python类构造函数作为另个函数的__init__参数。
class MyOdlHttp():
# username = ''
# password = ''
def __init__(self, username, password):
self.haha = username
self.passw = password
print(username)
print(self.haha)
my_old_http = MyOdlHttp('admin', '123')
print(my_old_http)
print(my_old_http.haha)
print(my_old_http.passw)
print('=====================')
class Test():
def __init__(self,input):
self.output = MyOdlHttp('admin', '123')
aa = Test('ass').output
print(aa.haha)
print(aa.passw)
#out = Test(MyOdlHttp('admin','123'))
print('=====================')
admin
admin
<__main__.MyOdlHttp object at 0x7fd1ea3f9350>
admin
123
=====================
admin
admin
admin
123
=====================
python delete()
motorcycles = ['honda', 'yamaha', 'suzuki']
print(motorcycles)
del motorcycles[0]
print(motorcycles)
['honda', 'yamaha', 'suzuki']
['yamaha', 'suzuki']
python setattr()函数
class Person:
name = "John"
age = 36
country = "Norway"
setattr(Person, 'age', 40)
print(Person.age)
print(Person().age)
40
40
class Config():
a = 1
b = 2
print(Config.a)
print(Config().a)
1
1
python assert()
#price 为原价,discount 为折扣力度
def apply_discount(price, discount):
updated_price = price * (1 - discount)
#assert 0 <= updated_price <= price, '折扣价应在 0 和原价之间'
assert 0 <= updated_price <= price
return updated_price
print(apply_discount(100,0.2))
print(apply_discount(100,1.1))
80.0
---------------------------------------------------------------------------
AssertionError Traceback (most recent call last)
<ipython-input-29-bf98d6e6aa6b> in <module>()
6 return updated_price
7 print(apply_discount(100,0.2))
----> 8 print(apply_discount(100,1.1))
<ipython-input-29-bf98d6e6aa6b> in apply_discount(price, discount)
3 updated_price = price * (1 - discount)
4 #assert 0 <= updated_price <= price, '折扣价应在 0 和原价之间'
----> 5 assert 0 <= updated_price <= price
6 return updated_price
7 print(apply_discount(100,0.2))
AssertionError:
print(1)
assert 1 == 2
print(2)
1
---------------------------------------------------------------------------
AssertionError Traceback (most recent call last)
<ipython-input-30-ca408cea71f3> in <module>()
1 print(1)
----> 2 assert 1 == 2
3 print(2)
AssertionError:
python arg、*args、**kwargs
def function(arg,*args,**kwargs):
print(arg,args,kwargs)
function(6,7,8,9,a=1, b=2, c=3)
6 (7, 8, 9) {'a': 1, 'b': 2, 'c': 3}
关于类:
class PredictGlaucoma():
"""主控制程序"""
def __init__(self, args):
self.a = args
def initial_disc(self, args):
# disc params
da = 8
self.dp = da
self.out = 9
p = PredictGlaucoma('2')
print(p.a)
print(p.initial_disc('3'))
print(p.dp)
print(p.out)
2
None
8
9
>>>class A(object):
... bar = 1
...
>>> a = A()
>>> getattr(a, 'bar') # 获取属性 bar 值
1
>>> setattr(a, 'bar', 5) # 设置属性 bar 值
>>> a.bar
5
>>>class A():
... name = "runoob"
...
>>> a = A()
>>> setattr(a, "age", 28)
>>> print(a.age)
28
>>>
class EllipseParams():
"""椭圆相关参数(用于视盘和视杯)"""
def __init__(self, mode) -> None:
self.mode = mode
self.status = -1 # 状态位
self.dic_status = None # 状态位之间,描述与数值间的映射
# self.initial_to_blank()
# self.thickness = (1 if self.mode == 'test'
# else int(cfg_disc['thickness']))
# self.is_visible = False # 是否检测出视盘(或视杯)
# self.model = None
# self.pred_path = None # 视盘(或视杯)的预测图路径
# self.gray_path = None # 视盘(或视杯)的灰度图路径
# self.path_miss = None # 未检测到视盘或视杯时的记录文件
# self.lr_mismatch = False # 算法推理的左右眼是否与提供的一致
# self.dir_mid = None # 中间结果的保存目录
# self.dir_final = None # 最终结果的保存目录
# self.thresh_pred = -1
# self.disc_outside_fundus = False
def initial_to_blank(self):
# center(x,y), size(w,h), angle
# self.params_512 = ((-1, -1), (-1, -1), -1)
self.center_512 = (-1, -1) # 512尺寸图像上的椭圆中心
self.angle = -1 # 椭圆旋转角度
self.size_512_pixel = (-1, -1) # 椭圆直径,单位为像素, size[0]<size[1]
self.is_width_gt_height = False # 是否宽度大于高度(即横向椭圆)
