2018级《算法分析与设计》练习5(java)
问题 A: 选房子
题目描述
栋栋和李剑已经大四了,想要出去找房子住。他们一共看中了n套房子。其中第i套房子已经住了ai个人了,它最多能住bi个人。栋栋和李剑想要住在一起,那么请问他们有几套可以选择的房子?
输入
输入的第一行为一个正整数T (T<=1000),代表一共有T组测试数据。
每组测试数据的第一行有一个正整数n (1<=n<=100),代表一共有n套房子。接下来n行,每行有两个正整数ai,bi (1<=ai<=bi<=100),分别代表现在已经住了ai个人和最多能住bi个人。
输出
对于每组测试数据,输出一行包含一个整数,代表他们可以选择房子的数量。
样例输入 Copy
2
2
1 2
1 3
3
1 10
2 10
3 10
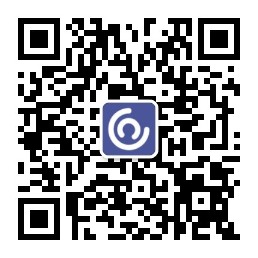
样例输出 Copy
1
3
import java.util.Scanner;
public class Main
{
public static void main(String[] args){
Scanner cin=new Scanner(System.in);
int T=cin.nextInt();
while(T>0) {
int count=0;
int n=cin.nextInt();
int a[][]=new int[n][2];
for(int i=0;i<n;i++) {
for(int j=0;j<2;j++) {
a[i][j]=cin.nextInt();
}
}
for(int i=0;i<n;i++) {
if(a[i][1]-a[i][0]>=2) {
count++;
}
}
System.out.println(count);
T--;
}
}
}
问题 B: 神秘的数字
题目描述
又一个神秘的数字,它是一个4位数,该4位数的千位上的数字和百位上的数字都被擦掉了,知道十位上的数字是1、个位上的数字是2,
又知道这个数字减去7就能被7整除,减去8就能被8整除,减去9就能被9整除。
输入
无
输出
输出这个数
import java.util.Scanner;
public class Main
{
public static void main(String[] args){
Scanner cin=new Scanner(System.in);
for(int a = 1; a <= 9; a++)
for(int b =0; b <= 9; b++){
int num = a * 1000 + b * 100 + 10 + 2;
if((num - 7) % 7 == 0 && (num - 8) % 8 == 0 && (num - 9) % 9 == 0){
System.out.println(num);
break;
}
}
}}
问题 C: 随机数
题目描述
有一个rand(n)的函数,它的作用是产生一个在[0,n)的随机整数。现在有另外一个函数,它的代码如下:
int random(int n, int m)
{
return rand(n)+m;
}
显而易见的是函数random(n,m)可以产生任意范围的随机数。现在问题来了,如果我想要产生范围在[a,b)内的一个随机数,那么对应的n,m分别为多少?
输入
输入的第一行为一个正整数T (T<=1000),表示一共有T组测试数据。
对于每组测试数据包含两个整数a,b (a<=b)。
输出
对于每组测试数据,输出一行包含两个整数n和m,两个整数中间有一个空格分隔。
样例输入 Copy
2
0 5
1 4
样例输出 Copy
5 0
3 1
import java.util.Scanner;
public class Main
{
public static void main(String[] args){
Scanner cin=new Scanner(System.in);
int n;
n=cin.nextInt();
while(n>0){
while(n>0){
int a,b;
a=cin.nextInt();
b=cin.nextInt();
int x,y;
x=b-a;
y=a;
System.out.println(x+" "+y);
n--;
}
}
}}
问题 D: 快速排序
题目描述
编程实现快速排序算法,深入理解快速排序算法的基本思想。
输入
多组输入,每组第一个数字为数组长度,然后输入一个一维整型数组。
输出
输出快速排序之后的一维整型数组(升序)
样例输入 Copy
6 1 8 6 5 3 4
5 12 42 2 5 8
样例输出 Copy
1 3 4 5 6 8
2 5 8 12 42
import java.util.*;
public class Main {
static void swap(int a[],int i,int j){
int temp=a[i];
a[i]=a[j];
a[j]=temp;
}
//分区函数
static int partion(int a[],int p,int q){
int x=a[p];
int i=p,j;
for(j=p+1;j<=q;j++){
if(a[j]<=x){
i++;
swap(a,i,j);
}
}
swap(a,p,i);
return i;
}
static void quckSort(int a[],int p,int q){
if(p<q){
int r=partion(a,p,q);
quckSort(a,p,r-1);
quckSort(a,r+1,q);
}
}
public static void main(String[] args) {
Scanner cin = new Scanner(System.in);
while(cin.hasNext()){
int n=cin.nextInt();
int arr[]=new int[n];
for(int i=0;i<arr.length;i++){
arr[i]=cin.nextInt();
}
quckSort(arr,0,arr.length-1);
for(int i=0;i<arr.length;i++){
System.out.print(arr[i]+" ");
}
System.out.println();
}
}
}
问题 E: 随机化快速排序
题目描述
使用Java或C++等语言中内置的随机函数实现随机化快速排序,在数组中随机选择一个元素作为分区的主元(Pivot)。
输入
多组样例输入,每组由一个一维整型数组组成。
输出
随机化快速排序之后的一维整型数组(升序排列)。
样例输入 Copy
6 1 8 6 5 3 4
5 12 42 2 5 8
样例输出 Copy
1 3 4 5 6 8
2 5 8 12 42
import java.util.*;
public class Main {
static void swap(int a[],int i,int j){
int temp=a[i];
