TreeSet底层实际是用TreeMap实现的,内部维持了一个简化版的TreeMap,通过key来存储Set的元素。
从TreeSet源码构造函数很容易就发现TreeSet底层构造是new的TreeMap
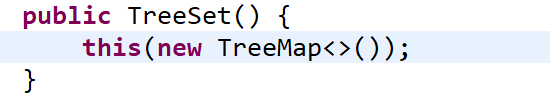

TreeSet内部需要对存储的元素进行排序,因此,我们对应的类需要实现Comparable接口。这样,才能根据compareTo()方法比较对象之间的大小,才能进行内部排序。
测试TreeSet
package cn.yzy.collection;
import java.util.Set;
import java.util.TreeSet;
public class testTreeSet {
public static void main(String[] args) {
Set<Integer> set = new TreeSet<>();
set.add(900);
set.add(300);
set.add(600);
for(Integer i:set)
System.out.println(i);
}
}
以上是存储基本数据类型的使用,如果是存储自定义类型的需要实现Comparable接口和compareTo接口
package cn.yzy.collection;
import java.util.Set;
import java.util.TreeSet;
//import cn.yzy.collection.Employee;
public class testTreeSet {
public static void main(String[] args) {
Set<Emp2> set1 = new TreeSet<Emp2>();
set1.add(new Emp2(256488, "张三", 29541));
set1.add(new Emp2(265411, "李四", 9541));
set1.add(new Emp2(254944, "王五", 11650));
set1.add(new Emp2(256459, "赵六", 35694));
for(Emp2 i:set1)
System.out.println(i);
}
}
class Emp2 implements Comparable<Emp2>{
int id;
String name;
double salary;
public Emp2(int id, String name, double salary) {
super();
this.id = id;
this.name = name;
this.salary = salary;
}
@Override
public String toString() {
return "id:"+id+" name:"+name+" salary"+salary;
}
@Override
public int compareTo(Emp2 o) {
if(this.salary > o.salary)
return 1;
else if(this.salary < o.salary)
return -1;
else {
if(this.id > o.id)
return 1;
else if(this.id < o.id)
return -1;
else
return 0;
}
}
}