Spring Boot与数据访问一–Spring Data介绍
Spring Boot与数据访问二–使用原生JDBC及源码解析
Spring Boot与数据访问三–整合Druid
Spring Boot与数据访问四–整合MyBatis(注解版)
Spring Boot与数据访问五–整合MyBatis(配置版)
Spring Boot与数据访问六–整合JPA
一、创建相应文件
创建mapper文件:
package com.huiq.springboot.mapper;
import com.huiq.springboot.entity.User;
import java.util.List;
// @Mapper或者@MapperScan将接口扫描装配到容器中
public interface UserMapper {
public User getUserId(Integer id);
public int insertUser(User user);
List<User> findAll();
}
可以参考MyBatis的官网
SQL映射文件:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.huiq.springboot.mapper.UserMapper">
<!-- 自定义返回结果集 -->
<resultMap id="userMap" type="User">
<id property="id" column="id" javaType="java.lang.Integer"></id>
<result property="name" column="name" javaType="java.lang.String"></result>
<result property="password" column="password" javaType="java.lang.String"></result>
</resultMap>
<select id="getUserId" resultType="com.huiq.springboot.entity.User">
SELECT * FROM tbl_user WHERE id=#{id}
</select>
<insert id="insertUser">
INSERT INTO tbl_user(na_me,password) VALUES (#{name},#{password})
</insert>
<select id="findAll" resultType="User">
SELECT * FROM tbl_user;
</select>
</mapper>
注意:
1.namespace中需要与使用@Mapper的接口对应
2.UserMapper.xml文件名称必须与使用@Mapper的接口一致
3.标签中的id必须与@Mapper的接口中的方法名一致,且参数一致
全局配置文件:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!-- 设置驼峰命名 -->
<settings>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
</configuration>
注意:
1.全局配置文件不是必须有,没有该文件也可以启动成功,且开启驼峰命名也可以在application.yml中实现
2.mybatis中的mapper-locations是mapper的xml文件位置
3.mybatis中的type-aliases-package是为了配置xml文件中resultType返回值的包位置,如果未配置请使用全包名如下:
<select id="findAll" resultType="com.huiq.springboot.entity.User">
SELECT * FROM tbl_user;
</select>
在application.yml文件中添加
mybatis:
config-location: classpath:mybatis/mybatis-config.xml # 指定全局配置文件的位置
# mapper-locations: classpth:mybatis/mapper/*.xml 或者下面的写法
mapper-locations: classpath:mybatis/mapper/UserMapper.xml # 指定sql映射文件的位置
type-aliases-package: com.huiq.springboot.entity
#开启驼峰命名
# configuration:
# map-underscore-to-camel-case: true
controller:
package com.huiq.springboot.controller;
import com.huiq.springboot.entity.User;
import com.huiq.springboot.mapper.UserMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class UserController {
@Autowired
UserMapper userMapper;
@GetMapping("/user/{id}")
public User getUser(@PathVariable("id") Integer id) {
return userMapper.getUserId(id);
}
@GetMapping("/user")
public User insertUser(User user) {
userMapper.insertUser(user);
return user;
}
@RequestMapping("/findAll")
public List<User> findAll(){
return userMapper.findAll();
}
}
启动程序测试
整个项目结构截图
二、优化为impl和service的形式
package com.huiq.springboot.service;
import com.huiq.springboot.entity.User;
import java.util.List;
public interface UserService {
User getUserId(Integer id);
int insertUser(User user);
List<User> findAll();
}
注意:需要在接口实现类中使用@Service注解,才能被SpringBoot扫描,在Controller中使用@Authwired注入
package com.huiq.springboot.service.impl;
import com.huiq.springboot.entity.User;
import com.huiq.springboot.mapper.UserMapper;
import com.huiq.springboot.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service("userService")
public class UserServiceimpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User getUserId(Integer id) {
return userMapper.getUserId(id);
}
@Override
public int insertUser(User user) {
return userMapper.insertUser(user);
}
@Override
public List<User> findAll() {
return userMapper.findAll();
}
}
修改UserController为
扫描二维码关注公众号,回复:
12920146 查看本文章
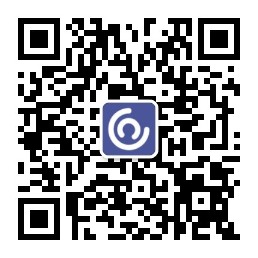
package com.huiq.springboot.controller;
import com.huiq.springboot.entity.User;
import com.huiq.springboot.mapper.UserMapper;
import com.huiq.springboot.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class UserController {
@Autowired
UserService userService;
@GetMapping("/user/{id}")
public User getUser(@PathVariable("id") Integer id) {
return userService.getUserId(id);
}
@GetMapping("/user")
public User insertUser(User user) {
userService.insertUser(user);
return user;
}
@RequestMapping("/findAll")
public List<User> findAll(){
return userService.findAll();
}
}
注:可以和我的另一篇不用Springboot搭建MyBatis文章作对比