a[i]=a[j];
a[j]=temp;
}
//分区函数
static int partion(int a[],int p,int q){
int x=a[p];
int i=p,j;
for(j=p+1;j<=q;j++){
if(a[j]<=x){
i++;
swap(a,i,j);
}
}
swap(a,p,i);
return i;
}
static void quckSort(int a[],int p,int q){
if(p<q){
int k=(int) ((Math.random()% (q-p+1))+ q);
//随机产出一个[p,q]之间的随机数
swap(a,k,p);//与p进行交换
int r=partion(a,p,q);
quckSort(a,p,r-1);
quckSort(a,r+1,q);
}
}
public static void main(String[] args) {
Scanner cin = new Scanner(System.in);
while(cin.hasNext()){
int n=cin.nextInt();
int arr[]=new int[n];
for(int i=0;i<arr.length;i++){
arr[i]=cin.nextInt();
}
quckSort(arr,0,arr.length-1);
for(int i=0;i<arr.length;i++){
System.out.print(arr[i]+" ");
}
System.out.println();
}
}
}
问题 F: 数组合并
题目描述
编写一个程序,将两个有序数组合并成一个更大的有序数组,要求时间复杂度为O(n)。
输入
多组数据输入,每组输入包括两行,每行第一个数字为数组长度n,然后输入n个有序整数。
输出
输出合并后的数组(升序),每组输出用一个空行隔开。
样例输入 Copy
3 1 3 5
3 2 4 6
2 1 2
4 3 4 5 6
样例输出 Copy
1 2 3 4 5 6
1 2 3 4 5 6
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
public class Main
{
public static void main(String[] args){
Scanner cin=new Scanner(System.in);
while(cin.hasNext()) {
//输入
int n1=cin.nextInt();
int a1[]=new int[n1];
for(int i=0;i<n1;i++) {
a1[i]=cin.nextInt();
}
int n2=cin.nextInt();
int a2[]=new int[n2];
for(int i=0;i<n2;i++) {
a2[i]=cin.nextInt();
}
//定义一个数组,用于存放排序后的结果
int arr[]=new int[n1+n2];
//对两个数组进行排序
Arrays.sort(a1);
Arrays.sort(a2);
int i = 0,j = 0;int q=0;
//如果两个数组均还有数
while(i<n1&&j<n2) {
if(a1[i]<a2[j]) {
arr[q]=a1[i];
i++;
q++;
}else {
arr[q]=a2[j];
j++;
q++;
}
}
//对于a1判断完的情况
while(j<n2) {
arr[q]=a2[j];
j++;
q++;
}
//对于a2判断完的情况
while(i<n1) {
arr[q]=a2[i];
i++;
q++;
}
for( i=0;i<arr.length;i++) {
System.out.print(arr[i]+" ");
if(i==arr.length-1) {System.out.println();}
}
System.out.println();
}
}
}
问题 G: 归并排序
题目描述
编写一个程序,使用分治策略实现二路归并排序(升序)。
输入
多组输入,每组第一个数字为数组长度,然后输入一个一维整型数组。
输出
输出排序之后(升序)的一维整型数组,每组输出占一行。
样例输入 Copy
6 1 8 6 5 3 4
5 12 42 2 5 8
样例输出 Copy
1 3 4 5 6 8
2 5 8 12 42
import java.util.Scanner;
public class Main {
static void Merge (int SR[ ], int TR[ ], int s, int m, int t )
{
int i=s;int j=m+1;int k=s;
while(i<=m&&j<=t){
if (SR[i]<=SR[j])
{
TR[k++]=SR[i++];
}
else
{
TR[k++]=SR[j++];
}
}
while (i<=m)
{
TR[k++]=SR[i++];
}
while (j<=t)
{
TR[k++]=SR[j++];
}
}
static void Meragesort(int SR[],int TR[],int s,int t){
if(s<t){
int m=(s+t)/2;
Meragesort(SR,TR,s,m);
Meragesort(SR,TR,m+1,t);
Merge(SR,TR,s,m,t);
}
}
static void MergeSort(int SR[],int TR[], int s, int t ) {
if (s < t) {
int m = (s+t)/2;
MergeSort(SR,TR, s, m);
MergeSort(SR,TR, m+1, t);
Merge(SR, TR, s, m, t);
for(int i=s;i<=t;i++)
{
SR[i] = TR[i];
}
}
}
public static void main(String[] args) {
Scanner cin=new Scanner(System.in);
while(cin.hasNext())
{
int n=cin.nextInt();
int arr[]=new int[n];
for(int i=0;i<n;i++)
{
arr[i]=cin.nextInt();
}
int[] r=new int[n];
MergeSort(arr, r, 0, n-1);
for(int j=0;j<n;j++)
{
System.out.print(r[j]+" ");
}
System.out.println();
}
}
}
问题 H: Strange fuction
题目描述
Now, here is a fuction:
F(x) = 6 * x7+8*x6+7x3+5*x2-yx (0 <= x <=100)
Can you find the minimum value when x is between 0 and 100.
