【数组排序算法】
1.冒泡排序
int[] arr = { 50, 60, 8, 5, 46, 90, 87, 13, 16, 14};
Console.WriteLine("原始数组为:");
for(int i=0;i<arr.Length;i++)
{
Console.Write(arr[i]+" ");
}
int temp;
for(int i=0;i<arr.Length-1;i++)
{
for(int j=i+1;j<arr.Length;j++)
{
if(arr[i]>arr[j]) //若实现逆向排序就把>换成<
{
temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
Console.WriteLine(" ");
Console.WriteLine("排序后的数组为:");
for (int i = 0; i < arr.Length; i++)
{
Console.Write(arr[i] + " ");
}
Console.ReadLine();
2.选择排序
int[] arr = { 50, 60, 8, 5, 46, 90, 87, 13, 16, 14 };
Console.WriteLine("原始数组为:");
for (int i = 0; i < arr.Length; i++)
{
Console.Write(arr[i] + " ");
}
int min;
for (int i = 0; i < arr.Length - 1; i++)
{
min = i;
for (int j = i + 1; j < arr.Length; j++)
{
int temp;
if (arr[min] > arr[j])
{
temp = arr[min];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
Console.WriteLine(" ");
Console.WriteLine("排序后的数组为:");
for (int i = 0; i < arr.Length; i++)
{
Console.Write(arr[i] + " ");
}
Console.ReadLine();
【ArrayList类】
命名空间为System.Collections。
可以定义动态数组,但只能是一维数组,可以添加和删除元素。
1.声明动态数组
语法一:
ArrayList 对象名 = new ArrayList();
ArrayList a = new ArrayList();
for(int i =0;i<10;i++) //给ArrayList对象添加10个int元素
{
List.Add(i);
}
语法二:
ArrayList 对象名 = new ArrayList(数组名);
int[] arr = {1,2,3,4,5,6};
ArrayList List = new ArrayList(arr);
语法三:
ArrayList 对象名 = new ArrayList(对象的空间大小);
ArrayList List = new ArrayList(10);//指定对象空间为10个元素
for(int i = 0;i<List.Length;i++)//添加10个int元素
{
List.Add(i);
}
2.ArrayList元素的添加
1)Add方法
用于添加元素至数组结尾处
int[] a = {1,2,3,4,5,6};
ArrayList List = new ArrayList(a);
List.Add(7);//向对象中添加元素7
foreach(int i in List)//遍历数组
{
Console.Write(i+" ");
}
Console.ReadLine();
2)Insert方法
用于将元素插入到指定数组索引处。
int[] a = {1,2,3,4,5,6};
ArrayList List = new ArrayList(a);
List.Insert(3,7);//在数组第3索引处插入元素7
foreach(int i in List)//遍历数组
{
Console.Write(i+" ");
}
Console.ReadLine();
3.ArrayList元素的删除
1)Clear方法
用于移除数组中所有元素
int[] a = {1,2,3,4,5,6};
ArrayList List = new ArrayList(a);
List.Clear();//移除所有元素
2)Remove方法
用于移除数组中与特定对象的第一个匹配项。
int[] a = {3, 1, 2, 3, 4, 5, 6 };
ArrayList List = new ArrayList(a);
List.Remove(3);//移除数组中与3匹配的第一个数
foreach (int i in List)//遍历数组
{
Console.Write(i + " ");
}
Console.ReadLine();
3)RemoveAt方法
用于移除指定索引处的元素。
int[] a = {1, 2, 3, 4, 5, 6 };
ArrayList List = new ArrayList(a);
List.RemoveAt(3);//索引从0开始,移除第3个数
foreach (int i in List)//遍历数组
{
Console.Write(i + " ");
}
Console.ReadLine();
4)RemoveRange方法
用于移除一定范围的数。
扫描二维码关注公众号,回复:
11927588 查看本文章
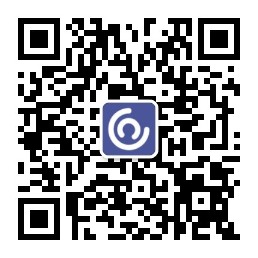
int[] a = {1, 2, 3, 4, 5, 6 };
ArrayList List = new ArrayList(a);
List.RemoveRange(3,2);//移除索引3开始的2个元素
foreach (int i in List)//遍历数组
{
Console.Write(i + " ");
}
Console.ReadLine();
4.ArrayList元素的查找
Contains方法。返回值为bool类型。
int[] arr = {1,2,3,4,5,6};
ArrayList List = new ArrayList(arr);
Console.WriteLine(List.Contains(2));//判断数组中是否存在元素2
【Hashtable哈希表】
键 值对的集合。键不可以为空引用,值可以为空。
Hashtable对象中一个元素表示一对键值。
声明Hashtable对象:
Hashtable 对象名 = new Hashtable();
Hashtable 对象名 = new Hashtable(元素个数);
1.Hashtable元素的添加
使用Add方法。
Hashtable ha = new Hashtable();
ha.Add("id","123"); //添加元素,一对键值
ha.Add("name","ZFL");
ha.Add("sex","男");
(Hashtable的遍历)
使用foreach语句,要注意Hashtable中的元素是键值对,需要使用DictionaryEntry结构来进行遍历。DictionaryEntry表示一个键值对的集合。
Hashtable ha = new Hashtable();
ha.Add("id","123"); //添加元素,一对键值
ha.Add("name","ZFL");
ha.Add("sex","男");
Console.WriteLine("键\t 值");
foreach(DictionaryEntry di in ha)
{
Console.WriteLine(di.Key+"\t"+di.Value);
}
2.Hashtable元素的删除
1)Clear方法
删除所有元素
Hashtable ha = new Hashtable();
ha.Add("id","123"); //添加元素,一对键值
ha.Add("name","ZFL");
ha.Add("sex","男");
ha.Clear(); //移除所有元素
2)Remove方法
移除指定键的元素
Hashtable ha = new Hashtable();
ha.Add("id","123"); //添加元素,一对键值
ha.Add("name","ZFL");
ha.Add("sex","男");
ha.Remove(“sex”); //移除键为sex的元素
Console.WriteLine("键\t 值");
foreach(DictionaryEntry di in ha)
{
Console.WriteLine(di.Key+"\t"+di.Value);
}
3.Hashtable元素的查找
1)Contains方法
查找是否包含指定键。
Hashtable ha = new Hashtable();
ha.Add("id","123"); //添加元素,一对键值
ha.Add("name","ZFL");
ha.Add("sex","男");
Console.WriteLine(ha.Contains("id"));//返回true
Console.WriteLine(ha.ContainsValue("123"));//返回false
2)ContainsValue方法
查找是否包含指定值
Hashtable ha = new Hashtable();
ha.Add("id","123"); //添加元素,一对键值
ha.Add("name","ZFL");
ha.Add("sex","男");
Console.WriteLine(ha.ContainsValue("123"));//返回true
Console.WriteLine(ha.Contains("id"));//返回false