Android Studio五子棋制作完整代码
因为学习了Android Studio开发工具,所以尝试着做了一个手机端的五子棋APP,可以播放背景音乐,可以通过下方的进度度条来判改变界面颜色,自作过程中还有一些不足的地方,希望能多多指教
完整代码如下,按照工程目录创建目录,将代码赋值即可用
APP界面图
工程目录结构
MaintActivity代码如下:
package com.example.gobang;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Context;
import android.graphics.Color;
import android.media.MediaPlayer;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.widget.Button;
import android.widget.SeekBar;
import static com.example.gobang.Cheek.cheekPaint;
import static com.example.gobang.ChessBoard.allChess;
import static com.example.gobang.ChessBoard.blackChessNum;
import static com.example.gobang.ChessBoard.chessBoardPaint;
import static com.example.gobang.ChessBoard.chessNum;
import static com.example.gobang.ChessBoard.chessx;
import static com.example.gobang.ChessBoard.chessy;
import static com.example.gobang.ChessBoard.isBlackWin;
import static com.example.gobang.ChessBoard.isEnClick;
import static com.example.gobang.ChessBoard.isWhiteWin;
import static com.example.gobang.ChessBoard.textpain;
import static com.example.gobang.ChessBoard.whiteChessNum;
import static com.example.gobang.FallingView.sonwrectF1Paint;
public class MainActivity extends Activity implements SeekBar.OnSeekBarChangeListener {
ChessBoard chessborad;
Cheek cheek;
private SeekBar sekbar;
private Button back;
private Button restart;
private Button start;
public static boolean blackWin = false;
public static boolean whiteWin = false;
private static Context mContext;
public static int setAlpha = 0;
private MediaPlayer mediaPlayer;
public static boolean isStart = false;
private FallingView snow;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//初始化画笔颜色
cheekPaint.setColor(Color.rgb(0, (int) (152 / 1.2), 199 ));
chessBoardPaint.setColor(Color.rgb( 0, (int) (152 / 1.2), 199 ));
textpain.setColor(Color.rgb( 0, (int) (152 / 1.2), 199 ));
sonwrectF1Paint.setColor(Color.rgb(0, (int) (152 / 1.2), 199 ));
chessborad = findViewById(R.id.chessboard);
cheek = findViewById(R.id.cheek);
sekbar = (SeekBar) findViewById(R.id.sekbar);
back = (Button) findViewById(R.id.back);
restart = (Button) findViewById(R.id.restart);
start = (Button) findViewById(R.id.start);
snow = findViewById(R.id.snow);
back.setTextColor(Color.rgb( 0, (int) (152 / 1.2), 199 ));
start.setTextColor(Color.rgb(0, (int) (152 / 1.2), 199 ));
restart.setTextColor(Color.rgb( 0, (int) (152 / 1.2), 199 ));
sekbar.setOnSeekBarChangeListener(this);
mContext = this;
}
public void Start(View view) {
isStart = !isStart;
if (isStart) {
snow.invalidate();
start.setText("暂停");
try {
mediaPlayer = MediaPlayer.create(this, R.raw.bg);
mediaPlayer.start();
mediaPlayer.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mediaPlayer) {
mediaPlayer.start();
mediaPlayer.setLooping(isStart);
}
});
} catch (Exception e) {
e.printStackTrace();
}
}
else {
start.setText("开始");
mediaPlayer.stop();
}
}
public void Back(View view) {
allChess[chessx][chessy] = null;
chessborad.invalidate();
}
//重开按钮点击事件
public void Restart(View view) {
for (int i = 0; i < 13; i++) {
for (int j = 0; j < 13; j++) {
allChess[i][j] = null;
}
}
blackChessNum = 0;
whiteChessNum = 0;
chessNum = 0;//进行棋子总数清零
isEnClick = true;
isBlackWin = isWhiteWin = false;
chessborad.invalidate();
}
@Override
public void onProgressChanged(SeekBar seekBar, int i, boolean b) {
setAlpha = i;
cheekPaint.setColor(Color.rgb(i * 2, (int) (152 - i / 1.2), 199 - i));
