(公司内部使用,本文为学习笔记)
-
-
-
- 1、通过配置权限(接口请求:无权限访问)隐藏功能
- 2、编辑和新增的内容写在同一个弹窗(.$api[url](params))
- 3、map将数组变成数组对象,反之亦然
- 4、vue-router query和params传参(接收参数)$router $route的区别
- 5、使用map将一维数组变为二维数组,且label与value的值相同;
- 6、vue模板
- 7、单文件组件和组件
- 8、将一维数组变成二维数组,
- 9、elementUI滚动条
- 10、Object.values(obj)
- 11、默认标段
- 12、数组变成字符串、字符串分割
- 13、iview-form输入监听。级联组件配置
- 14、setForm()
- 15、HTMl 中marquee标签实现无缝滚动跑马灯效果
- 16、JS文件中引入接口,使用vuex的store以及路由跳转判断位置
- 17、点击菜单栏默认第一个路由
-
-
1、通过配置权限(接口请求:无权限访问)隐藏功能
//$getPermissions('url')
v-if="$getPermissions('data/PavingRolling/index')"
2、编辑和新增的内容写在同一个弹窗(.$apiurl)
//接口文件js
import request from '@/utils/request'
// 新增
export function warningCreate(data) {
return request({
url: 'data!data/WarningSetting/create',
method: 'post',
data
})
}
// 编辑
export function warningEdit(data) {
return request({
url: 'data!data/WarningSetting/edit',
method: 'post',
data
})
}
let url, params = {
...form, ...{
settings: this.tableLookData } }
if (this.buttonType === 'add') {
url = 'warningCreate'
} else {
url = 'warningEdit'
}
//[url]取的是属性值。这里等同于this.$api.warningCreate(params)
await this.$api[url](params)
this.$success(`${
this.buttonType === 'add' ? '新增' : '编辑'}成功`)
this.getData()
3、map将数组变成数组对象,反之亦然
后端给一维数组时下拉框回显需要二维
let recipe_title = ["value1,value2,value3]
recipe_title = res.result.recipe_title.map((item) => {
return {
text: item,
value: item
}
})
console.log(recipe_title)
//recipe_title[{text: value1,value: value1},{text: value2,value: value2},{text: value3,value: value3}
4、vue-router query和params传参(接收参数)$router $route的区别
//使用this.$route.query.属性获取路由参数
this.tabName = this.$route.query.tabName ? this.$route.query.tabName : 'video'
1、 this.$router.push进行编程式路由跳转
2、 router-link 进行页面按钮式路由跳转
3、 this.$route.params获取路由传递参数
4、this.$route.query获取路由传递参数
5、 params 和 query 都是传递参数的,params不会在url上面出现,并且params参数是路由的一部分,是一定要存在的
query则是我们通常看到的url后面的跟在?后面的显示参数
5、使用map将一维数组变为二维数组,且label与value的值相同;
Array.from(new Set(arr))数组去重
//登录地址
let arr = this.tableData.map(item => item.login_url)
arr.push("https://hhy.xatasoft.com/serv/user/Login/in","http://bim2.xatasoft.com/serv/user/Login/in","https://dcp.xatasoft.com/serv/user/login/in","http://gljs.xatasoft.com/serv/user/Login/in","http://kh205.xatasoft.com/#/login")
this.formList[1].options = Array.from(new Set(arr)).map(val => {
// Array.from(new Set(arr))
return {
label: val,
value: val,
}
})
6、vue模板
<!-- -->
<template lang="pug"> </template>
<script>
export default {
components: {
},
data() {
return {
}
},
computed: {
},
watch: {
},
methods: {
},
created() {
},
mounted() {
},
}
</script>
<style lang="scss" scoped> </style>
7、单文件组件和组件
两个其实是一个东西,但一般一个单文件组件作为一个页面时,通过路由展示该页面,需要全局注册。当一个单文件组件作为一个页面的组件时,既可以全局注册,也可以局部祖册。选项:template、data、computed,但data必须是个函数,且要return出去。
7-1、路由注册
//使用vue-cli构建项目后,我们会在Router文件夹下面的index.js里面引入相关的路由组件
import Vue from "vue";
import VueRouter from "vue-router";
