JSON 是目前主流的前后端数据传输方式,尤其是现在前后端分离模式的盛行,后端返回JSON格式数据更是主流。在 Spring Boot 项目中,只要添加了 Web 依赖(spring-boot-starter-web),就可以很方便地实现 JSON 转换。
一、默认实现
Web 依赖默认加入了 jackson-databind 作为 JSON 处理器,我们不需要要添加额外的 JSON 处理器就可以返回一段 JSON。实例演示:
1、创建实体类
public class User {
private String username;
@JsonIgnore
private String password;
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
private LocalDateTime register;
// 省略 setter getter 方法
}
- @JsonIgnore:json 序列化时将 java bean 中的一些属性忽略掉。即生成 json 时不生成其标注的属性。
- @JsonFormat:将日期类型的数据格式化成指定格式的字符串。
2、创建 Controller
在这个 Contoller 中我们初始化一个 User 对象,然后将其直接返回。
@Controller
public class UserController {
@GetMapping("/user")
@ResponseBody
public User user(){
User user = new User();
user.setPassword("123456");
user.setUsername("admin");
user.setRegister(LocalDateTime.now());
return user;
}
}
- 返回 JSON格式数据 小在方法上添加 @ResponseBody注解,
- 如果不想在每个方式上都添加@ResponseBody注解,那么可以采用@RestController组合注解代替@Controller和@ResponseBody
3、启动服务,测试结果
二、自定义转换器
常见的JSON处理器除了jackson-databind之外,还有 Gson 和 fastjson。
1、使用Gson
(1)、添加依赖
Gson是Google的一个开源JSON解析框架。使用Gson,需要先除去默认的jackson-databind,然后加入Gson依赖,代码如下:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<!-- 移除jackson-databind 的依赖-->
<exclusion>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</exclusion>
</exclusions>
</dependency>
<!-- 添加 GSON 依赖 -->
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
</dependency>
- Spring Boot中默认提供了Gson的自动转换类GsonHttpMessageConvertersConfiguration,因此Gson的依赖添加成功后,可以像使用jackson-databind那样直接使用了。
- 但 Gson 进行转换时,如果想对日期数据进行格式化,那么还需要开发者自定义 HttpMessageConverter。
(2)、自定义HttpMessageConverter
@Configuration
public class GsonConfig {
@Bean
public HttpMessageConverter httpMessageConverter(){
GsonHttpMessageConverter converter = new GsonHttpMessageConverter();
GsonBuilder builder = new GsonBuilder();
//反序列化 LocalDateTime
builder.registerTypeAdapter(LocalDateTime.class, (JsonSerializer<LocalDateTime>) (src, typeOfSrc, context) -> {
String format = src.format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"));
return new JsonPrimitive(format);
});
// 解析 Date类型
builder.setDateFormat("yyyy-MM-dd HH:mm:ss");
//解析时修饰符为protected的字段被过滤掉
builder.excludeFieldsWithModifiers(Modifier.PROTECTED);
Gson gson = builder.create();
converter.setGson(gson);
return converter;
}
}
(3)、重启服务访问
2、使用fastjson
fastjson是阿里巴巴的一个开源JSON解析框架,是目前JSON解析速度最快的开源框架,该框架也可以集成到Spring Boot中。不同于Gson,fastjson继承完成之后并不能立马使用,需要开发者提供相应的HttpMessageConverter后才能使用,集成fastjson的步骤如下
扫描二维码关注公众号,回复:
12784324 查看本文章
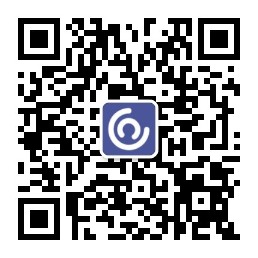
(1)、添加依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<!-- 移除jackson-databind 的依赖-->
<exclusion>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.47</version>
</dependency>
(2)、自定义HttpMessageConverter
@Configuration
public class CustomerFastJsonConfig {
@Bean
public HttpMessageConverter httpMessageConverter(){
FastJsonHttpMessageConverter converter = new FastJsonHttpMessageConverter();
FastJsonConfig config = new FastJsonConfig();
config.setDateFormat("yyyy-MM-dd HH:mm:ss");
config.setSerializerFeatures(
SerializerFeature.WriteClassName,
SerializerFeature.WriteMapNullValue,
SerializerFeature.PrettyFormat,
SerializerFeature.WriteNullListAsEmpty,
SerializerFeature.WriteNullStringAsEmpty
);
converter.setFastJsonConfig(config);
return converter;
}
}
- 可以通过在属性上添加 @JSONField(serialize=false) 注解排除某个属性序列化,类似于 jackson 中的 @JsonIgnore 注解
(3)、重启访问