程序运行结果:
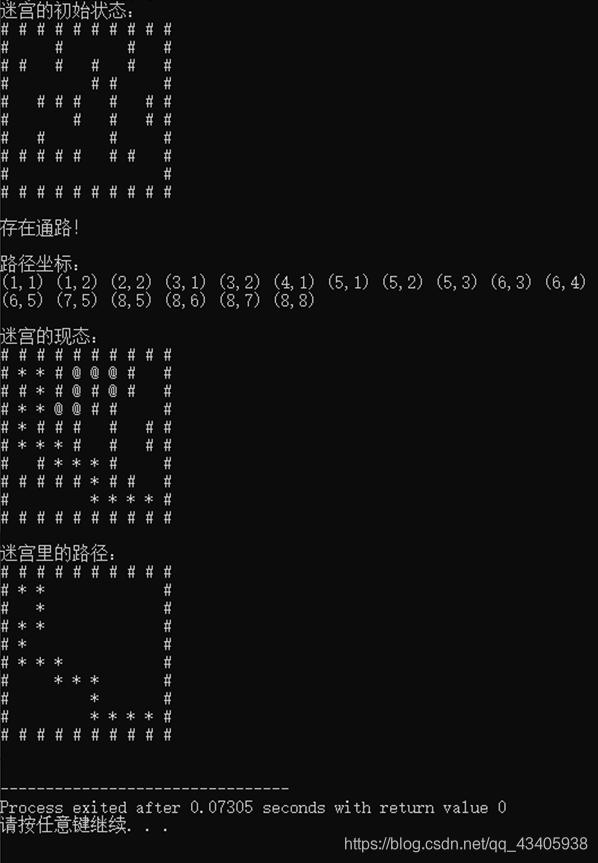
算法思想及程序实现:
- 求迷宫路径算法的基本思想:若当前位置“可通”,则纳入路径,继续前进;
若当前位置“不可通”,则后退,换方向继续探索; 若四周“均无通路”,则将当前位置从路径中删除出去。
- 定义相关结构体:
typedef struct {
int x;
int y;
} PosType;
typedef struct {
PosType seat;
int direction;
} SElemType;
typedef struct SqStack {
SElemType data[MAXSIZE];
int top;
} SqStack;
- 通过二维数组定义迷宫,并设置起点和终点坐标,(’ ‘表示通道块,’#‘表示墙壁,在后面的执行过程中,迷宫的元素可能变成’*‘表示路径,’@'表示曾经走过但是无法到达出口):
static char maze[mazeRowNum][mazeRowNum] = {
{
'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'},
{
'#', ' ', ' ', '#', ' ', ' ', ' ', '#', ' ', '#'},
{
'#', '#', ' ', '#', ' ', '#', ' ', '#', ' ', '#'},
{
'#', ' ', ' ', ' ', ' ', '#', '#', ' ', ' ', '#'},
{
'#', ' ', '#', '#', '#', ' ', '#', ' ', '#', '#'},
{
'#', ' ', ' ', ' ', '#', ' ', '#', ' ', '#', '#'},
{
'#', ' ', '#', ' ', ' ', ' ', '#', ' ', ' ', '#'},
{
'#', '#', '#', '#', '#', ' ', '#', '#', ' ', '#'},
{
'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{
'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'}
};
PosType start = {
1, 1};
PosType end = {
8, 8};
- 对曾经走过的通道块留下痕迹,以防止所求路径不是简单路径:
void footPrint(PosType curpos) {
maze[curpos.x][curpos.y] = '*';
}
- 曾走过的通道块但是无法到达出口,是“不通的”路,标记以免陷入“死胡同”:
void markPrint(PosType curpos) {
maze[curpos.x][curpos.y] = '@';
}
- 定义函数实现从当前位置“行走”到下一个位置:
PosType nextPos(PosType curpos, int direction) {
switch(direction) {
case 1:
curpos.y++;
break;
case 2:
curpos.x++;
break;
case 3:
curpos.y--;
break;
case 4:
curpos.x--;
break;
}
return curpos;
}
- 判断当前位置是否可通,即为’ ‘,而不是’#’、’*’(已走过)、’@’(已走过但不通):
bool pass(PosType curpos) {
if(maze[curpos.x][curpos.y] == ' ')
return true;
else
return false;
}
- 若迷宫中存在从入口start到出口end的通道,则求得一条路径存放在栈中(从栈底到栈顶),并返回TRUE,否则返回FALSE:
bool mazePath(PosType start, PosType end) {
SqStack s;
initStack(s);
PosType curpos = start;
do {
if(pass(curpos)) {
footPrint(curpos);
SElemType e;
e.seat = curpos;
e.direction = 1;
push(s, e);
if(curpos.x == end.x && curpos.y == end.y)
return true;
curpos = nextPos(curpos, 1);
} else {
SElemType e;
pop(s, e);
while(e.direction == 4 && !isEmpty(s)) {
markPrint(e.seat);
pop(s, e);
}
if(e.direction < 4) {
e.direction++;
push(s, e);
curpos = nextPos(e.seat, e.direction);
}
}
} while(!isEmpty(s));
return false;
}
- 定义函数打印迷宫:
void printMaze() {
for(int i = 0; i < mazeRowNum; i++) {
for(int j = 0; j < mazeColNum; j++) {
printf("%c ", maze[i][j]);
}
printf("\n");
}
printf("\n");
}
void printPath() {
for(int i = 0; i < mazeRowNum; i++) {
for(int j = 0; j < mazeColNum; j++) {
if(i == 0 || j == 0 || i == mazeRowNum-1 || j == mazeColNum-1 || maze[i][j] == '*') {
printf("%c ", maze[i][j]);
} else {
printf(" ");
}
}
printf("\n");
}
printf("\n");
}
void printPathOrder() {
for(int i = 0; i < mazeRowNum; i++) {
for(int j = 0; j < mazeColNum; j++) {
if( maze[i][j] == '*') {
printf("(%d,%d) ", i,j);
}
}
}
printf("\n");
}
- 主函数
int main() {
printf("迷宫的初始状态:\n");
printMaze();
if(mazePath(start, end)) {
printf("存在通路!\n\n");
printf("路径坐标:\n");
printPathOrder();
printf("\n迷宫的现态:\n");
printMaze();
printf("迷宫里的路径:\n");
printPath();
} else
printf("不存在通路!\n");
return 0;
}