1、输出保留位数
#include <stdio.h>
#include <iostream>
#include <iomanip>
using namespace std;
int main(){
double a;
cin>>a;
printf("%.12f",a);
}
NOI 保留输出小数点:https://blog.csdn.net/wuzhenzi5193/article/details/80295898
格式化输出3位:
printf("03d\n",n);
数据处理的时候注意0,例如三位数逆序输出,n%10判断是否为0,个位是否为0
2、字符串处理
字符串常用函数:https://blog.csdn.net/wuzhenzi5193/article/details/80489483
判断字符是否为字母、数字:
如果是数字则ch-'0'则为数字本身
#include <ctype.h>
isalpha()、isdigit()
tolower()、toupper()
字符串分割时:
c++:
使用substr()和find()函数
#include <stdio.h> #include <string> #include <iostream> using namespace std; void test1(){ char s[] = "Golden Global View,disk * desk"; const char *d = " ,*"; char *p; p = strtok(s,d); while(p) { printf("%s\n",p); p=strtok(NULL,d); } } void test2(){ string s="Golden Global View disk desk"; int position=0,position1=0; string tmp; if(s[s.size()-1]!=' ')s+=" "; while(s!=""){ position=s.find(" "); tmp=s.substr(0,position); cout<<tmp<<endl; s=s.substr(position+1,s.size()-position); } } int main() { test1(); cout<<endl; test2(); return 0; }
java:
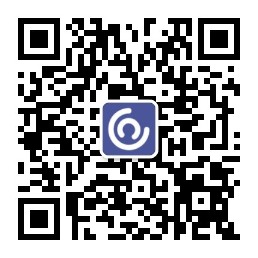
使用split()函数
String str = "1,2,3,4,5"; String[] split = str.split(","); for (int i = 0; i < split.length; i++) { System.out.println(split[i]); } String str2 = "Java string split test"; String[] strarray = str2.split(" "); for (int i = 0; i < strarray.length; i++) { System.out.println(strarray[i]); } System.out.println("//===========");
3、输入
在使用getchar()时注意换行符 \n
scanf("%d",&number)注意&符号
输入函数:https://blog.csdn.net/wuzhenzi5193/article/details/80489329
应用输入函数getline和getchar:NOI:1745 字符串判等:https://blog.csdn.net/wuzhenzi5193/article/details/80498272
NOI:1946 单词替换:https://blog.csdn.net/wuzhenzi5193/article/details/80498676
NOI:6247 过滤多余的空格:https://blog.csdn.net/wuzhenzi5193/article/details/80498761
NOI:7549 单词的长度(字符串处理):https://blog.csdn.net/wuzhenzi5193/article/details/80498876
4、舍入
四舍五入函数:https://mp.csdn.net/postedit/80489222
向0舍入可以直接使用强制类型转换:
cout<<(int)number<<endl;
或者自带的函数:
floor(x)返回的是小于或等于x的最大整数。如: floor(10.5) == 10 floor(-10.5) == -11 ceil(x)返回的是大于x的最小整数。如: ceil(10.5) == 11 ceil(-10.5) ==-10 floor()是向负无穷大舍入,floor(-10.5) == -11; ceil()是向正无穷大舍入,ceil(-10.5) == -10 floor朝负无穷方向取整,如floor(-1.3)=-2; floor(1.3)=1; ceil朝正无穷方向取整,如ceil(-1.3)=-1; ceil(1.3)=2; round四舍五入到最近的整数,如round(-1.3)=-1;round(-1.52)=-2;round(1.3)=1;round(1.52)=2
5、大整数问题
使用java和c++处理大整数问题:https://blog.csdn.net/wuzhenzi5193/article/details/80303428