1.1 项目结构

1.2 bean标签
bean标签作用
bean标签作用:配置javaBean对象。spring框架遇到bean标签,默认调用无参数构造方法实例化对象。
bean标签属性
属性 | 说明 |
---|---|
id | bean的唯一标识名称。 |
class | 类的全限定名称。 |
scope | 设置bean的作用范围。 取值: singleton : 单例,默认值。 prototype:多例 |
init-method | 指定类中初始化方法的名称,在构造方法执行完毕后立即执行。 |
destroy-method | 指定类中销毁方法名称,在销毁spring容器前执行。 |
bean作用范围
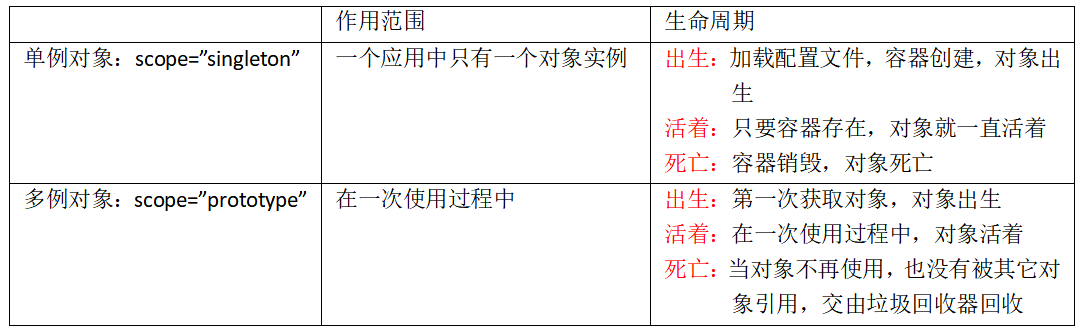
1.3 代码示例
持久层(Dao层)
dao接口
package cn.guardwhy.dao;
/**
* 客户dao接口
*/
public interface CustomerDao {
/**
* 保存客户操作
*/
void saveCustomer();
}
dao实现类
package cn.guardwhy.dao.impl;
import cn.guardwhy.dao.CustomerDao;
/**
* 客户dao实现类
*/
public class CustomerDaoImpl implements CustomerDao {
@Override
public void saveCustomer() {
System.out.println("保存客户操作");
}
}
业务层(Service)
service接口
package cn.guardwhy.service;
/**
* 客户service接口
*/
public interface CustomerService {
/**
* 保存客户操作
*/
void saveCustomer();
}
service实现类
package cn.guardwhy.service.impl;
import cn.guardwhy.dao.CustomerDao;
import cn.guardwhy.dao.impl.CustomerDaoImpl;
import cn.guardwhy.service.CustomerService;
/**
* 客户service实现类
*/
public class CustomerServiceImpl implements CustomerService {
// 定义客户dao
private CustomerDao customerDao = new CustomerDaoImpl();
/**
* 保存客户操作
*/
@Override
public void saveCustomer() {
customerDao.saveCustomer();
}
}
编写bean.xml
1.3.1 singleton标签
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--配置dao,说明:
标签:
bean:配置javaBean对象
属性:
id:bean的唯一标识名称
class:类的全路径信息
scope:设置bean的作用范围
取值:
singleton:单例(默认)
prototype:多例
细节:
默认使用无参数构造方法,创建对象
-->
<!--配置dao-->
<bean id="customerDao" class="cn.guardwhy.dao.impl.CustomerDaoImpl" scope="singleton"></bean>
</beans>
表现层(Controller)
CustomerController
package cn.guardwhy.controller;
import cn.guardwhy.dao.CustomerDao;
import cn.guardwhy.service.CustomerService;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
/**
* 客户controller
*/
public class CustomerController {
public static void main(String[] args) {
// 1.加载spring配置文件,创建ioc容器
ApplicationContext context = new ClassPathXmlApplicationContext("bean.xml");
// 2.获取两次dao对象,检查是否是同一个对象
CustomerDao customerDao1 = (CustomerDao) context.getBean("customerDao");
CustomerDao customerDao2 = (CustomerDao) context.getBean("customerDao");
System.out.println(customerDao1 == customerDao2);
// 3.条件判断
System.out.println(customerDao1.hashCode());
System.out.println(customerDao2.hashCode());
}
}
执行结果
编写bean.xml
1.3.2 prototype标签
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--配置dao,说明:
标签:
bean:配置javaBean对象
属性:
id:bean的唯一标识名称
class:类的全路径信息
scope:设置bean的作用范围
取值:
singleton:单例(默认)
prototype:多例
细节:
默认使用无参数构造方法,创建对象
-->
<!--配置dao-->
<bean id="customerDao" class="cn.guardwhy.dao.impl.CustomerDaoImpl" scope="prototype"></bean>
</beans>
执行结果
1.3.3 初始化和销毁
dao实现类
package cn.guardwhy.dao.impl;
import cn.guardwhy.dao.CustomerDao;
/**
* 客户dao实现类
*/
public class CustomerDaoImpl implements CustomerDao {
// 无参构造器:BeanFactory与ApplicationContext创建对象的区别
public CustomerDaoImpl() {
System.out.println("正在创建CustomerDaoImpl对象");
}
/**
* 初始化方法,init-method属性
*/
public void init(){
System.out.println("正在执行初始化操作....");
}
/**
* 销毁方法:destory-method属性。
*/
public void destroy(){
System.out.println("正在执行销毁操作");
}
/**
* 保存客户操作
*/
@Override
public void saveCustomer() {
System.out.println("保存客户操作");
}
}
编写bean.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--配置dao,说明:
标签:
bean:配置javaBean对象
属性:
id:bean的唯一标识名称
class:类的全路径信息
scope:设置bean的作用范围
取值:
singleton:单例(默认)
prototype:多例
init-method:初始化操作,在调用构造方法执行后执行
destroy-method:销毁操作,在销毁spring IOC容器前执行
注意:默认使用无参数构造方法,创建对象
-->
<!--配置dao-->
<bean id="customerDao" class="cn.guardwhy.dao.impl.CustomerDaoImpl" scope="singleton" init-method="init" destroy-method="destroy"></bean>
</beans>
表现层(Controller)
CustomerController
package cn.guardwhy.controller;
import cn.guardwhy.dao.CustomerDao;
import org.springframework.context.support.ClassPathXmlApplicationContext;
/**
* 客户controller
*/
public class CustomerController {
public static void main(String[] args) {
/**
bean标签作用:配置javaBean对象
属性:id:唯一标识名称,class:指定类的全路径
scope:设置bean的作用范围
属性取值:
singleton:单例。默认值
prototype:多例
init-method:初始化
destroy-method:执行销毁操作
注意:默认使用无参数构造方法实例化
*/
// 1.加载spring配置文件,创建ioc容器,大工厂接口中没有销毁的方法,在实现类中才存在
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("bean.xml");
// 2.获取dao对象
CustomerDao customerDao = (CustomerDao) context.getBean("customerDao");
// 3.保存客户
customerDao.saveCustomer();
// 4.销毁spring容器
context.close();
}
}
执行结果
4.4 import导入
这个import,一般用于团队开发使用,它可以将多个配置文件,导入合并成一个。
假设,现在项目中有多人开发。这三个人复制不同的类开发,不同的类需要注册在不同的bean中,可以用import将所有人的beans.xml合并成一个总的。
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--导入分支-->
<import resource="bean1.xml"/>
<import resource="bean2.xml"/>
<import resource="bean3.xml"/>
</beans>