一、Java9
1、接⼝私有⽅法
JDK8新增了静态⽅法和默认⽅法,但是不⽀持私有⽅法
JDK9中新增了私有⽅法
public interface OrderPay {
void pay();
default void defaultPay() {
privateMethod();
}
//接⼝的私有⽅法可以在JDK9中使⽤
private void privateMethod() {
System.out.println("调⽤接⼝的私有⽅法");
}
}
public class OrderPayImpl implements OrderPay {
@Override
public void pay() {
System.out.println("OrderPayImpl实现了pay()方法");
}
}
OrderPayImpl orderPay = new OrderPayImpl();
orderPay.defaultPay();
orderPay.pay();
面试题:
- 接⼝中的静态⽅法不能被实现类继承和⼦接⼝继承,但是接⼝中的⾮静态的默认⽅法可以被实现类继承
- 类的静态⽅法可以被继承
2、增强try-with-resource
1)、在JDK7中,新增了try-with-resources语句,可以在try后的括号中初始化资源,可以实现资源⾃动关闭
String path = "/Users/hanxiantao/Desktop/hello.txt";
try (OutputStream out = new FileOutputStream(path);) {
out.write("hello world".getBytes());
} catch (IOException e) {
e.printStackTrace();
}
2)、在JDK9中,改进了try-with-resources语句,在try外进⾏初始化,在括号内引⽤,即可实现资源⾃动关闭,多个变量则⽤分号进⾏分割
String path = "/Users/hanxiantao/Desktop/hello.txt";
OutputStream out = new FileOutputStream(path);
try (out) {
out.write("hello world".getBytes());
} catch (IOException e) {
e.printStackTrace();
}
3、快速创建只读集合
只读集合:集合只能读取,不能增加或者删除⾥⾯的元素
1)、JDK9之前创建只读集合
List<String> list = new ArrayList<>();
list.add("SpringBoot");
list.add("SpringCloud");
list.add("Redis");
//设置为只读list
list = Collections.unmodifiableList(list);
System.out.println(list);
list.add("Docker");
执行add操作时会抛出java.lang.UnsupportedOperationException
的异常
其他方法:
unmodifiableSet()
:设置为只读set
unmodifiableMap()
:设置为只读map
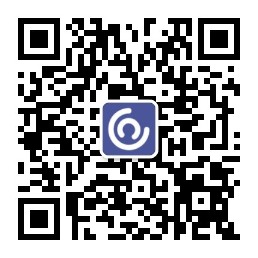
2)、JDK9创建只读集合
List<String> list = List.of("SpringBoot", "SpringCloud", "Redis");
System.out.println(list);
Set<String> set = Set.of("SpringBoot", "SpringCloud", "Redis");
System.out.println(set);
Map<String, String> map = Map.of("key1", "SpringBoot", "key2", "SpringCloud");
System.out.println(map);
4、新增的Stream API
takeWhile()
方法:从Stream中获取⼀部分数据,返回从头开始的尽可能多的元素,直到遇到第⼀个false结果(不包含该元素),如果第⼀个值不满⾜断⾔条件,将返回⼀个空的Stream
List<String> list = List.of("SpringBoot", "", "SpringCloud", "Redis").stream()
.takeWhile(obj -> !obj.isEmpty()).collect(Collectors.toList());
System.out.println(list);
执行结果:
[SpringBoot]
dropWhile()
方法:与takeWhile()
相反,返回第一个false结果和其之后的元素,和takeWhile()
⽅法形成互补
List<String> list = List.of("SpringBoot", "", "SpringCloud", "Redis").stream()
.dropWhile(obj -> !obj.isEmpty()).collect(Collectors.toList());
System.out.println(list);
执行结果:
[, SpringCloud, Redis]
二、Java10
1、局部变量类型推断var
JDK10可以使⽤var作为局部变量类型推断标识符
- 仅适⽤于局部变量,如增强for循环的索引,传统for循环局部变量
- 不能使⽤于⽅法形参、构造函数形参、⽅法返回类型或任何其他类型的变量声明
- 标识符var不是关键字,⽽是⼀个保留类型名称,⽽且不⽀持类或接⼝叫var,也不符合命名规范
var strVar = "SpringBoot";
System.out.println(strVar instanceof String);//true
//repeat(int count)方法:⽤于字符串循环输出
System.out.println(strVar.repeat(2));
var flagVar = Boolean.valueOf(true);
System.out.println(flagVar instanceof Boolean);//true
for (var i = 0; i < 10; ++i) {
System.out.println(i);
}
三、Java11
1、新增Http客户端
1)、常见类和接口
HttpClient.Builder
:HttpClient构建⼯具类
HttpRequest.Builder
:HttpRequest构建⼯具类
HttpRequest.BodyPublisher
:将Java对象转换为可发送的HTTP Request Body字节流,如form表单提交
HttpResponse.BodyHandler