import index from "../views/index.vue"; //方法一、普通加载。缺点:webpack在打包的时候会把整个路由打包成一个js文件,如果页面一多,会导致这个文件非常大,加载缓慢
const layout = resolve => import("../views/layout/layout.vue")
Vue.use(VueRouter);
{
path: "/login",
name: "login",
component: () => import"../views/login.vue"), //vue异步组件技术。在router中配置,使用这种方法可以实现按需加载,一个组件生成一个js文件
meta: {
keepAlive: false
}
},
const routes = [ //动态的import()语法(推荐使用这种)
{
path: "/index",
name: "index",
component: index,
meta: {
keepAlive: false
}
},
{
path: "/login",
name: "login",
component: () => import"../views/login.vue"),
meta: {
keepAlive: false
}
},
{
path: '/layout',
name: 'layout',
component: layout,
children: [
{
path: '/investmentProgress',
name: 'investmentProgress',
component: () => import("../views/investment-progress/investment-progress.vue"),
meta: {
title: '投资进度' }
},
//lfj
{
path: '/managementSettings',
name: 'managementSettings',
component: () => import("../views/project-manage/management-settings.vue"),
meta: {
title: '项管设置' }
},
]
},
{
path: '*',
redirect:'/index'
}
];
const router = new VueRouter({
routes
});
export default router;
7-2、组件局部注册
<!-- -->
<template lang="pug">
</template>
<script>
export default {
name:'management-settings',
components: {
//局部注册
projectNav,
processTree,
},
data() {
return {
}
},
computed: {
},
watch: {
},
methods: {
},
created() {
},
mounted() {
},
}
</script>
<style lang="scss" scoped>
</style>
7-2、组件全局注册
import XtUpload from '../xt-upload'
import XtTree from '../xt-tree'
import XtSearch from '../xt-search'
import XtExport from '../xt-export'
export const install = (Vue) => {
Vue.component(XtUpload.name, XtUpload)
Vue.component(XtTree.name, XtTree)
Vue.component(XtSearch.name, XtSearch)
Vue.component(XtExport.name, XtExport)
export {
XtTree,
XtSearch,
XtUpload,
XtExport,
}
export default install
8、将一维数组变成二维数组,
let aa = [1,2,3,4,5,6,7]
let bb = this.group(aa, 3)
function group(array, bbLen) {
let index = 0;
let newArray = [];
while (index < array.length) {
newArray.push(array.slice(index, (index += bbLen)));
}
return newArray;
}
console.log(bb)
//(3) [Array(3), Array(3), Array(1)]
//0: (3) [1, 2, 3]
//1: (3) [4, 5, 6]
//2: [7]
扫描二维码关注公众号,回复:
13345132 查看本文章
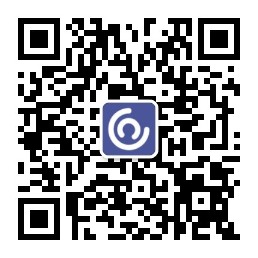
9、elementUI滚动条
.scrollbar-box
el-scrollbar.verticalScroll(style="height:100%;")
.onlineBox
el-row
el-col(:span="2" align="center")
i.iconfont.icon-biaoji
el-col(:span="10")
.name 沪杭甬北段钱塘江大桥顶推推进会
el-col(:span="6" align="center")
.status 会议结束
el-col(:span="6" align="center")
.time 2020-11-12
.onlineBox
el-row
el-col(:span="2" align="center")
i.iconfont.icon-biaoji
el-col(:span="10")
.name 杭淳开项目专题推进会
el-col(:span="6" align="center")
.status 会议结束
el-col(:span="6" align="center")
.time 2020-11-8
.onlineBox
el-row
el-col(:span="2" align="center")
i.iconfont.icon-biaoji
el-col(:span="10")
.name 杭淳开项目专题推进会
el-col(:span="6" align="center")
