一、传统Springmvc项目文件上传
web中我们用到的是 Apache fileupload这个组件来实现上传,在springmvc中对它进行了封装,让我们使用起来比较方便,但是底层还是由Apache fileupload来实现的。springmvc中由MultipartFile接口来实现文件上传。
1.pom.xml
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.2</version>
</dependency>
2.前端html页面
input的type设置为file
form表单的method设为post,
form表单的enctype设置为multipart/form-data,以二进制的形式传输数据。
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>组件库</title>
<script src="js/jquery-1.9.1.min.js"></script>
<style>
.btn {
display: inline-block;
line-height: 1;
white-space: nowrap;
cursor: pointer;
background: #fff;
border: 1px solid #dcdfe6;
text-align: center;
box-sizing: border-box;
outline: none;
margin: 0;
transition: .1s;
font-weight: 500;
color: #fff;
background-color: #409eff;
border-color: #409eff;
padding: 7px 15px;
font-size: 12px;
border-radius: 3px;
}
div {
margin: 6px;
}
/* css代码 */
.fileinput {
width: 50px;
height: 25px;
opacity: 0;
padding: 0;
margin: 0;
}
.filefont {
margin-top: -28px;
display: block;
line-height: 25px;
}
</style>
</head>
<body>
<div>
<div>
<div class="btn">
<input type="file" name="" id="upload_file_ajax" class="fileinput" onChange="importBtn(this)">
<span class="filefont">文件上传</span>
</div>
</div>
<div id="fileshow"></div>
</div>
</body>
</html>
<script type="text/javascript">
/* $(function(){
$('#send').click(function(){
$.ajax({
type: "post",
url: "/upload",
data: obj,
dataType: "file",
success: function(data){
$('#resText').empty(); //清空resText里面的所有内容
var html = '';
$.each(data, function(commentIndex, comment){
html += '<div class="comment"><h6>' + comment['username']
+ ':</h6><p class="para"' + comment['content']
+ '</p></div>';
});
$('#resText').html(html);
}
});
});
}); */
function importBtn(obj) {
var upload_file=$("#upload_file_ajax")[0].files[0];
var formData=new FormData(); // 生成FormData对象
formData.append("uploadfile",upload_file); // 组装文件类型的键值对
$.ajax({
type: "post",
url: "/upload",
data: formData,
datatype:"json",
contentType: false,
processData: false,
success: function (data) {
$('#fileshow').empty(); //清空fileshow里面的所有内容
var html ='';
html="<img src=\"img/note.png\" width=\"20px\" />"+data.filename;
$('#fileshow').html(html);
}, error: function (data) {
alert("error:" + data);
}
});
}
</script>
3.Controller层接收
使用MultipartFile对象作为参数,接收前端发送过来的文件,将文件写入本地文件中,就完成了上传操作
@RequestMapping("/upload")
public String upload(@RequestParam("uploadfile") MultipartFile file) throws IOException {
String path = "e:\\c\\";//一般会指定存储的服务器路径
// 获取原文件名
String fileName = file.getOriginalFilename();
// 创建文件实例
File filePath = new File(path, fileName);
// 如果文件目录不存在,创建目录
if (!filePath.getParentFile().exists()) {
filePath.getParentFile().mkdirs();
System.out.println("创建目录" + filePath);
}
// 写入文件
file.transferTo(filePath);
Map<String, Object> map = new HashMap<>();
map.put("result", "成功!");
map.put("filename", fileName);
//"[{\"result:\"\"success\"}]"
return JSONArray.toJSONString(map);
}
4.springmvc.xml配置CommonsMultipartResolver。
<bean id="multipartResolver"
class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<!--上传文件的最大大小,单位为字节 -->
<property name="maxUploadSize" value="17367648787"></property>
<!-- 上传文件的编码 -->
