react+redux仿微信聊天IM实战|react仿微信界面|react多人群聊天室
最近一直捣鼓react开发,就运用react开发了个仿微信聊天室reactChatRoom项目,基于react+react-dom+react-router-dom+redux+react-redux+webpack2.0+antdesign+wcPop等技术混合开发,实现了聊天记录界面下拉刷新、发送消息、表情(动图),图片、视频预览,打赏、红包等功能。
vue聊天室:https://blog.csdn.net/yanxinyun1990/article/details/89038427
◆ react入口页面index.js 及package.json依赖注入
/*
* @desc 入口页面index.js
*/
import React from 'react';
import ReactDOM from 'react-dom';
// import {HashRouter as Router, Route} from 'react-router-dom'
import App from './App';
// 引入状态管理
import {Provider} from 'react-redux'
import {store} from './store'
// 导入公共样式
import './assets/fonts/iconfont.css'
import './assets/css/reset.css'
import './assets/css/layout.css'
// 引入wcPop弹窗样式
import './assets/js/wcPop/skin/wcPop.css'
// 引入js
import './assets/js/fontSize'
ReactDOM.render(
<Provider store={store}>
{/* <Router>
<Route path="/" component={App} />
</Router> */}
<App />
</Provider>,
document.getElementById('app')
);
{
"name": "react-chatroom",
"version": "0.1.0",
"private": true,
"author": "andy",
"dependencies": {
"react": "^16.8.6",
"react-dom": "^16.8.6",
"react-redux": "^7.0.3",
"react-router-dom": "^5.0.0",
"react-scripts": "0.9.x",
"redux": "^4.0.1"
},
"devDependencies": {
"jquery": "^2.2.3",
"react-loadable": "^5.5.0",
"react-photoswipe": "^1.3.0",
"react-pullload": "^1.2.0",
"redux-thunk": "^2.3.0",
"swiper": "^4.5.0",
"webpack": "^1.13.1",
"webpack-dev-server": "^1.12.0"
},
"scripts": {
"start": "set HOST=localhost&&set PORT=3003 && react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test --env=jsdom",
"eject": "react-scripts eject"
}
}
◆ react登录注册验证、拦截
import React, { Component } from 'react';
import { Link } from 'react-router-dom'
import { connect } from 'react-redux';
import * as actions from '../../store/action'
// 引入wcPop弹窗插件
import { wcPop } from '../../assets/js/wcPop/wcPop.js'
class Login extends Component {
constructor(props) {
super(props)
this.state = {
tel: '',
pwd: '',
vcode: '',
vcodeText: '获取验证码',
disabled: false,
time: 0
}
}
componentDidMount(){
if(this.props.token){
this.props.history.push('/')
}
}
render() {
return (
<div className="wcim__lgregWrapper flexbox flex__direction-column">
......
</div>
)
}
// 提交表单
handleSubmit = (e) => {
e.preventDefault();
var that = this
this.state.tel = this.refs.tel.value
this.state.pwd = this.refs.pwd.value
this.state.vcode = this.refs.vcode.value
if (!this.state.tel) {
wcPop({ content: '手机号不能为空!', style: 'background:#ff3b30;color:#fff;', time: 2 });
} else if (!checkTel(this.state.tel)) {
wcPop({ content: '手机号格式不正确!', style: 'background:#ff3b30;color:#fff;', time: 2 });
} else if (!this.state.pwd) {
wcPop({ content: '密码不能为空!', style: 'background:#ff3b30;color:#fff;', time: 2 });
} else if (!this.state.vcode) {
wcPop({ content: '验证码不能为空!', style: 'background:#ff3b30;color:#fff;', time: 2 });
} else {
// 获取登录之前的页面地址
let redirectUrl = this.props.location.state ? this.props.location.state.from.pathname : '/'
// 设置token
this.props.authToken(getToken())
this.props.authUser(this.state.tel)
wcPop({
content: '注册成功!', style: 'background:#41b883;color:#fff;', time: 2,
end: function () {
that.props.history.push(redirectUrl)
}
});
}
}
// 60s倒计时
handleVcode = (e) => {
e.preventDefault();
this.state.tel = this.refs.tel.value
if (!this.state.tel) {
