Description
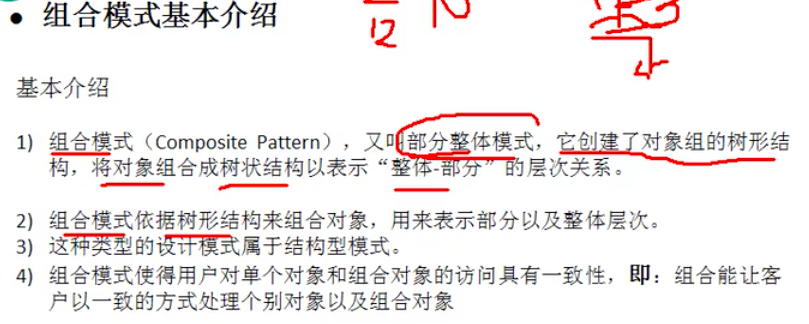
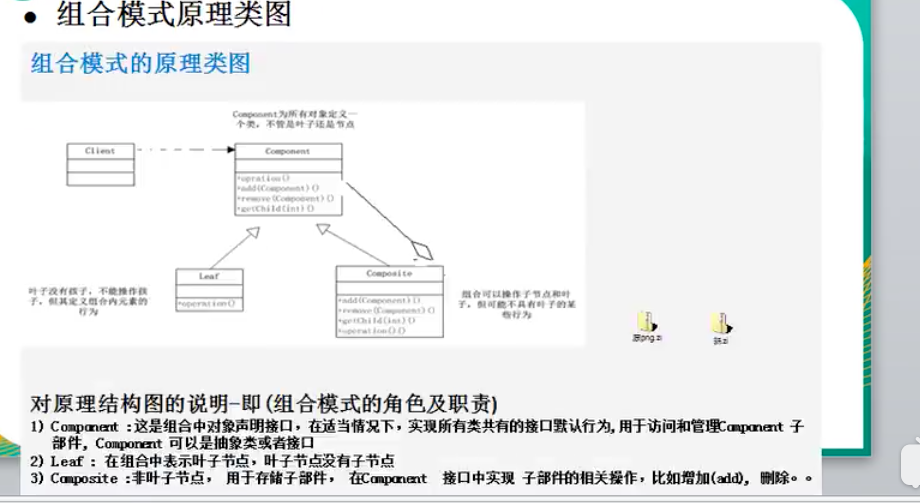
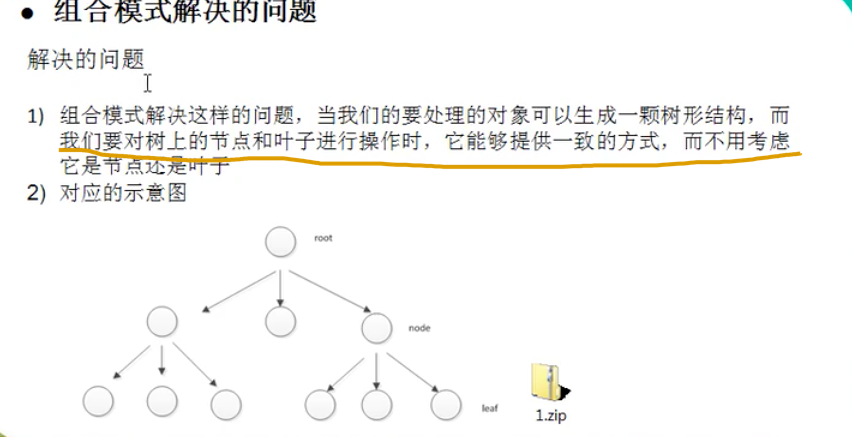
院校问题

//OrganizationComponent.java
public abstract class OrganizationComponent {
private String name;
private String description;
protected void add(OrganizationComponent organizationComponent){
//default
throw new UnsupportedOperationException();
}
protected void remove(OrganizationComponent organizationComponent){
//default
throw new UnsupportedOperationException();
}
public OrganizationComponent(String name, String description) {
this.name = name;
this.description = description;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
protected abstract void print();
}
//University.java
import java.util.ArrayList;
import java.util.List;
public class University extends OrganizationComponent {
// List中存放的是College
List<OrganizationComponent> organizationComponents = new ArrayList<OrganizationComponent>();
public University(String name, String description){
super(name, description);
}
@Override
protected void add(OrganizationComponent organizationComponent) {
organizationComponents.add(organizationComponent);
}
@Override
protected void remove(OrganizationComponent organizationComponent) {
organizationComponents.remove(organizationComponent);
}
@Override
public String getName() {
return super.getName();
}
@Override
public String getDescription() {
return super.getDescription();
}
@Override
protected void print() {
System.out.println("----------" + getName() + "------------");
for(OrganizationComponent list : organizationComponents){
list.print();
}
}
}
//College.java
import java.util.ArrayList;
import java.util.List;
public class College extends OrganizationComponent{
// List中存放的是 Department
List<OrganizationComponent> organizationComponents = new ArrayList<OrganizationComponent>();
public College(String name, String description){
super(name, description);
}
@Override
protected void add(OrganizationComponent organizationComponent) {
organizationComponents.add(organizationComponent);
}
@Override
protected void remove(OrganizationComponent organizationComponent) {
organizationComponents.remove(organizationComponent);
}
@Override
public String getName() {
return super.getName();
}
@Override
public String getDescription() {
return super.getDescription();
}
@Override
protected void print() {
System.out.println("----------" + getName() + "------------");
for(OrganizationComponent list : organizationComponents){
list.print();
}
}
}
// Department.java
public class Department extends OrganizationComponent{
public Department(String name, String description){
super(name, description);
}
@Override
public String getName() {
return super.getName();
}
@Override
public String getDescription() {
return super.getDescription();
}
@Override
protected void print() {
System.out.println(getName());
}
}
// Client.java
public class Client {
public static void main(String[] args) {
OrganizationComponent university = new University("Yale","Jerry");
OrganizationComponent college1 = new College("CS", "Coding");
OrganizationComponent college2 = new College("Pi", "Thinking");
college1.add(new Department("SE","TOM"));
college1.add(new Department("HU", "RI"));
college1.add(new Department("TA","NR"));
college2.add(new Department("TT", "JJ"));
college2.add(new Department("FF", "EE"));
college2.add(new Department("UU", "II"));
university.add(college1);
university.add(college2);
university.print();
college1.print();
college2.print();
}
// The output
// ----------Yale------------
// ----------CS------------
// SE
// HU
// TA
//----------Pi------------
// TT
// FF
// UU
//----------CS------------
// SE
// HU
// TA
//----------Pi------------
// TT
// FF
// UU
}
Use in JDK
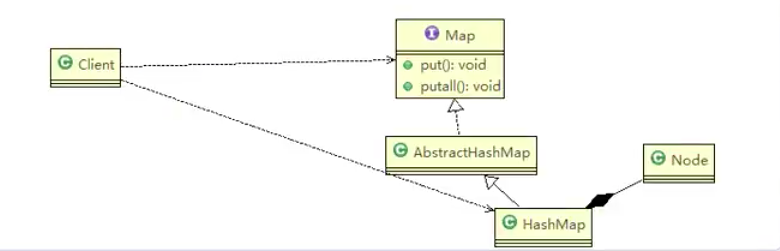
Attention