chessBoardPaint.setColor(Color.rgb(i * 2, (int) (152 - i / 1.2), 199 - i));
textpain.setColor(Color.rgb(i * 2, (int) (152 - i / 1.2), 199 - i));
sonwrectF1Paint.setColor(Color.rgb(i * 2, (int) (152 - i / 1.2), 199 - i));
back.setTextColor(Color.rgb(i * 2, (int) (152 - i / 1.2), 199 - i));
start.setTextColor(Color.rgb(i * 2, (int) (152 - i / 1.2), 199 - i));
restart.setTextColor(Color.rgb(i * 2, (int) (152 - i / 1.2), 199 - i));
chessborad.invalidate();
cheek.invalidate();
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
}
public static Handler myHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
if (msg.what == 1) {
whiteWin();
} else if (msg.what == 2) {
blackWin();
}else if(msg.what==0){
pleaseStart();
}
}
};
private static void blackWin() {
AlertDialog.Builder builder = new AlertDialog.Builder(mContext);
builder.setTitle("GoBang");
builder.setIcon(R.drawable.icon);
builder.setMessage("黑方获胜,请点击重开按钮重新开始");
builder.show();
}
private static void whiteWin() {
AlertDialog.Builder builder = new AlertDialog.Builder(mContext);
builder.setTitle("GoBang");
builder.setIcon(R.drawable.icon);
builder.setMessage("白方获胜,请点击重开按钮重新开始");
builder.show();
}
private static void pleaseStart(){
AlertDialog.Builder builder = new AlertDialog.Builder(mContext);
builder.setTitle("GoBang");
builder.setIcon(R.drawable.icon);
builder.setMessage("请点击开始按钮开始下棋");
builder.show();
}
}
FalllngView代码如下:
package com.example.gobang;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.util.AttributeSet;
import android.view.View;
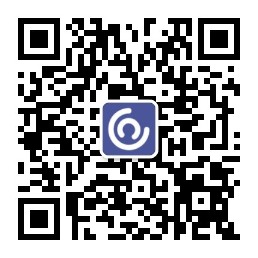
import androidx.annotation.Nullable;
import static com.example.gobang.MainActivity.isStart;
public class FallingView extends View {
private Context mContext;
private AttributeSet mAttrs;
private static final int defaultWidth = 600;//默认宽度
private static final int defaultHeight = 1000;//默认高度
private static final int intervalTime = 20;//重绘间隔时间
public static Paint sonwrectF1Paint;
public static Paint sonwrectF2Paint;
public static Paint sonwrectF3Paint;
public static Paint sonwrectF4Paint;
public static Paint sonwrectF5Paint;
private int snowY1;
private int sonwX1=31;
private int snowY2=1125;
private int sonwX2=101;
private int snowY3=1350;
private int sonwX3=101;
private int snowY4=1350;
private int sonwX4=600;
private int snowY5=1520;
private int sonwX5=101;
private static int roadFlag=1;
private static int roadFlag1=1;
private int roadFlag2=1;
private int roadFlag3=1;
private int roadFlag4=1;
public FallingView(Context context) {
super(context);
mContext = context;
init();
}
public FallingView(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
mContext = context;
mAttrs = attrs;
init();
}
private void init(){
sonwrectF1Paint = new Paint();
sonwrectF1Paint.setColor(Color.WHITE);
sonwrectF1Paint.setStyle(Paint.Style.FILL);
sonwrectF1Paint.setAntiAlias(true);
snowY1 = 0;
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
int height = measureSize(defaultHeight, heightMeasureSpec);
int width = measureSize(defaultWidth, widthMeasureSpec);
setMeasuredDimension(width, height);
}
private int measureSize(int defaultSize,int measureSpec) {
int result = defaultSize;
int specMode = View.MeasureSpec.getMode(measureSpec);
int specSize = View.MeasureSpec.getSize(measureSpec);
if (specMode == View.MeasureSpec.EXACTLY) {
result = specSize;
} else if (specMode == View.MeasureSpec.AT_MOST) {
result = Math.min(result, specSize);
}
return result;
