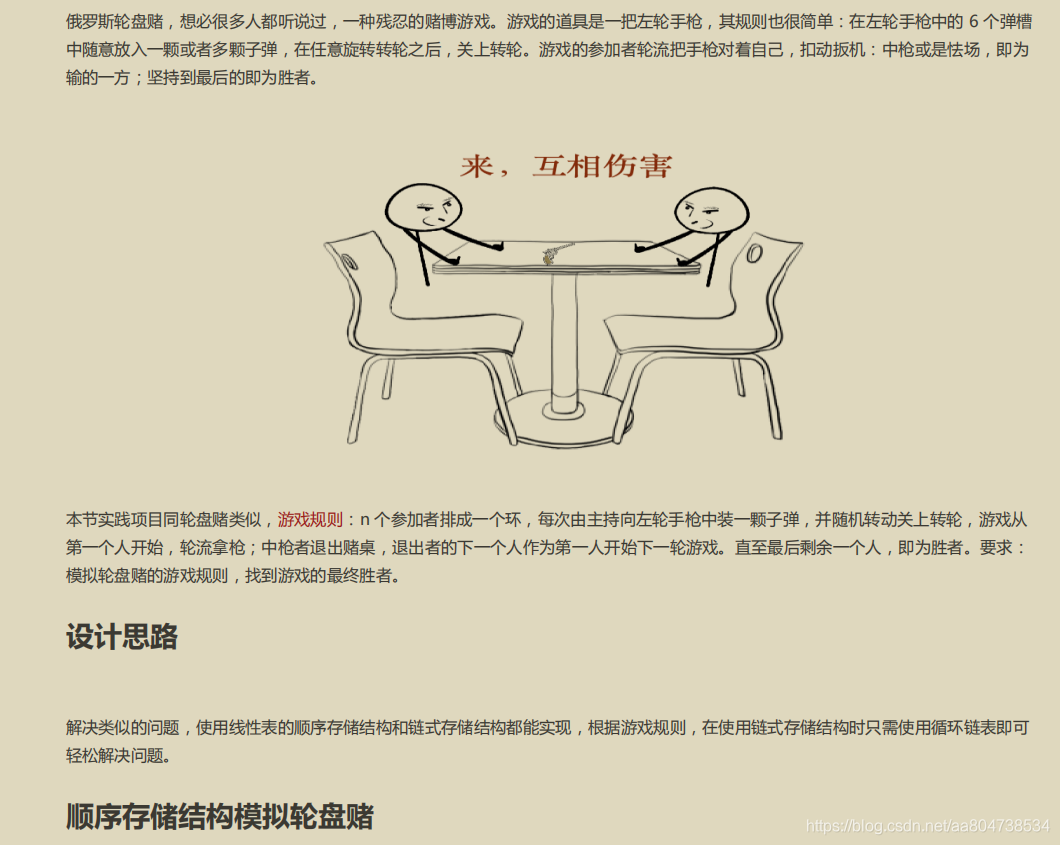
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <iostream>
using namespace std;
typedef struct gambler{
int number;
}gambler;
int main()
{
int n;
int round=1;
int location=1;
int shootNum;
int i,j;
srand((int)time(0)); //设置获得随机数的种子(固定代码,没有这句,随机数是固定不变的)
cout<<"输入赌徒的人数:"<<endl;
cin>>n;
cout<<"将赌徒依次编号为 1-"<< n << endl;
gambler gamblers[100]; //存储赌徒编号的数组
for(i=1;i<=n;i++)
{
gamblers[i].number=i;
}
//当只剩余一个人时,此场结束
while(n!=1)
{
cout<<"第"<< round <<"轮开始,从编号为"<< gamblers[location].number<<"的人开始"<<endl;
shootNum=rand()%6+1;
cout<<"枪在第"<<shootNum<<"次扣动扳机时会响"<<endl;
for(i=location;i<location+shootNum;i++);
i==i%n;//由于参与者排成的是环,所以需要对求得 i 值进行取余处理
if(i==1||i==0)//当 i=1 或者 i=0 时,实际上指的是位于数组开头和结尾的参与者,需要重新调整 i 的值
{
i=n+i;
}
cout<<"编号为"<<gamblers[i-1].number<<"的赌徒退出赌博,剩余赌徒编号依次:"<<endl;
//使用顺序存储时,如果删除元素,需要将其后序位置的元素进行全部前移
for(j=i-1;j<=n-1;j++)
{
gamblers[j]=gamblers[j+1];
}
//此时参与人数由 n 个人变为 n-1 个人
n--;
for(int k=1;k<=n;k++)
{
cout<<gamblers[k].number;
}
cout<<endl;
location=i-1; //location 表示的是下一轮开始的位置
if(location>n)
{
location%=n;
}
round++;
}
printf("最终胜利的赌徒编号是:%d\n",gamblers[1].number);
}
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
typedef struct line{
int No;
struct line* next;
}line;
//按照赌徒人数,初始化循环链表
void initLine(line **head,int n)
{
*head=(line*)malloc(sizeof(line));
(*head)->next=NULL;
(*head)->No=1;
line *list=*head;
for(int i=2;i<n;i++){
line* body=(line*)malloc(sizeof(line));
body->next=NULL;
body->No=i;
list->next=body;
list=list->next;
}
list->next=*head; //将链表成环
}
//输出链表中所有的结点信息
void display(line *head)
{
line* temp=head;
while(temp->next!=head)
{
printf("%d ",temp->No);
temp=temp->next;
}
printf("%d\n",temp->No);
}
int main()
{
line* head=NULL;
srand((int)time(0));
int n,shootNum,round=1;
printf("输入赌徒人数:");
scanf("%d",&n);
initLine(&head,n);
line* lineNext=head;
//仅当链表中只含有一个结点时,即头结点时,退出循环
while (head->next!=head) {
printf("第 %d 轮开始,从编号为 %d 的人开始,",round,lineNext->No);
shootNum=rand()%n+1;
printf("枪在第 %d 次扣动扳机时会响\n",shootNum);
line* temp=lineNext;
//遍历循环链表,找到将要删除结点的上一个结点
for (int i=1; i<shootNum-1; i++)
temp=temp->next;
//将要删除结点从链表中删除,并释放其占用空间
printf("编号为 %d 的赌徒退出赌博,剩余赌徒编号依次为:\n",temp->next->No);
line* del=temp->next;
temp->next=temp->next->next;
if(del==head)
{
head=head->next;
}
free(del);
display(head);
//赋值新一轮开始的位置
lineNext=temp->next;
round++;;//记录循环次数
}
printf("最终胜利的赌徒编号是:%d\n",head->No);
return 0;
}