DI依赖注入
依赖注入
DI,是dependence injection的简称,译为依赖注入,和IOC控制反转思想是一个意思,IOC是用来创建对象的思想,DI依赖注入,就是在配置Spring容器(xxx.xml文件)创建对象的同时为对象注入属性值。而依赖注入的方式有5种:Setter注入(set方法注入)、interface注入(接口注入)、constructor注入(构造注入)、autowire注入(自动注入)、Annotation注(注解注入)。
构造器注入
Set方式注入[重点]
依赖:bean对象的创建依赖于Spring容器
注入:bean对象中的所有属性,由Spring注入
搭建环境
1.Spring 基本环境
2.实体类
pojo
@Data
public class Student {
private String name;
private Address address;
private String[] books;
private List<String> hobbies;
private Map<String,String> card;
private Set<String> games;
private String wife;
private Properties info;
@Data
public class Address {
private String address;
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
3.applicationContext.xml
<beans>
<!--注册Address-->
<bean id="address" class="com.my.Address">
<property name="address" value="天津"/>
</bean>
<!--注册Student-->
<bean id="student" class="com.my.Student">
<property name="name" value="张三"/>
<!--Bean注入,ref-->
<property name="address" ref="address"/>
<!--数组-->
<property name="books">
<array>
<value>水浒传</value>
<value>西游记</value>
</array>
</property>
<!--集合-->
<property name="hobbies">
<list>
<value>打篮球</value>
<value>看电视</value>
</list>
</property>
<!--map-->
<property name="card">
<map>
<entry key="身份证" value="23142352346254"/>
<entry key="银行卡" value="235234236"/>
</map>
</property>
<!--set-->
<property name="games">
<set>
<value>gta5</value>
<value>lol</value>
<value>王者荣耀</value>
</set>
</property>
<!--properties-->
<property name="info">
<props>
<prop key="学号">423805345</prop>
<prop key="id">2523456234</prop>
</props>
</property>
<!--设空值-->
<property name="wife">
<null/>
</property>
</bean>
</beans>
4.Test
public static void main(String[] args) {
ApplicationContext pathXmlApplicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
Student student = (Student) pathXmlApplicationContext.getBean("student");
student.getName();
student.getAddress();
student.getBooks();
student.getHobbies();
student.getCard();
student.getGames();
student.getInfo();
student.getWife();
System.out.println(student);
}
拓展方式注入
p命名空间和c命名空间不能直接使用,需要导入官方头文件约束
p命名空间注入方式
p命名空间对应的是set方式注入
必须要有无参构造器(默认)
头文件约束
xmlns:p="http://www.springframework.org/schema/p"
演示
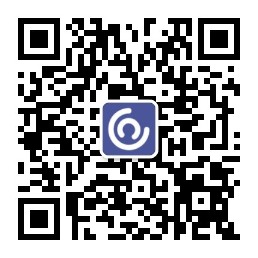
<!--property 可以直接注入属性的值-->
<bean id="user" class="com.my.User" p:name="张三" p:age="34"/>
c命名空间注入方式
c命名空间对应的是constructor方式注入
必须要有参构造器
头文件约束
<!--constructor-arg可以通过构造器注入-->xmlns:c="http://www.springframework.org/schema/c"
演示
<bean id="user1" class="com.my.User" c:name="张三" c:age="32"/>
自动注入
自动装配是Spring满足bean依赖的一种方式
Spring会在上下文中自动寻找,并自动给bean装配属性。
在Spring中有三种装配的方式
1.在xml中显示的配置
2.在java中显示配置
3.隐式的自动装配bean【重要】
一.byName
autowire="byName"会自动在容器上下文中找,和自己对象set方法的属性名(setCat(){})名字对应的beanId(id=“cat”)。
注意:id要和set后属性名一致。
<bean id="dog" class="com.my.pojo.Dog"/>
<bean id="cat" class="com.my.pojo.Cat"/>
<bean id="person" class="com.my.pojo.Person" autowire="byName">
<property name="name" value="张三"/>
</bean>
二.byType
byType会在 容器上下文中查找,和自己对象属性类型相同的bean。
注意:必须要保证类型全局唯一。
总结:实现二者前提是,类型都需要现在beans中注册。
注解注入( @Autowired)
使用注解前提:
1.导入约束
xmlns:context="http://www.springframework.org/schema/context"
======================================
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
2.配置注解的支持【重要】
<context:annotation-config/>
3.注意:最终还是通过byName实现,要注意实体类属性名和beanId一致。
@Qualifier可指定beanId
实例:
@Autowired直接在属性上使用即可,也可以在set方法上使用。(亦可忽略set方法因为注解是经过反射获取的属性)
@Data
public class Person {
//Cat和Dog只有输出语句,就不粘贴了
@Autowired
private Cat cat;
@Autowired
private Dog dog;
private String name;
}
application.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
<!--开启注解支持-->
<context:annotation-config/>
<bean id="dog" class="com.my.pojo.Dog"/>
<bean id="cat" class="com.my.pojo.Cat"/>
<bean id="person" class="com.my.pojo.Person">
<property name="name" value="张三"/>
</bean>
</beans>
Test
@Test
public void test1(){
ApplicationContext pathXmlApplicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
Person person = pathXmlApplicationContext.getBean("person", Person.class);
System.out.println(person.getName());
person.getCat().shot();
person.getDog().shot();
}
运行结果:
注解注入(@Resource)
@Resource是java层面的注解,也可实现自动装配。并且是一组合注解,@Resource(name=“beanId”)指定beanId
小结:
@Autowired和@Resource的区别,都可以实现自动装配,都可以放在属性字段上
@Autowired通过byName的方式实现。
@Resource默认通过byName的方式实现,找不到就通过byType。
扯弹的知识
@Nullable:字段标记了这个注解,说明这个字段可以为null;
如果显示定义了Autowired的required属性为false,说明这个对象可以为null,否则不允许为空