Scrollview标题栏滑动渐变
仿京东样式(上滑显示下滑渐变消失)
/**
* @ClassName MyScrollView
* @Author Rex
* @Date 2021/1/27 17:38
*/
public class MyScrollView extends ScrollView {
private TranslucentListener mTranslucentListener;
public void setTranslucentListener(TranslucentListener translucentListener) {
this.mTranslucentListener = translucentListener;
}
public MyScrollView(Context context) {
this(context, null);
}
public MyScrollView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public MyScrollView(Context context, AttributeSet attrs, int defStyleAttr) {
this(context, attrs, defStyleAttr, 0);
}
public MyScrollView(Context context, AttributeSet attrs, int defStyleAttr, int defStyleRes) {
super(context, attrs, defStyleAttr, defStyleRes);
}
@Override
protected void onScrollChanged(int l, int t, int oldl, int oldt) {
super.onScrollChanged(l, t, oldl, oldt);
if (mTranslucentListener != null) {
//ScrollView滑出高度
int scrollY = getScrollY();
//屏幕高度
int screenHeight = getContext().getResources().getDisplayMetrics().heightPixels;
//有效滑动距离为屏幕2分之一
// alpha = 滑动高度/(screenHeight/3f)
if (scrollY <= screenHeight / 2f) {
Log.d(">>>>>>>>>", "ScrollView划出高度:" + scrollY);
Log.d(">>>>>>>>>", "屏幕高度:" + screenHeight);
Log.d(">>>>>>>>>", "渐变值:" + (0 + scrollY / (screenHeight / 4f)));
// 渐变的过程 1~0
mTranslucentListener.onTranslucent(0 + scrollY / (screenHeight /4f));
}
}
}
}
Activity 设置
public class ToolbarActivity extends AppCompatActivity implements TranslucentListener {
private Toolbar mToolBar;
private MyScrollView mScrollView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_toobar);
mToolBar = findViewById(R.id.id_toolbar);
mScrollView = findViewById(R.id.id_scrollView);
//初始化渐变为0
mToolBar.setAlpha(0);
//设置渐变回调
mScrollView.setTranslucentListener(this);
}
@Override
public void onTranslucent(float alpha) {
mToolBar.setAlpha(alpha);
}
}
渐变回调接口
/**
* @ClassName TranslucentListener
* @Author rex
* @Date 2021/1/27 17:38
*/
public interface TranslucentListener {
/**
* 透明度的回调监听
*
* @param alpha 0~1 透明度
*/
public void onTranslucent(float alpha);
}
布局文件
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.rex.rxhttpdemo.MyScrollView
android:id="@+id/id_scrollView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:clipChildren="false"
android:clipToPadding="false"
>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button0" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button1" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button2" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button3" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button4" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button5" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button5" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button5" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button5" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button5" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button5" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button5" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button5" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
<Button
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Button" />
</LinearLayout>
</com.rex.rxhttpdemo.MyScrollView>
<androidx.appcompat.widget.Toolbar
android:id="@+id/id_toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/colorAccent"
app:title="title"
/>
</RelativeLayout>
下滑显示上滑渐变消失
/**
* @ClassName MyScrollView
* @Author Rex
* @Date 2021/1/27 17:38
*/
public class MyScrollView extends ScrollView {
private TranslucentListener mTranslucentListener;
public void setTranslucentListener(TranslucentListener translucentListener) {
this.mTranslucentListener = translucentListener;
}
public MyScrollView(Context context) {
this(context, null);
}
public MyScrollView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public MyScrollView(Context context, AttributeSet attrs, int defStyleAttr) {
this(context, attrs, defStyleAttr, 0);
}
public MyScrollView(Context context, AttributeSet attrs, int defStyleAttr, int defStyleRes) {
super(context, attrs, defStyleAttr, defStyleRes);
}
@Override
protected void onScrollChanged(int l, int t, int oldl, int oldt) {
super.onScrollChanged(l, t, oldl, oldt);
if (mTranslucentListener != null) {
//ScrollView滑出高度
int scrollY = getScrollY();
//屏幕高度
int screenHeight = getContext().getResources().getDisplayMetrics().heightPixels;
//有效滑动距离为屏幕2分之一
// alpha = 滑动高度/(screenHeight/3f)
if (scrollY <= screenHeight / 2f) {
Log.d(">>>>>>>>>", "ScrollView划出高度:" + scrollY);
Log.d(">>>>>>>>>", "屏幕高度:" + screenHeight);
Log.d(">>>>>>>>>", "渐变值:" + (1 - scrollY / (screenHeight / 4f)));
// 渐变的过程 1~0
mTranslucentListener.onTranslucent(1 - scrollY / (screenHeight /4f));
}
}
}
}
注意: 这里只是更改了 mTranslucentListener.onTranslucent 里的 渐变值
Activty 里 把初始化 mToolBar.setAlpha(0); 去掉
XML
<com.rex.rxhttpdemo.MyScrollView
android:id="@+id/id_scrollView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:clipChildren="false"
android:clipToPadding="false"
android:paddingTop="?attr/actionBarSize"
>
</com.rex.rxhttpdemo.MyScrollView>
xml 加入 paddingtop .
扫描二维码关注公众号,回复:
12395064 查看本文章
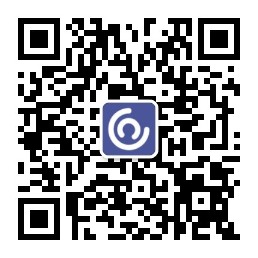
注意:
android:clipChildren=“false”
android:clipToPadding="false"
这俩个属性 如果不加会有留白
到这里结束了