一、题目
In this question, you need to write a simple program to determine if the given chemical equation is balanced. Balanced means that the amount of elements on both sides of the “=” sign is the same.
H2O+CO2=H2CO3
We guarantees that each chemical equation satisfies the basic rules.
If you don’t know the basic rules of chemical equation writing, you can refer to the following conditions:
The chemical equation contains exactly one “=” sign.
For every chemical element, the first letter of each element is uppercase, and the rest of the letters are lowercase.
The number at the end of the element represents the amount of this element needed, If there is no number, it means only one element is needed.
Every chemical object may contain several chemical elements like NaCl.
The number at the beginning of every chemical object represents the number of this object, If there is no number, it means only one object is needed.
Every chemical object will be connected by “+” sign.
Chemical reaction is considered to rearrange the atoms after they are broken up
Input
The first line contains a single integer T(1≤T≤100), indicating the number of test cases.
In the next T lines, each line contains a string S(1≤∣S∣≤100), representing a Chemical equation.
It is guaranteed that S only contains uppercase letters, lowercase letters, “=” sign and “+” sign, the sum of the amount of all elements will not exceed 2147483647.
Output
If the given chemical equation is balanced, please output “Easy!”, otherwise output “Hard!” (Without quotes).
Sample Input
3
H2O+CO2=H2CO3
2NaHCO3=Na2CO3+H2O+CO2
3Cu+8HNO3=3CuNO3+2NO+4H2O
Sample Output
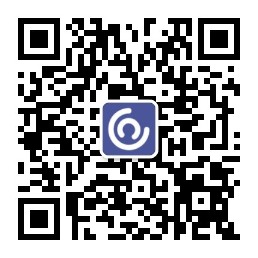
Easy!
Easy!
Hard!
二、思路
字符串的组成是 若干化合物(化学元素),’+’,‘=’这三部分。
将一个方程式分为左右两部分 -> 每部分根据’+'可分为化合物 -> 统计化合物中元素种类和个数。
三、源码
#include <iostream>
#include <string>
#include <vector>
#include <map>
using namespace std;
void div(string str, map<string, int>& mp) {
string str0, str1;
int front = 1; // 记录化合物系数
int back = 1; // 元素后跟的个数
for(int i = 0;i < str.size();) {
str0.clear(); // 每次都需要做的准备工作
str1.clear();
back = 1;
// 先取到系数
while(i < str.size() && str[i] >= '0' && str[i] <= '9') {
str0 += str[i];
i ++;
}
if(!str0.empty()) {
front = stoi(str0);
str0.clear();
}
// 取元素
if(i < str.size() && str[i] >= 'A' && str[i] <= 'Z') {
str0 += str[i];
i ++;
while(i < str.size() && str[i] >= 'a' && str[i] <= 'z') {
str0 += str[i];
i ++;
}
// 到上面元素就取完了,然后下面取它后面跟的个数
while(i < str.size() && str[i] >= '0' && str[i] <= '9') {
str1 += str[i];
i ++;
}
if(!str1.empty()) {
back = stoi(str1);
str1.clear();
}
}
// 这里元素和个数都取完了, 然后存入map
mp[str0] += front * back;
}
}
void get_elem(string str, map<string, int>& mp) {
if(!str.find('+')) {
div(str, mp);
} // 拿到了字符串, 如果没有'+', 则直接将字符串传入div()。
else {
string temp;
temp.clear();
for(int i = 0;i < str.size();) {
while(str[i] != '+' && i < str.size()) {
// 取到'+'或者字符串结尾 就结束
temp += str[i];
i ++;
}
div(temp, mp); // 把化合物传入div()
temp.clear();
if(i != str.size()) // 这里是一个条件判断,如果取到加号,下标向后移一位。如果到了字符串结尾,再向后移就不合适了 所以做一个条件判断
i ++;
}
}
}
bool match(const string& str) {
int equal_pos = str.find('='); // 找到 '=' 的位置,将其分为前后两个字符串
string str_front = str.substr(0,equal_pos);
string str_back = str.substr(equal_pos+1);
map<string, int> mp1; // 定义两个map容器,用来存储 (元素,个数)
map<string, int> mp2;
mp1.clear();
mp2.clear();
get_elem(str_front, mp1); // 该函数会解析前后字符串, 并更新map的存储
get_elem(str_back, mp2);
if(mp1 == mp2) return true; // 判断两个map中元素与个数是否想等,若相等这说明守恒
else return false; // 如果还要判断化合物的化学式是否正确 = =那就麻烦了 还是就题论题
}
int main()
{
int number;
cin >> number;
getchar(); // 吸收数字输入之后的空格
while(number --) {
string temp;
getline(cin, temp);
if(match(temp)) {
// match()函数 : 检查方程式是否正确
cout << "Easy!" << endl;
}
else {
cout << "Hard!" << endl;
}
}
return 0;
}
四、0.0
这个题想着复杂做起来倒挺简单的。我把方程式前后看为两个等价部分,所以不管前后,对字符串处理的方式都是一样的,先根据‘+’取化合物,然后取元素统计个数。当然也可以用一个map,前部分向map中加,后部分向map中减,然后判断 element.second == 0 ?
。
题目中所有测试都AC了。