1.前言
先来介绍一下栈和队列的区别。
栈:元素先进后出,从栈顶入栈和出栈;
队列:元素先进先出,元素从从队尾出队,从队首出队;
接下来将描述用栈模拟队列以及用队列模拟栈的实现过程;
2.栈模拟实现队列的过程
实现代码:
typedef int STDataType;
typedef struct Stack
{
STDataType *arr;
int _top;
int _capacite;
}stack;
void StackInit(stack *ps)//初始化
{
assert(ps);
STDataType *node = (STDataType*)malloc(sizeof(STDataType)* 2);
assert(node);
ps->arr = node;
ps->_top = 0;
ps->_capacite = 2;
}
void StackDestory(stack *ps)//销毁
{
assert(ps);
free(ps->arr);
ps->arr = NULL;
ps->_top = ps->_capacite = 0;
}
void StackPush(stack *ps,STDataType data)//入栈
{
assert(ps);
if (ps->_top == ps->_capacite)
{
ps->_capacite *= 2;
ps->arr = (STDataType*)realloc(ps->arr, sizeof(STDataType)*ps->_capacite);
}
ps->arr[ps->_top++] = data;
}
STDataType StackTop(stack *ps)//获取栈顶元素
{
assert(ps);
assert(ps->_top != 0);
return ps->arr[ps->_top-1];
}
void StackPop(stack *ps)//出栈
{
assert(ps);
assert(ps->_top != 0);
ps->_top--;
}
int StackSize(stack *ps)//获取栈内元素个数
{
assert(ps);
return ps->_top;
}
int StackEmpty(stack *ps)//判断是否为空
{
assert(ps);
if (ps->_top == 0)
return 1;
else
return 0;
}
typedef struct {
stack first_s;
stack second_s;
} MyQueue;
/** Initialize your data structure here. */
MyQueue* myQueueCreate() {
MyQueue* obj=(MyQueue*)malloc(sizeof(MyQueue));
//初始化栈
StackInit(&obj->first_s);
StackInit(&obj->second_s);
return obj;
}
/** Push element x to the back of queue. */
void myQueuePush(MyQueue* obj, int x) {
StackPush(&obj->first_s,x);//数据都从存入到栈1之中
}
int myQueuePeek(MyQueue* obj);
/** Removes the element from in front of queue and returns that element. */
int myQueuePop(MyQueue* obj) {
int front=myQueuePeek(obj);//调用写好的接口,减少代码冗余
StackPop(&obj->second_s);
return front;
}
/** Get the front element. */
int myQueuePeek(MyQueue* obj) {
if(StackEmpty(&obj->second_s))//栈二为空则从栈1导入元素
{
while(!StackEmpty(&obj->first_s))
{
int temp=StackTop(&obj->first_s);
StackPush(&obj->second_s,temp);
StackPop(&obj->first_s);
}
}
return StackTop(&obj->second_s);
}
/** Returns whether the queue is empty. */
bool myQueueEmpty(MyQueue* obj) {
//都为空才是空
return (StackEmpty(&obj->first_s)&&StackEmpty(&obj->second_s));
}
void myQueueFree(MyQueue* obj) {
StackDestory(&obj->first_s);
StackDestory(&obj->second_s);
free(obj);
}
}
3.队列模拟栈的过程
3.1一个队列模拟栈的过程
实现代码:
typedef int QDataType;
typedef struct QListNode
{
QDataType _data;
struct QListNode* _next;
}QNode;
typedef struct Queue
{
QNode *_front;
QNode *_rear;
}Queue;
void QueueInit(Queue *q)//初始化
{
assert(q);
q->_front = q->_rear = NULL;
}
void QueueDestory(Queue *q)//销毁
{
assert(q);
QNode *cur = q->_front;
QNode *next = NULL;
while (cur)
{
next = cur->_next;
free(cur);
cur = next;
}
q->_front = q->_rear = NULL;
}
void QueuePush(Queue *q, QDataType data)//入队
{
QNode *node = (QNode*)malloc(sizeof(QNode));
assert(node);
node->_data = data;
node->_next = NULL;
if (q->_front == NULL)//第一个
{
q->_front = q->_rear = node;
}
else
{
q->_rear->_next = node;
q->_rear = node;
}
}
void QueuePop(Queue *q)//出队
{
assert(q);
assert(q->_front);
QNode *next = q->_front->_next;
free(q->_front);
q->_front = next;
if (q->_front == NULL)
q->_rear = NULL;//队为空
}
QDataType QueueFront(Queue *q)//获取队首元素
{
assert(q);
assert(q->_front);
return q->_front->_data;
}
QDataType QueueBack(Queue *q)//获取队尾元素
{
assert(q);
assert(q->_rear);
return q->_rear->_data;
}
int QueueSize(Queue *q)//获取元素个数
{
assert(q);
QNode *cur = q->_front;
int size = 0;
while (cur)
{
cur = cur->_next;
size++;
}
return size;
}
int QueueEmpty(Queue *q)//判断是否为空
{
assert(q);
if (q->_front == NULL)
return 1;
else
return 0;
}
typedef struct {
Queue q;
} MyStack;
