本篇文章来给大家介绍下C++STL中String类一些比较常用的用法。
看完别忘了一键三连
文章目录
简介
string类是C++STL库(standard template library)中专门用来处理字符串的。string类中提供了我们常用的字符串处理方法(拼接,查找子串等),也重载了一些运算符(+、>、<、>=、<=等)。
string类是一个模板类
typedef basic_string<char> string;
在使用string类是。需要包含头文件<string>
#include<string>
string对象的初始化方法
string对象的初始化主要有以下几种方式,看代码
#include<bits/stdc++.h>
using namespace std;
int main() {
// 表示一个空字符串
string str;
// 构造函数中直接传入一个字符串
string str1("str1");
// 也可以用=的方式进行赋值
string str2 = "str2";
// 表示str3为长度为8的字符串,且都是字符'a'
string str3(8,'a');
// 讲其他字符串对象赋值给str4
string str4(str1.begin(), str1.end());
char s[20] = "hello world";
// 也可以传入一个字符串数组
string str5(s);
// 用等号方式把字符串数组赋值给str6
string str6 = s;
return 0;
}
错误的初始化方法如下
#include<bits/stdc++.h>
using namespace std;
int main() {
string estr1 = 'a';
string estr2('b');
string estr3 = 3;
string estr4(4);
return 0;
}
string对象的赋值
#include<bits/stdc++.h>
using namespace std;
int main() {
string str;
str = '1';
cout << str << endl;
string str2 = "hello";
string str3 = "world";
// 将str直接赋值给str
str = str2;
cout << str << endl;
// 将str2和str3字符串进行拼接后赋值给str
str = str2 + str3;
cout << str << endl;
string str4;
// 用assign方法将str2全部复制给str4
str4.assign(str2);
cout << str4 << endl;
// 从str2下标1开始复制2个字符给str5
string str5;
str5.assign(str2, 1, 2);
cout << str5 << endl;
return 0;
}
从输入流读取字符串
#include<bits/stdc++.h>
using namespace std;
int main() {
string str;
// 可以读取连续的字符,直到遇到空字符停止
cin >> str;
string str1;
// 可以读取一整行字符,即遇到'\n'才停止
getline(cin, str1);
return 0;
}
关系运算符的使用
可以用关系运算符比较string对象的大小(== , >, >=, <, <=, !=)
这些关系运算符比较的结果也是返回bool类型,true为成立,false为不成立。
扫描二维码关注公众号,回复:
12465276 查看本文章
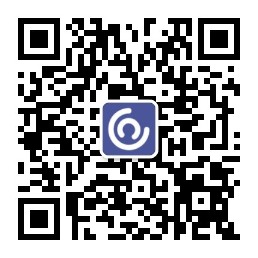
#include<bits/stdc++.h>
using namespace std;
int main() {
string str1 = "hello";
string str2 = "world";
cout << (str1 == str2) << endl;
cout << (str1 > str2) << endl;
cout << (str1 >= str2) << endl;
cout << (str1 < str2) << endl;
cout << (str1 <= str2) << endl;
cout << (str1 != str2) << endl;
return 0;
}
string类的遍历以及迭代器的使用
要访问顺序容器和关联容器中的元素,需要通过“迭代器(iterator)”进行。迭代器是一个变量,相当于容器和操纵容器的算法之间的中介。迭代器可以指向容器中的某个元素,通过迭代器就可以读写它指向的元素。从这一点上看,迭代器和指针类似
获取string类的迭代器如下
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
string::iterator it = str.begin();
return 0;
}
- str.begin()获取字符串第一个位置
- str.end()获取字符串最后一个字符的下一个位置。相当于
'\0'
利用迭代器遍历整个字符串
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
string::iterator it;
for (it = str.begin(); it != str.end(); it++) {
cout << *it << endl;
}
return 0;
}
遍历字符串除了用迭代器,也可以用遍历字符数组的方式。
string类内部重载了[]
,让我们可以像访问数组一样访问string对象中的单个字符。
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
for (int i = 0; i < str.size();i++) {
cout << str[i] << endl;
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int main() {
string str1 = "test ";
string str2 = "output ";
int a = 1111;
ostringstream outputString;
outputString << str1 << str2 << a;
cout << outputString.str() << endl;
return 0;
}
将数值遍历转成string
可以将int、double、longlong等类型遍历转成字符串
#include<bits/stdc++.h>
using namespace std;
int main() {
int a = 11;
double b = 22.22;
long long c = 33333;
string str1 = to_string(a);
string str2 = to_string(b);
string str3 = to_string(c);
cout << str1 << endl;
cout << str2 << endl;
cout << str3 << endl;
return 0;
}
字符串流处理
除了标准流和文件流输入输出外,还可以从string进行输入输出。具体实例如代码所示
#include<bits/stdc++.h>
using namespace std;
int main() {
string inputStr = "input string 1 3.1415 a";
istringstream inputString(inputStr);
string str1, str2;
int a;
double b;
char c;
inputString >> str1 >> str2 >> a >> b >> c;
cout << str1 << endl;
cout << str2 << endl;
cout << a << endl;
cout << b << endl;
cout << c << endl;
return 0;
}
string类常用的内置方法
使用size方法获取字符串长度
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
cout << (str.size()) << endl;
return 0;
}
使用assign方法进行赋值
#include<bits/stdc++.h>
using namespace std;
int main() {
string str2 = "hello";
string str3 = "world";
string str4;
// 用assign方法将str2全部复制给str4
str4.assign(str2);
cout << str4 << endl;
// 从str2下标1开始复制2个字符给str5
string str5;
str5.assign(str2, 1, 2);
cout << str5 << endl;
return 0;
}
使用append方法连接字符串
#include<bits/stdc++.h>
using namespace std;
int main() {
string str1 = "hello";
string str2 = "world";
string str3 = "hello";
// 把s2拼接到str1
str1.append(str2);
cout << str1 << endl;
// 从下标2开始,把str2中4个字符拼接到str3后面,如果不够,则到最后一次字符
str3.append(str2, 2, 4);
cout << str3 << endl;
return 0;
}
使用compare方法比较string对象大小
#include<bits/stdc++.h>
using namespace std;
int main() {
string str1 = "hello";
string str2 = "world";
string str3 = "hello";
string str4 = "hewww";
cout << (str1.compare(str2)) << endl; // -1
cout << (str2.compare(str1)) << endl; // 1
cout << (str1.compare(str3)) << endl; // 0
cout << (str3.compare(str4)) << endl; // -1
// 相当于 "he"和"he"进行比较
cout << (str3.compare(0, 1, str4, 0, 1)) << endl; // 0
// 相当于 "www"和"world"进行比较
cout << (str4.compare(2, str4.size(), str2)) << endl; // 1
return 0;
}
使用substr获得字串
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
string str1;
// 从下标1开始,5个字符
str1 = str.substr(1, 5);
cout << str1;
return 0;
}
使用find、rfind方法查找子串
- string::npos是string类中定义的静态常量,如果找不到字串,则返回string::npos
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
// find方法会从前往后找第一次出现目标串的位置,如果找到,则返回起始下标。
cout << (str.find("lo")) << endl;
// rfind方法会从后往前找第一次出现目标串的位置,如果找到,则返回起始下标。
cout << (str.rfind("o")) << endl;
// 从下标5的地方开始找字串"lo"
cout << (str.find("lo", 5)) << endl;
return 0;
}
使用find_first_not_of、find_last_not_of方法
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
// 从前向后查找不在 “aaa” 中的字母第一次出现的地方,如果找到,返回找到字母的位置,如果找不到,返回string::npos。
cout << (str.find_first_not_of("aaa")) << endl;
//从后向前查找不在 “abcd” 中的字母第一次出现的地方,如果找到,返回找到字母的位置,如果找不到,返回string::npos。
cout << (str.find_last_not_of("aaa")) << endl;
return 0;
}
使用erase方法删除字符
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
// 删除下标5以及之后的字符串
str.erase(5);
cout << str << endl;
str = "hello world";
// 从下标5开始删除1个字串
str.erase(5, 1);
cout << str << endl;
str = "hello world";
// 从str.begin()+2地方开始删除两个字符
str.erase(str.begin() + 2, str.begin() + 4);
cout << str << endl;
return 0;
}
使用replace方法替换string对象中的字符
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
// 将s1中下标0 开始的5个字符换成“nihao”
cout << (str.replace(0, 5, "nihao")) << endl;
return 0;
}
使用insert方法在string对象中插入字符
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
string str1 = "test insert";
// 将str1插入str下标4的位置
cout << (str.insert(4, str1));
return 0;
}
使用c_str获得char*字符串
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
printf("%s", str.c_str());
return 0;
}
使用algorithm中的函数处理字符串
使用swap交换两个字符串的值
#include<bits/stdc++.h>
using namespace std;
int main() {
string str1 = "hello";
string str2 = "world";
swap(str1, str2);
cout << str1 << endl;
cout << str2 << endl;
return 0;
}
使用reverse函数逆置字符串
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "hello world";
reverse(str.begin(), str.end());
cout << str;
return 0;
}
使用sort函数对string进行排序
#include<bits/stdc++.h>
using namespace std;
int main() {
string str = "advadfgasdfa";
sort(str.begin(), str.end());
cout << str << endl;
return 0;
}
拒绝白嫖,从点一键三连做起