一.dubbo-boot-provider模块
maven依赖:
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.dubbo/dubbo-spring-boot-starter --> <dependency> <groupId>org.apache.dubbo</groupId> <artifactId>dubbo-spring-boot-starter</artifactId> <version>2.7.3</version> </dependency> </dependencies>
application.properties
dubbo.application.name=dubbo-boot-provider dubbo.registry.address=192.168.10.132:2181 dubbo.registry.protocol=zookeeper dubbo.protocol.name=dubbo dubbo.protocol.port=10000
service类:
注意这里的@Service是Dubbo的注解,不是Spring的Service注解
@Service public class UserServiceImpl implements UserService { @Override public List<User> getAll() { User user1 = new User(1, "张三", 12, "北京"); User user2 = new User(2, "李四", 13, "北京"); return Arrays.asList(user1, user2); } }
启动类:
@SpringBootApplication @EnableDubbo//开启基于注解的dubbo功能 public class DubboBootProviderApplication { public static void main(String[] args) { SpringApplication.run(DubboBootProviderApplication.class, args); } }
二.dubbo-boot-consumer模块
maven依赖
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.apache.dubbo</groupId> <artifactId>dubbo-spring-boot-starter</artifactId> <version>2.7.3</version> </dependency>
消费者:
调用UserService时,用@Reference表示远程调用,而不是用@Autowired
@Controller public class UserController { @Reference //远程引用userService UserService userService; @RequestMapping("/getAll") @ResponseBody public List<User> getAll(){ return userService.getAll(); } }
application.properties
dubbo.application.name=dubbo-boot-consumer dubbo.registry.address=zookeeper://192.168.10.132:2181 server.port=7070
启动类:
@SpringBootApplication @EnableDubbo public class DubboBootConsumerApplication { public static void main(String[] args) { SpringApplication.run(DubboBootConsumerApplication.class, args); } }
三.运行两个boot主应用,在浏览器输入localhost:7070/getAll
扫描二维码关注公众号,回复:
11273453 查看本文章
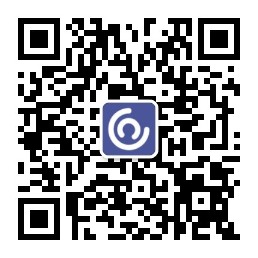