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
if(isStart) {
canvas.drawCircle(sonwX1, snowY1, 15, sonwrectF1Paint);
canvas.drawCircle(sonwX2, snowY2, 11, sonwrectF1Paint);
canvas.drawCircle(sonwX3, snowY3, 8, sonwrectF1Paint);
canvas.drawCircle(sonwX4, snowY4, 8, sonwrectF1Paint);
canvas.drawCircle(sonwX5, snowY5, 8, sonwrectF1Paint);
getHandler().postDelayed(runnable, intervalTime);//间隔一段时间再进行重绘
}
}
// 重绘线程
private Runnable runnable = new Runnable() {
@Override
public void run() {
if(roadFlag==1){
sonwrectF1Road1();
}else if(roadFlag==2){
sonwrectF1Road2();
}
if(roadFlag1==1){
sonwrectF2Road1();
}else if(roadFlag1==2){
sonwrectF2Road2();
}
if(roadFlag2==1){
sonwrectF3Road1();
}else if(roadFlag2==2){
sonwrectF3Road2();
}
if(roadFlag3==1){
sonwrectF4Road1();
}else if(roadFlag3==2){
sonwrectF4Road2();
}
if(roadFlag4==1){
sonwrectF5Road1();
}else if(roadFlag4==2){
sonwrectF5Road2();
}
invalidate();
}
};
//绘制最外边边框路线
private void sonwrectF1Road1(){
snowY1+=10;
if(snowY1>=1635){
snowY1=1635;
}
if(snowY1==1635){
sonwX1+=10;
if(sonwX1>=1052){
sonwX1=1052;
}
}
if(sonwX1==1052&&snowY1==1635){
roadFlag=2;
}
}
//绘制最外边边框路线
private void sonwrectF1Road2(){
snowY1-=10;
if(snowY1<=72){
snowY1=72;
}
if(snowY1==72){
sonwX1-=10;
if(sonwX1<=31){
sonwX1=31;
}
}
if(snowY1==72&&sonwX1==31){
roadFlag=1;
}
}
//绘制按钮边框路线
private void sonwrectF2Road1(){
snowY2+=12;
if(snowY2>=1336){
snowY2=1336;
}
if(snowY2==1336){
sonwX2+=12;
if(sonwX2>=980){
sonwX2=980;
}
}
if(sonwX2==980&&snowY2==1336){
roadFlag1=2;
}
}
//绘制按钮边框路线
private void sonwrectF2Road2(){
snowY2-=12;
if(snowY2<=1125){
snowY2=1125;
}
if(snowY2==1125){
sonwX2-=12;
if(sonwX2<=101){
sonwX2=101;
}
}
if(snowY2==1125&&sonwX2==101){
roadFlag1=1;
}
}
//绘制显示边框一路线
private void sonwrectF3Road1(){
snowY3+=9;
if(snowY3>=1506){
snowY3=1506;
}
if(snowY3==1506){
sonwX3+=9;
if(sonwX3>=490){
sonwX3=490;
}
}
if(sonwX3==490&&snowY3==1506){
roadFlag2=2;
}
}
//绘制显示边框一路线
private void sonwrectF3Road2(){
snowY3-=9;
if(snowY3<=1350){
snowY3=1350;
}
if(snowY3==1350){
sonwX3-=9;
if(sonwX3<=101){
sonwX3=101;
}
}
if(snowY3==1350&&sonwX3==101){
roadFlag2=1;
}
}
//绘制变框二显示路线
private void sonwrectF4Road1(){
snowY4+=9;
if(snowY4>=1506){
snowY4=1506;
}
if(snowY4==1506){
sonwX4+=9;
if(sonwX4>=980){
sonwX4=980;
}
}
if(sonwX4==980&&snowY4==1506){
roadFlag3=2;
}
}
//绘制显示边框二显示路线
private void sonwrectF4Road2(){
snowY4-=9;
if(snowY4<=1350){
snowY4=1350;
}
if(snowY4==1350){
sonwX4-=9;
if(sonwX4<=600){
sonwX4=600;
}
}
if(snowY4==1350&&sonwX4==600){
roadFlag3=1;
}
}
//绘制进度条显示路线
private void sonwrectF5Road1(){
sonwX5+=5;
if(sonwX5>=980){
sonwX5=980;
}
if(sonwX5==980){
snowY5+=5;
if(snowY5>=1600){
snowY5=1600;
}
}
if(snowY5==1600&&sonwX5==980){
roadFlag4=2;
}
}
private void sonwrectF5Road2(){
sonwX5-=5;
if(sonwX5<=101){
sonwX5=101;
}
if(sonwX5==101){
snowY5-=5;
if(snowY5<=1520){
snowY5=1520;
}
}
if(snowY5==1520&&sonwX5==101){
roadFlag4=1;
}
}
}
ChessBoard代码如下:
package com.example.gobang;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.RectF;
import android.graphics.Typeface;
import android.util.AttributeSet;
import android.view.MotionEvent;
import android.view.View;
import androidx.annotation.Nullable;
import static com.example.gobang.MainActivity.isStart;
public class ChessBoard extends View {
//定义变量来判断当前要下黑棋还是白棋
public static boolean isBlackWin = false;
public static boolean isWhiteWin = false;
public static int chessNum;//定义变脸来了保存棋子的总数,应为棋盘的大小时13*13,最大能存放169个棋子
