue();
}
}
//执行--->阻塞
void eventWait (PCB pcb) {
if (runningLQ.size == 0) {
cout << "Error ! 当前没有进程正在执行!\n";
} else if (readyLQ.size == 0) { //此时不发生调度
DeQueue(&runningLQ, &pcb);
EnQueue(&blockLQ, &pcb);
printAllQueue();
} else { //执行--->阻塞; 就绪---> 执行
DeQueue(&runningLQ, &pcb);
EnQueue(&blockLQ, &pcb);
DeQueue(&readyLQ, &pcb);
EnQueue(&runningLQ, &pcb);
printAllQueue();
}
}
//阻塞--->就绪
void eventComplete (PCB pcb) {
if (blockLQ.size == 0) {
cout << "Error! 阻塞队列为空!\n";
} else {
DeQueue(&blockLQ, &pcb);
EnQueue(&readyLQ, &pcb);
if (readyLQ.size == 1) {
Dispatch(pcb);
}
printAllQueue();
}
}
//创建--->就绪
void Creat(PCB pcb) {
if (creatLQ.size == 0) {
cout << "Error! 创建队列为空!\n";
} else {
DeQueue(&creatLQ, &pcb);
EnQueue(&readyLQ, &pcb);
if (readyLQ.size == 1) {
Dispatch(pcb);
}
printAllQueue();
}
}
//执行--->终止
void Exit (PCB pcb) {
if (runningLQ.size == 0) {
cout << "Error! 执行队列为空!\n";
} else { //创建--->就绪 就绪--->执行
DeQueue(&runningLQ, &pcb);
EnQueue(&exitLQ, &pcb);
Dispatch(pcb);
printAllQueue();
}
}
};
int main() {
LinkQueue q;
q.InitQueue(&runningLQ);
q.InitQueue(&readyLQ);
q.InitQueue(&blockLQ);
q.InitQueue(&creatLQ);
q.InitQueue(&exitLQ);
cout << "请输入创建进程的个数: ";
int num1;
cin >> num1;
cout << "请逐一输入进程名\n";
for (int i = 0; i < num1; i++) {
PCB pcb;
cout << "NO." << i + 1 << ' ';
cin >> pcb.proName;
q.EnQueue(&creatLQ, &pcb);
}
cout << "--------------------------\n";
cout << "请输入就绪进程的个数: ";
int num2;
cin >> num2;
cout << "请逐一输入进程名\n";
for (int i = 0; i < num2; i++) {
PCB pcb;
cout << "NO." << i + 1 << ' ';
cin >> pcb.proName;
q.EnQueue(&readyLQ, &pcb);
}
cout << "--------------------------\n";
cout << "请输入阻塞进程的个数: ";
int num3;
cin >> num3;
cout << "请逐一输入进程名\n";
for (int i = 0; i < num3; i++) {
PCB pcb;
cout << "NO." << i + 1 << ' ';
cin >> pcb.proName;
q.EnQueue(&blockLQ, &pcb);
}
Status status;
while(1) {
PCB pcb;
cout << "请选择情况:\n";
cout << "1: 就绪 ---> 执行\n 2: 执行--->就绪\n 3: 执行--->阻塞\n 4: 阻塞--->就绪\n 5: 创建 ---> 就绪\n 6: 执行--->终止\n 0: 退出\n";
int select;
cin >> select;
switch(select) {
case 0: return 0;
case 1: status.Dispatch(pcb); break;
case 2: status.TLE(pcb); break;
case 3: status.eventWait(pcb); break;
case 4: status.eventComplete(pcb); break;
case 5: status.Creat(pcb); break;
case 6: status.Exit(pcb); break;
default: cout << "Error! 输入错误!\n";
}
}
return 0;
}
运行结果
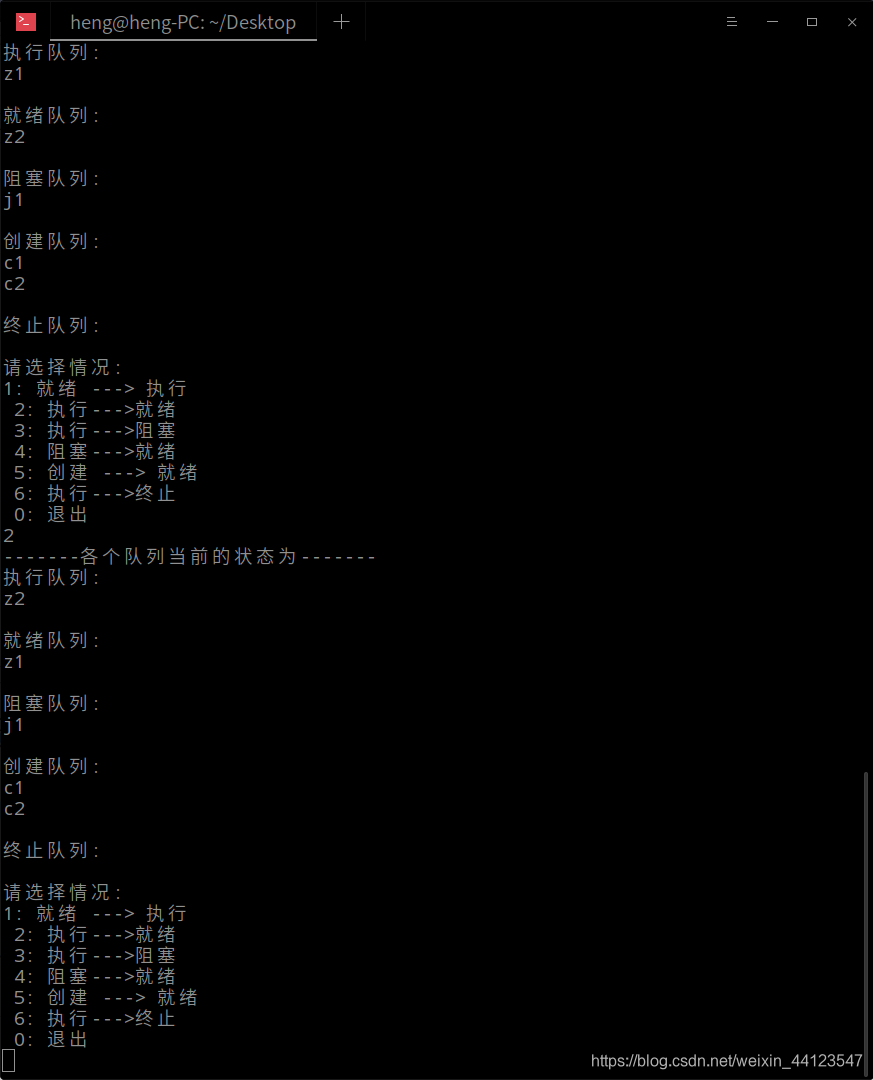