系列文章
JavaWeb 开发 01 —— 基本概念、Web服务器、HTTP、Maven
JavaWeb 开发 02 —— ServletContext、读取资源、下载文件、重定向和请求转发
JavaWeb 开发 03 —— Cookie 和 Session
JavaWeb 开发 04 —— JSP(原理、语法、指令、内置对象、JSP标签、JSTP标签)、JavaBean、MVC
实践:SMBMS项目
SMBMS是超市订单管理系统的英文缩写。
SMBMS项目SQL代码:https://download.csdn.net/download/qq_39763246/15352854
百度网盘:https://pan.baidu.com/s/16fNpMkGeXZud-U58Z5y67g 提取码: q3eq
项目架构:
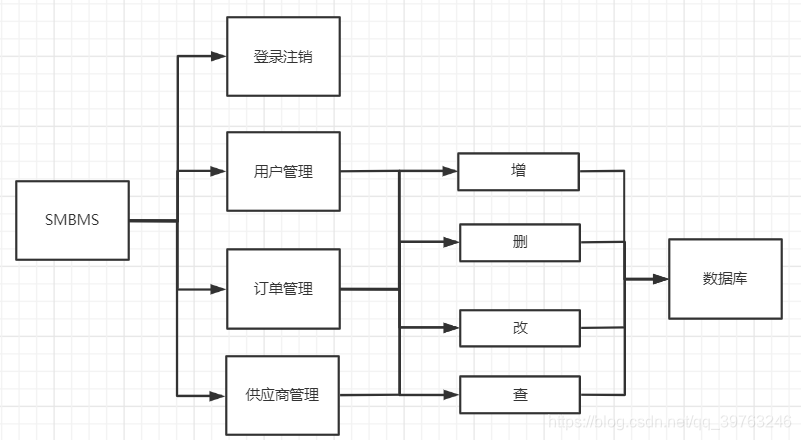
数据库架构:
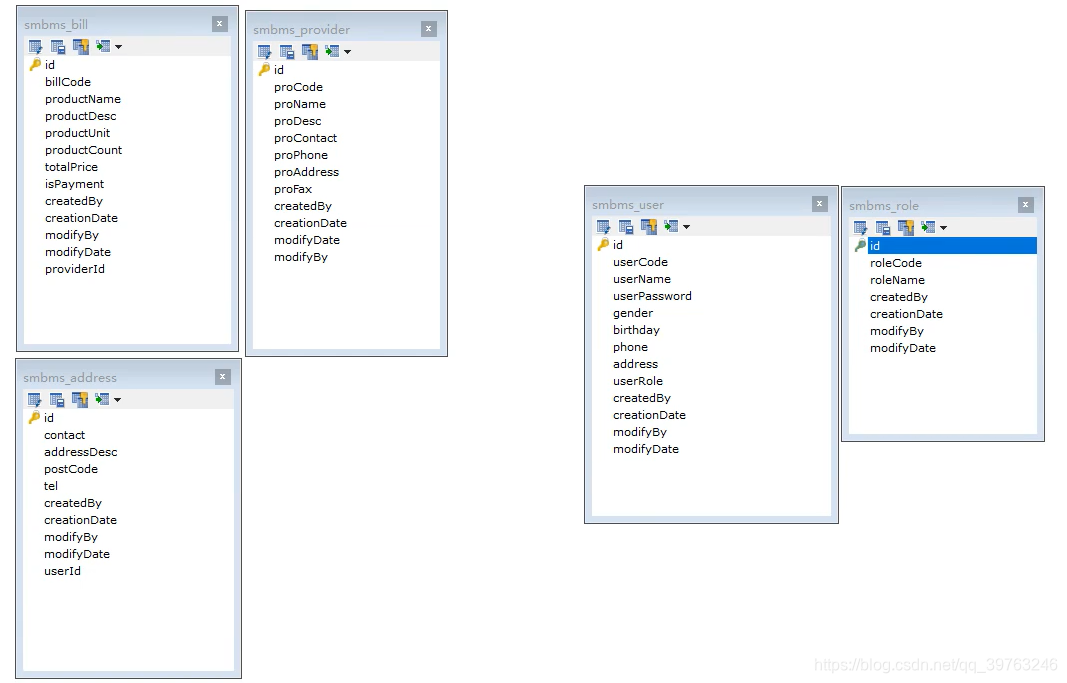
项目搭建准备工作
搭建项目时要先考虑是否使用Maven,如果用Maven则需要写依赖,如果不用Maven则要手动导入Jar包。这里选择使用Maven。
1、搭建一个Maven Web项目
2、配置Tomcat
3、启动Tomcat,测试项目能否跑起来
4、导入项目中会遇到的jar包
<dependencies>
<!-- Servlet依赖 -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
<!-- JSP依赖 -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>javax.servlet.jsp-api</artifactId>
<version>2.3.3</version>
<scope>provided</scope>
</dependency>
<!-- JSTL表达式依赖 -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- standard标签库 -->
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
</dependency>
<!-- 连接数据库 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
<!-- 代码测试 -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<!-- 导入阿里巴巴的fastjson, 处理json转化 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.62</version>
</dependency>
</dependencies>
5、创建项目包结构
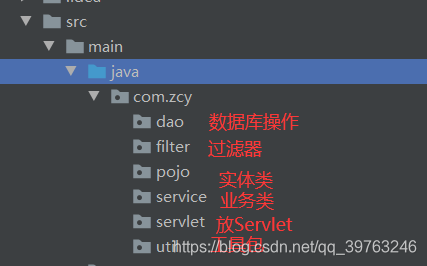
6、编写实体类
ORM映射:数据库 表—Java 类映射
Bill.java
package com.zcy.pojo;
import java.math.BigDecimal;
import java.util.Date;
public class Bill {
private Integer id; //id
private String billCode; //账单编码
private String productName; //商品名称
private String productDesc; //商品描述
private String productUnit; //商品单位
private BigDecimal productCount; //商品数量
private BigDecimal totalPrice; //总金额
private Integer isPayment; //是否支付
private Integer providerId; //供应商ID
private Integer createdBy; //创建者
private Date creationDate; //创建时间
private Integer modifyBy; //更新者
private Date modifyDate;//更新时间
private String providerName;//供应商名称
public String getProviderName() {
return providerName;
}
public void setProviderName(String providerName) {
this.providerName = providerName;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getBillCode() {
return billCode;
}
public void setBillCode(String billCode) {
this.billCode = billCode;
}
public String getProductName() {
return productName;
}
public void setProductName(String productName) {
this.productName = productName;
}
public String getProductDesc() {
return productDesc;
}
public void setProductDesc(String productDesc) {
this.productDesc = productDesc;
}
public String getProductUnit() {
return productUnit;
}
public void setProductUnit(String productUnit) {
this.productUnit = productUnit;
}
public BigDecimal getProductCount() {
return productCount;
}
public void setProductCount(BigDecimal productCount) {
this.productCount = productCount;
}
public BigDecimal getTotalPrice() {
return totalPrice;
}
public void setTotalPrice(BigDecimal totalPrice) {
this.totalPrice = totalPrice;
}
public Integer getIsPayment() {
return isPayment;
}
public void setIsPayment(Integer isPayment) {
this.isPayment = isPayment;
}
public Integer getProviderId() {
return providerId;
}
public void setProviderId(Integer providerId) {
this.providerId = providerId;
}
public Integer getCreatedBy() {
return createdBy;
}
public void setCreatedBy(Integer createdBy) {
this.createdBy = createdBy;
}
public Date getCreationDate() {
return creationDate;
}
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
public Integer getModifyBy() {
return modifyBy;
}
public void setModifyBy(Integer modifyBy) {
this.modifyBy = modifyBy;
}
public Date getModifyDate() {
return modifyDate;
}
public void setModifyDate(Date modifyDate) {
this.modifyDate = modifyDate;
}
}
Provider.java
package com.zcy.pojo;
import java.util.Date;
public class Provider {
private Integer id; //id
private String proCode; //供应商编码
private String proName; //供应商名称
private String proDesc; //供应商描述
private String proContact; //供应商联系人
private String proPhone; //供应商电话
private String proAddress; //供应商地址
private String proFax; //供应商传真
private Integer createdBy; //创建者
private Date creationDate; //创建时间
private Integer modifyBy; //更新者
private Date modifyDate;//更新时间
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getProCode() {
return proCode;
}
public void setProCode(String proCode) {
this.proCode = proCode;
}
public String getProName() {
return proName;
}
public void setProName(String proName) {
this.proName = proName;
}
public String getProDesc() {
return proDesc;
}
public void setProDesc(String proDesc) {
this.proDesc = proDesc;
}
public String getProContact() {
return proContact;
}
public void setProContact(String proContact) {
this.proContact = proContact;
}
public String getProPhone() {
return proPhone;
}
public void setProPhone(String proPhone) {
this.proPhone = proPhone;
}
public String getProAddress() {
