手动构建二叉树,并实现两种遍历方式
如下图,链表的每一个节点是由data 和next 指针构成。
树的每个节点,每个点都是由两个next 指针构成。
下面开始模拟构建树的节点
先创建 二叉树对象 类 TreeNode class
/**
* 二叉树的节点
*/
public class TreeNode{
private Integer data;
private TreeNode left; //左节点
private TreeNode right; //右节点
public TreeNode(Integer data){
this.data = data;
}
//set get 方法
public Integer getData(){
return data;
}
public void setData(Integer data){
this.data = data;
}
public TreeNode getLeft(){
return left;
}
public void setData(TreeNode left){
this.left = left;
}
public TreeNode getRight() {
return right;
}
public void setRight(TreeNode right) {
this.right = right;
}
}
上边内容是用来创建 TreeNode 对象,main测试及两种遍历在下面。
下面,创建 MainTest 来测试一下
public static void main(String[] args) {
//创建7个节点
TreeNode root = new TreeNode(1);
TreeNode node2 = new TreeNode(2);
TreeNode node3 = new TreeNode(3);
TreeNode node4 = new TreeNode(4);
TreeNode node5 = new TreeNode(5);
TreeNode node6 = new TreeNode(6);
TreeNode node7 = new TreeNode(7);
//将这些节点够成一个二叉树
root.setLeft(node2);
root.setRight(node3);
node2.setLeft(node4);
node2.setRight(node5);
node3.setLeft(node6);
node3.setRight(node7);
/**
* 构建成的二叉树 如下图
*
* [1]
* [2] [3]
* [4] [5][6] [7]
*
* // 树的遍历方式
* 1. 深度遍历 : 先序遍历(根左右)、中序遍历(左根右)、后续遍历(左右根)
* 先序遍历 1245367
* 中序遍历 4251637
* 后续遍历 4526731
* 2. 广度遍历 : 1 2 3 4 5 6 7
*
* */
//下面是测试一下这两种 遍历方式 广度遍历和深度遍历
System.out.println("按照广度遍历:");
printByLayer(root); //输出结果: 1 2 3 4 5 6 7
System.out.println("深度优先遍历的三种方式:")
System.out.println("先序遍历:");
pre_print(root);
System.out.println("");
System.out.println("中序遍历:");
mid_print(root);
System.out.println("");
System.out.println("后序遍历:");
last_print(root);
}//mian
//*******************************************************
/**
* 二叉树树的遍历方式一: 广度遍历(利用队列,先进先出原则) 就是按层遍历
* @param root
*/
//*******************************************************
public static void printByLayer(TreeNode root){
if (root == null) {
return;
}
//创建一个队列,来存储节点 : 队列的特点 先进先出原则
Queue<TreeNode> queue = new LinkedList<>();
queue.add(root); //把根节点 存到队列中
while(!queue.isEmpty()){
//如果队列非空,继续循环
//从队列中取出一个数据
TreeNode temp = queue.poll(); //从这里 输出结果
System.out.println(temp.getData());
if(temp.getLeft() != null){
queue.add(temp.getLeft()); //把左孩子添加到 队列中
}
if (temp.getRight() != null){
queue.add(temp.getRight()); //把右孩子添加到 队列中
}
}//while
}//printByLayer
//*******************************************************
/**
* 二叉树树的遍历方式二: 深度遍历(先序遍历、中序遍历、后续遍历)
* @param root
* 二叉树中经常会使用到 递归,
*/
//*******************************************************
/**
* 深度遍历 -- 先序遍历
*/
public static void pre_print(TreeNode root) {
if (root != null) {
//节点不空,才输出
//根
System.out.print(root.getData());
System.out.print(" ");
//左
if (root.getLeft() != null) {
pre_print(root.getLeft());
}
//右
if (root.getRight() != null) {
pre_print(root.getRight());
}
}
}//pre_print()
/**
* 中序遍历
*/
public static void mid_print(TreeNode root) {
if (root != null){
//左
if (root.getLeft()!=null){
mid_print(root.getLeft());
}
//根
System.out.print(" ");
System.out.print(root.getData());
//右
if (root.getRight()!=null){
mid_print(root.getRight());
}
}
}//mid_print()
/**
* 后续遍历
* */
public static void last_print(TreeNode root){
if (root!=null){
//左
if (root.getLeft()!=null){
last_print(root.getLeft());
}
//右
if (root.getRight()!=null){
last_print(root.getRight());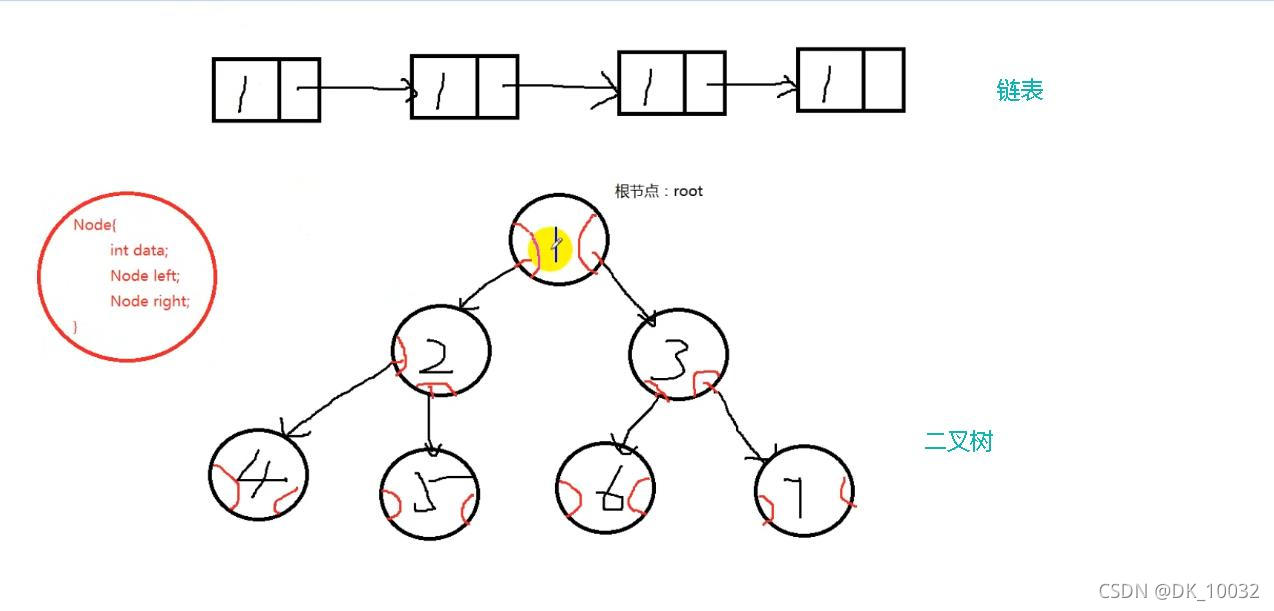
}
//根
System.out.print(root.getData());
System.out.print(" ");
}
}//last_print()