Okhttp获取数据代码:
public void Okhttp_GET(String urlStr){
Request request = new Request.Builder().url(urlStr).get().build();
OkHttpClient client = new OkHttpClient();
try {
Response response = client.newCall(request).execute();
if(response.isSuccessful()){
String json = response.body().string();
Log.e("###",json);
}else{
Log.e("###","对不起请求失败!!!");
}
} catch (Exception e) {
e.printStackTrace();
}
}
public void Okhttp_POST(String urlStr){
FormBody body = new FormBody.Builder()
.add("phone","13722655422")
.add("passwd","124")
.build();
Request request = new Request.Builder().url(urlStr).post(body).build();
OkHttpClient client = new OkHttpClient();
try {
Response response = client.newCall(request).execute();
if(response.isSuccessful()){
Log.e("###",response.body().string());
}else{
Log.e("###","请求失败!!!");
}
} catch (IOException e) {
e.printStackTrace();
}
}
public void AsyncGET(String urlStr){
Request request = new Request.Builder().url(urlStr).get().build();
OkHttpClient client = new OkHttpClient();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
Log.e("###","请求失败");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
Log.e("###",response.body().string());
}
});
}
public void AsyncPOST(String urlStr){
FormBody body = new FormBody.Builder()
.add("phone","983487889")
.add("passwd","123")
.build();
Request request = new Request.Builder().url(urlStr).post(body).build();
OkHttpClient client = new OkHttpClient();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
Log.e("###","请求失败!!!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
Log.e("###",response.body().string());
}
});
}
Volley获取数据代码:
public void VolleyGet(String strUrl, Context context){
RequestQueue queue = Volley.newRequestQueue(context);
StringRequest request = new StringRequest(StringRequest.Method.GET, strUrl, listener, errorListener);
queue.add(request);
queue.start();
}
com.android.volley.Response.Listener<String> listener = new com.android.volley.Response.Listener<String>() {
@Override
public void onResponse(String response) {
Log.e("###成功",response);
}
};
com.android.volley.Response.ErrorListener errorListener = new com.android.volley.Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
Log.e("###","请求失败!!!");
}
};
public void VolleyPost(String urlStr,Context context){
RequestQueue queue = Volley.newRequestQueue(context);
StringRequest request = new StringRequest(StringRequest.Method.POST,urlStr,listener,errorListener){
@Override
protected Map<String, String> getParams() throws AuthFailureError {
HashMap<String,String> map = new HashMap<>();
map.put("phone","1286041884");
map.put("passwd","123");
return map;
}
};
queue.add(request);
queue.start();
}
public void Volley_Image(String urlStr, final ImageView imageView, Context context){
ImageRequest imageRequest = new ImageRequest(urlStr, new com.android.volley.Response.Listener<Bitmap>() {
@Override
public void onResponse(Bitmap response) {
imageView.setImageBitmap(response);
}
}, 100, 100, Bitmap.Config.ARGB_8888, errorListener);
RequestQueue queue = Volley.newRequestQueue(context);
queue.add(imageRequest);
queue.start();
}
布局代码:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".work_6_25"
android:orientation="vertical"
>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/btn1"
android:text="HTTP_POST"
/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/btn2"
android:text="Okhttp_GET"
/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/btn3"
android:text="Okhttp_POST"
/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/btn4"
android:text="异步加载_GET"
/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/btn5"
android:text="异步加载_POST"
/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/btn6"
android:text="Volley_GET"
/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/btn7"
android:text="Volley_POST"
/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/btn8"
android:text="Volley_image"
/>
<ImageView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/img"
/>
</LinearLayout>
主类代码:
package com.example.work_6_25;
import android.content.Context;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
public class work_6_25 extends AppCompatActivity implements View.OnClickListener {
private MyUtils utils = new MyUtils();
private ImageView imageView;
private final String URL_1 = "https://www.apiopen.top/createUser?key=00d91e8e0cca2b76f515926a36db68f5&";
private final String URL_IMAGE = "http://upload.jianshu.io/users/upload_avatars/6416344/5e103cde-c77d-49f9-8a47-2ed4418c564a.jpg";
private final String URL_2 = "http://www.qubaobei.com/ios/cf/dish_list.php?stage_id=1&limit=20&page=1";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_work_6_25);
initView();
}
private void initView() {
findViewById(R.id.btn1).setOnClickListener(this);
findViewById(R.id.btn2).setOnClickListener(this);
findViewById(R.id.btn3).setOnClickListener(this);
findViewById(R.id.btn4).setOnClickListener(this);
findViewById(R.id.btn5).setOnClickListener(this);
findViewById(R.id.btn6).setOnClickListener(this);
findViewById(R.id.btn7).setOnClickListener(this);
findViewById(R.id.btn8).setOnClickListener(this);
imageView = findViewById(R.id.img);
}
@Override
public void onClick(View v) {
switch(v.getId()){
case R.id.btn1:
new Thread(){
@Override
public void run() {
utils.http_Post(URL_1);
}
}.start();
break;
case R.id.btn2:
new Thread(){
@Override
public void run() {
utils.Okhttp_GET(URL_2);
}
}.start();
break;
case R.id.btn3:
new Thread(){
@Override
public void run() {
utils.Okhttp_POST(URL_1);
}
}.start();
break;
case R.id.btn4:
utils.AsyncGET(URL_2);
break;
case R.id.btn5:
utils.AsyncPOST(URL_1);
break;
case R.id.btn6:
utils.VolleyGet(URL_2,this);
break;
case R.id.btn7:
utils.VolleyPost(URL_1,this);
break;
case R.id.btn8:
utils.Volley_Image(URL_IMAGE,imageView,this);
break;
}
}
}
界面样式:
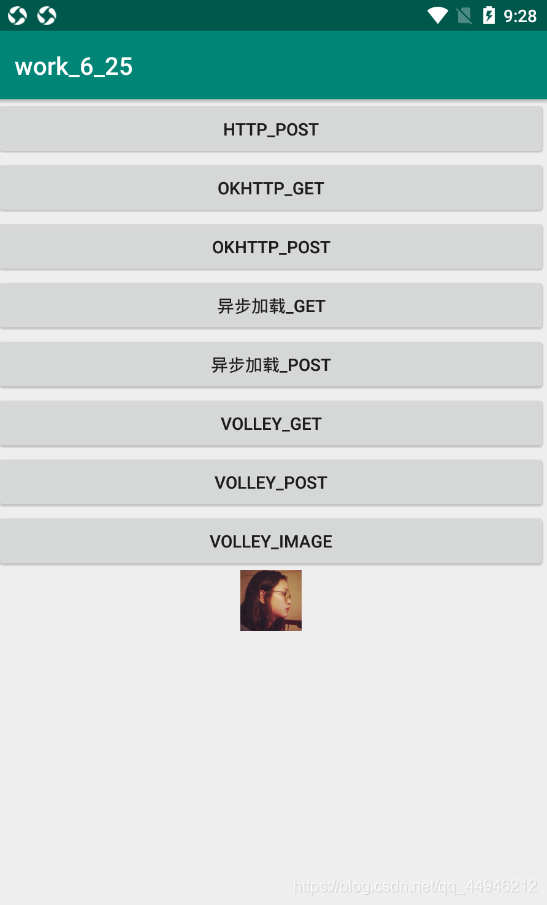