return proAddress;
}
public void setProAddress(String proAddress) {
this.proAddress = proAddress;
}
public String getProFax() {
return proFax;
}
public void setProFax(String proFax) {
this.proFax = proFax;
}
public Integer getCreatedBy() {
return createdBy;
}
public void setCreatedBy(Integer createdBy) {
this.createdBy = createdBy;
}
public Date getCreationDate() {
return creationDate;
}
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
public Integer getModifyBy() {
return modifyBy;
}
public void setModifyBy(Integer modifyBy) {
this.modifyBy = modifyBy;
}
public Date getModifyDate() {
return modifyDate;
}
public void setModifyDate(Date modifyDate) {
this.modifyDate = modifyDate;
}
}
Role.java
package com.zcy.pojo;
import java.util.Date;
public class Role {
private Integer id; //id
private String roleCode; //角色编码
private String roleName; //角色名称
private Integer createdBy; //创建者
private Date creationDate; //创建时间
private Integer modifyBy; //更新者
private Date modifyDate;//更新时间
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getRoleCode() {
return roleCode;
}
public void setRoleCode(String roleCode) {
this.roleCode = roleCode;
}
public String getRoleName() {
return roleName;
}
public void setRoleName(String roleName) {
this.roleName = roleName;
}
public Integer getCreatedBy() {
return createdBy;
}
public void setCreatedBy(Integer createdBy) {
this.createdBy = createdBy;
}
public Date getCreationDate() {
return creationDate;
}
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
public Integer getModifyBy() {
return modifyBy;
}
public void setModifyBy(Integer modifyBy) {
this.modifyBy = modifyBy;
}
public Date getModifyDate() {
return modifyDate;
}
public void setModifyDate(Date modifyDate) {
this.modifyDate = modifyDate;
}
}
User.java
package com.zcy.pojo;
import java.util.Date;
public class User {
private Integer id; //id
private String userCode; //用户编码
private String userName; //用户名称
private String userPassword; //用户密码
private Integer gender; //性别
private Date birthday; //出生日期
private String phone; //电话
private String address; //地址
private Integer userRole; //用户角色
private Integer createdBy; //创建者
private Date creationDate; //创建时间
private Integer modifyBy; //更新者
private Date modifyDate; //更新时间
private Integer age;//年龄
private String userRoleName; //用户角色名称
public String getUserRoleName() {
return userRoleName;
}
public void setUserRoleName(String userRoleName) {
this.userRoleName = userRoleName;
}
public Integer getAge() {
/*long time = System.currentTimeMillis()-birthday.getTime();
Integer age = Long.valueOf(time/365/24/60/60/1000).IntegerValue();*/
Date date = new Date();
Integer age = date.getYear()-birthday.getYear();
return age;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserCode() {
return userCode;
}
public void setUserCode(String userCode) {
this.userCode = userCode;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getUserPassword() {
return userPassword;
}
public void setUserPassword(String userPassword) {
this.userPassword = userPassword;
}
public Integer getGender() {
return gender;
}
public void setGender(Integer gender) {
this.gender = gender;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Integer getUserRole() {
return userRole;
}
public void setUserRole(Integer userRole) {
this.userRole = userRole;
}
public Integer getCreatedBy() {
return createdBy;
}
public void setCreatedBy(Integer createdBy) {
this.createdBy = createdBy;
}
public Date getCreationDate() {
return creationDate;
}
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
public Integer getModifyBy() {
return modifyBy;
}
public void setModifyBy(Integer modifyBy) {
this.modifyBy = modifyBy;
}
public Date getModifyDate() {
return modifyDate;
}
public void setModifyDate(Date modifyDate) {
this.modifyDate = modifyDate;
}
}
7、编写基础公共类
-
数据库配置文件(resources目录下db.properties)
driver=com.mysql.jdbc.Driver url=jdbc:mysql://localhost:3306/smbms?useUnicode=true&characterEncoding=utf8&useSSL=false username=root password=123456
-
数据库公共类(java目录下dao的BaseDao.java)
数据库参考我另一篇博客:数据库 —— Java操作MySQLpackage com.zcy.dao; import javax.servlet.jsp.jstl.sql.Result; import javax.servlet.jsp.jstl.sql.ResultSupport; import java.io.IOException; import java.io.InputStream; import java.sql.