三日不见,变刮目相看。——鲁肃
底部导航栏
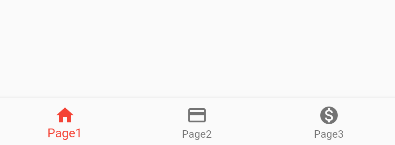
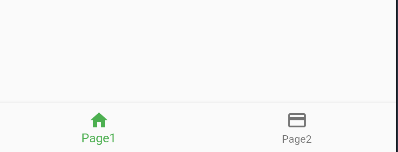
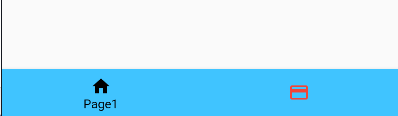
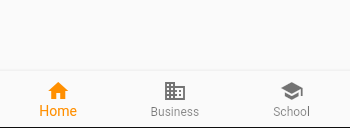
BottomNavigationBar 属性
items |
BottomNavigationBarItem 集合 |
onTap |
导航栏切换时触发的函数别名(回调函数) |
currentIndex |
导航条当前索引 |
elevation |
导航栏 z轴 坐标点 |
BottomNavigationBarType |
导航条类型 |
BottomNavigationBarType-fixed |
导航条固定宽度 |
BottomNavigationBarType-shifting |
导航条点击谈乳淡出效果 |
fixedColor |
导航条固定宽度颜色(文本和图标) |
backgroundColor |
导航条背景颜色 |
selectedItemColor |
选中导航条颜色(和fixedColor的值不能同时存在) |
unselectedItemColor |
未选中导航条颜色(和fixedColor的值能同时存在) |
showSelectedLabels |
显示选中项的文本 |
showUnselectedLabels |
显示未选中项的文本 |
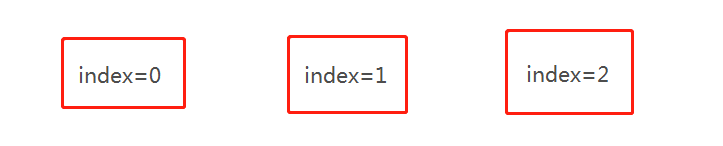
底部导航
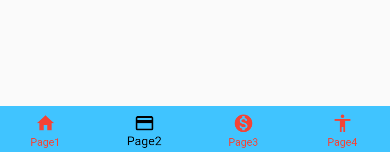
class BotNavBarWidget extends StatefulWidget {
BotNavBarWidget({Key key}) : super(key: key);
@override
_BotNavBarWidgetState createState() => _BotNavBarWidgetState();
}
class _BotNavBarWidgetState extends State<BotNavBarWidget> {
int _currentIndex = 0;
List<BottomNavigationBarItem> _botNavBarItems = <BottomNavigationBarItem>[
BottomNavigationBarItem(icon: Icon(Icons.home), title: Text('Page1')),
BottomNavigationBarItem(icon: Icon(Icons.payment), title: Text('Page2')),
BottomNavigationBarItem(icon: Icon(Icons.monetization_on), title: Text('Page3')),
BottomNavigationBarItem(icon: Icon(Icons.accessibility), title: Text('Page4'))
];
@override
Widget build(BuildContext context) {
return BottomNavigationBar(
type: BottomNavigationBarType.fixed,
onTap: onTabTapped,
currentIndex: _currentIndex,
backgroundColor: Colors.lightBlueAccent,
//selectedItemColor: Colors.black,
unselectedItemColor: Colors.red,
elevation: 0.0,
fixedColor: Colors.black,
showUnselectedLabels: true,
items: [
BottomNavigationBarItem(icon: Icon(Icons.home), title: Text('Page1')),
BottomNavigationBarItem(icon: Icon(Icons.payment), title: Text('Page2')),
BottomNavigationBarItem(icon: Icon(Icons.monetization_on), title: Text('Page3')),
BottomNavigationBarItem(icon: Icon(Icons.accessibility), title: Text('Page4'))
],
);
}
onTabTapped(int index) {
setState(() {});
_currentIndex=index;
}
}
class _MyHomePageState extends State<MyHomePage> {
@override
void initState() {
// TODO: implement initState
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
bottomNavigationBar: BotNavBarWidget(),
body: Container(),
);
}
}
底部导航切换page
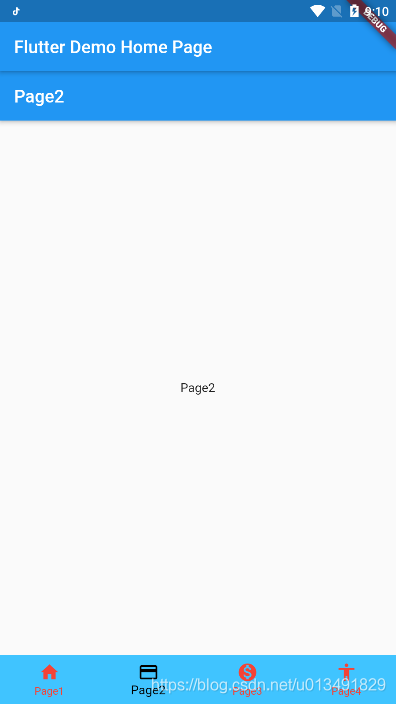
BotNavBarWidget 底部导航
class BotNavBarWidget extends StatefulWidget {
final ValueChanged stackValue;
BotNavBarWidget({Key key, this.stackValue}) : super(key: key);
@override
_BotNavBarWidgetState createState() => _BotNavBarWidgetState();
}
class _BotNavBarWidgetState extends State<BotNavBarWidget> {