<property name="defaultEncoding" value="UTF-8"></property>
</bean>
二、Springboot项目使用文件上传
1.pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
2.controller
/**
* Apache fileupload 文件上传,springmvc中对其进行封装,底层还是fileupload。
* springmvc中由 MultipartFile 接口来实现文件上传
* @return
*/
@RequestMapping("/upload")
public String upload(@RequestParam("uploadfile") MultipartFile file) throws IOException {
String path = "e:\\c\\";//一般会指定存储的服务器路径
// 获取原文件名
String fileName = file.getOriginalFilename();
// 创建文件实例
File filePath = new File(path, fileName);
// 如果文件目录不存在,创建目录
if (!filePath.getParentFile().exists()) {
filePath.getParentFile().mkdirs();
System.out.println("创建目录" + filePath);
}
// 写入文件
file.transferTo(filePath);
Map<String, Object> map = new HashMap<>();
map.put("result", "成功!");
map.put("filename", fileName);
//"[{\"result:\"\"success\"}]"
return JSONArray.toJSONString(map);
}
3.html
基于Springboot:静态资源默认放在resource/static目录下;动态资源放啊在resource/templates目录下;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>组件库</title>
<script src="js/jquery-1.9.1.min.js"></script>
<style>
.btn {
display: inline-block;
line-height: 1;
white-space: nowrap;
cursor: pointer;
background: #fff;
border: 1px solid #dcdfe6;
text-align: center;
box-sizing: border-box;
outline: none;
margin: 0;
transition: .1s;
font-weight: 500;
color: #fff;
background-color: #409eff;
border-color: #409eff;
padding: 7px 15px;
font-size: 12px;
border-radius: 3px;
}
div {
margin: 6px;
}
/* css代码 */
.fileinput {
width: 50px;
height: 25px;
opacity: 0;
padding: 0;
margin: 0;
}
.filefont {
margin-top: -28px;
display: block;
line-height: 25px;
}
</style>
</head>
<body>
<div>
<div>
<div class="btn">
<input type="file" name="" id="upload_file_ajax" class="fileinput" onChange="importBtn(this)">
<span class="filefont">文件上传</span>
</div>
</div>
<div id="fileshow"></div>
</div>
</body>
</html>
<script type="text/javascript">
/* $(function(){
$('#send').click(function(){
$.ajax({
type: "post",
url: "/upload",
data: obj,
dataType: "file",
success: function(data){
$('#resText').empty(); //清空resText里面的所有内容
var html = '';
$.each(data, function(commentIndex, comment){
html += '<div class="comment"><h6>' + comment['username']
+ ':</h6><p class="para"' + comment['content']
+ '</p></div>';
});
$('#resText').html(html);
}
});
});
}); */
function importBtn(obj) {
var upload_file=$("#upload_file_ajax")[0].files[0];
var formData=new FormData(); // 生成FormData对象
formData.append("uploadfile",upload_file); // 组装文件类型的键值对
$.ajax({
type: "post",
url: "/upload",
data: formData,
datatype:"json",
contentType: false,
processData: false,
success: function (data) {
$('#fileshow').empty(); //清空fileshow里面的所有内容
var html ='';
html="<img src=\"img/note.png\" width=\"20px\" />"+data.filename;
$('#fileshow').html(html);
}, error: function (data) {
alert("error:" + data);
}
});
}
</script>
4.安全配置application.properties
#文件上传==============================
# 上传文件总的最大值
spring.servlet.multipart.max-request-size=10MB
# 单个文件的最大值
spring.servlet.multipart.max-file-size=10MB
## jsp
spring.mvc.view.prefix=/
spring.mvc.view.suffix=.html
效果图:
扫描二维码关注公众号,回复:
13396873 查看本文章
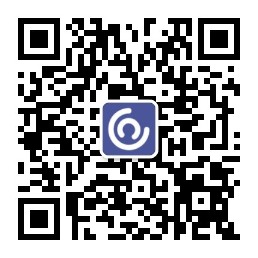
上传后: