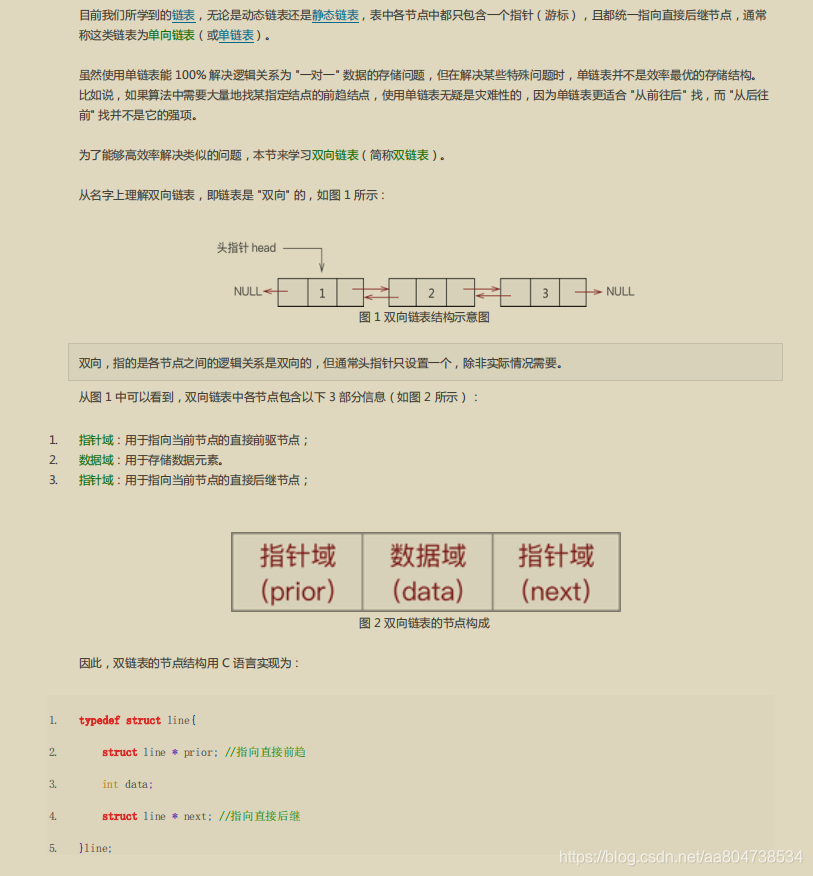
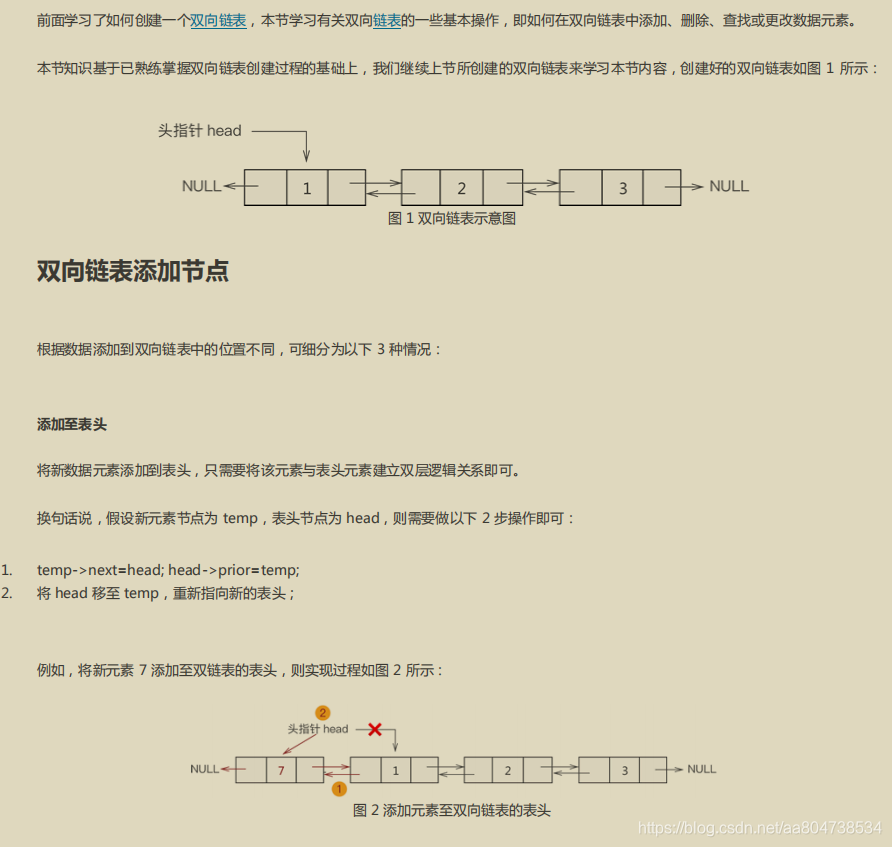
#include <stdio.h>
#include <stdlib.h>
//节点结构
typedef struct line{
struct line *prior;
int data;
struct line *next;
}line;
//双链表的创建函数
line* initLine(line *head)
{
//创建一个首元节点,链表的头指针为 head
head=(line *)malloc(sizeof(line));
//对节点进行初始化
head->prior=NULL;
head->next=NULL;
head->data=1;
//声明一个指向首元节点的指针,方便后期向链表中添加新创建的节点
line *list=head;
for(int i=2;i<=5;i++)
{
//创建新的节点并初始化
line* body=(line*)malloc(sizeof(line));
body->prior=NULL;
body->next=NULL;
body->data=i;
//新节点与链表最后一个节点建立关系
list->next=body;
body->prior=list;
//list 永远指向链表中最后一个节点
list=list->next;
}
//返回新创建的链表
return head;
}
void display(line *head)
{
line *temp=head;
while(temp)
{
//如果该节点无后继节点,说明此节点是链表的最后一个节点
if(temp->next==NULL)
{
printf("%d\n",temp->data);
}
else
{
printf("%d <-> ",temp->data);
}
temp=temp->next;
}
}
line *insertLine(line* head,int data,int add)
{
//新建数据域为 data 的结点
line *temp=(line*)malloc(sizeof(line));
temp->data=data;
temp->prior=NULL;
temp->next=NULL;
//插入到链表头,要特殊考虑
if(add==1)
{
temp->next=head;
head->prior=temp;
head=temp;
}
else
{
line *body=head;
//找到要插入位置的前一个结点
for(int i=1;i<add-1;i++)
{
body=body->next;
}
//判断条件为真,说明插入位置为链表尾
if(body->next==NULL)
{
body->next=temp;
temp->prior=body;
}
else
{
body->next->prior=temp;
temp->next=body->next;
body->next=temp;
temp->prior=body;
}
}
return head;
}
//删除
line* delLine(line* head,int data)
{
line* temp=head;
//遍历链表
while(temp)
{
//判断当前结点中数据域和 data 是否相等,若相等,摘除该结点
if(temp->data==data)
{
temp->prior->next=temp->next;
temp->next->prior=temp->prior;
free(temp);
return head;
}
temp=temp->next;
}
printf("链表中无该数据元素");
return head;
}
//查找
//head 为原双链表,elem 表示被查找元素
int selectElem(line* head,int elem)
{
//新建一个指针 t,初始化为头指针 head
line* t=head;
int i=1;
while(t)
{
if(t->data==elem)
{
printf("%d的位置在%d\r\n",elem,i);
return i;
}
i++;
t=t->next;
}
//程序执行至此处,表示查找失败
return -1;
}
//更新函数,其中,add 表示更改结点在双链表中的位置,newElem 为新数据的值
line *amendElem(line *p,int add,int newElem)
{
line* temp=p;
for(int i=1;i<add;i++)
{
temp=temp->next;
}
temp->data=newElem;
return p;
}
int main()
{
//创建一个头指针
line *head=NULL;
//调用链表创建函数
head=initLine(head);
//插入
insertLine(head,90,5);
selectElem(head,90);
amendElem(head,5,80);
//删除
// delLine(head,90);
//输出创建好的链表
display(head);
//显示双链表的优点
printf("链表中第 4 个节点的直接前驱是:%d",head->next->next->next->prior->data);
return 0;
}
root@book-virtual-machine:/mnt/hgfs/lua/C++# gcc salman_0119.cpp -o salman_0119
root@book-virtual-machine:/mnt/hgfs/lua/C++# ./salman_0119
90的位置在5
1 <-> 2 <-> 3 <-> 4 <-> 80 <-> 5
链表中第 4 个节点的直接前驱是:3root@book-virtual-machine:/mnt/hgfs/lua/C++#