public static String[][] allChess = new String[13][13];//定义数组来存储棋子的位置
public static boolean isEnClick = true;
public static int chessx, chessy;//定义变量来保存数组中棋子的位置
public static Paint chessBoardPaint = new Paint();
Paint chessPaint = new Paint();//定义用来绘制棋子的画笔
Paint chesscheek = new Paint();
public static Paint textpain = new Paint();//定义文字的画笔
private int x, y;//定义变量用来获取当前所点击屏幕的位置
public static int chessFiveCount = 1;//定一变量来获取棋子的连城数量
public static int blackChessNum = 0;
public static int whiteChessNum = 0;
public ChessBoard(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
chesscheek.setColor(Color.RED);
chesscheek.setStyle(Paint.Style.STROKE);
chessBoardPaint.setStrokeWidth(8);
textpain.setTextSize(100);//设置画笔画的字体尺寸
textpain.setTypeface(Typeface.DEFAULT_BOLD);//设置画笔字体
//画棋盘的行
for (int i = 104; i < 1144; i += 80) {
canvas.drawLine(58, i, 1022, i, chessBoardPaint);
}
//画棋盘的列
for (int i = 61; i < 1101; i += 80) {
canvas.drawLine(i, 102, i, 1065, chessBoardPaint);
}
canvas.drawText("黑棋:" + blackChessNum, 130, 1460, textpain);
canvas.drawText("白棋:" + whiteChessNum, 630, 1460, textpain);
//进行棋子的绘制
for (int i = 0; i < allChess.length; i++) {
for (int j = 0; j < allChess.length; j++) {
if (allChess[i][j] == "black") {
chessPaint.setColor(Color.BLACK);
canvas.drawCircle(i * 80 + 58, j * 80 + 102, 30, chessPaint);
} else if (allChess[i][j] == "white") {
chessPaint.setColor(Color.WHITE);
canvas.drawCircle(i * 80 + 58, j * 80 + 102, 30, chessPaint);
}
}
}
//当棋子数不为零时在当前棋子上添加一个边框
if (chessNum > 0) {
RectF cheek = new RectF(chessx * 80 + 28, chessy * 80 + 72, chessx * 80 + 88, chessy * 80 + 132);
canvas.drawRoundRect(cheek, 10, 10, chesscheek);
}
}
@Override
public boolean onTouchEvent(MotionEvent event) {
if (isStart) {
if (isEnClick) {
if (event.getAction() == MotionEvent.ACTION_DOWN) {
x = (int) event.getX();
y = (int) event.getY();
//判断点击的位置是否在棋盘之内
if ((x >= 40 && x <= 1045) && (y >= 95 && y <= 1090)) {
chessx = (x - 58) / 80;
chessy = (y - 102) / 80;
if (chessNum % 2 == 0) {
//检测当前位置是否右棋子
if (allChess[chessx][chessy] == null) {
allChess[chessx][chessy] = "black";
chessNum++;
blackChessNum++;
}
} else if (chessNum % 2 == 1) {
//检测当前位置是否有棋子
if (allChess[chessx][chessy] == null) {
allChess[chessx][chessy] = "white";
chessNum++;
whiteChessNum++;
}
}
checkFive();
invalidate();
}
}
}
}
else {
MainActivity.myHandler.obtainMessage(0).sendToTarget();
}
if (chessNum > 169) {
isEnClick = false;
}
if (isEnClick == false && event.getAction() == MotionEvent.ACTION_DOWN) {
if (isBlackWin) {
MainActivity.myHandler.obtainMessage(2).sendToTarget();
} else if (isWhiteWin) {
MainActivity.myHandler.obtainMessage(1).sendToTarget();
}
}
return true;
}
//定义方法用来检查是否有棋子连城五个
public void checkFive() {
String color = allChess[chessx][chessy];
chessFiveCount = 1;
//判断水平方向上是否有五个棋子连在一起
if (chessx != 1 && chessx != 11) {//如果当chessx等于11 and 1时需要进行其他判断
//首先向右边方向进行检测
for (int i = 1; i < 5; i++) {
if (x + i > 13) {
break;
}
if (color == allChess[chessx + i][chessy]) {
chessFiveCount++;
} else {
break;
}
}
if (chessFiveCount >= 5) {
isEnClick = false;
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
} else {
for (int i = 1; i < 5; i++) {
if (chessx - i <= 0) {
break;
}
if (color == allChess[chessx - i][chessy]) {
chessFiveCount++;
} else {
break;
}
if (chessFiveCount >= 5) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
}
chessFiveCount = 1;
} else if (chessx == 1) {//对chessx的位置进行重新判断