*; import java.util.Properties; public class BaseDao { private static String driver; private static String url; private static String userName; private static String password; //静态代码块:当类被加载时会执行 static{ //获取配置文件的流 Properties properties = new Properties(); InputStream is = BaseDao.class.getClassLoader().getResourceAsStream("db.properties"); try { properties.load(is); } catch (IOException e) { e.printStackTrace(); } driver = properties.getProperty("driver"); url = properties.getProperty("url"); userName = properties.getProperty("username"); password = properties.getProperty("password"); } //获取数据库连接 public static Connection getConnection(){ Connection connection = null; try { Class.forName(driver); connection = DriverManager.getConnection(url, userName, password); } catch (Exception e) { e.printStackTrace(); } return connection; } //查询公共方法 public static ResultSet execute(Connection connection,PreparedStatement preparedStatement, Object[] param) throws SQLException { //这里i+1是因为PreparedStatement 用的占位符是从1开始 for (int i = 0; i < param.length; i++) { preparedStatement.setObject(i+1, param[i]); } ResultSet resultSet = preparedStatement.executeQuery(); return resultSet; } //更新公共方法 public static int update(Connection connection, PreparedStatement preparedStatement, Object[] param) throws SQLException { for (int i = 0; i < param.length; i++) { preparedStatement.setObject(i+1, param[i]); } int updateRows = preparedStatement.executeUpdate(); return updateRows; } public static boolean closeResource(Connection connection, PreparedStatement preparedStatement, ResultSet resultSet){ boolean flag = true; if (resultSet != null){ try { resultSet.close(); //让JVM回收,GC 垃圾回收机制 resultSet = null; } catch (SQLException e) { e.printStackTrace(); flag = false; } } if (preparedStatement != null){ try { preparedStatement.close(); //让JVM回收,GC 垃圾回收机制 preparedStatement = null; } catch (SQLException e) { e.printStackTrace(); flag = false; } } if (connection != null){ try { connection.close(); //让JVM回收,GC 垃圾回收机制 connection = null; } catch (SQLException e) { e.printStackTrace(); flag = false; } } return flag; } }
-
编写过滤器
package com.zcy.filter; import javax.servlet.*; import java.io.IOException; public class CharacterEncodingFilter implements Filter { public void doFilter( ServletRequest request, ServletResponse response, FilterChain chain ) throws IOException, ServletException { request.setCharacterEncoding("utf-8"); response.setCharacterEncoding("utf-8"); response.setContentType("text/html;charset=utf-8"); chain.doFilter(request, response); } }
-
web.xml中注册
<!-- 过滤所有请求,处理中文乱码 --> <filter> <filter-name>CharacterEncodingFilter</filter-name> <filter-class>com.zcy.filter.CharacterEncodingFilter</filter-class> </filter> <filter-mapping> <filter-name>CharacterEncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
8、导入静态资源,放在webapp目录下。
下载链接: CSDN
百度网盘:https://pan.baidu.com/s/16fNpMkGeXZud-U58Z5y67g 提取码: q3eq
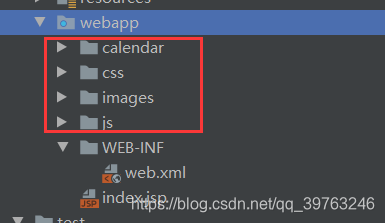
登录功能
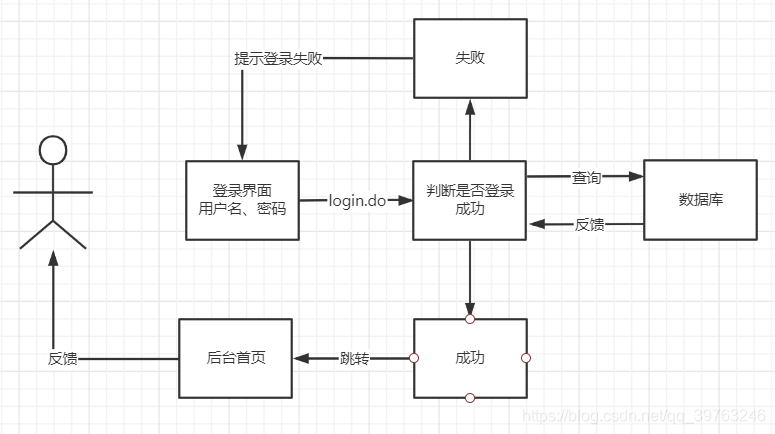
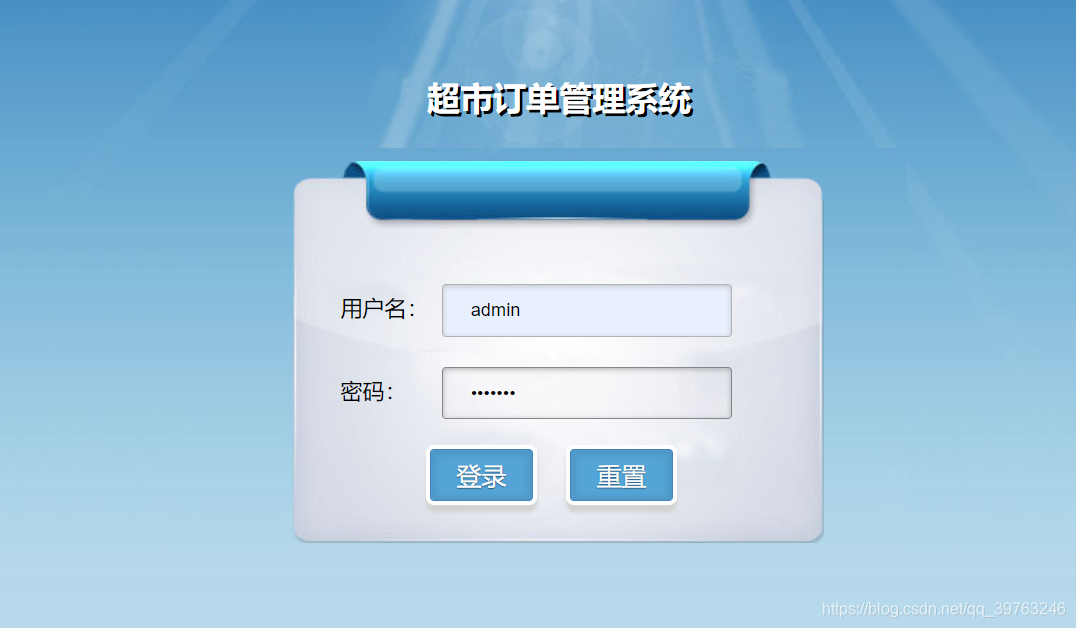
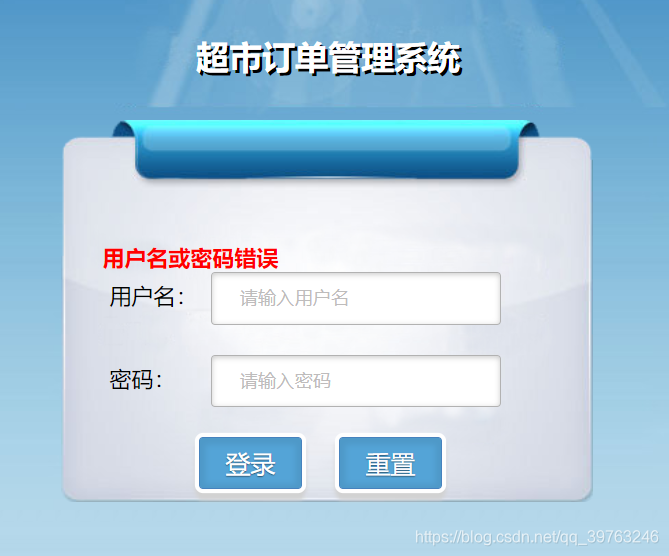
1、编写前端页面(login.jsp)
login.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>系统登录 - 超市订单管理系统</title>
<link type="text/css" rel="stylesheet" href="${pageContext.request.contextPath}/css/style.css" />
</head>
<body class="login_bg">
<section class="loginBox">
<header class="loginHeader">
<h1>超市订单管理系统</h1>
</header>
<section class="loginCont">
<form class="loginForm" action="${pageContext.request.contextPath}/login.do" name="actionForm" id="actionForm" method="post" >
<div class="info">${error }</div>
<div class="inputbox">
<label>用户名:</label>
<input type="text" class="input-text" id="userCode" name="userCode" placeholder="请输入用户名" required/>
</div>
<div class="inputbox">
<label>密码:</label>
<input type="password" id="userPassword" name="userPassword" placeholder="请输入密码" required/>
</div>
<div class="subBtn">
<input type="submit" value="登录"/>
<input type="reset" value="重置"/>
</div>
</form>
</section>
</section>
</body>
</html>
2、设置欢迎页,web.xml中设置
<!-- 设置欢迎页(首页) -->
<welcome-file-list>
<welcome-file>login.jsp</welcome-file>
</welcome-file-list>
3、编写Dao层 得到用户登录的接口
package com.zcy.dao.user;
import com.zcy.pojo.User;
import java.sql.Connection;
import java.sql.SQLException;
//操作用户的Dao
public interface UserDao {
/**
* 获得想要登录的用户
* @param connection 连接参数
* @param userCode 用户账号
* @return 返回想登录的用户对象,为null则没有该用户
*/
public User getLoginUser(Connection connection, String userCode) throws SQLException;
}
4、编写Dao 接口的实现类
package com.zcy.dao.user;
import com.zcy.dao.BaseDao;
import com.zcy.pojo.User;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class UserDaoImpl implements UserDao {
/**
*
* @param connection 连接参数
* @param userCode 用户账号
* @return 返回想登录的用户对象,为null则没有该用户
* @throws SQLException
*/
public User getLoginUser(Connection connection, String userCode) throws SQLException {
ResultSet resultSet = null;
User user = null;
if (connection != null){
String sql = "select * from smbms_user where userCode = ?";
Object[] params = {
userCode};
resultSet = BaseDao.execute(connection, sql, params);
if (resultSet.next()){
user = new User();
user.setId(resultSet.getInt("id"));
user.setUserCode(resultSet.getString("userCode"));
user.setUserName(resultSet.getString("userName"));
user.setUserPassword(resultSet.getString("userPassword"));