List<int> tabInt = [0];
int _currentIndex = 0;
List<BottomNavigationBarItem> _botNavBarItems = <BottomNavigationBarItem>[
BottomNavigationBarItem(icon: Icon(Icons.home), title: Text('Page1')),
BottomNavigationBarItem(icon: Icon(Icons.payment), title: Text('Page2')),
BottomNavigationBarItem(
icon: Icon(Icons.monetization_on), title: Text('Page3')),
BottomNavigationBarItem(
icon: Icon(Icons.accessibility), title: Text('Page4'))
];
@override
Widget build(BuildContext context) {
return BottomNavigationBar(
type: BottomNavigationBarType.fixed,
onTap: onTabTapped,
currentIndex: _currentIndex,
backgroundColor: Colors.lightBlueAccent,
//selectedItemColor: Colors.black,
unselectedItemColor: Colors.red,
elevation: 0.0,
fixedColor: Colors.black,
showUnselectedLabels: true,
items: _botNavBarItems,
);
}
onTabTapped(int index) {
setState(() {});
_currentIndex = index;
if (!tabInt.contains(index)) {
tabInt.add(index);
}
if (widget.stackValue != null) {
widget.stackValue(_currentIndex,tabInt);
}
}
}
typedef ValueChanged = void Function(
int currentIndex,
List<int> tabInt,
);
StackWidget 容纳多个page的容器
class StackWidget extends StatefulWidget {
StackWidget({Key key, this.currentIndex = 0, this.tabInt}) : super(key: key);
final int currentIndex;
final List<int> tabInt;
@override
StackWidgetState createState() => StackWidgetState();
}
class StackWidgetState extends State<StackWidget> {
final List<StatefulWidget> _children = [
Page1(
title: "Page1",
),
Page2(
title: "Page2",
),
Page3(
title: "Page3",
),
Page4(
title: "Page4",
),
];
int _currentIndex = 0;
List<int> _tabInt;
@override
void initState() {
// TODO: implement initState
super.initState();
_tabInt = widget.tabInt == null ? [0] : widget.tabInt;
}
@override
Widget build(BuildContext context) {
return Container(
width: MediaQuery.of(context).size.width,
child: _stack(),
);
}
void changeStack(int currentIndex, List<int> tabInt) {
setState(() {});
_currentIndex = currentIndex;
_tabInt = tabInt;
}
Stack _stack() {
return Stack(
children: <Widget>[
_child(0),
_child(1),
_child(2),
_child(3),
],
);
}
//Page的显示和隐藏
_child(int _index) {
return Offstage(
offstage: !(_currentIndex == _index),
child: _tabInt.contains(_index) ? _children[_index] : Container(),
);
}
}
BotNavBarWidget + StackWidget -> Scaffold
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
GlobalKey<StackWidgetState> _stackGk = GlobalKey<StackWidgetState>();
@override
void initState() {
// TODO: implement initState
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
bottomNavigationBar: BotNavBarWidget(
stackValue: (int currentIndex, List<int> tabInt) {
_stackGk.currentState.changeStack(currentIndex, tabInt);
},
),
body: StackWidget(
key: _stackGk,
));
}
}
收集