效果
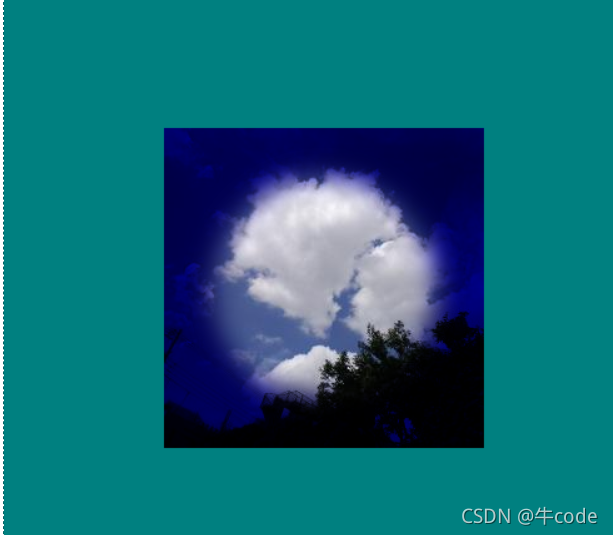
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="/static/css/common.css">
<title>16.使用多幅纹理</title>
</head>
<body onload="main()">
<canvas id="example" width="512" height="512">
游览器不支持
</canvas>
</body>
<script src="/static/js/cuon-utils.js"></script>
<script src="/static/js/webgl-debug.js"></script>
<script src="/static/js/webgl-utils.js"></script>
<script>
// 顶点着色器
var vertex_shader_source = '' +
'attribute vec4 a_Position;' +
'attribute vec2 a_TexCoord;' +
'varying vec2 v_TexCoord;' +
'void main() {' +
' gl_Position = a_Position;' +
' gl_PointSize = 10.0;' +
' v_TexCoord = a_TexCoord;' +
'}';
// 片元着色器
var fragment_shader_source = '' +
'precision mediump float;' +
'uniform sampler2D u_Sampler0;' +
'uniform sampler2D u_Sampler1;' +
'varying vec2 v_TexCoord;' +
'void main(){' +
' vec4 color0 = texture2D(u_Sampler0,v_TexCoord);' +
' vec4 color1 = texture2D(u_Sampler1,v_TexCoord);' +
' gl_FragColor = color0*color1;' +
'}';
function main() {
var canvas = document.getElementById('example');
if(canvas==null){
alert("获取失败")
}
// 获取webgl 上下文对象
var gl = getWebGLContext(canvas);
// 初始化着色器
if (!initShaders(gl, vertex_shader_source, fragment_shader_source)) {
console.log('初始化着色器失败');
}
// 设置顶点位置
var n = initVertexBuffer(gl);
if (n < 0) {
console.log('顶点写入缓存失败!');
return false;
}
//指定清空<canvas>颜色,就是指定canvas的背景色,第四个参数是透明度,范围是(0-1)
gl.clearColor(0.0,0.5,0.5,1.0)
// S配置纹理
if (!initTextures(gl, n)) {
console.log('Failed to intialize the texture.');
return;
}
}
// 将顶点信息写入缓存区
function initVertexBuffer(gl) {
var vertices = new Float32Array([
-0.5, 0.5, 0.0,1.0,
-0.5, -0.5, 0.0,0.0,
0.5, 0.5, 1.0,1.0,
0.5, -0.5, 1.0,0.0,
]);
var n = 4;
// 创建缓冲区对象
var vertexBuffer = gl.createBuffer();
if (!vertexBuffer) {
console.log('创建缓冲区对象失败!');
return -1;
}
// 绑定缓冲区对象到目标
gl.bindBuffer(gl.ARRAY_BUFFER, vertexBuffer);
// 将数据写入到缓冲区对象
gl.bufferData(gl.ARRAY_BUFFER, vertices, gl.STATIC_DRAW);
var FSIZE = vertices.BYTES_PER_ELEMENT;
var a_Position = gl.getAttribLocation(gl.program, 'a_Position');
if (a_Position < 0) {
console.log('获取attribute变量失败!');
return -1;
}
// 将缓冲区对象分配给attribute变量
gl.vertexAttribPointer(a_Position, 2, gl.FLOAT, false, FSIZE * 4, 0);
// 开启attribute变量
gl.enableVertexAttribArray(a_Position);
var a_TexCoord = gl.getAttribLocation(gl.program, 'a_TexCoord');
if (a_TexCoord < 0) {
console.log('获取attribute变量失败!');
return -1;
}
// 将缓冲区对象分配给attribute变量
gl.vertexAttribPointer(a_TexCoord, 2, gl.FLOAT, false, FSIZE * 4, FSIZE * 2);
// 开启attribute变量
gl.enableVertexAttribArray(a_TexCoord);
return n;
}
function initTextures(gl, n) {
var texture = gl.createTexture(); // Create a texture object
var texture1 = gl.createTexture(); // Create a texture object
if (!texture) {
console.log('Failed to create the texture object');
return false;
}
if (!texture1) {
console.log('Failed to create the texture object');
return false;
}
// Get the storage location of u_Sampler
var u_Sampler = gl.getUniformLocation(gl.program, 'u_Sampler0');
var u_Sampler1 = gl.getUniformLocation(gl.program, 'u_Sampler1');
if (!u_Sampler) {
console.log('Failed to get the storage location of u_Sampler');
return false;
}
if (!u_Sampler1) {
console.log('Failed to get the storage location of u_Sampler');
return false;
}
var image = new Image(); // Create the image object
var image1 = new Image(); // Create the image object
if (!image||!image1) {
console.log('Failed to create the image object');
return false;
}
// Register the event handler to be called on loading an image
image.onload = function(){
loadTexture(gl, n, texture, u_Sampler, image,0); };
image1.onload = function(){
loadTexture(gl, n, texture1, u_Sampler1, image1,1); };
// Tell the browser to load an image
image.src = '../static/img/sky.jpg';
image1.src = '../static/img/circle.gif';
return true;
}
//标记纹理单元是否已经就绪
var g_texUnit0 = false,g_texUnit1=false;
function loadTexture(gl, n, texture, u_Sampler, image,texUnit) {
gl.pixelStorei(gl.UNPACK_FLIP_Y_WEBGL, 1); // Flip the image's y axis
//激活纹理
if(texUnit==0){
// Enable texture unit0
gl.activeTexture(gl.TEXTURE0);
g_texUnit0 = true;
}else{
gl.activeTexture(gl.TEXTURE1);
g_texUnit1 = true;
}
// Bind the texture object to the target
gl.bindTexture(gl.TEXTURE_2D, texture);
// Set the texture parameters
gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_MIN_FILTER, gl.LINEAR);
// Set the texture image
gl.texImage2D(gl.TEXTURE_2D, 0, gl.RGB, gl.RGB, gl.UNSIGNED_BYTE, image);
// Set the texture unit 0 to the sampler
gl.uniform1i(u_Sampler, texUnit);
gl.clear(gl.COLOR_BUFFER_BIT); // Clear <canvas>
if(g_texUnit0 && g_texUnit1){
gl.drawArrays(gl.TRIANGLE_STRIP, 0, n); // Draw the rectangle
}
}
</script>
</html>