user.setGender(resultSet.getInt("gender"));
user.setBirthday(resultSet.getDate("birthday"));
user.setPhone(resultSet.getString("phone"));
user.setAddress(resultSet.getString("address"));
user.setUserRole(resultSet.getInt("userRole"));
user.setCreatedBy(resultSet.getInt("createdBy"));
user.setCreationDate(resultSet.getTimestamp("creationDate"));
user.setModifyBy(resultSet.getInt("modifyBy"));
user.setModifyDate(resultSet.getTimestamp("modifyDate"));
}
BaseDao.closeResource(null, null, resultSet);
}
return user;
}
}
5、业务层接口
package com.zcy.service.user;
import com.zcy.pojo.User;
public interface UserService {
/**
* 用户登录
* @param userCode 用户编号
* @param password 用户密码
* @return 存在该用户,则返回用户对象,不存在则为null
*/
public User login(String userCode, String password);
}
6、业务层实现类
package com.zcy.service.user;
import com.zcy.dao.BaseDao;
import com.zcy.dao.user.UserDao;
import com.zcy.dao.user.UserDaoImpl;
import com.zcy.pojo.User;
import org.junit.Test;
import java.sql.Connection;
import java.sql.SQLException;
public class UserServiceImpl implements UserService {
//业务层都会调用Dao层,所有我们要先引入Dao层
private UserDao userDao;
/**
* 实例化Dao层的对象
*/
public UserServiceImpl(){
userDao = new UserDaoImpl();
}
/**
* 在这个业务层调用Dao层的具体数据库操作,获得要登录的用户对象
* @param userCode 用户编码
* @param password 用户密码
* @return 要登录的用户对象,为null则没有该用户
*/
public User login(String userCode, String password) {
Connection connection = null;
User user = null;
try {
connection = BaseDao.getConnection();
//在业务层调用对应的具体数据库操作,这里就是获取要登录的用户对象
user = userDao.getLoginUser(connection, userCode);
} catch (SQLException e) {
e.printStackTrace();
}finally {
BaseDao.closeResource(connection, null, null);
}
if (user == null || !user.getUserPassword().equals(password))
return null;
else
return user;
}
/**
* 用于测试,可直接删除
*/
@Test
public void test(){
UserServiceImpl userService = new UserServiceImpl();
User admin = userService.login("admin", "abcdefg");
System.out.println(admin.getUserPassword());
}
}
7、编写Servlet
package com.zcy.servlet.user;
import com.zcy.pojo.User;
import com.zcy.service.UserService;
import com.zcy.service.UserServiceImpl;
import com.zcy.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class LoginServlet extends HttpServlet {
//Servlet:控制层,调用业务层代码
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("LoginServlet————Start....");
//获取用户编码和密码
String userCode = req.getParameter("userCode");
String userPassword = req.getParameter("userPassword");
UserService userService = new UserServiceImpl();
User user = userService.login(userCode, userPassword);
if (user!=null){
//有此人,登录成功
//将用户信息放在session中
req.getSession().setAttribute(Constants.USER_SESSION, user);
//使用重定向到主页面
resp.sendRedirect("jsp/frame.jsp");
}
else {
//没有此人或密码错误,返回登录界面并给出提示
req.setAttribute("error", "用户名或密码错误");
//这里之所以用转发而不是重定向,是因为前端是调用request里的error变量
//转发可以将请求转发出去,保证了error的存活
req.getRequestDispatcher("login.jsp").forward(req, resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
之所以能够提示用户名或密码错误,是因为login.jsp中引用了error变量
在页面头部中,也引用了session中的用户信息
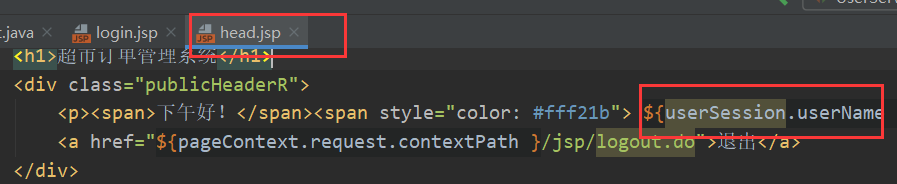
8、注册Servlet
<servlet>
<servlet-name>LoginServlet</servlet-name>
<servlet-class>com.zcy.servlet.user.LoginServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>LoginServlet</servlet-name>
<url-pattern>/login.do</url-pattern>
</servlet-mapping>
注销拦截
1、注销功能:移除用户session并返回登录界面
LogoutServlet.java
package com.zcy.servlet.user;
import com.zcy.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class LogoutServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req,resp);
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//注销:移除用户session
req.getSession().removeAttribute(Constants.USER_SESSION);
resp.sendRedirect(req.getContextPath()+"/login.jsp");
}
}
注册Servlet
<servlet>
<servlet-name>LogoutServlet</servlet-name>
<servlet-class>com.zcy.servlet.user.LogoutServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>LogoutServlet</servlet-name>
<url-pattern>/jsp/logout.do</url-pattern>
</servlet-mapping>
2、登录拦截
package com.zcy.filter;
import com.sun.deploy.net.HttpResponse;
import com.zcy.pojo.User;
import com.zcy.util.Constants;
import javax.servlet.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class LoginFilter implements Filter {
public void init(FilterConfig filterConfig) throws ServletException {
}
public void doFilter(
ServletRequest req, ServletResponse resp, FilterChain chain
) throws IOException, ServletException {
HttpServletRequest request = (HttpServletRequest)req;
HttpServletResponse response = (HttpServletResponse) resp;
//获得已登录的用户
User user = (User) request.getSession().getAttribute(Constants.USER_SESSION);
//如果用户没有登录或者已经注销,则再想进入主页面就会被拦截,然后重定向
if(user == null)
response.sendRedirect(request.getContextPath()+"/error.jsp");
else
chain.doFilter(req, resp);
}
public void destroy() {
}
}
注册
<!-- 过滤未登录用户 -->
<filter>