for (int i = 1; i < 5; i++) {
if (chessx + i >= 13) {
break;
}
if (color == allChess[chessx + i][chessy]) {
chessFiveCount++;
} else {
break;
}
if (chessFiveCount > 3) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
chessFiveCount = 1;
} else if (chessx == 11) {
for (int i = 1; i < 5; i++) {
if (chessx - i <= 0) {
break;
}
if (color == allChess[chessx - i][chessy]) {
chessFiveCount++;
} else {
break;
}
if (chessFiveCount > 3) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
chessFiveCount = 1;
}
chessFiveCount = 1;
//进行垂直方向上的判断
if (chessy != 1 && chessy != 11) {
for (int i = 1; i < 5; i++) {
if (chessy + i >= 13) {
break;
}
if (color == allChess[chessx][chessy + i]) {
chessFiveCount++;
} else {
break;
}
}
if (chessFiveCount >= 5) {
isEnClick = false;
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
} else {
for (int i = 1; i < 5; i++) {
if (chessy - i <= 0) {
break;
}
if (color == allChess[chessx][chessy - i]) {
chessFiveCount++;
} else {
break;
}
if (chessFiveCount >= 5) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
chessFiveCount = 1;
}
} else if (chessy == 1) {
for (int i = 1; i < 5; i++) {
if (chessy + i >= 13) {
break;
}
if (color == allChess[chessx][chessy + i]) {
chessFiveCount++;
} else {
break;
}
if (chessFiveCount > 3 && color == allChess[0][1]) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
chessFiveCount = 1;
} else if (chessy == 11) {
for (int i = 1; i < 5; i++) {
if (chessy - i <= 0) {
break;
}
if (color == allChess[chessx][chessy - i]) {
chessFiveCount++;
} else {
break;
}
if (chessFiveCount > 3 && color == allChess[1][12]) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
chessFiveCount = 1;
}
//对对角方向进行判断
if ((chessx != 1 && chessx != 11) && (chessy != 1 && chessy != 11)) {
for (int i = 1; i < 5; i++) {
if (chessx + i >= 13 || chessy + i >= 13) {
break;
}
if (color == allChess[chessx + i][chessy + i]) {
chessFiveCount++;
} else {
break;
}
}
if (chessFiveCount >= 5) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
} else {
for (int i = 1; i < 5; i++) {
if (chessx - i <= 0 || chessy - i <= 0) {
break;
}
if (color == allChess[chessx - i][chessy - i]) {
chessFiveCount++;
} else {
break;
}
if (chessFiveCount >= 5) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
chessFiveCount = 1;
}
chessFiveCount = 1;
if (isEnClick) {
for (int i = 1; i < 5; i++) {
if (chessx + i >= 13 || chessy - i <= 0) {
break;
}
if (color == allChess[chessx + i][chessy - i]) {
chessFiveCount++;
} else {
break;
}
}
if (chessFiveCount >= 5) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
} else {
for (int i = 1; i < 5; i++) {
if (chessx - i <= 0 || chessy + i >= 13) {
break;
}
if (color == allChess[chessx - i][chessy + i]) {
chessFiveCount++;
} else {
break;
}
if (chessFiveCount >= 5) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
}
}
} else if (chessx == 1 && chessy == 1) {
for (int i = 1; i < 5; i++) {
if (chessx + i >= 13 || chessy >= 13) {
break;
}
if (color == allChess[chessx + i][chessy + i]) {
chessFiveCount++;
} else {
break;
}
if (color == allChess[0][0] && chessFiveCount > 3) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
} else if (chessx == 11 && chessy == 11) {
for (int i = 1; i < 5; i++) {
if (chessx - i <= 0 && chessy - i <= 0) {
break;
}
if (color == allChess[chessx - i][chessy - i]) {
chessFiveCount++;
} else {
break;
}
if (color == allChess[12][12] && chessFiveCount > 3) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