.status 会议结束
el-col(:span="6" align="center")
.time 2020-11-8
10、Object.values(obj)
var obj = {
foo: 'bar', baz: 42 };
console.log(Object.values(obj)); // ['bar', 42]
11、默认标段
import {
mapGetters } from 'vuex'
computed: {
...mapGetters([ 'currentProject']),
},
this.paragraph_id = this.currentProject.id //标段:必选(给默认值)
12、数组变成字符串、字符串分割
13、iview-form输入监听。级联组件配置
if(item.type === 'cascader' || item.name === 'class_id') {
let obj = {
title: item.label,
type: 'cascader',
key: item.name,
defaultValue: [],
isShow: item.is_show || this.isShow,
rule: {
required: item.is_require, message: item.placeholder, trigger: 'blur'},
props: {
props: {
text: 'label',
value: 'id',
checkStrictly: true,
},
placeholder: `请选择${
item.label}`
},
options: this.treeData,
onInput: function(value, data, form) {
form[data.key] = value
vm.$set(form, data.key, value)
}
}
// this.searchList[0].children.splice(index,0,obj)//将搜索条件插入
return obj
}
14、setForm()
分开赋值
edit(row,type) {
//row 为完整数据,需分开赋值
for(let item of this.formListA1) {
this.$refs.basicForm.setForm({
//表单1
[item.key]: row[item.key]
})
}
for(let item of this.formListA2) {
//表单2
this.$refs.basicForm.setForm({
[item.key]: row[item.key]
})
}
}
//传值要传键值对的形式
this.$refs.fileForm.setForm({
enclosure: row.enclosure})
15、HTMl 中marquee标签实现无缝滚动跑马灯效果
marquee.projectAbout(onMouseOut="this.start()" onMouseOver="this.stop()" style="height: 166px;" behavior="scroll" direction="up" scrollamount="3" @click="$refs.projectAbout.open()")
16、JS文件中引入接口,使用vuex的store以及路由跳转判断位置
JS文件中引入接口
//引入
import {
getDogCode } from '@/api/safety-production/environmental-monitoring'
//使用
const res = await getDogCode(data)
从store里拿currentProject.id
import store from './store'//
if (to.path === '/login') {
// next({ path: '/cockpit/index?sign=0' })
next({
path: '/' })
NProgress.done()
} else if (to.path === '/safetyProduction/mechanicalEquipmentMonitoring') {
window.open('https://login.zhgcloud.com/login-token?token=R1g3RHhiNU11a1ByWXpWZ0lYeXlZUG9HNEcrQ2dZemlJQTVOd3I4dVoyWT0')
next()//继续切换路由(页面)
NProgress.done()
} else if (to.path === '/safetyProduction/safetyHelmet') {
store._vm.currentProject.id //store同等于 computed: {...mapGetters(['userinfo', 'projects', 'currentProject',]),}后 this.currentProject.id
let _this = this
const data = {
projectid: store._vm.currentProject.id
}
const res = await getDogCode(data)
let url = 'https://igh.zolbon.com/#/auth/login?code=' + res.result.code
window.open(url)
NProgress.done()
}
17、点击菜单栏默认第一个路由
//将store里的clickTab改为true,取消默认模块第一个路由
this.$store.commit('SET_CLICK_TAB', true)
this.$router.push({
path: '/officeManage/notice' })
if (v && v.length) {
if (this.clickTab) return
let item = this.getFirstRoute(v[0])
this.$store.commit('SET_ROUTE', item)
this.$store.commit('SET_CURRENT_ROUTE', item.path)
if (this.$route.path != '/dashboard') {
this.$router.push({
path: item.path
})
}
}