一,高可用
1,zookeeper宕机
现象:
zookeeper注册中心宕机,还可以消费dubbo暴露的服务。
注册中心全部宕掉后,服务提供者和服务消费者仍能通过本地缓存通讯。
2,集群下dubbo负载均衡配置
3,服务降级
4,集群容错
整合hystrix
二,原理
1,服务消费方(client)以本地方式调用服务
2,client agent接收到调用后,将方法、参数等组装成能够进行网络传输的消息体。
3,client agent找到服务地址,并将消息发送到服务端。
4,server agent收到消息后进行解码。
5,server agent根据解码结果调用本地的服务。
6,本地服务执行并将结果返回给server agent。
7,server agent将返回结果打包成消息并发送到消费方。
8,client agent接收到消息,并进行解码。
9,服务消费方得到最终结果。
RPC框架的目标就是把2--8这些步骤都封装起来。
dubbo是基于netty(NIO)进行通信的。
三,服务提供方
1,pom.xml
<!-- 父级依赖 -->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.4.RELEASE</version>
<relativePath />
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<!-- 使用springmvc和spring的jar包 -->
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.boot</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>0.2.0</version>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
<version>2.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>com.yu.taobao</groupId>
<artifactId>taobao-interface</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
2,application.properties
server.port=8096
dubbo.application.name=boot-user-provider
dubbo.registry.address=zookeeper://127.0.0.1:2181
dubbo.protocol.name=dubbo
dubbo.protocol.port=20882
dubbo.monitor.protocol=registry
3,ApplicationController
/*
* springboot与dubbo整合
*1): 导入引用
* 1,导入dubbo-starter,在application.properties中配置属性,
* 使用@Service暴露服务,使用@Reference应用服务
* @EnableDubbo
* 2,导入其它引用
* 2):
* 保留dubbo xml配置,去掉application.properties中配置
* 使用@ImportResource(locations="classpath:provider.xml")
* 暴露服务不用@Service
* 3):
* 使用注解API
*/
@EnableDubbo //开启基于注解的dubbo功能
@EnableHystrix //开启服务容错
@EnableAsync //开启异步调用
@SpringBootApplication(scanBasePackages= {"com.taobao.*"},
exclude= {RedisAutoConfiguration.class})
public class ApplicationController {
//入口
public static void main(String[] args) {
SpringApplication.run(ApplicationController.class, args);
}
}
4,UserServiceImpl
/*
*1,将服务提供者注册到注册中心
* a,导入dubbo,zookeeper依赖
* b,配置服务提供者
*2,让服务消费者去注册中心订阅服务提供者的服务地址
*
*/
@Service //暴露服务
@Component
public class UserServiceImpl implements UserService {
@HystrixCommand
public List<UserAddress> getUserAddressList(String userId) {
System.out.println("3号");
UserAddress userAddress1=new UserAddress(1, "合肥", "1", "李四", "123654789", "Y");
UserAddress userAddress2=new UserAddress(2, "淮北", "1", "张三", "231133333", "N");
if(Math.random()>0.5) {
throw new RuntimeException();
}
return Arrays.asList(userAddress1,userAddress2);
}
}
四,服务消费者
1,pom.xml
<!-- 父级依赖 -->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.4.RELEASE</version>
<relativePath />
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<!-- 使用springmvc和spring的jar包 -->
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.boot</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>0.2.0</version>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
<version>2.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>com.yu.taobao</groupId>
<artifactId>taobao-interface</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
2,application.properties
server.port=8092
dubbo.application.name=boot-user-consumer
dubbo.registry.address=zookeeper://127.0.0.1:2181
dubbo.monitor.protocol=registry
3,ApplicationController
@EnableDubbo
@SpringBootApplication(scanBasePackages= {"com.taobao.*"},
exclude= {RedisAutoConfiguration.class})
@EnableHystrix
@EnableAsync //开启异步调用
public class ApplicationController {
//入口
public static void main(String[] args) {
SpringApplication.run(ApplicationController.class, args);
}
}
4,OrderServiceImpl
@Service
public class OrderServiceImpl implements OrderService {
//@Reference(url="127.0.0.1:20880") //没有注册中心时,与dubbo直连
@Reference
UserService userService;
@HystrixCommand(fallbackMethod="error")
public List<UserAddress> initOrder(String userId) {
List<UserAddress> addressList = userService.getUserAddressList(userId);
for (UserAddress userAddress : addressList) {
System.out.println(userAddress.getUserAddress());
}
return addressList;
}
public List<UserAddress> error(String userId) {
return null;
}
}
5,UserController
扫描二维码关注公众号,回复:
11627508 查看本文章
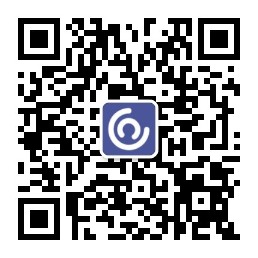
@Controller
public class UserController {
@Autowired
private OrderService orderService;
@ResponseBody
@RequestMapping("initorder")
public List<UserAddress> initOrder(String userId) {
List<UserAddress> order = orderService.initOrder(userId);
return order;
}
}