myBatis xml文件SQL编写( if、where、if-else、set 、foreach )
MyBatis 的强大特性之一便是它的动态 SQL。如果你有使用 JDBC 或其它类似框架的经验,你就能体会到根据不同条件拼接 SQL 语句的痛苦。例如拼接时要确保不能忘记添加必要的空格,还要注意去掉列表最后一个列名的逗号。利用动态 SQL 这一特性可以彻底摆脱这种痛苦。
虽然在以前使用动态 SQL 并非一件易事,但正是 MyBatis 提供了可以被用在任意 SQL 映射语句中的强大的动态 SQL 语言得以改进这种情形。
动态 SQL 元素和 JSTL 或基于类似 XML 的文本处理器相似。在 MyBatis 之前的版本中,有很多元素需要花时间了解。MyBatis 3 大大精简了元素种类,现在只需学习原来一半的元素便可。MyBatis 采用功能强大的基于 OGNL 的表达式来淘汰其它大部分元素。
1. if 标签
mapper中编写AQL,使用<if test = ' '> </if>
标签,可以使你的接口很便捷。
代码如下(示例):
<select id="getStudentByName" parameterType="java.util.Map" resultType="java.util.Map">
select * from student
<if test = " record.name != null ">
where name =#{record.name}
</if>
</select>
<select id="getStudentByIdOrName" parameterType="java.util.Map" resultType="java.util.Map">
select * from student
where
<if test = " record.id != null ">
id = #{record.id}
</if>
<if test = " record.name != null and record.name != '' ">
and name = #{record.name}
</if>
</select>
如上面的代码,如果当你的id为空时,name前面的and是没有必要的,运行会抛异常
或者当这两个都为空时,只剩一个空的where,还是会报错,这个时候就需要<where>
标签了。
2. where 标签
<where>
标签 只会在至少有一个子元素的条件返回 SQL 子句的情况下才去插入WHERE子句。而且,若语句的开头为AND或OR,<where>
标签也会将它们去除。
使用<where>
标签,代码将会变得很优美。
代码如下(示例):
<select id="getStudentByIdOrName" parameterType="java.util.Map" resultType="java.util.Map">
select * from student
<where>
<if test = " record.id != null ">
and id = #{record.id}
</if>
<if test = " record.name != null and record.name != '' ">
and name = #{record.name}
</if>
</where>
</select>
3. choose, when, otherwise 标签 (if - else if - else )
在myBatis中是不支持 if - else 的,想要是用 if - else 的话,可以使用<choose>
标签代替。
<choose>
标签 、<when>
标签、<otherwise>
标签 ,<otherwise>
有点像 Java 中的switch语句。
代码如下(示例):
<select id="getStudentByKeyword" parameterType="java.util.Map" resultType="java.util.Map">
SELECT * FROM student
WHERE state = '已入学'
<choose>
<when test="record.name != null and record.name != ''">
AND name like #{record.name}
</when>
<when test="record.remark != null and record.remark != ''">
AND remark like #{record.remark }
</when>
<otherwise>
AND status = 1
</otherwise>
</choose>
</select>
4. set 标签
代码如下(示例):
<update id="updateStudentBySet" parameterType="java.util.Map" resultType="java.util.Map">
update student
<set>
<if test="record.name != null and record.name != ''">
name = #{record.name},
</if>
<if test="record.sex != null and record.sex != ''">
sex = #{record.sex },
</if>
</set>
where id = #{record.id}
</update>
<set>
标签会在成功拼接的条件前加上SET单词且最后一个”,”号会被无视掉,但是有可能需要”,”的地方不能省略”,”否则异常。
扫描二维码关注公众号,回复:
12824610 查看本文章
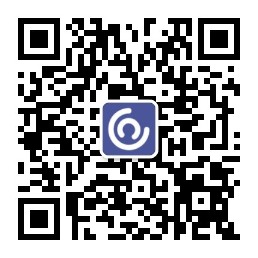
5. foreach 标签
foreach标签用于遍历生成单个或多个数据。
代码如下(示例):
<select id="getStudentCount" parameterType="java.util.Map" resultType="java.util.Map">
select COUNT(*) count from student
<where>
<if test="record.ids != null">
and id in
<foreach item="item" index="index" collection="record.ids " open="(" separator="," close=")">
#{item}
</foreach>
</if>
</where>
</select>
提示:关于mybatis的动态sql,建议查看,官方文档