self.width_roi = -1 # roi(视盘或视杯)的宽度
self.height_roi = -1 # roi的高度
self.size_512_mm: Tuple[float, float] = (-1, -1) # 椭圆直径,单位为毫米, (w, h)
self.area = [-1, -1, -1] # area_mm, area_pixel, area_ratio
self.curvature = (-1, -1) # 曲率
self.color_contrast = -1 # 颜色对比度(内比外)
test = EllipseParams('12')
test.initial_to_blank()
print(test.status)
print(test.angle)
print(test.initial_to_blank().angle)
-1
-1
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
<ipython-input-11-5125d31ef93f> in <module>()
38 print(test.status)
39 print(test.angle)
---> 40 print(test.initial_to_blank().angle)
AttributeError: 'NoneType' object has no attribute 'angle'
hasattr() 函数用于判断对象是否包含对应的属性。
class CLanguage:
'''这是一个学习Python定义的一个类'''
def __init__(self,name,add):
self.a = name
self.b = add
print(name,"的网址为:",add)
#创建 add 对象,并传递参数给构造函数
add = CLanguage("C语言中文网","http://c.biancheng.net")
print(add.a)
print(add.b)
print(CLanguage('a','b').a)
C语言中文网 的网址为: http://c.biancheng.net
C语言中文网
http://c.biancheng.net
a 的网址为: b
a
python __call__()方法
class CLanguage:
# 定义__call__方法
def __call__(self,name,add):
print("调用__call__()方法",name,add)
return 'nihao'
clangs = CLanguage()
c = clangs("C语言中文网","http://c.biancheng.net")
print(c)
print('===============')
clangs("C语言中文网","http://c.biancheng.net")
调用__call__()方法 C语言中文网 http://c.biancheng.net
nihao
===============
调用__call__()方法 C语言中文网 http://c.biancheng.net
nihao
class A():
def m(self):
print('a')
def n(self):
print('b')
a = A()
a.m()
a
# 使用__call__后
class A():
def __call__(self):
print('a')
def n(self):
print('b')
a = A()
a()
a
python __getitem__()
class DataTest:
def __init__(self,id,address):
self.id=id
self.address=address
self.d={self.id:1,
self.address:"192.168.1.1"
}
def __getitem__(self,key):
return "hello"
data=DataTest(1,"192.168.2.11")
print(data)
print(type(data))
print (data[11000])
<__main__.DataTest object at 0x7fc227f1da90>
<class '__main__.DataTest'>
hello
在用 for..in.. 迭代对象时,如果对象没有实现 __iter__ __next__ 迭代器协议,Python的解释器就会去寻找__getitem__ 来迭代对象,如果连__getitem__ 都没有定义,这解释器就会报对象不是迭代器的错误:
TypeError: 'Animal' object is not iterable
扫描二维码关注公众号,回复:
13163332 查看本文章
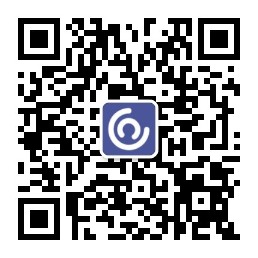
class Animal:
def __init__(self, animal_list):
self.animals_name = animal_list
def __getitem__(self, index):
return self.animals_name[index]
animals = Animal(["dog","cat","fish"])
for animal in animals:
print(animal)
dog
cat
fish
class Tag:
def __init__(self,id):
self.id=id
def __getitem__(self, item):
print('这个方法被调用')
return self.id
a=Tag('This is id')
print(a.id)
out = print(a[1681])
print(out)
This is id
这个方法被调用
This is id
None
class Tag:
def __init__(self):
self.change={'python':'This is python'}
def __getitem__(self, item):
print('这个方法被调用')
return self.change[item]
a=Tag()
print(a.change)
print(a['python'])
{'python': 'This is python'}
这个方法被调用
This is python
python __len__()方法:
class Employee:
#name = ''
def __init__(self, n):
self.name = n
print(self.name)
print(n)
print('=============')
def __len__(self):
print(self.name)
return len(self.name)
e = Employee('Pankaj')
print('employee object length =', len(e))
Pankaj
Pankaj
=============
Pankaj
employee object length = 6
class CountList:
def __init__(self, *args):
self.values = [x for x in args]
self.count = {}.fromkeys(range(len(self.values)),0)
def __len__(self):
return len(self.values)
def __getitem__(self, key):
self.count[key] += 1
print(self.count[key])
return self.values[key]
c1 = CountList(1,3,5,7,9)
print(c1.values)
print(c1.count)
print(len(c1))
print(len(c1.values))
print(len(c1.count))
print('=========================')
print(c1[3])
print(c1[2])
[1, 3, 5, 7, 9]
{0: 0, 1: 0, 2: 0, 3: 0, 4: 0}
5
5
5
=========================
1
7
1
5