目录
许多小伙伴在刚接触 Golang 的时候不理解为什么会有两个用于分配内存的函数: new 和 make,俗话说:存在即合理,下面就来详细说明下这两者的区别。
一、new
先看函数声明:
func new(Type) *Type
new 是 Golang 的内建函数,用于分配内存,其中,第一个参数是类型,返回值是类型的指针,其值被初始化为“零”(类型对应的零值,int 初始化为0,bool初始化为 false 等)。
例如:
package main
import "fmt"
func main() {
id := new(int)
name := new(string)
flag := new(bool)
fmt.Printf("id type: %T value: %v\n", id, *id)
fmt.Printf("name type: %T value: %v\n", name, *name)
fmt.Printf("flag type: %T value: %v\n", flag, *flag)
}
输出:
id type: *int value: 0
name type: *string value:
flag type: *bool value: false
从上述例子可以看到,初始化的“零”值根据类型不同而不同,整数初始化为 0,字符串初始化为空,bool类型初始化为 false。
二、make
先看函数声明:
扫描二维码关注公众号,回复:
12452847 查看本文章
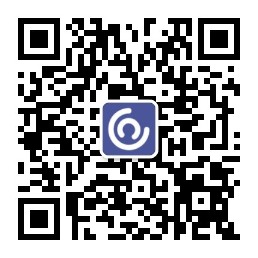
func make(t Type, size ...IntegerType) Type
make 是 Golang 的内建函数,仅用于分配和初始化 slice、map 以及 channel 类型的对象,三种类型都是结构。返回值为类型,而不是指针。
slice 源码结构:
type slice struct {
array unsafe.Pointer //指针
len int //长度
cap int //容量
}
map 源码结构:
// A header for a Go map.
type hmap struct {
// Note: the format of the hmap is also encoded in cmd/compile/internal/gc/reflect.go.
// Make sure this stays in sync with the compiler's definition.
count int // # live cells == size of map. Must be first (used by len() builtin)
flags uint8
B uint8 // log_2 of # of buckets (can hold up to loadFactor * 2^B items)
noverflow uint16 // approximate number of overflow buckets; see incrnoverflow for details
hash0 uint32 // hash seed
buckets unsafe.Pointer // array of 2^B Buckets. may be nil if count==0.
oldbuckets unsafe.Pointer // previous bucket array of half the size, non-nil only when growing
nevacuate uintptr // progress counter for evacuation (buckets less than this have been evacuated)
extra *mapextra // optional fields
}
channel 源码结构:
type hchan struct {
qcount uint // total data in the queue
dataqsiz uint // size of the circular queue
buf unsafe.Pointer // points to an array of dataqsiz elements
elemsize uint16
closed uint32
elemtype *_type // element type
sendx uint // send index
recvx uint // receive index
recvq waitq // list of recv waiters
sendq waitq // list of send waiters
// lock protects all fields in hchan, as well as several
// fields in sudogs blocked on this channel.
//
// Do not change another G's status while holding this lock
// (in particular, do not ready a G), as this can deadlock
// with stack shrinking.
lock mutex
}
例如:
package main
import "fmt"
func main() {
//map
fmt.Println("map:")
var nameId = make(map[string]int, 0)
fmt.Printf("nameId \ntype: %#v\n", nameId)
nameId["Golang"] = 1
nameId["C++"] = 2
nameId["PHP"] = 3
for name, id := range nameId {
fmt.Printf("name = %v, id = %v\n", name, id)
}
// slice
var hobby = make([]string, 2, 100) // 其中 2是长度,100是容量
hobby[0] = "打篮球"
hobby[1] = "乒乓球"
fmt.Println("\nslice:")
fmt.Printf("length = %v caps = %v\n", len(hobby), cap(hobby))
for _, name := range hobby {
fmt.Println(name)
}
// channel
ch := make(chan int, 3)
ch <- 1
ch <- 2
ch <- 8
close(ch)
fmt.Println("\nchannel:")
for val := range ch { // 遍历数据
fmt.Println(val)
}
}
输出:
[root@localhost test]# go run main.go
map:
nameId
type: map[string]int{}
name = Golang, id = 1
name = C++, id = 2
name = PHP, id = 3
slice:
length = 2 caps = 100
打篮球
乒乓球
channel:
1
2
8
[root@localhost test]#
三、new 和 make的区别
1. new 和 make 都用于分配内存;
2. new 和 make 都是在堆上分配内存;
3. new 对指针类型分配内存,返回值是分配类型的指针,new不能直接对 slice 、map、channel 分配内存;
4. make 仅用于 slice、map和 channel 的初始化,返回值为类型本身,而不是指针;