chessFiveCount = 1;
} else if (chessx == 1 && chessy == 11) {
for (int i = 1; i < 5; i++) {
if (chessx + i >= 13 || chessy - i <= 0) {
break;
}
if (color == allChess[chessx + i][chessy - i]) {
chessFiveCount++;
} else {
break;
}
if (color == allChess[0][12] && chessFiveCount > 3) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
} else if (chessx == 11 && chessy == 1) {
for (int i = 1; i < 5; i++) {
if (chessx - i <= 0 || chessy + i >= 13) {
break;
}
if (color == allChess[chessx - i][chessy + i]) {
chessFiveCount++;
} else {
break;
}
if (color == allChess[12][0] && chessFiveCount > 3) {
isEnClick = false;//失能下棋点击
//判断为黑棋赢
if (color == "black") {
isBlackWin = true;
isWhiteWin = false;
}
//判断为黑棋赢
else if (color == "white") {
isBlackWin = false;
isWhiteWin = true;
}
chessFiveCount = 1;
break;
}
}
chessFiveCount = 1;
}
if (isWhiteWin) {
MainActivity.myHandler.obtainMessage(1).sendToTarget();
} else if (isBlackWin) {
MainActivity.myHandler.obtainMessage(2).sendToTarget();
}
}
}
Cheek代码如下:
package com.example.gobang;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.Path;
import android.graphics.RectF;
import android.util.AttributeSet;
import android.view.View;
import androidx.annotation.Nullable;
import static com.example.gobang.MainActivity.setAlpha;
public class Cheek extends View {
public static Paint cheekPaint = new Paint();
Paint heartPaint = new Paint();
public Cheek(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
}
@Override
public void draw(Canvas canvas) {
super.draw(canvas);
cheekPaint.setStrokeWidth(3);
cheekPaint.setStyle(Paint.Style.STROKE);
heartPaint.setAntiAlias(true);//抗锯齿
heartPaint.setStrokeWidth(8);//画笔宽度
heartPaint.setColor(Color.RED);//画笔颜色
heartPaint.setStyle(Paint.Style.STROKE);//画笔样式
heartPaint.setAlpha(100-setAlpha);
int width = getWidth();
int height = getHeight()-40;
// 绘制心形
Path path = new Path();
path.moveTo(width/2,height/4);
path.cubicTo((width*6)/7,height/9,(width*12)/13,(height*2)/5,width/2,(height*7)/12);
canvas.drawPath(path,heartPaint);
Path path2 = new Path();
path2.moveTo(width/2,height/4);
path2.cubicTo(width / 7, height / 9, width / 13, (height * 2) / 5, width / 2, (height * 7) / 12);
canvas.drawPath(path2,heartPaint);
//画棋盘边框
RectF rectF0 = new RectF(51,92,1032,1075);
canvas.drawRoundRect(rectF0,25,25,cheekPaint);
//画最外边的边框
RectF rectF1 = new RectF(31,72,1052,1635);
canvas.drawRoundRect(rectF1,25,25,cheekPaint);
//画按钮边框
RectF rectF2 = new RectF(101,1125,980,1336);
canvas.drawRoundRect(rectF2,25,25,cheekPaint);
//画显示边框
RectF rectF3 = new RectF(101,1350,490,1506);
RectF rectF4 = new RectF(600,1350,980,1506);
canvas.drawRoundRect(rectF3,25,25,cheekPaint);
canvas.drawRoundRect(rectF4,25,25,cheekPaint);
//画进度条边框
RectF rectF5 = new RectF(101,1520,980,1600);
canvas.drawRoundRect(rectF5,25,25,cheekPaint);
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<com.example.gobang.FallingView
android:id="@+id/snow"
android:layout_width=“match_parent”
android:layout_height=“match_parent”></com.example.gobang.FallingView>
<Button
android:id="@+id/back"
android:layout_width="80dp"
android:layout_height="60dp"
android:layout_marginLeft="20dp"
android:layout_marginTop="380dp"
android:layout_toRightOf="@+id/start"
android:background="@drawable/buttonselector"
android:onClick="Back"
android:text="悔棋"
android:textSize="30dp"></Button>
<Button
android:id="@+id/restart"
android:layout_width="80dp"
