HDU1006 Tick and Tick
Problem Description
The three hands of the clock are rotating every second and meeting each other many times everyday. Finally, they get bored of this and each of them would like to stay away from the other two. A hand is happy if it is at least D degrees from any of the rest. You are to calculate how much time in a day that all the hands are happy.
Input
The input contains many test cases. Each of them has a single line with a real number D between 0 and 120, inclusively. The input is terminated with a D of -1.
Output
For each D, print in a single line the percentage of time in a day that all of the hands are happy, accurate up to 3 decimal places.
Sample Input
0
120
90
-1
Sample Output
100.000
0.000
6.251
题目大意:
找到一天时针分针秒针三者所产生的三个夹角均大于所给角D的百分比。
题目中秒针可以看做是划过,时针分针每时每刻都在跟着运动,所以是不能将最小单位设成秒或度来计算。(一开始将秒无限细分来比较,超时比较严重)
解题思路:
首先一天24小时分成两个12小时,然后将其分成12*60分钟,计算每分钟里的happytime。而计算happytime需要解方程,这样无精度问题。
时针 | 分针 | 秒针 | |
---|---|---|---|
每秒角速度 | 1/120 | 1/10 | 6 |
一度所用时间 | 120 | 10 | 1/6 |
遍历12小时*60分钟,设当前时间走了s秒(s取值范围为[0,60])。例如6时40分s秒,此时遍历小时数h和分钟数m都是已知数。
计算当前每个指针的角度,这三个公式可以自己手推一遍。
hh=30*h+m/2+s/120;
mm=6*m+s/10;
ss=6*s;
例如:hh时针走过角度=时针*360度/12小时+分针*360度/60分钟/12小时+秒针*360度/60秒/60分钟/12小时。
然后计算三个不等式和区间[0,60]交集。
D<=|hw-mw|<=360-D
D<=|hw-sw|<=360-D
D<=|mw-sw|<=360-D
上面给出公式hh、mm、ss=xxxx代入绝对值不等式只含一个未知数秒s,转化为
D<=|ax+b|<=360-D
的问题,x为秒s。
求出三个区间,与[0,60]求交集,得出h和m,h和s,m和s的三个两两指针的happy区间,将这三个区间求交集就为这一分钟的happy区间。
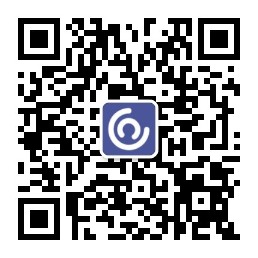
思路要清晰,解题前最好在纸上推好公式。
代码如下:
#include <bits/stdc++.h>
using namespace std;
struct qujian{
double l,r;
};
double D;
qujian jiao(qujian a,qujian b){
qujian p;
p.l=max(a.l,b.l);
p.r=min(a.r,b.r);
if (p.l>=p.r) p.l=p.r=0;
return p;
}
qujian solve(double a,double b){
qujian p;
///D<=|ax+b|<=360-D;
if (a>0){
p.l=(D-b)/a;
p.r=(360-D-b)/a;
}
else {
p.l=(360-D-b)/a;
p.r=(D-b)/a;
}
if (p.l<0) p.l=0;
if (p.r>60) p.r=60;
if (p.l>=p.r) p.l=p.r=0;
return p;
}
double happytime(double h,double m){
double a,b;
qujian s[3][2];
qujian ss;
///D<=|hw-mw|<=360-D;
///D<=|hw-sw|<=360-D;
///D<=|mw-sw|<=360-D;
///[0,60]
///hh=30*h+m/2+s/120;
///mm=6*m+s/10;
///ss=6*s;
/// |xx-xx|=a*x+b
///D<=|hw-mw|<=360-D;
a=1.0/120-1.0/10;
b=30*h+m/2-6*m;
s[0][0]=solve(a,b);
s[0][1]=solve(-a,-b);
///D<=|hw-sw|<=360-D;
a=1.0/120-6;
b=30*h+m/2;
s[1][0]=solve(a,b);
s[1][1]=solve(-a,-b);
///D<=|mw-sw|<=360-D;
a=1.0/10-6;
b=6*m;
s[2][0]=solve(a,b);
s[2][1]=solve(-a,-b);
double res=0;
for (int i=0; i<2; i++){
for (int j=0; j<2; j++){
for (int k=0; k<2; k++){
ss = jiao( jiao(s[0][i],s[1][j]) , s[2][k] );
res=res+ss.r-ss.l;
}
}
}
return res;
}
int main() {
double h,m,s,sum;
while (~scanf("%lf",&D) && D!=-1){
sum=0;
for (int i=0; i<12; i++){
for (int j=0; j<60; j++){
h=i; m=j;
sum=sum+happytime(h,m);
}
}
sum = sum*100.0 / (12*60*60);
printf("%.3f\n",sum);
}
return 0;
}
开始时不会做,参考大神博客后做的题,并写此题解(侵删)。
果冻0_0 https://www.cnblogs.com/huangguodong/p/5553939.html
kuangbin的博客园https://www.cnblogs.com/kuangbin/archive/2011/12/04/2275470.html