<filter-name>LoginFilter</filter-name>
<filter-class>com.zcy.filter.LoginFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>LoginFilter</filter-name>
<url-pattern>/jsp/*</url-pattern>
</filter-mapping>
密码修改
1、UserDao.java中添加方法
/**
* 修改用户密码
* @param connection 连接参数
* @param id 用户ID号
* @param password 用户新密码
* @return 返回修改的行数(1表示成功 0表示失败)
* @throws SQLException
*/
public int updatePassword(Connection connection, int id, String password) throws SQLException;
2、UserDaoImpl.java中添加方法
/**
*
* @param connection 连接参数
* @param id 用户ID号
* @param password 用户新密码
* @return 返回修改的行数(1表示成功 0表示失败)
* @throws SQLException
*/
public int updatePassword(Connection connection, int id, String password) throws SQLException {
PreparedStatement preparedStatement = null;
int updateRows = 0;
if (connection != null){
String sql = "update smbms_user set userPassword = ? where id = ?";
preparedStatement = connection.prepareStatement(sql);
Object[] params = {
password, id};
updateRows = BaseDao.update(connection, preparedStatement, params);
BaseDao.closeResource(null, preparedStatement, null);
}
return updateRows;
}
3、UserServiceImp.java中添加方法
/**
* 根据用户id和密码修改用户密码
* @param id 用户id
* @param password 用户密码
* @return 返回true 或 false
*/
public boolean updatePassword(int id, String password) {
Connection connection = null;
boolean flag = false;
try {
connection = BaseDao.getConnection();
if (userDao.updatePassword(connection, id, password) > 0)
flag = true;
} catch (SQLException e) {
e.printStackTrace();
}finally {
BaseDao.closeResource(connection, null, null);
}
return flag;
}
4、UserServlet.java
package com.zcy.servlet.user;
import com.alibaba.fastjson.JSONArray;
import com.mysql.jdbc.StringUtils;
import com.zcy.pojo.User;
import com.zcy.service.UserService;
import com.zcy.service.UserServiceImpl;
import com.zcy.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.HashMap;
import java.util.Map;
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//从前端获取参数,根据参数执行对应方法
String method = req.getParameter("method");
if (method.equals("savepwd"))
updatePassword(req,resp);
else if (method.equals("pwdmodify"))
pwdModify(req, resp);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
//更新密码
public void updatePassword(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
Object o = req.getSession().getAttribute(Constants.USER_SESSION);
String newPassword = req.getParameter("newpassword");
boolean flag = false;
if (o != null && !StringUtils.isEmptyOrWhitespaceOnly(newPassword)){
UserService userService = new UserServiceImpl();
flag = userService.updatePassword(((User)o).getId(), newPassword);
if (flag){
//因为前端pwdmodify.jsp中留有一个message的位置,可以显示数据
req.setAttribute("message", "密码修改成功,请重新用新密码登录");
//移除用户Session,相当于自动注销
req.getSession().removeAttribute(Constants.USER_SESSION);
}
else
req.setAttribute("message", "密码修改失败");
}
else
req.setAttribute("message", "新密码有问题");
req.getRequestDispatcher("pwdmodify.jsp").forward(req,resp);
}
//验证旧密码,无需查询数据库,直接从当前session中的user对象获取
public void pwdModify(HttpServletRequest req, HttpServletResponse resp){
//从session中拿取用户对象
Object o = req.getSession().getAttribute(Constants.USER_SESSION);
//获取前端(Ajax部分)传过来的旧密码,并于user中的密码比对
String oldPassword = req.getParameter("oldpassword");
//用Map结果集作为响应,返回给前端(Ajax部分)
Map<String, String> resultMap = new HashMap<String, String>();
if (o == null){
//session失效或过期时(真实网站的session会设置过期时间)
resultMap.put("result", "sessionerror");
}
else if(StringUtils.isEmptyOrWhitespaceOnly(oldPassword)){
//输入的旧密码为空
resultMap.put("result", "error");
}else {
String userPassword = ((User)o).getUserPassword();//已登录用户的现在密码
//比对输入的旧密码和session中用户密码
if (oldPassword.equals(userPassword))
resultMap.put("result", "true");
else
resultMap.put("result", "false");
}
try {
resp.setContentType("application/json");
PrintWriter writer = resp.getWriter();
//JSONArray 阿里巴巴的JSON工具类,将Map类转换为json(前端Ajax接受的是json格式)
writer.write(JSONArray.toJSONString(resultMap));
writer.flush();
writer.close();
}catch (IOException e){
e.printStackTrace();
}
}
}
配合pwdmodify.js中的Ajax,看旧密码验证部分
oldpassword.on("blur",function(){
$.ajax({
type:"GET",
url:path+"/jsp/user.do",
data:{
method:"pwdmodify",oldpassword:oldpassword.val()},
dataType:"json",
success:function(data){
if(data.result == "true"){
//旧密码正确
validateTip(oldpassword.next(),{
"color":"green"},imgYes,true);
}else if(data.result == "false"){
//旧密码输入不正确
validateTip(oldpassword.next(),{
"color":"red"},imgNo + " 原密码输入不正确",false);
}else if(data.result == "sessionerror"){
//当前用户session过期,请重新登录
validateTip(oldpassword.next(),{
"color":"red"},imgNo + " 当前用户session过期,请重新登录",false);
}else if(data.result == "error"){
//旧密码输入为空
validateTip(oldpassword.next(),{
"color":"red"},imgNo + " 请输入旧密码",false);
}
},
error:function(data){
//请求出错
validateTip(oldpassword.next(),{
"color":"red"},imgNo + " 请求错误",false);
}
});
5、注册UserServlet
