问题:
难度:medium
说明:
给出一个数组,还有K,求数组内 有多少个 两两相加 得到 K值 的对数,每找到一对,就移出数组,那么数组内可以弄出多少对这样的元素。
题目连接:https://leetcode.com/problems/max-number-of-k-sum-pairs/
输入范围:
1 <= nums.length <= 105
1 <= nums[i] <= 109
1 <= k <= 109
输入案例:
Example 1:
Input: nums = [1,2,3,4], k = 5
Output: 2
Explanation: Starting with nums = [1,2,3,4]:
- Remove numbers 1 and 4, then nums = [2,3]
- Remove numbers 2 and 3, then nums = []
There are no more pairs that sum up to 5, hence a total of 2 operations.
Example 2:
Input: nums = [3,1,3,4,3], k = 6
Output: 1
Explanation: Starting with nums = [3,1,3,4,3]:
- Remove the first two 3's, then nums = [1,4,3]
There are no more pairs that sum up to 6, hence a total of 1 operation.
我的代码:
水题,一开始我想到了用 cache ,毕竟这道题确实和 tow sum 一样意思,然后我看其他代码居然还可以排序一边,然后再用双指针处理,确实高明。
先用cache:
Java:
class Solution {
private static Map<Integer, Integer> cache = new HashMap<>();
public int maxOperations(int[] nums, int k) {
int count = 0;
for(int i : nums) {
int temp = k - i;
if(temp > 0) {
if(cache.getOrDefault(temp, 0) > 0){
count ++;
cache.put(temp, cache.get(temp) - 1);
} else cache.put(i, cache.getOrDefault(i, 0) + 1);
}
}
for(Integer i : cache.keySet()) cache.put(i, 0); // 不用删除节点,暴力清零快点,java的map红黑树就是创建和删除节点麻烦
return count;
}
}
再改为 sort 和双指针:
Java:
扫描二维码关注公众号,回复:
12506180 查看本文章
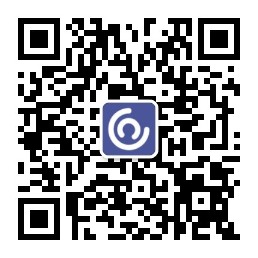
class Solution {
public int maxOperations(int[] nums, int k) {
Arrays.sort(nums);
int count = 0, left = 0, right = nums.length - 1;
while(right > left) {
if(nums[right] + nums[left] < k) left ++;
else if(nums[right] + nums[left] > k) right --;
else {
count ++; right --; left ++;
}
}
return count;
}
}
C++:
class Solution {
public:
int maxOperations(vector<int>& nums, int k) {
sort(nums.begin(), nums.end());
int count = 0, left = 0, right = nums.size() - 1;
while(right > left) {
if(nums[right] + nums[left] < k) left ++;
else if(nums[right] + nums[left] > k) right --;
else {
count ++; left ++; right --;
}
}
return count;
}
};