:处理接收到的Response Body
2)、创建HttpClient
HttpClient httpClient = HttpClient.newBuilder().build();
或者:
HttpClient httpClient = HttpClient.newHttpClient();
3)、创建Get请求
URI uri = URI.create("http://localhost:8080/api/hello");
//设置建立连接的超时时间5秒
var httpClient = HttpClient.newBuilder().connectTimeout(Duration.ofMillis(5000)).build();
//设置读取数据超时read timeout
var httpRequest = HttpRequest.newBuilder().timeout(Duration.ofMillis(3000))
.header("key1", "v1")
.header("key2", "v2")
.uri(uri).build();
try {
HttpResponse<String> response = httpClient.send(httpRequest, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
} catch (Exception e) {
e.printStackTrace();
}
4)、提交Post请求
@Test
public void testPost() {
URI uri = URI.create("http://localhost:8080/api/insertStudent");
//设置建立连接的超时时间5秒
var httpClient = HttpClient.newBuilder().connectTimeout(Duration.ofMillis(5000)).build();
Student student = new Student("小明", 13);
//使用的是fastjson
//设置读取数据超时read timeout
var httpRequest = HttpRequest.newBuilder().timeout(Duration.ofMillis(3000))
.uri(uri)
.header("Content-Type", "application/json")
.POST(HttpRequest.BodyPublishers.ofString(JSONObject.toJSONString(student)))
.build();
try {
HttpResponse<String> response = httpClient.send(httpRequest, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
JSONObject jsonObject = JSONObject.parseObject(response.body());
Student result = JSONObject.toJavaObject(jsonObject, Student.class);
System.out.println(result);
} catch (Exception e) {
e.printStackTrace();
}
}
5)、提交异步请求
@Test
public void testAsync() {
URI uri = URI.create("http://localhost:8080/api/insertStudent");
//设置建立连接的超时时间5秒
var httpClient = HttpClient.newBuilder().connectTimeout(Duration.ofMillis(5000)).build();
Student student = new Student("小明", 13);
//使用的是fastjson
//设置读取数据超时read timeout
var httpRequest = HttpRequest.newBuilder().timeout(Duration.ofMillis(3000))
.uri(uri)
.header("Content-Type", "application/json")
.POST(HttpRequest.BodyPublishers.ofString(JSONObject.toJSONString(student)))
.build();
try {
CompletableFuture<String> response = httpClient.sendAsync(httpRequest, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body);
System.out.println(response.get());
JSONObject jsonObject = JSONObject.parseObject(response.get());
Student result = JSONObject.toJavaObject(jsonObject, Student.class);
System.out.println(result);
} catch (Exception e) {
e.printStackTrace();
}
}
6)、提交HTTP2请求
URI uri = URI.create("https://http2.akamai.com/demo");
//设置建立连接的超时时间5秒
var httpClient = HttpClient.newBuilder()
.connectTimeout(Duration.ofMillis(5000))
//指定HTTP2
.version(HttpClient.Version.HTTP_2)
.build();
//设置读取数据超时read timeout
var httpRequest = HttpRequest.newBuilder().timeout(Duration.ofMillis(3000))
.uri(uri)
.build();
try {
HttpResponse<String> response = httpClient.send(httpRequest, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
System.out.println(response.version());
} catch (Exception e) {
e.printStackTrace();
}
2、javac和java命令优化
JDK11前运⾏java程序:
编译:javac xxx.java
运⾏:java xxx
JDK11后运⾏java程序(本地不会⽣成class⽂件):
java xxx.java