<servlet>
<servlet-name>UserServlet</servlet-name>
<servlet-class>com.zcy.servlet.user.UserServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>UserServlet</servlet-name>
<url-pattern>/jsp/user.do</url-pattern>
</servlet-mapping>
用户管理
先写 PageSupport.java 分页工具类
package com.zcy.util;
public class PageSupport {
//当前页码-来自于用户输入
private int currentPageNo = 1;
//总数量(表)
private int totalCount = 0;
//页面容量
private int pageSize = 0;
//总页数-totalCount/pageSize(+1)
private int totalPageCount = 1;
public int getCurrentPageNo() {
return currentPageNo;
}
public void setCurrentPageNo(int currentPageNo) {
if(currentPageNo > 0){
this.currentPageNo = currentPageNo;
}
}
public int getTotalCount() {
return totalCount;
}
public void setTotalCount(int totalCount) {
if(totalCount > 0){
this.totalCount = totalCount;
//设置总页数
this.setTotalPageCountByRs();
}
}
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
if(pageSize > 0){
this.pageSize = pageSize;
}
}
public int getTotalPageCount() {
return totalPageCount;
}
public void setTotalPageCount(int totalPageCount) {
this.totalPageCount = totalPageCount;
}
public void setTotalPageCountByRs(){
if(this.totalCount % this.pageSize == 0){
this.totalPageCount = this.totalCount / this.pageSize;
}else if(this.totalCount % this.pageSize > 0){
this.totalPageCount = this.totalCount / this.pageSize + 1;
}else{
this.totalPageCount = 0;
}
}
}
1、获取用户数量
1、UserDao.java添加方法
/**
* 根据用户名或角色类型 获取用户数量
* @param connection 连接参数
* @param userName 用户名
* @param userRole 用户角色类型
* @return 用户数量
*/
public int getUserCount(Connection connection, String userName, int userRole) throws SQLException;
2、UserDaoImpl.java添加方法
/**
* 根据用户id和密码修改密码
* @param connection 连接参数
* @param userName 用户名
* @param userRole 用户角色类型
* @return 用户数量
* @throws SQLException
*/
public int getUserCount(Connection connection, String userName, int userRole) throws SQLException {
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
int count = 0;//这里用int更好,Integer不合适,因为count必定不为null
if (connection != null){
StringBuffer sql = new StringBuffer();
sql.append("select count(1) as count from smbms_user u, smbms_role r where u.userRole = r.id");
ArrayList<Object> list = new ArrayList<Object>();//存放参数
if (!StringUtils.isNullOrEmpty(userName)){
sql.append(" and u.userName like ?");
list.add("%"+userName+"%");//like 模糊查询
}
//因为角色的值是 1 2 3
if (userRole>0){
sql.append(" and r.id = ?");
list.add(userRole);
}
Object[] params = list.toArray();
//输出完整SQL
System.out.println("UserDaoImpl——>getUserCount:"+sql.toString());
preparedStatement = connection.prepareStatement(sql.toString());
resultSet = BaseDao.query(connection, preparedStatement, params);
if (resultSet.next())
count = resultSet.getInt("count");
BaseDao.closeResource(null, preparedStatement, resultSet);
}
return count;
}
3、UserService.java添加方法
/**
* 根据用户名或用户角色类型获取用户数量
* @param userName 用户名
* @param userRole 用户角色类型
* @return 用户数量
*/
public int getUserCount(String userName, int userRole);
4、UserServiceImpl.java添加方法
/**
* 根据用户名或用户角色类型获取用户数量
* @param userName 用户名
* @param userRole 用户角色类型
* @return 用户数量
*/
public int getUserCount(String userName, int userRole) {
Connection connection = null;
int count = 0;
try {
connection = BaseDao.getConnection();
count = userDao.getUserCount(connection, userName, userRole);
} catch (SQLException e) {
e.printStackTrace();
}finally {
BaseDao.closeResource(connection, null, null);
}
return count;
}
@Test
public void test(){
UserServiceImpl userService = new UserServiceImpl();
int userCount = userService.getUserCount(null, 3);
System.out.println(userCount);
}
2、获取用户列表
1、UserDao.java添加方法
/**
* 通过条件查询
* @param connection 连接参数
* @param userName 用户名
* @param userRole 用户角色
* @param currentPageNo 当前页
* @param pageSize 页面大小
* @return 用户列表
*/
public List<User> getUserList(Connection connection, String userName, int userRole,
int currentPageNo, int pageSize) throws Exception;
2、UserDaoImpl.java添加方法
/**
* 根据条件查询
* @param connection 连接参数
* @param userName 用户名
* @param userRole 用户角色
* @param currentPageNo 当前页
* @param pageSize 页面大小
* @return 用户列表
* @throws Exception
*/
public List<User> getUserList(Connection connection, String userName,int userRole,int currentPageNo, int pageSize)
throws Exception {
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
List<User> userList = new ArrayList<User>();
if(connection != null){
StringBuffer sql = new StringBuffer();
sql.append("select u.*, r.roleName as userRoleName from smbms_user u, smbms_role r where u.userRole = r.id");
List<Object> list = new ArrayList<Object>();
if(!StringUtils.isNullOrEmpty(userName)){
sql.append(" and u.userName like ?");
list.add("%"+userName+"%");
}
if(userRole > 0){
sql.append(" and u.userRole = ?");
list.add(userRole);
}
// 排序和分页
// 分页:limit 开始下标, 页面大小
// 假如每页3个数据,我想从第2页开始: 第一页 0 1 2, 第二页 3 4 5
// 开始下标为 2 - 1 = 1, 1 * 3 = 3
