contains方法
package com.lichennan.collection;
import java.util.ArrayList;
import java.util.Collection;
public class CollectionTest04 {
public static void main(String[] args) {
Collection c = new ArrayList();
String s1 = new String("abc");
c.add(s1);
String s2 = new String("def");
c.add(s2);
System.out.println("元素的个数是:"+c.size());
String x = new String("abc");
System.out.println(c.contains(x));
}
}
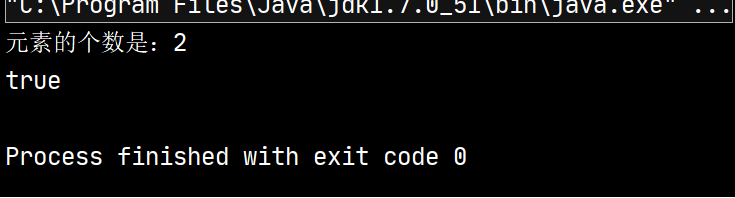
remove方法
package com.lichennan.collection;
import java.util.ArrayList;
import java.util.Collection;
public class CollectionTest05 {
public static void main(String[] args) {
Collection cc = new ArrayList();
String s1 = new String("hello");
cc.add(s1);
String s2 = new String("welcome");
cc.add(s2);
String s5 = new String("welcome");
String s3 = new String("goodbye");
cc.add(s3);
System.out.println(cc.size());
cc.remove(s5);
System.out.println(cc.size());
}
}
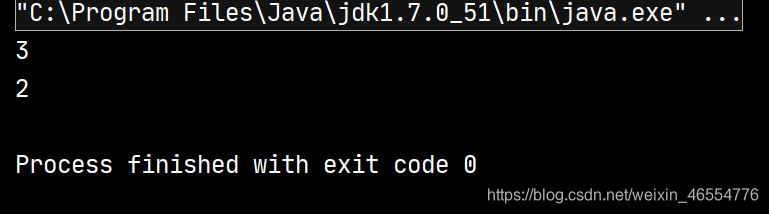
关于集合中元素的删除
package com.lichennan.collection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
public class CollectionTest06 {
public static void main(String[] args) {
Collection c = new ArrayList();
c.add(1);
c.add(2);
c.add(3);
Iterator it = c.iterator();
while (it.hasNext()){
Object obj =it.next();
System.out.println(obj);
}
System.out.println("==============");
Collection c2 = new ArrayList();
c2.add("abc");
c2.add("def");
c2.add("xyz");
Iterator it2 = c2.iterator();
while (it2.hasNext()){
Object o = it2.next();
it2.remove();
System.out.println(o);
}
System.out.println(c2.size());
}
}
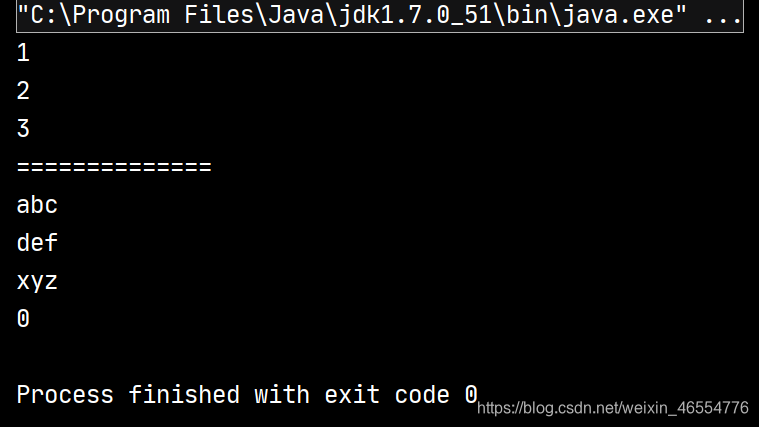