/** Initialize your data structure here. */
MyStack* myStackCreate() {
MyStack* obj=(MyStack*)malloc(sizeof(MyStack));
QueueInit(&obj->q);//初始化
return obj;
}
/** Push element x onto stack. */
void myStackPush(MyStack* obj, int x) {
//先入栈,再将其其余的元素出队再入队
QueuePush(&obj->q,x);
int size=QueueSize(&obj->q);//获取队列中元素个数
while(size>1)
{
int temp=QueueFront(&obj->q);//获取队首元素
QueuePop(&obj->q);//出队
QueuePush(&obj->q,temp);//再入队
size--;
}
}
/** Removes the element on top of the stack and returns that element. */
int myStackPop(MyStack* obj) {
//出栈
int data=QueueFront(&obj->q);
QueuePop(&obj->q);
return data;
}
/** Get the top element. */
int myStackTop(MyStack* obj) {
return QueueFront(&obj->q);
}
/** Returns whether the stack is empty. */
bool myStackEmpty(MyStack* obj) {
if(QueueEmpty(&obj->q))
return true;
return false;
}
void myStackFree(MyStack* obj) {
QueueDestory(&obj->q);
free(obj);
}
3.2用两个队列来模拟栈的过程
实现代码:
扫描二维码关注公众号,回复:
12414171 查看本文章
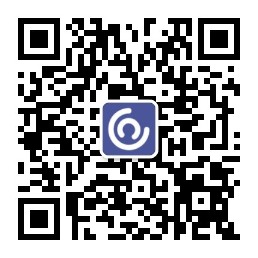
typedef int QDataType;
typedef struct QListNode
{
QDataType _data;
struct QListNode* _next;
}QNode;
typedef struct Queue
{
QNode *_front;
QNode *_rear;
}Queue;
void QueueInit(Queue *q)//初始化
{
assert(q);
q->_front = q->_rear = NULL;
}
void QueueDestory(Queue *q)//销毁
{
assert(q);
QNode *cur = q->_front;
QNode *next = NULL;
while (cur)
{
next = cur->_next;
free(cur);
cur = next;
}
q->_front = q->_rear = NULL;
}
void QueuePush(Queue *q, QDataType data)//入队
{
QNode *node = (QNode*)malloc(sizeof(QNode));
assert(node);
node->_data = data;
node->_next = NULL;
if (q->_front == NULL)//第一个
{
q->_front = q->_rear = node;
}
else
{
q->_rear->_next = node;
q->_rear = node;
}
}
void QueuePop(Queue *q)//出队
{
assert(q);
assert(q->_front);
QNode *next = q->_front->_next;
free(q->_front);
q->_front = next;
if (q->_front == NULL)
q->_rear = NULL;//队为空
}
QDataType QueueFront(Queue *q)//获取队首元素
{
assert(q);
assert(q->_front);
return q->_front->_data;
}
QDataType QueueBack(Queue *q)//获取队尾元素
{
assert(q);
assert(q->_rear);
return q->_rear->_data;
}
int QueueSize(Queue *q)//获取元素个数
{
assert(q);
QNode *cur = q->_front;
int size = 0;
while (cur)
{
cur = cur->_next;
size++;
}
return size;
}
int QueueEmpty(Queue *q)//判断是否为空
{
assert(q);
if (q->_front == NULL)
return 1;
else
return 0;
}
typedef struct {
Queue first_q;//创建队列;
Queue second_q;
} MyStack;
/** Initialize your data structure here. */
MyStack* myStackCreate() {
MyStack *ms=(MyStack *)malloc(sizeof(MyStack));
QueueInit(&ms->first_q);//初始化队列
QueueInit(&ms->second_q);
return ms;
}
/** Push element x onto stack. */
void myStackPush(MyStack* obj, int x) {
//入到非空队列
if(!QueueEmpty(&obj->first_q))
QueuePush(&obj->first_q,x);
else
QueuePush(&obj->second_q,x);
}
/** Removes the element on top of the stack and returns that element. */
int myStackPop(MyStack* obj) {
Queue *empty=&obj->first_q;
Queue *unempty=&obj->second_q;
if(!QueueEmpty(empty))//不为空则交换过来
{
empty=&obj->second_q;
unempty=&obj->first_q;
}
int size=QueueSize(unempty);
while(size>1)//将元素全部入队到空队列,只剩下一个
{
QueuePush(empty,QueueFront(unempty));
QueuePop(unempty);
size--;
}
int ret=QueueFront(unempty);
QueuePop(unempty);
return ret;
}
/** Get the top element. */
int myStackTop(MyStack* obj) {
//两个队列一个为空,一个不为空
if(!QueueEmpty(&obj->first_q))
{
return QueueBack(&obj->first_q);
}
return QueueBack(&obj->second_q);
}
/** Returns whether the stack is empty. */
bool myStackEmpty(MyStack* obj) {
return QueueEmpty(&obj->first_q)&&QueueEmpty(&obj->second_q);//都为空才是空
}
void myStackFree(MyStack* obj) {
QueueDestory(&obj->first_q);
QueueDestory(&obj->second_q);
free(obj);
}