// limit 3, 3
sql.append(" order by creationDate DESC limit ?,?");
currentPageNo = (currentPageNo-1)*pageSize;
list.add(currentPageNo);
list.add(pageSize);
Object[] params = list.toArray();
System.out.println("UserDaoImpl——>getUserList:" + sql.toString());
preparedStatement = connection.prepareStatement(sql.toString());
resultSet = BaseDao.query(connection, preparedStatement, params);
while(resultSet.next()){
User user = new User();
user.setId(resultSet.getInt("id"));
user.setUserCode(resultSet.getString("userCode"));
user.setUserName(resultSet.getString("userName"));
user.setGender(resultSet.getInt("gender"));
user.setBirthday(resultSet.getDate("birthday"));
user.setPhone(resultSet.getString("phone"));
user.setUserRole(resultSet.getInt("userRole"));
user.setUserRoleName(resultSet.getString("userRoleName"));
userList.add(user);
}
BaseDao.closeResource(null, preparedStatement, resultSet);
}
return userList;
}
3、UserService.java添加方法
/**
* 根据条件查询用户列表
* @param userName 用户名
* @param userRole 用户角色
* @param currentPageNo 当前页号
* @param pageSize 页面大小
* @return 用户列表
*/
public List<User> getUserList(String userName, int userRole, int currentPageNo, int pageSize);
4、UserServiceImpl.java添加方法
public List<User> getUserList(String userName, int userRole, int currentPageNo, int pageSize) {
Connection connection = null;
List<User> users = null;
System.out.println("UserServiceImpl——>getUserList:");
System.out.println("userName:"+userName);
System.out.println("userRole:"+userRole);
System.out.println("currentPageNo:"+currentPageNo);
System.out.println("pageSize:"+pageSize);
System.out.println("-------------------------------");
try {
connection = BaseDao.getConnection();
userDao.getUserList(connection, userName, userRole, currentPageNo, pageSize);
} catch (Exception e) {
e.printStackTrace();
}finally {
BaseDao.closeResource(connection, null, null);
}
return null;
}
3、获取角色列表
为了职责更好统一,把角色的操作放在另一个包里,和POJO类对应。
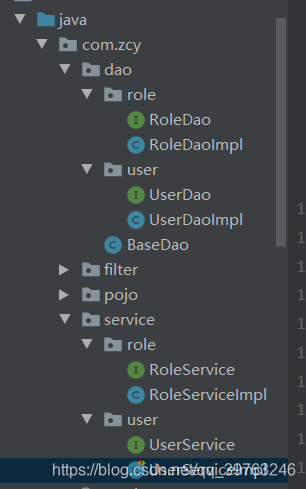
1、RoleDao.java
package com.zcy.dao.role;
import com.zcy.pojo.Role;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
public interface RoleDao {
/**
* 角色列表是固定的,只有三个数据,系统管理员1、经理2、普通员工3
* @param connection 连接参数
* @return 角色列表
*/
public List<Role> getRoleList(Connection connection) throws SQLException;
}
2、RoleDaoImpl.java
package com.zcy.dao.role;
import com.zcy.dao.BaseDao;
import com.zcy.pojo.Role;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
public class RoleDaoImpl implements RoleDao {
public List<Role> getRoleList(Connection connection) throws SQLException {
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
ArrayList<Role> list = new ArrayList<Role>();
if(connection != null){
String sql = "select * from smbms_role";
Object[] params = {
};
preparedStatement = connection.prepareStatement(sql);
resultSet = BaseDao.query(connection, preparedStatement, params);
while (resultSet.next()){
Role role = new Role();
role.setRoleName(resultSet.getString("roleName"));
role.setId(resultSet.getInt("id"));
role.setRoleCode(resultSet.getString("roleCode"));
list.add(role);
}
BaseDao.closeResource(null, preparedStatement, resultSet);
}
return list;
}
}
3、RoleService.java
package com.zcy.service.role;
import com.zcy.pojo.Role;
import java.util.List;
public interface RoleService {
//获取角色列表
public List<Role> getRoleList();
}
4、RoleServiceImpl.java
package com.zcy.service.role;
import com.zcy.dao.BaseDao;
import com.zcy.dao.role.RoleDao;
import com.zcy.dao.role.RoleDaoImpl;
import com.zcy.pojo.Role;
import java.awt.image.RasterOp;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
public class RoleServiceImpl implements RoleService {
//引入Dao
private RoleDao roleDao;
public RoleServiceImpl(){
roleDao = new RoleDaoImpl();
}
public List<Role> getRoleList() {
Connection connection = null;
List<Role> roleList = null;
try {
connection = BaseDao.getConnection();
roleList = roleDao.getRoleList(connection);
} catch (SQLException e) {
e.printStackTrace();
}finally {
BaseDao.closeResource(connection, null, null);
}
return roleList;
}
}
5、显示前面的各种列表的Servlet
在UserServlet.java中增加方法 query
//反馈给前端 用户列表、角色列表、分页(重难点)
public void query(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//获取用户前端的数据
String queryUserName = req.getParameter("queryname");
String temp = req.getParameter("queryUserRole");
String pageIndex = req.getParameter("pageIndex");
int queryUserRole = 0;//角色类型在数据库整型的,默认0,不代表任何角色
UserServiceImpl userService = new UserServiceImpl();
RoleServiceImpl roleService = new RoleServiceImpl();
int pageSize = 5;//默认页面大小显示5个数据
int currentPageNo = 1;//默认当前页为第一页
//判断请求是否需要执行,根据参数的值判断
if (queryUserName == null){
//请求中未包含用户名参数,角色名设置为空
queryUserName = "";
}
if (!StringUtils.isNullOrEmpty(temp)){
//请求包含了角色参数,将字符串转为整型
queryUserRole = new Integer(temp);
}
if (pageIndex != null){
//请求中包含页码,表明想看某一页数据,设置当前页为想看的那一页
