1. tensorflow安装
- 安装tensorflow:
pip3 install tensorflow==1.11.0
安装后报错DLL load failed,原因是protobuf的版本不匹配,pip install --user protobuf==3.6.0后错误消失。–user是因为pip安装时报错PermissionError: [WinError 5] 拒绝访问。
- 安装CUDA
import tensorflow时报错Could not load dynamic library 'cudart64_101.dll,原因是CUDA安装失败,CUDA是GPU的并行计算框架。与tensorflow_gpu配套使用,需要注意二者的版本对应
2.安装与卸载中的问题
- 安装了tensorflow-gpu之后,发现本机没有gpu可以使用,想要卸载时,报错,更新完pip(python -m pip install --upgrade pip)后再卸载成功
Could not install packages due to an EnvironmentError: [Errno 2] No such file or directory: 'C:\\Users\\ADMINI~1\\AppData\\Local\\Temp\\pip-uninstall-i11j_cat\\users\\administrator\\appdata\\local\\programs\\python\\python36\\lib\\site-packages\\tensorflow\\include\\tensorflow\\include\\external\\eigen_archive\\eigen\\src\\iterativelinearsolvers\\leastsquareconjugategradient.h'
3. tf-变量与常量
tf可以采用不同的方式创建常量、变量,需要指定数据类型与维度
a = tf.constant(2, tf.int16) # 常量
label = tf.Variable(
tf.ones([4,1]), # 生成4行1列,值为1的数组
name='label')
# 从截断的正态分布中随机抽取的值的张量,其中下上限是标准偏差的两倍
w1 = tf.Variable(tf.truncated_normal([256 * 256, 10]))
# 从正态分布中随机抽取
w2 = tf.Variable(
tf.random_normal(
[4, 3], # 数据维度
0.0, # 正态分布的均值
0.01), # 正态分布的标准差
name='feature_embeddings')
4. tf-会话
tf创建变量后,变量需要在session(会话)中初始化后才能使用
f = tf.Variable(8, tf.float32)
with tf.Session() as session:
tf.global_variables_initializer().run()
print(f) # <tf.Variable 'Variable_2:0' shape=() dtype=int32_ref>
print(session.run(f)) # 8
5. tf-占位符
占位符,不传入输入数据,仅分配内存,建立session后,通过feed_dict()函数向占位符传入数据
def calculate_eucledian_distance(point1, point2):
"""
计算两点的欧式距离
:param point1:
:param point2:
:return:
"""
difference = tf.subtract(point1, point2)
power2 = tf.pow(difference, tf.constant(2.0, shape=(1,2)))
add = tf.reduce_sum(power2)
eucledian_distance = tf.sqrt(add)
return eucledian_distance
list_of_points1_ = [[1,2], [3,4], [5,6], [7,8]]
list_of_points2_ = [[15,16], [13,14], [11,12], [9,10]]
list_of_points1 = np.array([np.array(elem).reshape(1,2) for elem in list_of_points1_])
list_of_points2 = np.array([np.array(elem).reshape(1,2) for elem in list_of_points2_])
point1 = tf.placeholder(tf.float32, shape=(1, 2))
point2 = tf.placeholder(tf.float32, shape=(1, 2))
dist = calculate_eucledian_distance(point1, point2)
with tf.Session() as session:
tf.global_variables_initializer().run()
for ii in range(len(list_of_points1)):
point1_ = list_of_points1[ii]
point2_ = list_of_points2[ii]
feed_dict = {
point1: point1_, point2: point2_}
distance = session.run([dist], feed_dict=feed_dict)
print("the distance between {} and {} -> {}".format(point1_, point2_, distance))
【补充】
tf的常用函数
tf.pow() # 指数乘积
tf.reduce_sum() # 在某一维度上求和
b = tf.nn.embedding_lookup( # 选取一个张量中索引对应的元素
weights, # 张量表
[1, 3] # 指定的索引,选取索引为1,3的元素值
)
# tf.nn.dropout():减轻过拟合,在不同的训练过程中随机扔掉一部分神经元,即:让某个神经元的激活值以一定的概率p,停止工作,本次训练过程中不更新权值,也不参加神经网络的计算。train时使用
g = tf.nn.dropout(
weights,
keep_prob=0.8 # 保留的概率
)
6. tf-训练
测试和验证数据集可以放在tf.constant()中。而训练数据集被放在tf.placeholder()中,这样它可以在训练期间分批输入(batch)(随机梯度下降)。
训练集在执行反向传播时需要用到梯度下降算法,当使用所有样本进行训练时,训练时间大大增加。因此,每次训练使用batch_size个数据进行参数寻优。
对于时间序列的数据集,模型的输入格式为[batch_size, seq_length, input_dim], 其中,batch_size表示一个batch中的样本的个数,seq_length表示序列的长度,input_dim表示输入样本的维度。关于batch_size对训练的影响参见:https://blog.csdn.net/qq_36588760/article/details/105267896
一个batch中应该包含的数据个数为batch_size * seq_length,那么迭代次数iterations= data_length//(batch_size * seq_length),即利用numpy中的矩阵技巧,先将序列reshpe成[batch_size, seq_length* iterations]
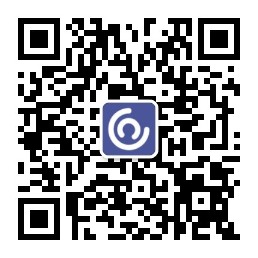
import numpy as np
batch_size = 4
seq_length = 3
raw_data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13,
14, 15, 16, 17, 18, 19, 20, 21, 22,
23, 24, 25, 26, 27, 28, 29, 30,
31, 32, 33, 34, 35, 36, 37, 38, 39, 40]
def get_batch(raw_data, batch_size, seq_length):
data = np.array(raw_data)
data_length = data.shape[0]
iterations = (data_length - 1) // (batch_size * seq_length)
round_data_len = iterations * batch_size * seq_length
xdata = data[:round_data_len].reshape(batch_size, iterations*seq_length)
ydata = data[1:round_data_len+1].reshape(batch_size, iterations*seq_length)
for i in range(iterations):
x = xdata[:, i*seq_length:(i+1)*seq_length]
y = ydata[:, i*seq_length:(i+1)*seq_length]
print(x, y)
7. tensorflow应用示例
参见:https://github.com/zhaozhengcoder/Machine-Learning/tree/master/tensorflow_tutorials
包括采用tensorflow实现线性回归、RNN、CNN等算法的应用示例。
8. 张量(Tensor)
参考:https://zhuanlan.zhihu.com/p/48982978
在学习tensorflow的时候,对张量的概念不是很清楚,实质上,张量是更高维度的向量,但光凭想象有点费力,上述链接的讲解蛮清晰的。