android:layout_height="60dp"
android:layout_marginLeft="20dp"
android:layout_marginTop="380dp"
android:layout_toRightOf="@+id/back"
android:background="@drawable/buttonselector"
android:onClick="Restart"
android:text="重开"
android:textSize="30dp"></Button>
<ImageView
android:id="@+id/iv"
android:layout_width="320dp"
android:layout_height="320dp"
android:layout_marginLeft="20dp"
android:layout_marginTop="34dp"></ImageView>
<com.example.gobang.Cheek
android:id="@+id/cheek"
android:layout_width="wrap_content"
android:layout_height="wrap_content"></com.example.gobang.Cheek>
<com.example.gobang.ChessBoard
android:id="@+id/chessboard"
android:layout_width="wrap_content"
android:layout_height="wrap_content"></com.example.gobang.ChessBoard>
<SeekBar
android:id="@+id/sekbar"
android:layout_width="310dp"
android:layout_height="40dp"
android:layout_below="@+id/start"
android:layout_marginLeft="25dp"
android:layout_marginTop="60dp"
android:padding="20dp"
android:progressDrawable="@drawable/seekbar"
android:thumb="@drawable/select_seekbar"></SeekBar>
自定义按钮
buttonselector.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/buttonshapetwo" android:state_pressed="true"></item>
<item android:drawable="@drawable/buttonshapetwo" android:state_focused="true"></item>
<item android:drawable="@drawable/buttonshapeone" android:state_enabled="true"></item>
<item android:drawable="@drawable/buttonshapeone"></item>
</selector>
buttonshapeone.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/buttonshapetwo" android:state_pressed="true"></item>
<item android:drawable="@drawable/buttonshapetwo" android:state_focused="true"></item>
<item android:drawable="@drawable/buttonshapeone" android:state_enabled="true"></item>
<item android:drawable="@drawable/buttonshapeone"></item>
</selector>
buttonshapetwo.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle">
<solid android:color="#007077" ></solid>
<stroke android:width="5dp" android:color="#00574B"></stroke>
<corners android:radius="10dp"></corners>
</shape>
自定义进度条
select_seekbar.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<!-- 按下状态-->
<item android:state_pressed="true" android:drawable="@drawable/seekbarshape1"/>
<!-- 有焦点状态 -->
<item android:state_focused="true" android:drawable="@drawable/seekbarshape"/>
<!-- 普通状态 -->
<item android:drawable="@drawable/seekbarshape" />
</selector>
seekbarshape.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="oval">
android:useLevel="false">
<solid android:color="@color/colorPrimary"></solid> <!-- 颜色-->
<size
android:width="12dp"
android:height="12dp"></size>
</shape>
seekbarshape1.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="oval">
android:useLevel="false">
<solid android:color="@color/colorAccent"></solid> <!-- 颜色-->
<size
android:width="14dp"
android:height="14dp"></size>
</shape>
seekbar.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/background" android:height="1dp">
<shape>
<corners android:radius="10dp"
></corners>
<solid android:color="#C9C7C7"></solid>
</shape>
</item>
<item android:id="@+id/secondaryProgress" android:height="1dp">
<clip>
<shape>
<corners android:radius="5dp"></corners>
<solid android:color="#C9C7C7"></solid>
</shape>
</clip>
</item>
<item android:id="@android:id/progress" android:height="1dp">
<clip>
<shape>
<corners android:radius="5dp"/>
<solid android:color="@color/colorPrimary"/> <!-- 颜色-->
</shape>
</clip>
</item>
</layer-list>