currentPageNo = Integer.parseInt(pageIndex);
}
// 3. 为了实现分页,需要计算出当前页面、总页面、页面大小
int totalCount = userService.getUserCount(queryUserName, queryUserRole);//用户总数
//总页数支持
PageSupport pageSupport = new PageSupport();
pageSupport.setCurrentPageNo(currentPageNo);
pageSupport.setPageSize(pageSize);
pageSupport.setTotalCount(totalCount);
int totalPageCount = pageSupport.getTotalPageCount();//总页数
//控制首页和尾页,如果页面要小于1或者大于总页数,则进行限制
if (currentPageNo < 1)
currentPageNo = 1;
else if (currentPageNo > totalPageCount)
currentPageNo = totalPageCount;
//获得用户列表数据和角色数据列表
List<User> userList = userService.getUserList(queryUserName, queryUserRole, currentPageNo, pageSize);
List<Role> roleList = roleService.getRoleList();
//将数据放入request
req.setAttribute("userList", userList);
req.setAttribute("roleList", roleList);
req.setAttribute("totalCount", totalCount);
req.setAttribute("currentPageNo", currentPageNo);
req.setAttribute("totalPageCount", totalPageCount);
req.setAttribute("queryUserName", queryUserName);
req.setAttribute("queryUserRole", queryUserRole);
//返回前端,用请求转发,因为数据放在request中
req.getRequestDispatcher("userlist.jsp").forward(req, resp);
}
同时,修改doGet里的程序
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//从前端获取参数,根据参数执行对应方法
String method = req.getParameter("method");
if (method!=null && method.equals("savepwd"))
this.updatePassword(req,resp);
else if (method!=null && method.equals("pwdmodify"))
this.pwdModify(req, resp);
else if (method!=null && method.equals("query")){
this.query(req, resp);
}
}
4、添加用户
1、UserDao.java添加方法
/**
* 增加一个用户
* @param connection
* @param user
* @return 操作成功的行数
*/
public int addUser(Connection connection, User user) throws SQLException;
2、UserDaoImpl.java添加方法
public int addUser(Connection connection, User user) throws SQLException {
PreparedStatement preparedStatement = null;
int updateRows = 0;
if (connection != null){
String sql = "insert into smbms_user" +
" (userCode, userName, userPassword, gender, birthday, phone, address, userRole, creationDate, createdBy)" +
"values (?,?,?,?,?,?,?,?,?,?)";
preparedStatement = connection.prepareStatement(sql);
Object[] params = {
user.getUserCode(),user.getUserName(),user.getUserPassword(),
user.getGender(),user.getBirthday(), user.getPhone(),user.getAddress(), user.getUserRole(),
user.getCreationDate(),user.getCreatedBy()};
updateRows = BaseDao.update(connection, preparedStatement, params);
BaseDao.closeResource(null, preparedStatement, null);
}
return updateRows;
}
3、UserService.java添加方法
/**
* 添加一个用户
* @param user 用户对象
* @return true 添加成功,false 添加失败
*/
public boolean addUser(User user);
4、UserServiceImpl.java添加方法
/**
* 添加一个用户
* @param user 用户对象
* @return true 添加成功,false 添加失败
*/
public boolean addUser(User user) {
Connection connection = null;
boolean flag = false;
try {
connection = BaseDao.getConnection();
connection.setAutoCommit(false);//关闭自动提交,开启事务管理
int updateRows = userDao.addUser(connection, user);
connection.commit();//提交
if (updateRows > 0){
flag = true;
System.out.println("UserServiceImpl——>addUser:成功添加用户");
}
else
System.out.println("UserServiceImpl——>addUser:添加用户失败");
} catch (Exception e) {
e.printStackTrace();
try {
connection.rollback();
System.out.println("UserServiceImpl——>addUser:回滚");
} catch (SQLException ex) {
ex.printStackTrace();
}
}finally {
//Service层关闭connection连接
BaseDao.closeResource(connection, null, null);
}
return flag;
}
5、UserServlet.java添加方法
//添加用户
public void add(HttpServletRequest req, HttpServletResponse resp) throws IOException, ServletException {
String userCode = req.getParameter("userCode");
String userName = req.getParameter("userName");
String userPassword = req.getParameter("userPassword");
String gender = req.getParameter("gender");
String birthday = req.getParameter("birthday");
String phone = req.getParameter("phone");
String address = req.getParameter("address");
String userRole = req.getParameter("userRole");
User user = new User();
user.setUserCode(userCode);
user.setUserName(userName);
user.setUserPassword(userPassword);
user.setGender(Integer.parseInt(gender));
try {
user.setBirthday(new SimpleDateFormat("yyyy-MM-dd").parse(birthday));
} catch (ParseException e) {
e.printStackTrace();
}
user.setPhone(phone);
user.setAddress(address);
user.setUserRole(Integer.parseInt(userRole));
user.setCreationDate(new Date());
user.setCreatedBy(((User)req.getSession().getAttribute(Constants.USER_SESSION)).getId());
UserServiceImpl userService = new UserServiceImpl();
//添加成功,就直接重定向到查询页面显示数据;添加失败,则请求转发,重新添加。(请求转发能保留request的数据)
if (userService.addUser(user))
resp.sendRedirect(req.getContextPath()+"/jsp/user.do?method=query");
else
req.getRequestDispatcher("useradd.jsp").forward(req, resp);
}
总结
项目的功能没有全部实现完,整个项目逻辑比较简单,主要是将之前学的巩固练手,其他的功能实现方法都是类似的。
百度网盘:https://pan.baidu.com/s/16fNpMkGeXZud-U58Z5y67g 提取码: q3eq