输入
The first line of the input contains an integer T(1<=T<=100) which means the number of test cases. Then T lines follow, each line has only one real numbers Y.(0 < Y <1e10)
输出
Just the minimum value (accurate up to 4 decimal places),when x is between 0 and 100.
样例输入 Copy
2
100
200
样例输出 Copy
-74.4291
-178.8534
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
public class Main
{
static double y;
public static double calc(double x) {
return 6 * Math.pow(x, 7) + 8 *Math. pow(x, 6) + 7 *Math. pow(x, 3) + 5 * x * x - y * x;
}
public static void main(String[] args){
Scanner cin=new Scanner(System.in);
int T=cin.nextInt();
while(T>0) {
y=cin.nextDouble();
double l = 0, r = 100;
double midl = 0, midr;
while (r - l > 1e-8) {
midl = (l + r) / 2;
midr = (midl + r) / 2;
double cmidl = calc(midl);
double cmidr = calc(midr);
if (cmidl < cmidr) {
r = midr;
}
else l = midl;
}
System.out.println(String.format("%.4f",calc(midl)));
T--;
}
}
}
问题 I: Who’s in the Middle
题目描述
FJ is surveying his herd to find the most average cow. He wants to know how much milk this ‘median’ cow gives: half of the cows give as much or more than the median; half give as much or less.
Given an odd number of cows N (1 <= N < 10,000) and their milk output (1…1,000,000), find the median amount of milk given such that at least half the cows give the same amount of milk or more and at least half give the same or less.
输入
-
Line 1: A single integer N
-
Lines 2…N+1: Each line contains a single integer that is the milk output of one cow.
输出
- Line 1: A single integer that is the median milk output.
样例输入 Copy
5
2
4
1
3
5
样例输出 Copy
3
提示
INPUT DETAILS:
Five cows with milk outputs of 1…5
OUTPUT DETAILS:
1 and 2 are below 3; 4 and 5 are above 3.
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
public class Main
{
static double y;
public static double calc(double x) {
return 6 * Math.pow(x, 7) + 8 *Math. pow(x, 6) + 7 *Math. pow(x, 3) + 5 * x * x - y * x;
}
public static void main(String[] args){
Scanner cin=new Scanner(System.in);
while(cin.hasNext()) {
int n=cin.nextInt();
int arr[]=new int[n];
if(n%2!=0) {
for(int i=0;i<arr.length;i++) {
arr[i]=cin.nextInt();
}
Arrays.sort(arr);
System.out.println(arr[arr.length/2]);
}
}
}
}
问题 J: 第k大元素问题
题目描述
输入n个整数和一个正整数k(1<=k<=n),输出这些整数从大到小排序后的第k个。(要求时间复杂度为O(n),需使用随机化分区) 。
输入
多组数据输入,每组第一个数字为数组的长度n, 然后接下输入n个整数,最后输入整数k(1<=k<=n)。
输出
输出数组降序排序后的第k个整数。
样例输入 Copy
5 1 5 2 4 3 3
6 1 2 3 4 5 6 1
样例输出 Copy
3
6
import java.util.Random;
import java.util.Scanner;
public class Main
{
//交换函数
public static void change(int a[],int p,int q) {
int t;
t=a[p];
a[p]=a[q];
a[q]=t;
}
//分区函数
public static int partition(int a[],int p,int q) {
int x=a[p];
int i=p,j;
for(j=p+1;j<=q;j++) {
if(a[j]>x) {
i++;
change(a,i,j);
}
}
change(a,p,i);
return i;
}
//随机生成[p,q]的随机函数
int randomswap(int a[],int p,int q)
{
int k=(int) ((Math.random()% (q-p+1))+ q);
change(a,k,p);
return partition(a,p,q);
}
public static int quickSelect(int a[],int s,int t,int k){
if(s==t)return a[s];
int i=partition(a,s,t);
int j=i-s+1;//比a[i]大的个数
if(k<=j)return quickSelect(a,s,i,k);
else return quickSelect(a,i+1,t,k);
}
public static void main(String[] args){
Scanner cin=new Scanner(System.in);
while(cin.hasNext()) {
int n=cin.nextInt();
int a[]=new int[n];
for(int i=0;i<a.length;i++) {
a[i]=cin.nextInt();
}
int k=cin.nextInt();
System.out.println( quickSelect(a,0,a.length-1,k));
}
}
}