文章目录
三、 Python 语法基础
学过其他语言如 JAVA、C++等很容易理解 python,这里只总结了它的特点。
- 使用缩进整理代码「其他语言用括号」。冒号代表缩进的开始,结尾不需要分号。分号可用来给同一行语句切分。
for x in array:
if x < 10:
less.append(x)
else:
greater.append(x)
a = 5; b = 6; c = 7
- 万物皆对象「包括数字,字符串,数据结构,函数,类,模块等」。每个对象有类型和内部数据。
- 注释用 #
- 变量和参数传递。
赋值只是把名字传递给一个对象;将对象作为参数传递给函数时,新的局域变量创建了对原始对象的引用,而不是复制,所以原始对象会变。
a = [1,2,3]
b=a
a.append(4)
print(b)
# [1,2,3,4]
def app(some_list, element):
some_list.append(element)
data = [1,2,3]
app(data, 4)
print(data)
# [1,2,3,4]
- 对象的类型。用 isinstance 检查对象是某个类型的实例。
a = 5
isinstance(a, int)
# True
- 对象的属性。用 obj.attribute_name 或 getattr 访问属性和方法
a = 'foo'
a.<Press Tab>
getattr(a, 'split')
- 鸭子类型。不关心对象类型,只关心对象方法和属性。如判断一个对象是否迭代,看它是否有 _iter_ 魔术方法,或 用 iter() 函数。
def isiterable(obj):
try:
iter(obj)
return True
except TypeError: # not iterrable
return False
isiterable(''a string) # True
isiterable([1,2,3]) # True
isiterable(5) # False
- 引入模块。模块是一个有 .py 扩展名、包含 python 代码的文件。
假设有模块 a.py:
# a.py
PI = 3.19
def f(x):
return x + 2
def g(a,b):
return a + b
同一目录访问 a.py 的变量和函数,用关键字 import 和 as 关键字
import a # 方法一
result = a.f(5)
from a import PI, f # 方法二
result = g(5,PI)
import a as a1 # 方法三
from a import PI as pi, g as gf
r1 = a1.f(pi)
r2 = gf(6,pi)
- 二元运算符和比较符
3 / 2 # 1.5
3 // 2 # 1
- 可变与不可变对象。list,dict,numpy数组,用户定义的类都可变,元组和字符串不可变。
- 字符串
单引号双引号都可以;换行的字符串用 ‘’’ 或 ‘’’’’’;许多 python 对象可以用 str 函数转化为字符串
a = 'a string'
b = "another sring"
c = '''
a string
with multiple lines
'''
d = 5.6
e = str(d)
字符串是一个序列的Unicode字符,因此可以像其它序列,一样处理:
s = 'python'
list(s) # ['p', 'y', 't', 'h', 'o', 'n']
s[:3] # 'pyt'
反斜杠是转义字符,意思是它备用来表示特殊字符,比如换行符\n或Unicode字
符。要写一个包含反斜杠的字符串,需要进行转义:
s = '12\\34'
print(s) # 12\34
如果字符串中包含许多反斜杠,但没有特殊字符,这样做就很麻烦。在字符串前面加一个r,表明字符就是它自身:
s = r'this\has\no\special\characters'
print(s) # 'this\\has\\no\\special\\characters'
将两个字符串合并,会产生一个新的字符串:
a = 'hello'
b = 'world'
a + b # 'hello world'
- 字节和 unicode
在Python 3及以上版本中,Unicode是一级的字符串类型;老的Python版本中,字符串都是字节,不使用Unicode编码。
val = "español"
val_utf8 = val.encode('utf-8') # 字符串编码为UTF-8
val_utf8 # b'espa\xc3\xb1ol'
val_utf8.decode('utf-8') # 已知Unicode编码,用decode解码
type(val_utf8) # bytes
虽然UTF-8编码已经变成主流,但因为历史的原因,你仍然可能碰到其它编码的数据:
val.encode('latin1') # b'espa\xf1ol'
val.encode('utf-16') # b'\xff\xfee\x00s\x00p\x00a\x00\xf1\x00o\x00l\x00'
val.encode('utf-16le') # b'e\x00s\x00p\x00a\x00\xf1\x00o\x00l\x00'
字节对象编码为unicode:盲目地将所有数据编码为Unicode是不可取的,虽然用的不多,你可以在字节文本的前面加上一个b:
扫描二维码关注公众号,回复:
11158505 查看本文章
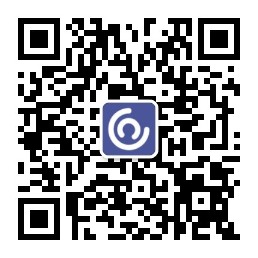
bytes_val = b'this is bytes'
bytes_val # b'this is bytes'
decoded = bytes_val.decode('utf8')
decoded # 'this is bytes'
- 类型转换。str、bool、int和float也是函数,可以用来转换类型
- None是Python的空值类型。如果一个函数没有明确的返回值,就会默认返回
None;常常作为函数的默认参数;它还是唯一的NoneType的实例
def add_and_maybe_multiply(a, b, c=None):
result = a + b
if c is not None:
result = result * c
return result
type(None) # NoneType
- 日期和时间
Python内建的datetime 模块提供了datetime 、date 和time 类型。
datetime 类型结合了date 和time ,是最常使用的。
from datetime import datetime, date, time
dt = datetime(2011, 10, 29, 20, 30, 21)
dt.day # 29
dt.minute # 30
dt.date() # datetime.date(2011, 10, 29)
dt.time() # datetime.time(20, 30, 21)
strftime:datetime->字符串,strptime:字符串->datetime
dt.strftime('%m/%d/%Y %H:%M') # '10/29/2011 20:30'
datetime.strptime('20091031', '%Y%m%d') # datetime.datetime(2009, 10, 31, 0, 0)
两个datetime对象的差会产生一个 datetime.timedelta 类型
dt2 = datetime(2011, 11, 15, 22, 30)
delta = dt2 - dt
delta # datetime.timedelta(17, 7179)
type(delta) # datetime.timedelta
将 timedelta 添加到 datetime ,会产生一个新的偏移datetime
dt # datetime.datetime(2011, 10, 29, 20, 30, 21)
dt + delta # datetime.datetime(2011, 11, 15, 22, 30)
- 控制流
if for while pass range 五种
if x < 0 :
print('It's negative')
elif x == 0:
print('Equal to zero')
elif 0 < x < 5:
print('Positive but smaller than 5')
else:
print('Positive and larger than or equal to 5')
if a < b or c > d:
print('Made it')
for
for value in collection:
# do something with value
for a, b, c in iterator:
# do something
while
while x > 0:
# do sth
pass 不需要任何动作时可以使用
if x < 0:
print('negative!')
elif x == 0:
pass
else:
print('positive!')
range 返回一个迭代器,它产生一个均匀分布的整数序列,三个参数是(起点,终点,步进)
range(10)
range(0, 20, 2)
for i in range(len(seq)):
val = seq[i]
- 三元表达式
Python中的三元表达式可以将if-else语句放到一行里,下面两者等价:
value = true-expr if condition else false-expr
if condition:
value = true-expr
else:
value = false-expr