wcPop({ content: '手机号不能为空!', style: 'background:#ff3b30;color:#fff;', time: 2 });
} else if (!checkTel(this.state.tel)) {
wcPop({ content: '手机号格式不正确!', style: 'background:#ff3b30;color:#fff;', time: 2 });
} else {
this.state.time = 60
this.state.disabled = true
this.countDown();
}
}
countDown = (e) => {
if(this.state.time > 0){
this.state.time--
this.setState({
vcodeText: '获取验证码(' + this.state.time + ')'
})
// setTimeout(this.countDown, 1000);
setTimeout(() => {
this.countDown()
}, 1000);
}else{
this.setState({
time: 0,
vcodeText: '获取验证码',
disabled: false
})
}
}
}
const mapStateToProps = (state) => {
return {
...state.auth
}
}
export default connect(mapStateToProps, {
authToken: actions.setToken,
authUser: actions.setUser
})(Login)
constructor(props){
super(props)
console.log('群聊页面参数:\n' + JSON.stringify(props, null, 2))
}
// ******react-photoswipe配置选项
state = {
images: [
{
// src: 'http://lorempixel.com/1200/900/sports/1',
src: require('../../assets/img/placeholder/wchat__img03.jpg'),
w: 345,
h: 700,
// title: 'Image 1'
},
{
// src: 'http://lorempixel.com/1200/900/sports/2',
src: require('../../assets/img/placeholder/wchat__img01.jpg'),
w: 405,
h: 275,
// title: 'Image 2'
},
{
src: require('../../assets/img/placeholder/wchat__img02.jpg'),
w: 865,
h: 265,
}
],
isOpen: false,
// 配置参数
options: {
// index: 1, //显示图片索引
loop: false, //循环显示
fullscreenEl: false, //全屏按钮
shareEl: false, //分享按钮
arrowEl: false, //左右箭头
captionEl: false, //标题描述
}
}
photoSwipeClose = () => {
this.setState({
isOpen: false
})
}
photoSwipeOpen(index){
this.setState({
isOpen: true
})
this.state.options.index = index
}
◆ react地址路由管理
/*
* @desc 页面地址路由js
*/
// 引入页面组件
import Login from '../views/auth/login'
import Register from '../views/auth/register'
import Index from '../views/index'
import Contact from '../views/contact'
import Uinfo from '../views/contact/uinfo'
import Ucenter from '../views/ucenter'
import Wallet from '../views/wallet'
import GroupChat from '../views/chat/group-chat'
import SingleChat from '../views/chat/single-chat'
import GroupInfo from '../views/chat/group-info'
// export default {Index, Contact, Ucenter};
export default [
// 登录、注册
{
path: '/login', name: 'Login', component: Login,
},
{
path: '/register', name: 'Register', component: Register,
},
// 首页、联系人、我
{
path: '/index', name: 'App', component: Index,
meta: { showHeader: true, showTabBar: true, requireAuth: true },
},
{
path: '/contact', name: 'Contact', component: Contact,
meta: { showHeader: true, showTabBar: true, requireAuth: true },
},
{
path: '/contact/uinfo', name: 'Uinfo', component: Uinfo,
},
{
path: '/ucenter', name: 'Ucenter', component: Ucenter,
meta: { showHeader: true, showTabBar: true, requireAuth: true },
},
// 聊天页面
{
path: '/chat/group-chat', name: 'GroupChat', component: GroupChat,
meta: { requireAuth: true },
},
{
path: '/chat/single-chat', name: 'SingleChat', component: SingleChat,
meta: { requireAuth: true },
},
{
path: '/chat/group-info', name: 'GroupInfo', component: GroupInfo,
meta: { requireAuth: true },
},
// 钱包
{
path: '/wallet', name: 'Wallet', component: Wallet,
meta: { requireAuth: true },
}
// ...
]
欢迎关注我的技术博客:https://www.cnblogs.com/xiaoyan2017
一起学习,一起进步,有问题随时联系,一起解决!!!
扫描二维码关注公众号,回复:
11189184 查看本文章
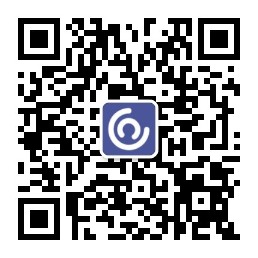